Separating out router and model logic
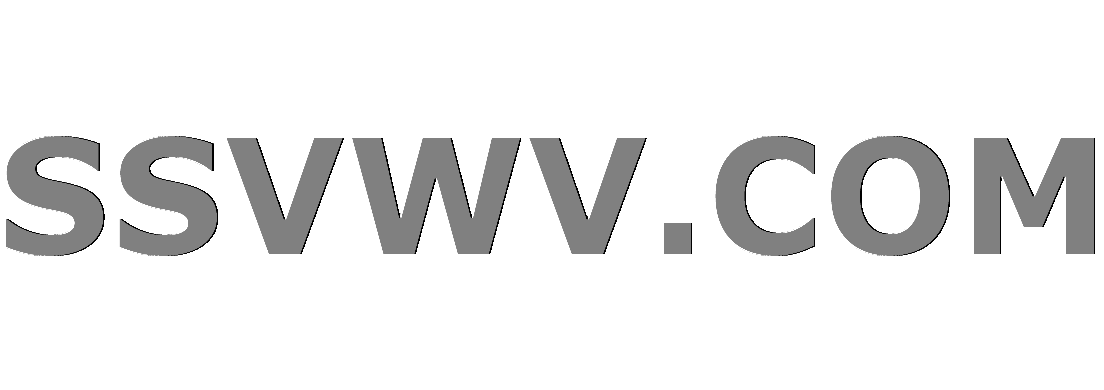
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I have an Express router that is doing all the heavy lifting. Should the router be handling all this stuff or is a layer required between these two like maybe a service that makes these calls?
// Modules
import Router from 'express'
import Passport from 'passport'
import Sequelize from 'sequelize'
import _ from 'lodash'
import JWT from 'jsonwebtoken'
import process from 'process'
import User from '../models/User'
import JwtAuth from '../../config/authStrategy'
const router = new Router()
router.use(Passport.initialize())
JwtAuth(Passport)
router.post('/api/register', (req, res) =>
User.create(req.body)
.then(user =>
res.status(201).json(
success: true,
message: `User $user.name with id $
user.email
successfully created. Please login to continue.`
)
)
.catch(Sequelize.UniqueConstraintError, err =>
res.status(409).json(
success: false,
message: `User with id $err.fields.email already exists!`
)
)
.catch(Sequelize.ValidationError, err =>
const fields = _.capitalize(
err.errors.map(error => error.path).join(', ')
)
res.status(400).json(
success: false,
message: `$fields was not filled correctly. Please retry regestering.`
)
)
)
router.post('/api/login', (req, res) =>
User.findOne( where: email: req.body.email )
.then(user =>
if (user === null)
unsuccessfulLogin(res)
return
user.comparePassword(req.body.password).then(isCorrect =>
if (isCorrect)
let token = JWT.sign(
user.dataValues,
process.env.JWT_SECRET,
expiresIn: 10000
)
res.cookie('token', token)
res.status(303).json(
success: true,
message: 'Login successful',
token: `JWT $token`
)
else
unsuccessfulLogin(res)
)
)
.catch(err =>
console.error(err)
unsuccessfulLogin(res)
)
)
router.post(
'/api/logout',
Passport.authenticate('jwt', session: false ),
(req, res) =>
req.logout()
res.cookie('token', '')
res.json(
success: true,
message: 'Successfully logged out'
)
)
function unsuccessfulLogin(res)
res.status(401).json(
success: false,
message: 'Login failed!'
)
export default router
javascript design-patterns node.js express.js
add a comment |Â
up vote
1
down vote
favorite
I have an Express router that is doing all the heavy lifting. Should the router be handling all this stuff or is a layer required between these two like maybe a service that makes these calls?
// Modules
import Router from 'express'
import Passport from 'passport'
import Sequelize from 'sequelize'
import _ from 'lodash'
import JWT from 'jsonwebtoken'
import process from 'process'
import User from '../models/User'
import JwtAuth from '../../config/authStrategy'
const router = new Router()
router.use(Passport.initialize())
JwtAuth(Passport)
router.post('/api/register', (req, res) =>
User.create(req.body)
.then(user =>
res.status(201).json(
success: true,
message: `User $user.name with id $
user.email
successfully created. Please login to continue.`
)
)
.catch(Sequelize.UniqueConstraintError, err =>
res.status(409).json(
success: false,
message: `User with id $err.fields.email already exists!`
)
)
.catch(Sequelize.ValidationError, err =>
const fields = _.capitalize(
err.errors.map(error => error.path).join(', ')
)
res.status(400).json(
success: false,
message: `$fields was not filled correctly. Please retry regestering.`
)
)
)
router.post('/api/login', (req, res) =>
User.findOne( where: email: req.body.email )
.then(user =>
if (user === null)
unsuccessfulLogin(res)
return
user.comparePassword(req.body.password).then(isCorrect =>
if (isCorrect)
let token = JWT.sign(
user.dataValues,
process.env.JWT_SECRET,
expiresIn: 10000
)
res.cookie('token', token)
res.status(303).json(
success: true,
message: 'Login successful',
token: `JWT $token`
)
else
unsuccessfulLogin(res)
)
)
.catch(err =>
console.error(err)
unsuccessfulLogin(res)
)
)
router.post(
'/api/logout',
Passport.authenticate('jwt', session: false ),
(req, res) =>
req.logout()
res.cookie('token', '')
res.json(
success: true,
message: 'Successfully logged out'
)
)
function unsuccessfulLogin(res)
res.status(401).json(
success: false,
message: 'Login failed!'
)
export default router
javascript design-patterns node.js express.js
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have an Express router that is doing all the heavy lifting. Should the router be handling all this stuff or is a layer required between these two like maybe a service that makes these calls?
// Modules
import Router from 'express'
import Passport from 'passport'
import Sequelize from 'sequelize'
import _ from 'lodash'
import JWT from 'jsonwebtoken'
import process from 'process'
import User from '../models/User'
import JwtAuth from '../../config/authStrategy'
const router = new Router()
router.use(Passport.initialize())
JwtAuth(Passport)
router.post('/api/register', (req, res) =>
User.create(req.body)
.then(user =>
res.status(201).json(
success: true,
message: `User $user.name with id $
user.email
successfully created. Please login to continue.`
)
)
.catch(Sequelize.UniqueConstraintError, err =>
res.status(409).json(
success: false,
message: `User with id $err.fields.email already exists!`
)
)
.catch(Sequelize.ValidationError, err =>
const fields = _.capitalize(
err.errors.map(error => error.path).join(', ')
)
res.status(400).json(
success: false,
message: `$fields was not filled correctly. Please retry regestering.`
)
)
)
router.post('/api/login', (req, res) =>
User.findOne( where: email: req.body.email )
.then(user =>
if (user === null)
unsuccessfulLogin(res)
return
user.comparePassword(req.body.password).then(isCorrect =>
if (isCorrect)
let token = JWT.sign(
user.dataValues,
process.env.JWT_SECRET,
expiresIn: 10000
)
res.cookie('token', token)
res.status(303).json(
success: true,
message: 'Login successful',
token: `JWT $token`
)
else
unsuccessfulLogin(res)
)
)
.catch(err =>
console.error(err)
unsuccessfulLogin(res)
)
)
router.post(
'/api/logout',
Passport.authenticate('jwt', session: false ),
(req, res) =>
req.logout()
res.cookie('token', '')
res.json(
success: true,
message: 'Successfully logged out'
)
)
function unsuccessfulLogin(res)
res.status(401).json(
success: false,
message: 'Login failed!'
)
export default router
javascript design-patterns node.js express.js
I have an Express router that is doing all the heavy lifting. Should the router be handling all this stuff or is a layer required between these two like maybe a service that makes these calls?
// Modules
import Router from 'express'
import Passport from 'passport'
import Sequelize from 'sequelize'
import _ from 'lodash'
import JWT from 'jsonwebtoken'
import process from 'process'
import User from '../models/User'
import JwtAuth from '../../config/authStrategy'
const router = new Router()
router.use(Passport.initialize())
JwtAuth(Passport)
router.post('/api/register', (req, res) =>
User.create(req.body)
.then(user =>
res.status(201).json(
success: true,
message: `User $user.name with id $
user.email
successfully created. Please login to continue.`
)
)
.catch(Sequelize.UniqueConstraintError, err =>
res.status(409).json(
success: false,
message: `User with id $err.fields.email already exists!`
)
)
.catch(Sequelize.ValidationError, err =>
const fields = _.capitalize(
err.errors.map(error => error.path).join(', ')
)
res.status(400).json(
success: false,
message: `$fields was not filled correctly. Please retry regestering.`
)
)
)
router.post('/api/login', (req, res) =>
User.findOne( where: email: req.body.email )
.then(user =>
if (user === null)
unsuccessfulLogin(res)
return
user.comparePassword(req.body.password).then(isCorrect =>
if (isCorrect)
let token = JWT.sign(
user.dataValues,
process.env.JWT_SECRET,
expiresIn: 10000
)
res.cookie('token', token)
res.status(303).json(
success: true,
message: 'Login successful',
token: `JWT $token`
)
else
unsuccessfulLogin(res)
)
)
.catch(err =>
console.error(err)
unsuccessfulLogin(res)
)
)
router.post(
'/api/logout',
Passport.authenticate('jwt', session: false ),
(req, res) =>
req.logout()
res.cookie('token', '')
res.json(
success: true,
message: 'Successfully logged out'
)
)
function unsuccessfulLogin(res)
res.status(401).json(
success: false,
message: 'Login failed!'
)
export default router
javascript design-patterns node.js express.js
edited May 1 at 1:36


Jamal♦
30.1k11114225
30.1k11114225
asked Apr 17 at 4:21
ayushgp
1139
1139
add a comment |Â
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f192256%2fseparating-out-router-and-model-logic%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password