My first QThread with Python + Qt
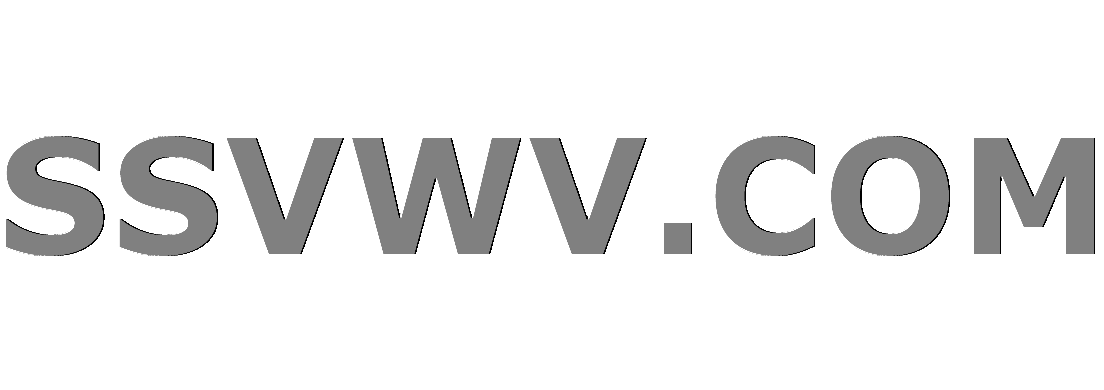
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
0
down vote
favorite
This is the first time that I try to use threads on any programming language. I am learning PySide2 so I tried there.
Note: I removed from __init__
all the window information related to buttons, lines and UI as I consider this snippet is big enough already.
Please let me know if this is the correct way to create a Qthread
. Also, please let me know if my docstrings are wrong, and how to improve them.
from time import sleep
from PySide2.QtCore import QThread, SIGNAL
from PySide2 import QtWidgets
class MainForm(QtWidgets.QMainWindow, Ui_MainWindow):
"""Main window of the program."""
def __init__(self, parent=None):
QtWidgets.QMainWindow.__init__(self, parent)
self.setupUi(self)
self.thread_stats_start()
######################################################
################# THREADING HANDLERS #################
######################################################
def thread_stats_start(self):
"""Create a secondary thread that deals with stats"""
self.THREAD_STATS = ProjectStats()
self.connect(self.THREAD_STATS, SIGNAL("thread_stats_update(QString)"), self.thread_stats_update)
self.THREAD_STATS.start()
def thread_stats_update(self, value):
"""Update the value when you receive the signal"""
current_seconds = alexfunctions.parse_seconds(self.line_ProjectTime.text(), reverse=True)
new_seconds = alexfunctions.parse_seconds(current_seconds + int(value))
self.line_ProjectTime.setText(str(new_seconds))
def thread_stats_stop(self):
"""Stop the Threading and save the data."""
if self.THREAD_STATS.isRunning():
self.THREAD_STATS.terminate()
self.save_stats()
#######################################################
################# THREADING CLASSES ###################
#######################################################
class ProjectStats(QThread):
"""Thread class for Stats updating."""
def __init__(self, parent=None):
QThread.__init__(self, parent)
def run(self):
"""Run the main loop and choose what to send to the main thread"""
while True:
self.emit(SIGNAL('thread_stats_update(QString)'), "1")
sleep(1)
Edit: I forgot to add my parse_seconds method.
def parse_seconds(seconds, reverse=False):
"""Transform seconds into a more valid data
Seconds must be integer for normal mode
Seconds must have <1d, 1h, 1m, 1s> sintax for reverse mode"""
if reverse:
d, h, m, s = seconds.split(", ")
d = int(d[:-1]) * 86400
h = int(h[:-1]) * 3600
m = int(m[:-1]) * 60
s = int(s[:-1])
return s + m + h + d
else:
m, s = divmod(seconds, 60)
h, m = divmod(m, 60)
d, h = divmod(h, 24)
return "0d, 1h, 2m, 3s".format(d, h, m, s)
python python-3.x qt pyside
add a comment |Â
up vote
0
down vote
favorite
This is the first time that I try to use threads on any programming language. I am learning PySide2 so I tried there.
Note: I removed from __init__
all the window information related to buttons, lines and UI as I consider this snippet is big enough already.
Please let me know if this is the correct way to create a Qthread
. Also, please let me know if my docstrings are wrong, and how to improve them.
from time import sleep
from PySide2.QtCore import QThread, SIGNAL
from PySide2 import QtWidgets
class MainForm(QtWidgets.QMainWindow, Ui_MainWindow):
"""Main window of the program."""
def __init__(self, parent=None):
QtWidgets.QMainWindow.__init__(self, parent)
self.setupUi(self)
self.thread_stats_start()
######################################################
################# THREADING HANDLERS #################
######################################################
def thread_stats_start(self):
"""Create a secondary thread that deals with stats"""
self.THREAD_STATS = ProjectStats()
self.connect(self.THREAD_STATS, SIGNAL("thread_stats_update(QString)"), self.thread_stats_update)
self.THREAD_STATS.start()
def thread_stats_update(self, value):
"""Update the value when you receive the signal"""
current_seconds = alexfunctions.parse_seconds(self.line_ProjectTime.text(), reverse=True)
new_seconds = alexfunctions.parse_seconds(current_seconds + int(value))
self.line_ProjectTime.setText(str(new_seconds))
def thread_stats_stop(self):
"""Stop the Threading and save the data."""
if self.THREAD_STATS.isRunning():
self.THREAD_STATS.terminate()
self.save_stats()
#######################################################
################# THREADING CLASSES ###################
#######################################################
class ProjectStats(QThread):
"""Thread class for Stats updating."""
def __init__(self, parent=None):
QThread.__init__(self, parent)
def run(self):
"""Run the main loop and choose what to send to the main thread"""
while True:
self.emit(SIGNAL('thread_stats_update(QString)'), "1")
sleep(1)
Edit: I forgot to add my parse_seconds method.
def parse_seconds(seconds, reverse=False):
"""Transform seconds into a more valid data
Seconds must be integer for normal mode
Seconds must have <1d, 1h, 1m, 1s> sintax for reverse mode"""
if reverse:
d, h, m, s = seconds.split(", ")
d = int(d[:-1]) * 86400
h = int(h[:-1]) * 3600
m = int(m[:-1]) * 60
s = int(s[:-1])
return s + m + h + d
else:
m, s = divmod(seconds, 60)
h, m = divmod(m, 60)
d, h = divmod(h, 24)
return "0d, 1h, 2m, 3s".format(d, h, m, s)
python python-3.x qt pyside
The title and description leave something to be desired. Is this really about usingQThread
, or are you usingQThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.
– Daniel
Jul 15 at 9:22
2
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23
add a comment |Â
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This is the first time that I try to use threads on any programming language. I am learning PySide2 so I tried there.
Note: I removed from __init__
all the window information related to buttons, lines and UI as I consider this snippet is big enough already.
Please let me know if this is the correct way to create a Qthread
. Also, please let me know if my docstrings are wrong, and how to improve them.
from time import sleep
from PySide2.QtCore import QThread, SIGNAL
from PySide2 import QtWidgets
class MainForm(QtWidgets.QMainWindow, Ui_MainWindow):
"""Main window of the program."""
def __init__(self, parent=None):
QtWidgets.QMainWindow.__init__(self, parent)
self.setupUi(self)
self.thread_stats_start()
######################################################
################# THREADING HANDLERS #################
######################################################
def thread_stats_start(self):
"""Create a secondary thread that deals with stats"""
self.THREAD_STATS = ProjectStats()
self.connect(self.THREAD_STATS, SIGNAL("thread_stats_update(QString)"), self.thread_stats_update)
self.THREAD_STATS.start()
def thread_stats_update(self, value):
"""Update the value when you receive the signal"""
current_seconds = alexfunctions.parse_seconds(self.line_ProjectTime.text(), reverse=True)
new_seconds = alexfunctions.parse_seconds(current_seconds + int(value))
self.line_ProjectTime.setText(str(new_seconds))
def thread_stats_stop(self):
"""Stop the Threading and save the data."""
if self.THREAD_STATS.isRunning():
self.THREAD_STATS.terminate()
self.save_stats()
#######################################################
################# THREADING CLASSES ###################
#######################################################
class ProjectStats(QThread):
"""Thread class for Stats updating."""
def __init__(self, parent=None):
QThread.__init__(self, parent)
def run(self):
"""Run the main loop and choose what to send to the main thread"""
while True:
self.emit(SIGNAL('thread_stats_update(QString)'), "1")
sleep(1)
Edit: I forgot to add my parse_seconds method.
def parse_seconds(seconds, reverse=False):
"""Transform seconds into a more valid data
Seconds must be integer for normal mode
Seconds must have <1d, 1h, 1m, 1s> sintax for reverse mode"""
if reverse:
d, h, m, s = seconds.split(", ")
d = int(d[:-1]) * 86400
h = int(h[:-1]) * 3600
m = int(m[:-1]) * 60
s = int(s[:-1])
return s + m + h + d
else:
m, s = divmod(seconds, 60)
h, m = divmod(m, 60)
d, h = divmod(h, 24)
return "0d, 1h, 2m, 3s".format(d, h, m, s)
python python-3.x qt pyside
This is the first time that I try to use threads on any programming language. I am learning PySide2 so I tried there.
Note: I removed from __init__
all the window information related to buttons, lines and UI as I consider this snippet is big enough already.
Please let me know if this is the correct way to create a Qthread
. Also, please let me know if my docstrings are wrong, and how to improve them.
from time import sleep
from PySide2.QtCore import QThread, SIGNAL
from PySide2 import QtWidgets
class MainForm(QtWidgets.QMainWindow, Ui_MainWindow):
"""Main window of the program."""
def __init__(self, parent=None):
QtWidgets.QMainWindow.__init__(self, parent)
self.setupUi(self)
self.thread_stats_start()
######################################################
################# THREADING HANDLERS #################
######################################################
def thread_stats_start(self):
"""Create a secondary thread that deals with stats"""
self.THREAD_STATS = ProjectStats()
self.connect(self.THREAD_STATS, SIGNAL("thread_stats_update(QString)"), self.thread_stats_update)
self.THREAD_STATS.start()
def thread_stats_update(self, value):
"""Update the value when you receive the signal"""
current_seconds = alexfunctions.parse_seconds(self.line_ProjectTime.text(), reverse=True)
new_seconds = alexfunctions.parse_seconds(current_seconds + int(value))
self.line_ProjectTime.setText(str(new_seconds))
def thread_stats_stop(self):
"""Stop the Threading and save the data."""
if self.THREAD_STATS.isRunning():
self.THREAD_STATS.terminate()
self.save_stats()
#######################################################
################# THREADING CLASSES ###################
#######################################################
class ProjectStats(QThread):
"""Thread class for Stats updating."""
def __init__(self, parent=None):
QThread.__init__(self, parent)
def run(self):
"""Run the main loop and choose what to send to the main thread"""
while True:
self.emit(SIGNAL('thread_stats_update(QString)'), "1")
sleep(1)
Edit: I forgot to add my parse_seconds method.
def parse_seconds(seconds, reverse=False):
"""Transform seconds into a more valid data
Seconds must be integer for normal mode
Seconds must have <1d, 1h, 1m, 1s> sintax for reverse mode"""
if reverse:
d, h, m, s = seconds.split(", ")
d = int(d[:-1]) * 86400
h = int(h[:-1]) * 3600
m = int(m[:-1]) * 60
s = int(s[:-1])
return s + m + h + d
else:
m, s = divmod(seconds, 60)
h, m = divmod(m, 60)
d, h = divmod(h, 24)
return "0d, 1h, 2m, 3s".format(d, h, m, s)
python python-3.x qt pyside
edited Jul 15 at 7:24


Jamal♦
30.1k11114225
30.1k11114225
asked Jul 15 at 4:18


Saelyth
1263
1263
The title and description leave something to be desired. Is this really about usingQThread
, or are you usingQThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.
– Daniel
Jul 15 at 9:22
2
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23
add a comment |Â
The title and description leave something to be desired. Is this really about usingQThread
, or are you usingQThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.
– Daniel
Jul 15 at 9:22
2
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23
The title and description leave something to be desired. Is this really about using
QThread
, or are you using QThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.– Daniel
Jul 15 at 9:22
The title and description leave something to be desired. Is this really about using
QThread
, or are you using QThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.– Daniel
Jul 15 at 9:22
2
2
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f199526%2fmy-first-qthread-with-python-qt%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
The title and description leave something to be desired. Is this really about using
QThread
, or are you usingQThread
as part of a larger program? If it's the former, this is likely off-topic. If it's the latter (which I suspect), please explain what your code does.– Daniel
Jul 15 at 9:22
2
"Note: I removed from init all the window information related to buttons, lines and UI as I consider this snippet is big enough already." Please don't. We prefer to see your whole code untouched. Character limit on posts on code review is greater than on the rest of the stack exchange network for precisely this reason.
– Mathias Ettinger
Jul 15 at 10:32
This is indeed part of a larger program, which is why I also removed 300 lines of code from init. I don't think you guys would benefit from seeing info related to 50 UI items so I just kept it simple. The thread is suppose to just update an specific QlineEdit each second, adding the time of that second.
– Saelyth
Jul 15 at 21:23