print array of previous smaller elements
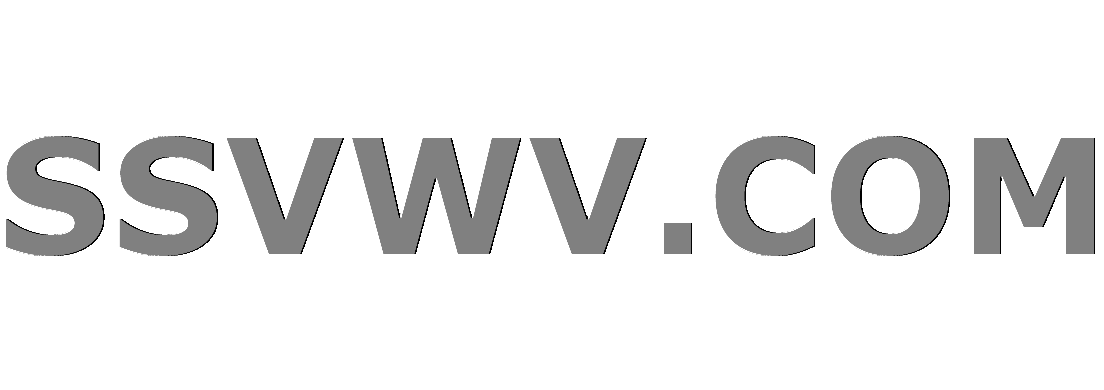
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
9
down vote
favorite
I am solving interview question from here.
Problem : Given an array, find the nearest smaller element G[i] for every element A[i] in the array such that the element has an index smaller than i.
More formally,
G[i]
for an elementA[i]
= an elementA[j]
such that
j is maximum possible
andj < i
andA[j] < A[i]
.
Elements for which no smaller element exist, consider next smaller element as-1
.
Example: Input :
A : [4, 5, 2, 10, 8]
Return :[-1, 4, -1, 2, 2]
How can I optimise my solution:
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i,-1,-1):
if arr[i]>arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34,35,27,42,5,28,39,20,28]) == [-1,34,-1,27,-1,5,28,5,20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1,4,5,6]) ==[-1,1,4,5]
python array
add a comment |Â
up vote
9
down vote
favorite
I am solving interview question from here.
Problem : Given an array, find the nearest smaller element G[i] for every element A[i] in the array such that the element has an index smaller than i.
More formally,
G[i]
for an elementA[i]
= an elementA[j]
such that
j is maximum possible
andj < i
andA[j] < A[i]
.
Elements for which no smaller element exist, consider next smaller element as-1
.
Example: Input :
A : [4, 5, 2, 10, 8]
Return :[-1, 4, -1, 2, 2]
How can I optimise my solution:
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i,-1,-1):
if arr[i]>arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34,35,27,42,5,28,39,20,28]) == [-1,34,-1,27,-1,5,28,5,20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1,4,5,6]) ==[-1,1,4,5]
python array
add a comment |Â
up vote
9
down vote
favorite
up vote
9
down vote
favorite
I am solving interview question from here.
Problem : Given an array, find the nearest smaller element G[i] for every element A[i] in the array such that the element has an index smaller than i.
More formally,
G[i]
for an elementA[i]
= an elementA[j]
such that
j is maximum possible
andj < i
andA[j] < A[i]
.
Elements for which no smaller element exist, consider next smaller element as-1
.
Example: Input :
A : [4, 5, 2, 10, 8]
Return :[-1, 4, -1, 2, 2]
How can I optimise my solution:
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i,-1,-1):
if arr[i]>arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34,35,27,42,5,28,39,20,28]) == [-1,34,-1,27,-1,5,28,5,20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1,4,5,6]) ==[-1,1,4,5]
python array
I am solving interview question from here.
Problem : Given an array, find the nearest smaller element G[i] for every element A[i] in the array such that the element has an index smaller than i.
More formally,
G[i]
for an elementA[i]
= an elementA[j]
such that
j is maximum possible
andj < i
andA[j] < A[i]
.
Elements for which no smaller element exist, consider next smaller element as-1
.
Example: Input :
A : [4, 5, 2, 10, 8]
Return :[-1, 4, -1, 2, 2]
How can I optimise my solution:
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i,-1,-1):
if arr[i]>arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34,35,27,42,5,28,39,20,28]) == [-1,34,-1,27,-1,5,28,5,20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1,4,5,6]) ==[-1,1,4,5]
python array
asked Jun 4 at 7:00
Latika Agarwal
861216
861216
add a comment |Â
add a comment |Â
3 Answers
3
active
oldest
votes
up vote
10
down vote
This can be done in a single pass over input list with a stack to keep track of previous smaller elements. Note that the stack will have a sub-sequence that is sorted at any given point.
def FastSolve(v):
res =
s = [-1]
for x in v:
while s[-1] >= x:
s.pop()
res.append(s[-1])
s.append(x)
return res
Note that this exact question has been asked before here and a very similar variation (next smaller instead of last) here - the latter question has an excellent detailed explanation of the O(n) solution and code examples.
add a comment |Â
up vote
8
down vote
Optimisation aside, there are some ways to make your code more pythonic
iterating
In Python, you almost never have to iterate over the index, so for i in range(len(arr)):
can better be expressed for i, item in enumerate(arr):
.
for j in range(i,-1,-1)
can be for item_2 in arr[i::-1]
for: .. else:
thanks to the for-else
clause, you can ditch the status
-flag
generator
Instead of the new_stack =
and new_stack.append
, you can use yield
to pass on a next item, and use list()
in the end to turn it into a list.
def prev_smaller_generator(arr):
for i, item in enumerate(arr):
for item2 in arr[i::-1]:
if item2 < item:
yield item2
break
else:
yield -1
assert list(prev_smaller_generator([34,35,27,42,5,28,39,20,28])) == [-1,34,-1,27,-1,5,28,5,20]
assert list(prev_smaller_generator([1])) == [-1]
assert list(prev_smaller_generator([1,4,5,6])) ==[-1,1,4,5]
1
Please don't usefor-else
, it's ugly and this Python feature makes porting between languages less obvious.
– KeyWeeUsr
Jun 4 at 14:48
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
add a comment |Â
up vote
5
down vote
@Alex answer solves the problem in only one pass over the data. However, you could also consider "cosmetic" improvements.
if name == main
Imagine you want to reuse this code. After writing more tests, you may not want to run the tests every time the script is loaded. The following will be run only if you call python myscript.py
if __name__ == "__main__":
# execute only if run as a script
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
PEP 8
This is a set of convention to write python code. But there are several plugins to automatically follow these guidelines (I used Autopep 8 with VIM). More info about PEP8
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i, -1, -1):
if arr[i] > arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
4
I don't like your linebreak onassert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or soprev_smaller(
new_line + indent[34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
add a comment |Â
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
10
down vote
This can be done in a single pass over input list with a stack to keep track of previous smaller elements. Note that the stack will have a sub-sequence that is sorted at any given point.
def FastSolve(v):
res =
s = [-1]
for x in v:
while s[-1] >= x:
s.pop()
res.append(s[-1])
s.append(x)
return res
Note that this exact question has been asked before here and a very similar variation (next smaller instead of last) here - the latter question has an excellent detailed explanation of the O(n) solution and code examples.
add a comment |Â
up vote
10
down vote
This can be done in a single pass over input list with a stack to keep track of previous smaller elements. Note that the stack will have a sub-sequence that is sorted at any given point.
def FastSolve(v):
res =
s = [-1]
for x in v:
while s[-1] >= x:
s.pop()
res.append(s[-1])
s.append(x)
return res
Note that this exact question has been asked before here and a very similar variation (next smaller instead of last) here - the latter question has an excellent detailed explanation of the O(n) solution and code examples.
add a comment |Â
up vote
10
down vote
up vote
10
down vote
This can be done in a single pass over input list with a stack to keep track of previous smaller elements. Note that the stack will have a sub-sequence that is sorted at any given point.
def FastSolve(v):
res =
s = [-1]
for x in v:
while s[-1] >= x:
s.pop()
res.append(s[-1])
s.append(x)
return res
Note that this exact question has been asked before here and a very similar variation (next smaller instead of last) here - the latter question has an excellent detailed explanation of the O(n) solution and code examples.
This can be done in a single pass over input list with a stack to keep track of previous smaller elements. Note that the stack will have a sub-sequence that is sorted at any given point.
def FastSolve(v):
res =
s = [-1]
for x in v:
while s[-1] >= x:
s.pop()
res.append(s[-1])
s.append(x)
return res
Note that this exact question has been asked before here and a very similar variation (next smaller instead of last) here - the latter question has an excellent detailed explanation of the O(n) solution and code examples.
edited Jun 4 at 9:01
answered Jun 4 at 7:55
Alex
1015
1015
add a comment |Â
add a comment |Â
up vote
8
down vote
Optimisation aside, there are some ways to make your code more pythonic
iterating
In Python, you almost never have to iterate over the index, so for i in range(len(arr)):
can better be expressed for i, item in enumerate(arr):
.
for j in range(i,-1,-1)
can be for item_2 in arr[i::-1]
for: .. else:
thanks to the for-else
clause, you can ditch the status
-flag
generator
Instead of the new_stack =
and new_stack.append
, you can use yield
to pass on a next item, and use list()
in the end to turn it into a list.
def prev_smaller_generator(arr):
for i, item in enumerate(arr):
for item2 in arr[i::-1]:
if item2 < item:
yield item2
break
else:
yield -1
assert list(prev_smaller_generator([34,35,27,42,5,28,39,20,28])) == [-1,34,-1,27,-1,5,28,5,20]
assert list(prev_smaller_generator([1])) == [-1]
assert list(prev_smaller_generator([1,4,5,6])) ==[-1,1,4,5]
1
Please don't usefor-else
, it's ugly and this Python feature makes porting between languages less obvious.
– KeyWeeUsr
Jun 4 at 14:48
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
add a comment |Â
up vote
8
down vote
Optimisation aside, there are some ways to make your code more pythonic
iterating
In Python, you almost never have to iterate over the index, so for i in range(len(arr)):
can better be expressed for i, item in enumerate(arr):
.
for j in range(i,-1,-1)
can be for item_2 in arr[i::-1]
for: .. else:
thanks to the for-else
clause, you can ditch the status
-flag
generator
Instead of the new_stack =
and new_stack.append
, you can use yield
to pass on a next item, and use list()
in the end to turn it into a list.
def prev_smaller_generator(arr):
for i, item in enumerate(arr):
for item2 in arr[i::-1]:
if item2 < item:
yield item2
break
else:
yield -1
assert list(prev_smaller_generator([34,35,27,42,5,28,39,20,28])) == [-1,34,-1,27,-1,5,28,5,20]
assert list(prev_smaller_generator([1])) == [-1]
assert list(prev_smaller_generator([1,4,5,6])) ==[-1,1,4,5]
1
Please don't usefor-else
, it's ugly and this Python feature makes porting between languages less obvious.
– KeyWeeUsr
Jun 4 at 14:48
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
add a comment |Â
up vote
8
down vote
up vote
8
down vote
Optimisation aside, there are some ways to make your code more pythonic
iterating
In Python, you almost never have to iterate over the index, so for i in range(len(arr)):
can better be expressed for i, item in enumerate(arr):
.
for j in range(i,-1,-1)
can be for item_2 in arr[i::-1]
for: .. else:
thanks to the for-else
clause, you can ditch the status
-flag
generator
Instead of the new_stack =
and new_stack.append
, you can use yield
to pass on a next item, and use list()
in the end to turn it into a list.
def prev_smaller_generator(arr):
for i, item in enumerate(arr):
for item2 in arr[i::-1]:
if item2 < item:
yield item2
break
else:
yield -1
assert list(prev_smaller_generator([34,35,27,42,5,28,39,20,28])) == [-1,34,-1,27,-1,5,28,5,20]
assert list(prev_smaller_generator([1])) == [-1]
assert list(prev_smaller_generator([1,4,5,6])) ==[-1,1,4,5]
Optimisation aside, there are some ways to make your code more pythonic
iterating
In Python, you almost never have to iterate over the index, so for i in range(len(arr)):
can better be expressed for i, item in enumerate(arr):
.
for j in range(i,-1,-1)
can be for item_2 in arr[i::-1]
for: .. else:
thanks to the for-else
clause, you can ditch the status
-flag
generator
Instead of the new_stack =
and new_stack.append
, you can use yield
to pass on a next item, and use list()
in the end to turn it into a list.
def prev_smaller_generator(arr):
for i, item in enumerate(arr):
for item2 in arr[i::-1]:
if item2 < item:
yield item2
break
else:
yield -1
assert list(prev_smaller_generator([34,35,27,42,5,28,39,20,28])) == [-1,34,-1,27,-1,5,28,5,20]
assert list(prev_smaller_generator([1])) == [-1]
assert list(prev_smaller_generator([1,4,5,6])) ==[-1,1,4,5]
answered Jun 4 at 8:37
Maarten Fabré
3,204214
3,204214
1
Please don't usefor-else
, it's ugly and this Python feature makes porting between languages less obvious.
– KeyWeeUsr
Jun 4 at 14:48
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
add a comment |Â
1
Please don't usefor-else
, it's ugly and this Python feature makes porting between languages less obvious.
– KeyWeeUsr
Jun 4 at 14:48
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
1
1
Please don't use
for-else
, it's ugly and this Python feature makes porting between languages less obvious.– KeyWeeUsr
Jun 4 at 14:48
Please don't use
for-else
, it's ugly and this Python feature makes porting between languages less obvious.– KeyWeeUsr
Jun 4 at 14:48
3
3
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
in cases like this, it allows for very concise code, that easily expresses what you mean
– Maarten Fabré
Jun 4 at 15:27
add a comment |Â
up vote
5
down vote
@Alex answer solves the problem in only one pass over the data. However, you could also consider "cosmetic" improvements.
if name == main
Imagine you want to reuse this code. After writing more tests, you may not want to run the tests every time the script is loaded. The following will be run only if you call python myscript.py
if __name__ == "__main__":
# execute only if run as a script
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
PEP 8
This is a set of convention to write python code. But there are several plugins to automatically follow these guidelines (I used Autopep 8 with VIM). More info about PEP8
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i, -1, -1):
if arr[i] > arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
4
I don't like your linebreak onassert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or soprev_smaller(
new_line + indent[34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
add a comment |Â
up vote
5
down vote
@Alex answer solves the problem in only one pass over the data. However, you could also consider "cosmetic" improvements.
if name == main
Imagine you want to reuse this code. After writing more tests, you may not want to run the tests every time the script is loaded. The following will be run only if you call python myscript.py
if __name__ == "__main__":
# execute only if run as a script
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
PEP 8
This is a set of convention to write python code. But there are several plugins to automatically follow these guidelines (I used Autopep 8 with VIM). More info about PEP8
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i, -1, -1):
if arr[i] > arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
4
I don't like your linebreak onassert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or soprev_smaller(
new_line + indent[34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
add a comment |Â
up vote
5
down vote
up vote
5
down vote
@Alex answer solves the problem in only one pass over the data. However, you could also consider "cosmetic" improvements.
if name == main
Imagine you want to reuse this code. After writing more tests, you may not want to run the tests every time the script is loaded. The following will be run only if you call python myscript.py
if __name__ == "__main__":
# execute only if run as a script
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
PEP 8
This is a set of convention to write python code. But there are several plugins to automatically follow these guidelines (I used Autopep 8 with VIM). More info about PEP8
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i, -1, -1):
if arr[i] > arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
@Alex answer solves the problem in only one pass over the data. However, you could also consider "cosmetic" improvements.
if name == main
Imagine you want to reuse this code. After writing more tests, you may not want to run the tests every time the script is loaded. The following will be run only if you call python myscript.py
if __name__ == "__main__":
# execute only if run as a script
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
PEP 8
This is a set of convention to write python code. But there are several plugins to automatically follow these guidelines (I used Autopep 8 with VIM). More info about PEP8
def prev_smaller(arr):
new_stack =
status = False
for i in range(len(arr)):
for j in range(i, -1, -1):
if arr[i] > arr[j]:
status = True
new_stack.append(arr[j])
break
if not status:
new_stack.append(-1)
status = False
return new_stack
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
assert prev_smaller([1]) == [-1]
assert prev_smaller([1, 4, 5, 6]) == [-1, 1, 4, 5]
answered Jun 4 at 9:37


RUser4512
541217
541217
4
I don't like your linebreak onassert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or soprev_smaller(
new_line + indent[34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
add a comment |Â
4
I don't like your linebreak onassert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or soprev_smaller(
new_line + indent[34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
4
4
I don't like your linebreak on
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line ) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or so prev_smaller(
new_line + indent [34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line ) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
I don't like your linebreak on
assert prev_smaller([34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line ) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
. Personally, I would either replace the long array with a variable, or so prev_smaller(
new_line + indent [34, 35, 27, 42, 5, 28, 39, 20, 28]
new_line ) == [-1, 34, -1, 27, -1, 5, 28, 5, 20]
– Maarten Fabré
Jun 4 at 9:41
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f195787%2fprint-array-of-previous-smaller-elements%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password