Custom caching class in Swift
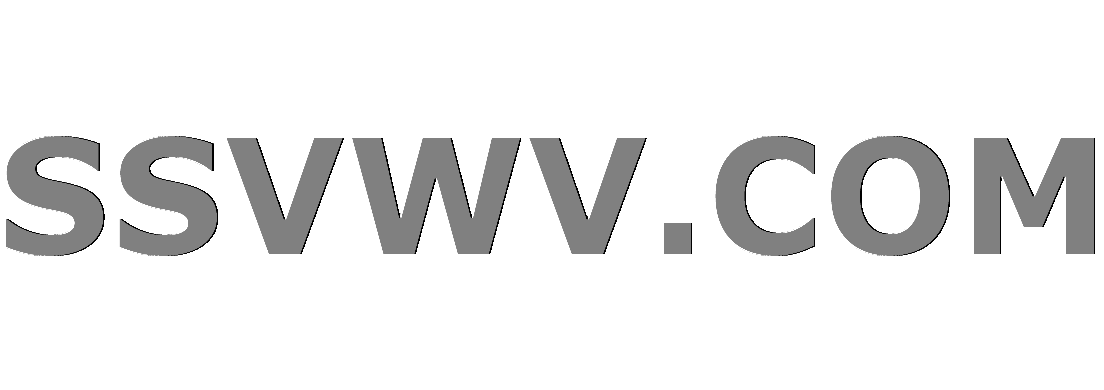
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
I'm using Swift 4.0 and I'm using UserDefaults
in my project for storing Strings like login or something. I have a class:
class Cache: UserDefaultsManager {
static var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID) as? Int
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
static var login: String?
get
return value(forKey: Constants.Cache.Login) as? String
set
set(newValue, forKey: Constants.Cache.Login)
// ... and there are more of this kind
, where UserDefaultsManager
looks like this:
class UserDefaultsManager
private static var uds: UserDefaults
return UserDefaults.standard
static func value(forKey: String) -> Any?
if let encoded = uds.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded)
return nil
static func set(_ data: Any?, forKey: String)
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
uds.set(encodedData, forKey: forKey)
if (!uds.synchronize())
NSLog("Failed sync UserDefaults?")
Constants.Cache
is a structure that holds my keys. My question is:
What would be a good approach to make my
Cache
class lighter with
less code?
I imagine the final product should look like:
class Cache
static var hash: UDSObject<String>?
static var currentProfileID: UDSObject<Int>?
static var login: UDSObject<String>?
static var selectedWindowID: UDSObject<Int>?
swift cache
add a comment |Â
up vote
3
down vote
favorite
I'm using Swift 4.0 and I'm using UserDefaults
in my project for storing Strings like login or something. I have a class:
class Cache: UserDefaultsManager {
static var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID) as? Int
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
static var login: String?
get
return value(forKey: Constants.Cache.Login) as? String
set
set(newValue, forKey: Constants.Cache.Login)
// ... and there are more of this kind
, where UserDefaultsManager
looks like this:
class UserDefaultsManager
private static var uds: UserDefaults
return UserDefaults.standard
static func value(forKey: String) -> Any?
if let encoded = uds.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded)
return nil
static func set(_ data: Any?, forKey: String)
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
uds.set(encodedData, forKey: forKey)
if (!uds.synchronize())
NSLog("Failed sync UserDefaults?")
Constants.Cache
is a structure that holds my keys. My question is:
What would be a good approach to make my
Cache
class lighter with
less code?
I imagine the final product should look like:
class Cache
static var hash: UDSObject<String>?
static var currentProfileID: UDSObject<Int>?
static var login: UDSObject<String>?
static var selectedWindowID: UDSObject<Int>?
swift cache
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I'm using Swift 4.0 and I'm using UserDefaults
in my project for storing Strings like login or something. I have a class:
class Cache: UserDefaultsManager {
static var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID) as? Int
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
static var login: String?
get
return value(forKey: Constants.Cache.Login) as? String
set
set(newValue, forKey: Constants.Cache.Login)
// ... and there are more of this kind
, where UserDefaultsManager
looks like this:
class UserDefaultsManager
private static var uds: UserDefaults
return UserDefaults.standard
static func value(forKey: String) -> Any?
if let encoded = uds.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded)
return nil
static func set(_ data: Any?, forKey: String)
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
uds.set(encodedData, forKey: forKey)
if (!uds.synchronize())
NSLog("Failed sync UserDefaults?")
Constants.Cache
is a structure that holds my keys. My question is:
What would be a good approach to make my
Cache
class lighter with
less code?
I imagine the final product should look like:
class Cache
static var hash: UDSObject<String>?
static var currentProfileID: UDSObject<Int>?
static var login: UDSObject<String>?
static var selectedWindowID: UDSObject<Int>?
swift cache
I'm using Swift 4.0 and I'm using UserDefaults
in my project for storing Strings like login or something. I have a class:
class Cache: UserDefaultsManager {
static var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID) as? Int
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
static var login: String?
get
return value(forKey: Constants.Cache.Login) as? String
set
set(newValue, forKey: Constants.Cache.Login)
// ... and there are more of this kind
, where UserDefaultsManager
looks like this:
class UserDefaultsManager
private static var uds: UserDefaults
return UserDefaults.standard
static func value(forKey: String) -> Any?
if let encoded = uds.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded)
return nil
static func set(_ data: Any?, forKey: String)
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
uds.set(encodedData, forKey: forKey)
if (!uds.synchronize())
NSLog("Failed sync UserDefaults?")
Constants.Cache
is a structure that holds my keys. My question is:
What would be a good approach to make my
Cache
class lighter with
less code?
I imagine the final product should look like:
class Cache
static var hash: UDSObject<String>?
static var currentProfileID: UDSObject<Int>?
static var login: UDSObject<String>?
static var selectedWindowID: UDSObject<Int>?
swift cache
edited Jul 10 at 21:53


200_success
123k14143399
123k14143399
asked Jul 10 at 21:32
Bartosz Woźniak
1185
1185
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Your Cache
class cannot be lighter than it already is. The most you can do is, introduce generics to remove the redundant casting every time you are trying to fetch from user defaults.
However, I see a major problem with the implementation here:
Is Cache also a UserDefaultsManager
? Do you see Cache
as something that extends the functionalities of the UserDefaultsManager
class, or does your Cache
use the functionalities of the UserDefaultsManager
? I feel that composition is the way to go here than inheritance. I'd refactor this code to something like this:
protocol CacheHandling
func value<T>(forKey: String) -> T?
func set<T>(_ data: T?, forKey: String)
extension CacheHandling
func value<T>(forKey: String) -> T?
let userDefaults = UserDefaults.standard
if let encoded = userDefaults.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded) as? T
return nil
func set<T>(_ data: T?, forKey: String)
let userDefaults = UserDefaults.standard
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
userDefaults.set(encodedData, forKey: forKey)
if (!userDefaults.synchronize())
NSLog("Failed sync UserDefaults?")
struct Cache : CacheHandling
var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID)
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
var login: String?
get
return value(forKey: Constants.Cache.Login)
set
set(newValue, forKey: Constants.Cache.Login)
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Your Cache
class cannot be lighter than it already is. The most you can do is, introduce generics to remove the redundant casting every time you are trying to fetch from user defaults.
However, I see a major problem with the implementation here:
Is Cache also a UserDefaultsManager
? Do you see Cache
as something that extends the functionalities of the UserDefaultsManager
class, or does your Cache
use the functionalities of the UserDefaultsManager
? I feel that composition is the way to go here than inheritance. I'd refactor this code to something like this:
protocol CacheHandling
func value<T>(forKey: String) -> T?
func set<T>(_ data: T?, forKey: String)
extension CacheHandling
func value<T>(forKey: String) -> T?
let userDefaults = UserDefaults.standard
if let encoded = userDefaults.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded) as? T
return nil
func set<T>(_ data: T?, forKey: String)
let userDefaults = UserDefaults.standard
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
userDefaults.set(encodedData, forKey: forKey)
if (!userDefaults.synchronize())
NSLog("Failed sync UserDefaults?")
struct Cache : CacheHandling
var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID)
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
var login: String?
get
return value(forKey: Constants.Cache.Login)
set
set(newValue, forKey: Constants.Cache.Login)
add a comment |Â
up vote
2
down vote
accepted
Your Cache
class cannot be lighter than it already is. The most you can do is, introduce generics to remove the redundant casting every time you are trying to fetch from user defaults.
However, I see a major problem with the implementation here:
Is Cache also a UserDefaultsManager
? Do you see Cache
as something that extends the functionalities of the UserDefaultsManager
class, or does your Cache
use the functionalities of the UserDefaultsManager
? I feel that composition is the way to go here than inheritance. I'd refactor this code to something like this:
protocol CacheHandling
func value<T>(forKey: String) -> T?
func set<T>(_ data: T?, forKey: String)
extension CacheHandling
func value<T>(forKey: String) -> T?
let userDefaults = UserDefaults.standard
if let encoded = userDefaults.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded) as? T
return nil
func set<T>(_ data: T?, forKey: String)
let userDefaults = UserDefaults.standard
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
userDefaults.set(encodedData, forKey: forKey)
if (!userDefaults.synchronize())
NSLog("Failed sync UserDefaults?")
struct Cache : CacheHandling
var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID)
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
var login: String?
get
return value(forKey: Constants.Cache.Login)
set
set(newValue, forKey: Constants.Cache.Login)
add a comment |Â
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Your Cache
class cannot be lighter than it already is. The most you can do is, introduce generics to remove the redundant casting every time you are trying to fetch from user defaults.
However, I see a major problem with the implementation here:
Is Cache also a UserDefaultsManager
? Do you see Cache
as something that extends the functionalities of the UserDefaultsManager
class, or does your Cache
use the functionalities of the UserDefaultsManager
? I feel that composition is the way to go here than inheritance. I'd refactor this code to something like this:
protocol CacheHandling
func value<T>(forKey: String) -> T?
func set<T>(_ data: T?, forKey: String)
extension CacheHandling
func value<T>(forKey: String) -> T?
let userDefaults = UserDefaults.standard
if let encoded = userDefaults.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded) as? T
return nil
func set<T>(_ data: T?, forKey: String)
let userDefaults = UserDefaults.standard
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
userDefaults.set(encodedData, forKey: forKey)
if (!userDefaults.synchronize())
NSLog("Failed sync UserDefaults?")
struct Cache : CacheHandling
var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID)
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
var login: String?
get
return value(forKey: Constants.Cache.Login)
set
set(newValue, forKey: Constants.Cache.Login)
Your Cache
class cannot be lighter than it already is. The most you can do is, introduce generics to remove the redundant casting every time you are trying to fetch from user defaults.
However, I see a major problem with the implementation here:
Is Cache also a UserDefaultsManager
? Do you see Cache
as something that extends the functionalities of the UserDefaultsManager
class, or does your Cache
use the functionalities of the UserDefaultsManager
? I feel that composition is the way to go here than inheritance. I'd refactor this code to something like this:
protocol CacheHandling
func value<T>(forKey: String) -> T?
func set<T>(_ data: T?, forKey: String)
extension CacheHandling
func value<T>(forKey: String) -> T?
let userDefaults = UserDefaults.standard
if let encoded = userDefaults.object(forKey: forKey) as? Data
return NSKeyedUnarchiver.unarchiveObject(with: encoded) as? T
return nil
func set<T>(_ data: T?, forKey: String)
let userDefaults = UserDefaults.standard
let encodedData: Data = NSKeyedArchiver.archivedData(withRootObject: data as AnyObject)
userDefaults.set(encodedData, forKey: forKey)
if (!userDefaults.synchronize())
NSLog("Failed sync UserDefaults?")
struct Cache : CacheHandling
var currentProfileID: Int?
get
return value(forKey: Constants.Cache.CurrentProfileID)
set
set(newValue, forKey: Constants.Cache.CurrentProfileID)
var login: String?
get
return value(forKey: Constants.Cache.Login)
set
set(newValue, forKey: Constants.Cache.Login)
edited Jul 11 at 16:05
answered Jul 11 at 11:13
avismara
1365
1365
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f198246%2fcustom-caching-class-in-swift%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password