Simple locale loader
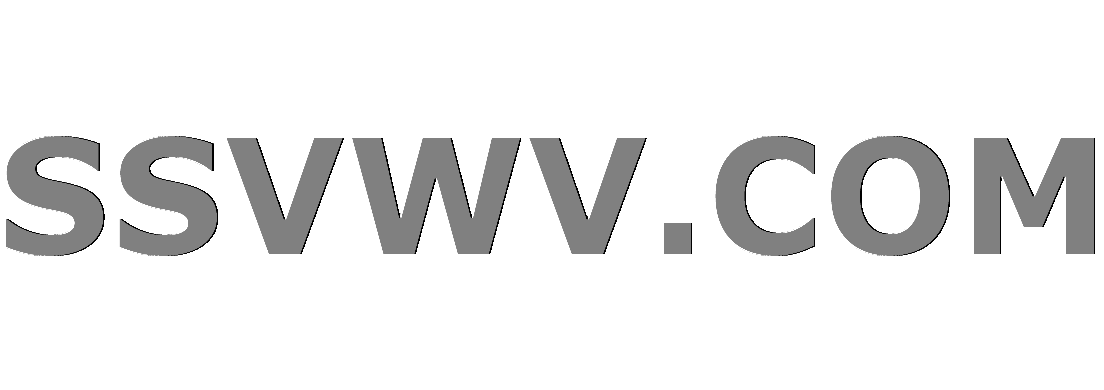
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
4
down vote
favorite
I have a class that loads locales from a database. I'm not sure if locale is the right word. It's basically loading texts for a game, generic sayings like alerts and error messages.
I'm also not sure if it should be called WhateverHandler
or WhateverController
, maybe even WhateverManager
. I tend to get really OCD about my code, so some advice would be nice.
public class LocaleHandler
private static readonly ILogger Logger = new ConsoleLogger(typeof(LanguageLocale));
private readonly ConcurrentDictionary<string, string> _localeKeyValue;
public LocaleHandler()
using (var dbConnection = PlusEnvironment.GetDatabaseManager().Connection)
_localeKeyValue = FetchLocale(dbConnection);
Logger.Trace($"Fetched _localeKeyValue.Count language locales from the database.");
private static ConcurrentDictionary<string, string> FetchLocale(DatabaseConnection dbConnection)
dbConnection.SetQuery("SELECT * FROM `server_locale`");
using (var reader = dbConnection.ExecuteReader())
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
var locale = new ConcurrentDictionary<string, string>();
while (reader.Read())
locale.TryAdd(reader.GetString("key"), reader.GetString("value"));
return locale;
public bool TryGetLocale(string key, out string value)
return _localeKeyValue.TryGetValue(key, out value);
c# database t-sql ado.net localization
add a comment |Â
up vote
4
down vote
favorite
I have a class that loads locales from a database. I'm not sure if locale is the right word. It's basically loading texts for a game, generic sayings like alerts and error messages.
I'm also not sure if it should be called WhateverHandler
or WhateverController
, maybe even WhateverManager
. I tend to get really OCD about my code, so some advice would be nice.
public class LocaleHandler
private static readonly ILogger Logger = new ConsoleLogger(typeof(LanguageLocale));
private readonly ConcurrentDictionary<string, string> _localeKeyValue;
public LocaleHandler()
using (var dbConnection = PlusEnvironment.GetDatabaseManager().Connection)
_localeKeyValue = FetchLocale(dbConnection);
Logger.Trace($"Fetched _localeKeyValue.Count language locales from the database.");
private static ConcurrentDictionary<string, string> FetchLocale(DatabaseConnection dbConnection)
dbConnection.SetQuery("SELECT * FROM `server_locale`");
using (var reader = dbConnection.ExecuteReader())
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
var locale = new ConcurrentDictionary<string, string>();
while (reader.Read())
locale.TryAdd(reader.GetString("key"), reader.GetString("value"));
return locale;
public bool TryGetLocale(string key, out string value)
return _localeKeyValue.TryGetValue(key, out value);
c# database t-sql ado.net localization
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
1
Is there any reason you are using theConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.
– t3chb0t
Jun 5 at 6:12
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00
add a comment |Â
up vote
4
down vote
favorite
up vote
4
down vote
favorite
I have a class that loads locales from a database. I'm not sure if locale is the right word. It's basically loading texts for a game, generic sayings like alerts and error messages.
I'm also not sure if it should be called WhateverHandler
or WhateverController
, maybe even WhateverManager
. I tend to get really OCD about my code, so some advice would be nice.
public class LocaleHandler
private static readonly ILogger Logger = new ConsoleLogger(typeof(LanguageLocale));
private readonly ConcurrentDictionary<string, string> _localeKeyValue;
public LocaleHandler()
using (var dbConnection = PlusEnvironment.GetDatabaseManager().Connection)
_localeKeyValue = FetchLocale(dbConnection);
Logger.Trace($"Fetched _localeKeyValue.Count language locales from the database.");
private static ConcurrentDictionary<string, string> FetchLocale(DatabaseConnection dbConnection)
dbConnection.SetQuery("SELECT * FROM `server_locale`");
using (var reader = dbConnection.ExecuteReader())
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
var locale = new ConcurrentDictionary<string, string>();
while (reader.Read())
locale.TryAdd(reader.GetString("key"), reader.GetString("value"));
return locale;
public bool TryGetLocale(string key, out string value)
return _localeKeyValue.TryGetValue(key, out value);
c# database t-sql ado.net localization
I have a class that loads locales from a database. I'm not sure if locale is the right word. It's basically loading texts for a game, generic sayings like alerts and error messages.
I'm also not sure if it should be called WhateverHandler
or WhateverController
, maybe even WhateverManager
. I tend to get really OCD about my code, so some advice would be nice.
public class LocaleHandler
private static readonly ILogger Logger = new ConsoleLogger(typeof(LanguageLocale));
private readonly ConcurrentDictionary<string, string> _localeKeyValue;
public LocaleHandler()
using (var dbConnection = PlusEnvironment.GetDatabaseManager().Connection)
_localeKeyValue = FetchLocale(dbConnection);
Logger.Trace($"Fetched _localeKeyValue.Count language locales from the database.");
private static ConcurrentDictionary<string, string> FetchLocale(DatabaseConnection dbConnection)
dbConnection.SetQuery("SELECT * FROM `server_locale`");
using (var reader = dbConnection.ExecuteReader())
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
var locale = new ConcurrentDictionary<string, string>();
while (reader.Read())
locale.TryAdd(reader.GetString("key"), reader.GetString("value"));
return locale;
public bool TryGetLocale(string key, out string value)
return _localeKeyValue.TryGetValue(key, out value);
c# database t-sql ado.net localization
edited Jun 5 at 6:13


t3chb0t
31.9k54195
31.9k54195
asked Jun 4 at 23:31
serialize
211
211
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
1
Is there any reason you are using theConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.
– t3chb0t
Jun 5 at 6:12
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00
add a comment |Â
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
1
Is there any reason you are using theConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.
– t3chb0t
Jun 5 at 6:12
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
1
1
Is there any reason you are using the
ConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.– t3chb0t
Jun 5 at 6:12
Is there any reason you are using the
ConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.– t3chb0t
Jun 5 at 6:12
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00
add a comment |Â
2 Answers
2
active
oldest
votes
up vote
1
down vote
First, since you are concerned about naming: this class doesn't really handle, manage, or control anything. It does two things: queries a database, and makes those results available via a TryGet interface. In fact, you could split it into two classes, LocaleLoader
and Locale
. Though I wonder if "locale" is even the correct term since there is no locale supplied (like "en-us"), it's more of a string table. On the topic of naming, "_localeKeyValue" is not very good; it describes the implementation and not the purpose. Something like "Strings" would be better. Even "_localeDict" would be an improvement.
As noted in the comments, the ConcurrentDictionary is unnecessary; Dictionary supports concurrent readers as long as there are no writers. In your case, all reading occurs after all writing.
@paparazzo is right about checking for no results being unnecessary. The result is the same, it's no faster, and it's more code.
Consider allowing the constructor to be passed in a database connection to use. This makes it more flexible and removes a dependency.
TryGetLocale: are you really getting a locale? I think you are getting a string, translation, or localization. Without seeing how this code is used, I question the usefulness of the TryGet-style interface. For (what I imagine to be) my similar code, I like to just return the key if it's not found. Something like:
public String GetString(String key)
String value;
return _localeKeyValue.TryGetValue(key, out value) ? value : key;
This means that when the code encounters an untranslated string it's obvious to the devs and testers what is missing. The other possibility would be to throw an exception when you can't find the key, but on the off-chance that you ship software with a missing string it's better for the user to see a message box like "IMG_CONVERSION_JPEG_SUCCESS" than "Illegal argument: key".
add a comment |Â
up vote
0
down vote
This is not required
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
add a comment |Â
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
First, since you are concerned about naming: this class doesn't really handle, manage, or control anything. It does two things: queries a database, and makes those results available via a TryGet interface. In fact, you could split it into two classes, LocaleLoader
and Locale
. Though I wonder if "locale" is even the correct term since there is no locale supplied (like "en-us"), it's more of a string table. On the topic of naming, "_localeKeyValue" is not very good; it describes the implementation and not the purpose. Something like "Strings" would be better. Even "_localeDict" would be an improvement.
As noted in the comments, the ConcurrentDictionary is unnecessary; Dictionary supports concurrent readers as long as there are no writers. In your case, all reading occurs after all writing.
@paparazzo is right about checking for no results being unnecessary. The result is the same, it's no faster, and it's more code.
Consider allowing the constructor to be passed in a database connection to use. This makes it more flexible and removes a dependency.
TryGetLocale: are you really getting a locale? I think you are getting a string, translation, or localization. Without seeing how this code is used, I question the usefulness of the TryGet-style interface. For (what I imagine to be) my similar code, I like to just return the key if it's not found. Something like:
public String GetString(String key)
String value;
return _localeKeyValue.TryGetValue(key, out value) ? value : key;
This means that when the code encounters an untranslated string it's obvious to the devs and testers what is missing. The other possibility would be to throw an exception when you can't find the key, but on the off-chance that you ship software with a missing string it's better for the user to see a message box like "IMG_CONVERSION_JPEG_SUCCESS" than "Illegal argument: key".
add a comment |Â
up vote
1
down vote
First, since you are concerned about naming: this class doesn't really handle, manage, or control anything. It does two things: queries a database, and makes those results available via a TryGet interface. In fact, you could split it into two classes, LocaleLoader
and Locale
. Though I wonder if "locale" is even the correct term since there is no locale supplied (like "en-us"), it's more of a string table. On the topic of naming, "_localeKeyValue" is not very good; it describes the implementation and not the purpose. Something like "Strings" would be better. Even "_localeDict" would be an improvement.
As noted in the comments, the ConcurrentDictionary is unnecessary; Dictionary supports concurrent readers as long as there are no writers. In your case, all reading occurs after all writing.
@paparazzo is right about checking for no results being unnecessary. The result is the same, it's no faster, and it's more code.
Consider allowing the constructor to be passed in a database connection to use. This makes it more flexible and removes a dependency.
TryGetLocale: are you really getting a locale? I think you are getting a string, translation, or localization. Without seeing how this code is used, I question the usefulness of the TryGet-style interface. For (what I imagine to be) my similar code, I like to just return the key if it's not found. Something like:
public String GetString(String key)
String value;
return _localeKeyValue.TryGetValue(key, out value) ? value : key;
This means that when the code encounters an untranslated string it's obvious to the devs and testers what is missing. The other possibility would be to throw an exception when you can't find the key, but on the off-chance that you ship software with a missing string it's better for the user to see a message box like "IMG_CONVERSION_JPEG_SUCCESS" than "Illegal argument: key".
add a comment |Â
up vote
1
down vote
up vote
1
down vote
First, since you are concerned about naming: this class doesn't really handle, manage, or control anything. It does two things: queries a database, and makes those results available via a TryGet interface. In fact, you could split it into two classes, LocaleLoader
and Locale
. Though I wonder if "locale" is even the correct term since there is no locale supplied (like "en-us"), it's more of a string table. On the topic of naming, "_localeKeyValue" is not very good; it describes the implementation and not the purpose. Something like "Strings" would be better. Even "_localeDict" would be an improvement.
As noted in the comments, the ConcurrentDictionary is unnecessary; Dictionary supports concurrent readers as long as there are no writers. In your case, all reading occurs after all writing.
@paparazzo is right about checking for no results being unnecessary. The result is the same, it's no faster, and it's more code.
Consider allowing the constructor to be passed in a database connection to use. This makes it more flexible and removes a dependency.
TryGetLocale: are you really getting a locale? I think you are getting a string, translation, or localization. Without seeing how this code is used, I question the usefulness of the TryGet-style interface. For (what I imagine to be) my similar code, I like to just return the key if it's not found. Something like:
public String GetString(String key)
String value;
return _localeKeyValue.TryGetValue(key, out value) ? value : key;
This means that when the code encounters an untranslated string it's obvious to the devs and testers what is missing. The other possibility would be to throw an exception when you can't find the key, but on the off-chance that you ship software with a missing string it's better for the user to see a message box like "IMG_CONVERSION_JPEG_SUCCESS" than "Illegal argument: key".
First, since you are concerned about naming: this class doesn't really handle, manage, or control anything. It does two things: queries a database, and makes those results available via a TryGet interface. In fact, you could split it into two classes, LocaleLoader
and Locale
. Though I wonder if "locale" is even the correct term since there is no locale supplied (like "en-us"), it's more of a string table. On the topic of naming, "_localeKeyValue" is not very good; it describes the implementation and not the purpose. Something like "Strings" would be better. Even "_localeDict" would be an improvement.
As noted in the comments, the ConcurrentDictionary is unnecessary; Dictionary supports concurrent readers as long as there are no writers. In your case, all reading occurs after all writing.
@paparazzo is right about checking for no results being unnecessary. The result is the same, it's no faster, and it's more code.
Consider allowing the constructor to be passed in a database connection to use. This makes it more flexible and removes a dependency.
TryGetLocale: are you really getting a locale? I think you are getting a string, translation, or localization. Without seeing how this code is used, I question the usefulness of the TryGet-style interface. For (what I imagine to be) my similar code, I like to just return the key if it's not found. Something like:
public String GetString(String key)
String value;
return _localeKeyValue.TryGetValue(key, out value) ? value : key;
This means that when the code encounters an untranslated string it's obvious to the devs and testers what is missing. The other possibility would be to throw an exception when you can't find the key, but on the off-chance that you ship software with a missing string it's better for the user to see a message box like "IMG_CONVERSION_JPEG_SUCCESS" than "Illegal argument: key".
edited Jul 23 at 21:37
answered Jul 21 at 2:14
Pierre Menard
1,412618
1,412618
add a comment |Â
add a comment |Â
up vote
0
down vote
This is not required
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
add a comment |Â
up vote
0
down vote
This is not required
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
add a comment |Â
up vote
0
down vote
up vote
0
down vote
This is not required
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
This is not required
if (!reader.HasRows)
return new ConcurrentDictionary<string, string>();
answered Jun 5 at 4:36


paparazzo
4,8131730
4,8131730
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f195847%2fsimple-locale-loader%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
I think the term you are looking for is localization.
– Nkosi
Jun 5 at 0:33
1
Is there any reason you are using the
ConcurrentDictionary
everywhere? There doesn't seem to by any concurrency.– t3chb0t
Jun 5 at 6:12
You can't see if there's any concurrency from just that class, although the class does get accessed by many other classes.
– serialize
Jun 5 at 22:00