Check Unique Chars in A String
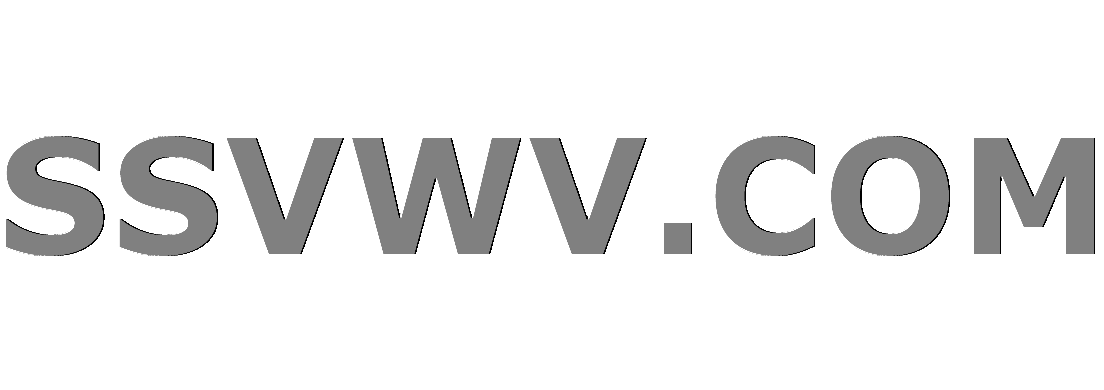
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
-1
down vote
favorite
Two solutions to check if a String contains unique characters.
Wanted to know if these solutions can be optimized further.
The Code:
public class UniqueCharactersInString
//running time O(N) and O(N) space
public static boolean hasUniqueChars(String text)
//running time O(N ^ 2) and O(1) space
public static boolean hasUniqueChars2(String text)
if (text == null
public static void main(String... args)
System.out.println(hasUniqueChars("test123"));
System.out.println(hasUniqueChars2("test123"));
java strings comparative-review
add a comment |Â
up vote
-1
down vote
favorite
Two solutions to check if a String contains unique characters.
Wanted to know if these solutions can be optimized further.
The Code:
public class UniqueCharactersInString
//running time O(N) and O(N) space
public static boolean hasUniqueChars(String text)
//running time O(N ^ 2) and O(1) space
public static boolean hasUniqueChars2(String text)
if (text == null
public static void main(String... args)
System.out.println(hasUniqueChars("test123"));
System.out.println(hasUniqueChars2("test123"));
java strings comparative-review
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54
add a comment |Â
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
Two solutions to check if a String contains unique characters.
Wanted to know if these solutions can be optimized further.
The Code:
public class UniqueCharactersInString
//running time O(N) and O(N) space
public static boolean hasUniqueChars(String text)
//running time O(N ^ 2) and O(1) space
public static boolean hasUniqueChars2(String text)
if (text == null
public static void main(String... args)
System.out.println(hasUniqueChars("test123"));
System.out.println(hasUniqueChars2("test123"));
java strings comparative-review
Two solutions to check if a String contains unique characters.
Wanted to know if these solutions can be optimized further.
The Code:
public class UniqueCharactersInString
//running time O(N) and O(N) space
public static boolean hasUniqueChars(String text)
//running time O(N ^ 2) and O(1) space
public static boolean hasUniqueChars2(String text)
if (text == null
public static void main(String... args)
System.out.println(hasUniqueChars("test123"));
System.out.println(hasUniqueChars2("test123"));
java strings comparative-review
edited Jul 13 at 16:53


200_success
123k14143399
123k14143399
asked Jul 13 at 15:30
DntFrgtDSemiCln
20826
20826
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54
add a comment |Â
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Both solutions
Both your solutions check for text.lenght() == 1
. This is not really much of an optimization and it makes it seem like it is a special case, but your code will do just fine without that check. Remove it.
First solution
Your first solution using a HashSet can be improved by using the return value of the add(...)
method, which is true
if the value is added, and false
if the value was already there. See the documentation
This allows you to simplify the inner condition from:
if (textChars.contains(c))
return false;
textChars.add(c);
to
if (!textChars.add(c))
return false;
In general, while your complexity assessments are accurate, there is a significant amount of space involved in converting char
to Character
instances and then the overheads of the HashSet
are significant. I imagine for even reasonably large strings, it will be slower than your second option... which also has problems.
Second solution
This solution is neatly written, but has a significant performance issue. Instead of the j
loop running from 0
to text.length()
it can instead run from 0
to i
. The solution you currently have compares many values redundantly (for example, in the word hello
it will compare h
to e
and also e
to h
)
The resulting code would look like:
//nested loop to check
for (int i = 0; i < text.length(); i++)
for (int j = 0; j < i; j++)
if (text.charAt(i) == text.charAt(j))
return false;
Bug
While answering this section, though, I see your code actually has a bug, which irritates me, because this has to be something you have seen..... your code's j
loop looks like:
for (int j = 0; i < text.length(); j++) {
and that contains the condition i < text.lenght()
instead of j < text.lenght()
.... so you have an infinite loop. Your code is broken. If you had run this code, you would know that....
My recommendation
... is for a 3rd option to try. You should get the characters in an array, and then sort them, and compare neighbours....
for example:
//running time O(N log(N)) and O(N) space
public static boolean hasUniqueChars3(String text)
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Both solutions
Both your solutions check for text.lenght() == 1
. This is not really much of an optimization and it makes it seem like it is a special case, but your code will do just fine without that check. Remove it.
First solution
Your first solution using a HashSet can be improved by using the return value of the add(...)
method, which is true
if the value is added, and false
if the value was already there. See the documentation
This allows you to simplify the inner condition from:
if (textChars.contains(c))
return false;
textChars.add(c);
to
if (!textChars.add(c))
return false;
In general, while your complexity assessments are accurate, there is a significant amount of space involved in converting char
to Character
instances and then the overheads of the HashSet
are significant. I imagine for even reasonably large strings, it will be slower than your second option... which also has problems.
Second solution
This solution is neatly written, but has a significant performance issue. Instead of the j
loop running from 0
to text.length()
it can instead run from 0
to i
. The solution you currently have compares many values redundantly (for example, in the word hello
it will compare h
to e
and also e
to h
)
The resulting code would look like:
//nested loop to check
for (int i = 0; i < text.length(); i++)
for (int j = 0; j < i; j++)
if (text.charAt(i) == text.charAt(j))
return false;
Bug
While answering this section, though, I see your code actually has a bug, which irritates me, because this has to be something you have seen..... your code's j
loop looks like:
for (int j = 0; i < text.length(); j++) {
and that contains the condition i < text.lenght()
instead of j < text.lenght()
.... so you have an infinite loop. Your code is broken. If you had run this code, you would know that....
My recommendation
... is for a 3rd option to try. You should get the characters in an array, and then sort them, and compare neighbours....
for example:
//running time O(N log(N)) and O(N) space
public static boolean hasUniqueChars3(String text)
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
add a comment |Â
up vote
2
down vote
accepted
Both solutions
Both your solutions check for text.lenght() == 1
. This is not really much of an optimization and it makes it seem like it is a special case, but your code will do just fine without that check. Remove it.
First solution
Your first solution using a HashSet can be improved by using the return value of the add(...)
method, which is true
if the value is added, and false
if the value was already there. See the documentation
This allows you to simplify the inner condition from:
if (textChars.contains(c))
return false;
textChars.add(c);
to
if (!textChars.add(c))
return false;
In general, while your complexity assessments are accurate, there is a significant amount of space involved in converting char
to Character
instances and then the overheads of the HashSet
are significant. I imagine for even reasonably large strings, it will be slower than your second option... which also has problems.
Second solution
This solution is neatly written, but has a significant performance issue. Instead of the j
loop running from 0
to text.length()
it can instead run from 0
to i
. The solution you currently have compares many values redundantly (for example, in the word hello
it will compare h
to e
and also e
to h
)
The resulting code would look like:
//nested loop to check
for (int i = 0; i < text.length(); i++)
for (int j = 0; j < i; j++)
if (text.charAt(i) == text.charAt(j))
return false;
Bug
While answering this section, though, I see your code actually has a bug, which irritates me, because this has to be something you have seen..... your code's j
loop looks like:
for (int j = 0; i < text.length(); j++) {
and that contains the condition i < text.lenght()
instead of j < text.lenght()
.... so you have an infinite loop. Your code is broken. If you had run this code, you would know that....
My recommendation
... is for a 3rd option to try. You should get the characters in an array, and then sort them, and compare neighbours....
for example:
//running time O(N log(N)) and O(N) space
public static boolean hasUniqueChars3(String text)
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
add a comment |Â
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Both solutions
Both your solutions check for text.lenght() == 1
. This is not really much of an optimization and it makes it seem like it is a special case, but your code will do just fine without that check. Remove it.
First solution
Your first solution using a HashSet can be improved by using the return value of the add(...)
method, which is true
if the value is added, and false
if the value was already there. See the documentation
This allows you to simplify the inner condition from:
if (textChars.contains(c))
return false;
textChars.add(c);
to
if (!textChars.add(c))
return false;
In general, while your complexity assessments are accurate, there is a significant amount of space involved in converting char
to Character
instances and then the overheads of the HashSet
are significant. I imagine for even reasonably large strings, it will be slower than your second option... which also has problems.
Second solution
This solution is neatly written, but has a significant performance issue. Instead of the j
loop running from 0
to text.length()
it can instead run from 0
to i
. The solution you currently have compares many values redundantly (for example, in the word hello
it will compare h
to e
and also e
to h
)
The resulting code would look like:
//nested loop to check
for (int i = 0; i < text.length(); i++)
for (int j = 0; j < i; j++)
if (text.charAt(i) == text.charAt(j))
return false;
Bug
While answering this section, though, I see your code actually has a bug, which irritates me, because this has to be something you have seen..... your code's j
loop looks like:
for (int j = 0; i < text.length(); j++) {
and that contains the condition i < text.lenght()
instead of j < text.lenght()
.... so you have an infinite loop. Your code is broken. If you had run this code, you would know that....
My recommendation
... is for a 3rd option to try. You should get the characters in an array, and then sort them, and compare neighbours....
for example:
//running time O(N log(N)) and O(N) space
public static boolean hasUniqueChars3(String text)
Both solutions
Both your solutions check for text.lenght() == 1
. This is not really much of an optimization and it makes it seem like it is a special case, but your code will do just fine without that check. Remove it.
First solution
Your first solution using a HashSet can be improved by using the return value of the add(...)
method, which is true
if the value is added, and false
if the value was already there. See the documentation
This allows you to simplify the inner condition from:
if (textChars.contains(c))
return false;
textChars.add(c);
to
if (!textChars.add(c))
return false;
In general, while your complexity assessments are accurate, there is a significant amount of space involved in converting char
to Character
instances and then the overheads of the HashSet
are significant. I imagine for even reasonably large strings, it will be slower than your second option... which also has problems.
Second solution
This solution is neatly written, but has a significant performance issue. Instead of the j
loop running from 0
to text.length()
it can instead run from 0
to i
. The solution you currently have compares many values redundantly (for example, in the word hello
it will compare h
to e
and also e
to h
)
The resulting code would look like:
//nested loop to check
for (int i = 0; i < text.length(); i++)
for (int j = 0; j < i; j++)
if (text.charAt(i) == text.charAt(j))
return false;
Bug
While answering this section, though, I see your code actually has a bug, which irritates me, because this has to be something you have seen..... your code's j
loop looks like:
for (int j = 0; i < text.length(); j++) {
and that contains the condition i < text.lenght()
instead of j < text.lenght()
.... so you have an infinite loop. Your code is broken. If you had run this code, you would know that....
My recommendation
... is for a 3rd option to try. You should get the characters in an array, and then sort them, and compare neighbours....
for example:
//running time O(N log(N)) and O(N) space
public static boolean hasUniqueChars3(String text)
edited Jul 13 at 15:49
answered Jul 13 at 15:43
rolfl♦
90.2k13186390
90.2k13186390
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
add a comment |Â
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
thanks for your comments and recommendation! I have fixed the bug and updated the review..I had run the second option for a false case only and did not notice it!
– DntFrgtDSemiCln
Jul 13 at 16:19
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f198432%2fcheck-unique-chars-in-a-string%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
I have rolled back Rev 4 to 3. Please see What to do when someone answers.
– 200_success
Jul 13 at 16:54