Array-based unordered list implementation in Java
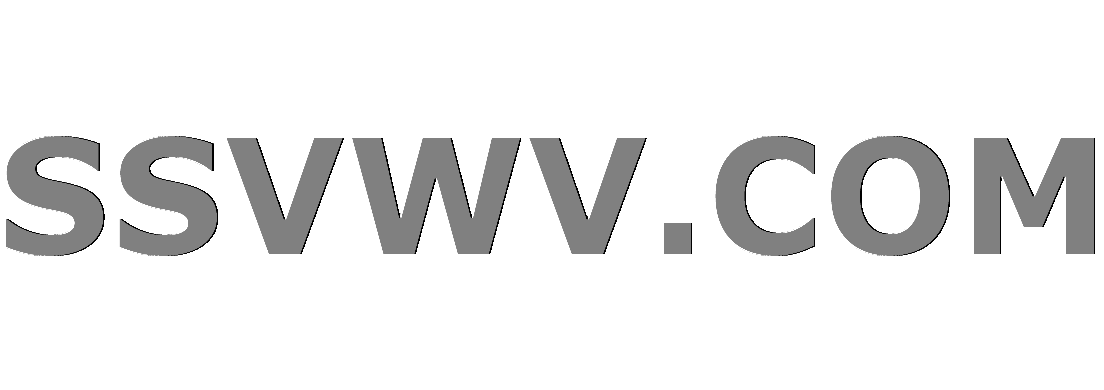
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
This class, UnorderedList
, implements an Unordered list in Java. In this class, duplicates are allowed. remove(argument)
will delete the first occurrence of the argument. I was wondering to add other functions named add(int index, int data)
and set(int index, int data)
or not. Since the class is unordered, I thought I would not need it. The size of the class is limited although that does not concern me (UnorderedLinkedList
coming up next).
I tried to make sure all the functionality and behaviours are correct. If this class is lacking any method(s) and/or correct usage of exception and anything else please comment below. Any kind of suggestions and advice is appreciated.
//Node class
package lists.arrayLists.unorderedList;
public class Node
private int data;
public Node(int data)
this.data = data;
public int getData()
return data;
//UnorderedList class
package lists.arrayLists.unorderedList;
import java.lang.IndexOutOfBoundsException;
import java.util.NoSuchElementException;
public class UnorderedList
public static final int MAX_SIZE = 100;
private int size;
private Node nodeElements;
private int currentIndex; // to navigate/iterate over list
public UnorderedList()
nodeElements = new Node[MAX_SIZE];
public boolean isFull()
return (size() == MAX_SIZE);
public boolean isEmpty()
return (size() == 0);
public int size()
return size;
public boolean add(int data)
try
Node node = new Node(data);
nodeElements[size++] = node;
return true;
catch (IndexOutOfBoundsException ex)
System.out.println(ex.getMessage());
throw new IndexOutOfBoundsException();
public void remove(int data)
int index = locate(data);
try
nodeElements[index] = nodeElements[size() - 1];
size--;
catch (IndexOutOfBoundsException ex)
throw new NoSuchElementException();
public boolean find(int data)
boolean found = false;
try
if(locate(data) >= 0)
found = true;
return found;
catch (NoSuchElementException ex)
throw new NoSuchElementException();
// locate function looks for an element in the array, if there is one then returns its index
private int locate(int data)
int i = 0;
int index = -1;
boolean found = false;
while (i < size() && !found)
if (data == nodeElements[i].getData())
found = true;
index = i;
i++;
return index;
//Navigator methods
public void reset()
currentIndex = 0;
public boolean hasNext()
return (currentIndex == size());
public void next()
if (!hasNext()) currentIndex++;
public int currentElement()
return nodeElements[currentIndex].getData();
java object-oriented
add a comment |Â
up vote
3
down vote
favorite
This class, UnorderedList
, implements an Unordered list in Java. In this class, duplicates are allowed. remove(argument)
will delete the first occurrence of the argument. I was wondering to add other functions named add(int index, int data)
and set(int index, int data)
or not. Since the class is unordered, I thought I would not need it. The size of the class is limited although that does not concern me (UnorderedLinkedList
coming up next).
I tried to make sure all the functionality and behaviours are correct. If this class is lacking any method(s) and/or correct usage of exception and anything else please comment below. Any kind of suggestions and advice is appreciated.
//Node class
package lists.arrayLists.unorderedList;
public class Node
private int data;
public Node(int data)
this.data = data;
public int getData()
return data;
//UnorderedList class
package lists.arrayLists.unorderedList;
import java.lang.IndexOutOfBoundsException;
import java.util.NoSuchElementException;
public class UnorderedList
public static final int MAX_SIZE = 100;
private int size;
private Node nodeElements;
private int currentIndex; // to navigate/iterate over list
public UnorderedList()
nodeElements = new Node[MAX_SIZE];
public boolean isFull()
return (size() == MAX_SIZE);
public boolean isEmpty()
return (size() == 0);
public int size()
return size;
public boolean add(int data)
try
Node node = new Node(data);
nodeElements[size++] = node;
return true;
catch (IndexOutOfBoundsException ex)
System.out.println(ex.getMessage());
throw new IndexOutOfBoundsException();
public void remove(int data)
int index = locate(data);
try
nodeElements[index] = nodeElements[size() - 1];
size--;
catch (IndexOutOfBoundsException ex)
throw new NoSuchElementException();
public boolean find(int data)
boolean found = false;
try
if(locate(data) >= 0)
found = true;
return found;
catch (NoSuchElementException ex)
throw new NoSuchElementException();
// locate function looks for an element in the array, if there is one then returns its index
private int locate(int data)
int i = 0;
int index = -1;
boolean found = false;
while (i < size() && !found)
if (data == nodeElements[i].getData())
found = true;
index = i;
i++;
return index;
//Navigator methods
public void reset()
currentIndex = 0;
public boolean hasNext()
return (currentIndex == size());
public void next()
if (!hasNext()) currentIndex++;
public int currentElement()
return nodeElements[currentIndex].getData();
java object-oriented
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
This class, UnorderedList
, implements an Unordered list in Java. In this class, duplicates are allowed. remove(argument)
will delete the first occurrence of the argument. I was wondering to add other functions named add(int index, int data)
and set(int index, int data)
or not. Since the class is unordered, I thought I would not need it. The size of the class is limited although that does not concern me (UnorderedLinkedList
coming up next).
I tried to make sure all the functionality and behaviours are correct. If this class is lacking any method(s) and/or correct usage of exception and anything else please comment below. Any kind of suggestions and advice is appreciated.
//Node class
package lists.arrayLists.unorderedList;
public class Node
private int data;
public Node(int data)
this.data = data;
public int getData()
return data;
//UnorderedList class
package lists.arrayLists.unorderedList;
import java.lang.IndexOutOfBoundsException;
import java.util.NoSuchElementException;
public class UnorderedList
public static final int MAX_SIZE = 100;
private int size;
private Node nodeElements;
private int currentIndex; // to navigate/iterate over list
public UnorderedList()
nodeElements = new Node[MAX_SIZE];
public boolean isFull()
return (size() == MAX_SIZE);
public boolean isEmpty()
return (size() == 0);
public int size()
return size;
public boolean add(int data)
try
Node node = new Node(data);
nodeElements[size++] = node;
return true;
catch (IndexOutOfBoundsException ex)
System.out.println(ex.getMessage());
throw new IndexOutOfBoundsException();
public void remove(int data)
int index = locate(data);
try
nodeElements[index] = nodeElements[size() - 1];
size--;
catch (IndexOutOfBoundsException ex)
throw new NoSuchElementException();
public boolean find(int data)
boolean found = false;
try
if(locate(data) >= 0)
found = true;
return found;
catch (NoSuchElementException ex)
throw new NoSuchElementException();
// locate function looks for an element in the array, if there is one then returns its index
private int locate(int data)
int i = 0;
int index = -1;
boolean found = false;
while (i < size() && !found)
if (data == nodeElements[i].getData())
found = true;
index = i;
i++;
return index;
//Navigator methods
public void reset()
currentIndex = 0;
public boolean hasNext()
return (currentIndex == size());
public void next()
if (!hasNext()) currentIndex++;
public int currentElement()
return nodeElements[currentIndex].getData();
java object-oriented
This class, UnorderedList
, implements an Unordered list in Java. In this class, duplicates are allowed. remove(argument)
will delete the first occurrence of the argument. I was wondering to add other functions named add(int index, int data)
and set(int index, int data)
or not. Since the class is unordered, I thought I would not need it. The size of the class is limited although that does not concern me (UnorderedLinkedList
coming up next).
I tried to make sure all the functionality and behaviours are correct. If this class is lacking any method(s) and/or correct usage of exception and anything else please comment below. Any kind of suggestions and advice is appreciated.
//Node class
package lists.arrayLists.unorderedList;
public class Node
private int data;
public Node(int data)
this.data = data;
public int getData()
return data;
//UnorderedList class
package lists.arrayLists.unorderedList;
import java.lang.IndexOutOfBoundsException;
import java.util.NoSuchElementException;
public class UnorderedList
public static final int MAX_SIZE = 100;
private int size;
private Node nodeElements;
private int currentIndex; // to navigate/iterate over list
public UnorderedList()
nodeElements = new Node[MAX_SIZE];
public boolean isFull()
return (size() == MAX_SIZE);
public boolean isEmpty()
return (size() == 0);
public int size()
return size;
public boolean add(int data)
try
Node node = new Node(data);
nodeElements[size++] = node;
return true;
catch (IndexOutOfBoundsException ex)
System.out.println(ex.getMessage());
throw new IndexOutOfBoundsException();
public void remove(int data)
int index = locate(data);
try
nodeElements[index] = nodeElements[size() - 1];
size--;
catch (IndexOutOfBoundsException ex)
throw new NoSuchElementException();
public boolean find(int data)
boolean found = false;
try
if(locate(data) >= 0)
found = true;
return found;
catch (NoSuchElementException ex)
throw new NoSuchElementException();
// locate function looks for an element in the array, if there is one then returns its index
private int locate(int data)
int i = 0;
int index = -1;
boolean found = false;
while (i < size() && !found)
if (data == nodeElements[i].getData())
found = true;
index = i;
i++;
return index;
//Navigator methods
public void reset()
currentIndex = 0;
public boolean hasNext()
return (currentIndex == size());
public void next()
if (!hasNext()) currentIndex++;
public int currentElement()
return nodeElements[currentIndex].getData();
java object-oriented
edited Jun 8 at 2:24


Jamal♦
30.1k11114225
30.1k11114225
asked Jun 8 at 2:21


Hamidur Rahman
436
436
add a comment |Â
add a comment |Â
2 Answers
2
active
oldest
votes
up vote
2
down vote
locate
never throws, so whatfind
expects to catch? Considerpublic boolean find(int data)
return locate(data) >= 0;locate
is overcomplicated. Considerprivate int locate(data) {
for (int index = 0; index < size(); index++)
if (data == nodeElements[i].getData())
return index;
return -1;reset
,hasNext
, andnext
do not belong to the list class; they are the methods of a list iterator. For starters, notice that your implementation only allows one list traversal at a time.That said, the semantics of
hasNext()
is wrong. The conditioncurrentIndex == size()
means that there is no next. A client of your class would be badly confused.Along the same line,
next()
should not silently ignore the end-of-list condition.
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define aniterator
class holdingcurrentIndex
, withnext
andhasNext
methods, and pass your list to its constructor,
– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
add a comment |Â
up vote
2
down vote
I would recommend using Log4j
instead of System.out.println()
with some meaningful message to the user. Also, you can implement locate()
method as mentioned below to avoid initializing the flag
private int locate(int data)
int i = 0;
int index = -1;
while (i < size())
if (data == nodeElements[i].getData())
index = i;
break;
i++;
return index;
Change: Use break;
to come out of the loop rather than using flag to check it.
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
add a comment |Â
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
locate
never throws, so whatfind
expects to catch? Considerpublic boolean find(int data)
return locate(data) >= 0;locate
is overcomplicated. Considerprivate int locate(data) {
for (int index = 0; index < size(); index++)
if (data == nodeElements[i].getData())
return index;
return -1;reset
,hasNext
, andnext
do not belong to the list class; they are the methods of a list iterator. For starters, notice that your implementation only allows one list traversal at a time.That said, the semantics of
hasNext()
is wrong. The conditioncurrentIndex == size()
means that there is no next. A client of your class would be badly confused.Along the same line,
next()
should not silently ignore the end-of-list condition.
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define aniterator
class holdingcurrentIndex
, withnext
andhasNext
methods, and pass your list to its constructor,
– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
add a comment |Â
up vote
2
down vote
locate
never throws, so whatfind
expects to catch? Considerpublic boolean find(int data)
return locate(data) >= 0;locate
is overcomplicated. Considerprivate int locate(data) {
for (int index = 0; index < size(); index++)
if (data == nodeElements[i].getData())
return index;
return -1;reset
,hasNext
, andnext
do not belong to the list class; they are the methods of a list iterator. For starters, notice that your implementation only allows one list traversal at a time.That said, the semantics of
hasNext()
is wrong. The conditioncurrentIndex == size()
means that there is no next. A client of your class would be badly confused.Along the same line,
next()
should not silently ignore the end-of-list condition.
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define aniterator
class holdingcurrentIndex
, withnext
andhasNext
methods, and pass your list to its constructor,
– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
add a comment |Â
up vote
2
down vote
up vote
2
down vote
locate
never throws, so whatfind
expects to catch? Considerpublic boolean find(int data)
return locate(data) >= 0;locate
is overcomplicated. Considerprivate int locate(data) {
for (int index = 0; index < size(); index++)
if (data == nodeElements[i].getData())
return index;
return -1;reset
,hasNext
, andnext
do not belong to the list class; they are the methods of a list iterator. For starters, notice that your implementation only allows one list traversal at a time.That said, the semantics of
hasNext()
is wrong. The conditioncurrentIndex == size()
means that there is no next. A client of your class would be badly confused.Along the same line,
next()
should not silently ignore the end-of-list condition.
locate
never throws, so whatfind
expects to catch? Considerpublic boolean find(int data)
return locate(data) >= 0;locate
is overcomplicated. Considerprivate int locate(data) {
for (int index = 0; index < size(); index++)
if (data == nodeElements[i].getData())
return index;
return -1;reset
,hasNext
, andnext
do not belong to the list class; they are the methods of a list iterator. For starters, notice that your implementation only allows one list traversal at a time.That said, the semantics of
hasNext()
is wrong. The conditioncurrentIndex == size()
means that there is no next. A client of your class would be badly confused.Along the same line,
next()
should not silently ignore the end-of-list condition.
answered Jun 8 at 6:48


vnp
36.4k12890
36.4k12890
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define aniterator
class holdingcurrentIndex
, withnext
andhasNext
methods, and pass your list to its constructor,
– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
add a comment |Â
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define aniterator
class holdingcurrentIndex
, withnext
andhasNext
methods, and pass your list to its constructor,
– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
how do I separate Iterator class from this class? Any demonstration or link to this solution would be very helpful. @vnp
– Hamidur Rahman
Jun 8 at 19:06
@HamidurRahman I don't know Java conventions, but normally you'd define an
iterator
class holding currentIndex
, with next
and hasNext
methods, and pass your list to its constructor,– vnp
Jun 8 at 19:09
@HamidurRahman I don't know Java conventions, but normally you'd define an
iterator
class holding currentIndex
, with next
and hasNext
methods, and pass your list to its constructor,– vnp
Jun 8 at 19:09
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
Thanks. I will look for it and find the solution and then post it in this comment section. Is that okay? @vnp
– Hamidur Rahman
Jun 8 at 19:12
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
@HamidurRahman Better post a follow-up question.
– vnp
Jun 8 at 19:13
add a comment |Â
up vote
2
down vote
I would recommend using Log4j
instead of System.out.println()
with some meaningful message to the user. Also, you can implement locate()
method as mentioned below to avoid initializing the flag
private int locate(int data)
int i = 0;
int index = -1;
while (i < size())
if (data == nodeElements[i].getData())
index = i;
break;
i++;
return index;
Change: Use break;
to come out of the loop rather than using flag to check it.
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
add a comment |Â
up vote
2
down vote
I would recommend using Log4j
instead of System.out.println()
with some meaningful message to the user. Also, you can implement locate()
method as mentioned below to avoid initializing the flag
private int locate(int data)
int i = 0;
int index = -1;
while (i < size())
if (data == nodeElements[i].getData())
index = i;
break;
i++;
return index;
Change: Use break;
to come out of the loop rather than using flag to check it.
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
add a comment |Â
up vote
2
down vote
up vote
2
down vote
I would recommend using Log4j
instead of System.out.println()
with some meaningful message to the user. Also, you can implement locate()
method as mentioned below to avoid initializing the flag
private int locate(int data)
int i = 0;
int index = -1;
while (i < size())
if (data == nodeElements[i].getData())
index = i;
break;
i++;
return index;
Change: Use break;
to come out of the loop rather than using flag to check it.
I would recommend using Log4j
instead of System.out.println()
with some meaningful message to the user. Also, you can implement locate()
method as mentioned below to avoid initializing the flag
private int locate(int data)
int i = 0;
int index = -1;
while (i < size())
if (data == nodeElements[i].getData())
index = i;
break;
i++;
return index;
Change: Use break;
to come out of the loop rather than using flag to check it.
edited Jun 8 at 7:27
answered Jun 8 at 6:18


Stack Victor
1294
1294
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
add a comment |Â
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
Please summarize the changes you made. Code only answers are considered off-topic.
– t3chb0t
Jun 8 at 6:50
1
1
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
@t3chb0t, done:).
– Stack Victor
Jun 8 at 7:27
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f196083%2farray-based-unordered-list-implementation-in-java%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password