Fetching data from API in JavaScript
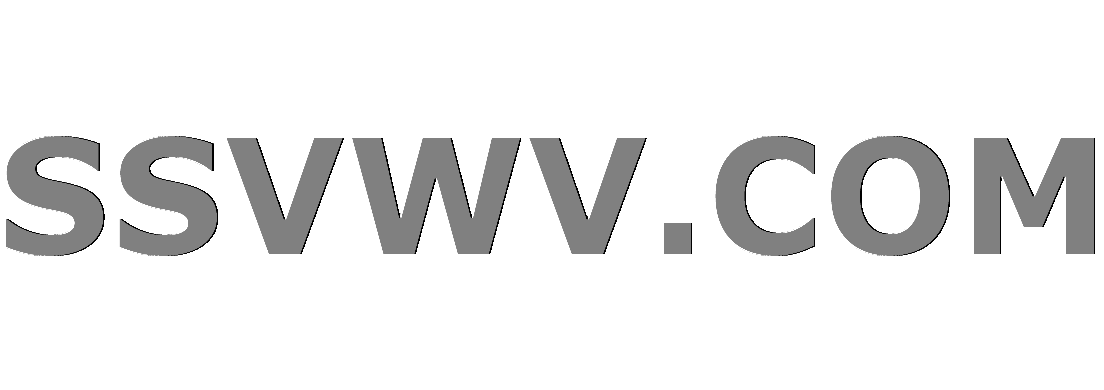
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
I'm using Axios to fetch data from an API, and don't know if this is the best way to deal with APIs in ReactJS or not. I want to know if anyone can suggest edits or something to make the code more efficient.
api.js
import axios from 'axios';
export const APIendpoint = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
matchesAction.js
import FETCH_MATCHES from './actionTypes';
import APIendpoint from '../api/api';
export const fetchMatches = () => dispatch =>
const matchInstance = APIendpoint.get('/matches');
matchInstance.then(response =>
dispatch(
type: FETCH_MATCHES,
payload: response.data
)
);
;
javascript react.js axios
add a comment |Â
up vote
2
down vote
favorite
I'm using Axios to fetch data from an API, and don't know if this is the best way to deal with APIs in ReactJS or not. I want to know if anyone can suggest edits or something to make the code more efficient.
api.js
import axios from 'axios';
export const APIendpoint = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
matchesAction.js
import FETCH_MATCHES from './actionTypes';
import APIendpoint from '../api/api';
export const fetchMatches = () => dispatch =>
const matchInstance = APIendpoint.get('/matches');
matchInstance.then(response =>
dispatch(
type: FETCH_MATCHES,
payload: response.data
)
);
;
javascript react.js axios
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm using Axios to fetch data from an API, and don't know if this is the best way to deal with APIs in ReactJS or not. I want to know if anyone can suggest edits or something to make the code more efficient.
api.js
import axios from 'axios';
export const APIendpoint = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
matchesAction.js
import FETCH_MATCHES from './actionTypes';
import APIendpoint from '../api/api';
export const fetchMatches = () => dispatch =>
const matchInstance = APIendpoint.get('/matches');
matchInstance.then(response =>
dispatch(
type: FETCH_MATCHES,
payload: response.data
)
);
;
javascript react.js axios
I'm using Axios to fetch data from an API, and don't know if this is the best way to deal with APIs in ReactJS or not. I want to know if anyone can suggest edits or something to make the code more efficient.
api.js
import axios from 'axios';
export const APIendpoint = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
matchesAction.js
import FETCH_MATCHES from './actionTypes';
import APIendpoint from '../api/api';
export const fetchMatches = () => dispatch =>
const matchInstance = APIendpoint.get('/matches');
matchInstance.then(response =>
dispatch(
type: FETCH_MATCHES,
payload: response.data
)
);
;
javascript react.js axios
edited Jul 11 at 19:28
asked Jul 11 at 19:20
gretty volk
112
112
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
0
down vote
It can be done in many ways based on your use case & code structure, but i feel this can be optimal way of structuring.
api/services/index.js
import axios from 'axios';
export default as MATCHES_API from "./services/matches.js";
export const CONFIG_API = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
api/services/matches.js
import CONFIG_API from "api/services";
const ERROR_MSG = "<generic message>";
export const matches = () =>
return CONFIG_API.get("/matches").then(res =>
// do some operations on data - if required
return success: true, data: res.payload;
).catch(err =>
return success: false, message: ERROR_MSG ;
);
;
actions/matchesAction.js
import FETCH_MATCHES from './actionTypes';
import MATCHES_API from "/api/services";
export const fetchMatches = () => dispatch =>
matches.then((response) =>
const success = response;
if (success)
dispatch( type: FETCH_MATCHES, payload);
else
dispatch( type: FETCH_MATCHES_FAILED); /* or show notification */ .
);
;
Let me know if this works out.
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It can be done in many ways based on your use case & code structure, but i feel this can be optimal way of structuring.
api/services/index.js
import axios from 'axios';
export default as MATCHES_API from "./services/matches.js";
export const CONFIG_API = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
api/services/matches.js
import CONFIG_API from "api/services";
const ERROR_MSG = "<generic message>";
export const matches = () =>
return CONFIG_API.get("/matches").then(res =>
// do some operations on data - if required
return success: true, data: res.payload;
).catch(err =>
return success: false, message: ERROR_MSG ;
);
;
actions/matchesAction.js
import FETCH_MATCHES from './actionTypes';
import MATCHES_API from "/api/services";
export const fetchMatches = () => dispatch =>
matches.then((response) =>
const success = response;
if (success)
dispatch( type: FETCH_MATCHES, payload);
else
dispatch( type: FETCH_MATCHES_FAILED); /* or show notification */ .
);
;
Let me know if this works out.
add a comment |Â
up vote
0
down vote
It can be done in many ways based on your use case & code structure, but i feel this can be optimal way of structuring.
api/services/index.js
import axios from 'axios';
export default as MATCHES_API from "./services/matches.js";
export const CONFIG_API = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
api/services/matches.js
import CONFIG_API from "api/services";
const ERROR_MSG = "<generic message>";
export const matches = () =>
return CONFIG_API.get("/matches").then(res =>
// do some operations on data - if required
return success: true, data: res.payload;
).catch(err =>
return success: false, message: ERROR_MSG ;
);
;
actions/matchesAction.js
import FETCH_MATCHES from './actionTypes';
import MATCHES_API from "/api/services";
export const fetchMatches = () => dispatch =>
matches.then((response) =>
const success = response;
if (success)
dispatch( type: FETCH_MATCHES, payload);
else
dispatch( type: FETCH_MATCHES_FAILED); /* or show notification */ .
);
;
Let me know if this works out.
add a comment |Â
up vote
0
down vote
up vote
0
down vote
It can be done in many ways based on your use case & code structure, but i feel this can be optimal way of structuring.
api/services/index.js
import axios from 'axios';
export default as MATCHES_API from "./services/matches.js";
export const CONFIG_API = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
api/services/matches.js
import CONFIG_API from "api/services";
const ERROR_MSG = "<generic message>";
export const matches = () =>
return CONFIG_API.get("/matches").then(res =>
// do some operations on data - if required
return success: true, data: res.payload;
).catch(err =>
return success: false, message: ERROR_MSG ;
);
;
actions/matchesAction.js
import FETCH_MATCHES from './actionTypes';
import MATCHES_API from "/api/services";
export const fetchMatches = () => dispatch =>
matches.then((response) =>
const success = response;
if (success)
dispatch( type: FETCH_MATCHES, payload);
else
dispatch( type: FETCH_MATCHES_FAILED); /* or show notification */ .
);
;
Let me know if this works out.
It can be done in many ways based on your use case & code structure, but i feel this can be optimal way of structuring.
api/services/index.js
import axios from 'axios';
export default as MATCHES_API from "./services/matches.js";
export const CONFIG_API = axios.create(
baseURL: 'https://1eb19101-0790-406f-90e0-ee35a2ff2d1f.mock.pstmn.io',
timeout: 2000
);
api/services/matches.js
import CONFIG_API from "api/services";
const ERROR_MSG = "<generic message>";
export const matches = () =>
return CONFIG_API.get("/matches").then(res =>
// do some operations on data - if required
return success: true, data: res.payload;
).catch(err =>
return success: false, message: ERROR_MSG ;
);
;
actions/matchesAction.js
import FETCH_MATCHES from './actionTypes';
import MATCHES_API from "/api/services";
export const fetchMatches = () => dispatch =>
matches.then((response) =>
const success = response;
if (success)
dispatch( type: FETCH_MATCHES, payload);
else
dispatch( type: FETCH_MATCHES_FAILED); /* or show notification */ .
);
;
Let me know if this works out.
answered Jul 15 at 14:49


Sandeep kumar H R
243
243
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f198311%2ffetching-data-from-api-in-javascript%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password