Convert decimal to binary and find maximum consecuitive 1's
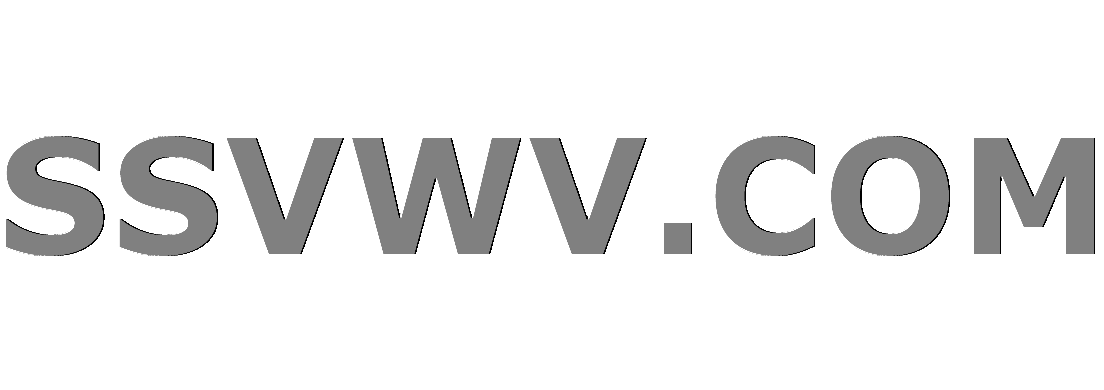
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
-4
down vote
favorite
I am given an integer "n" as an input and must convert it to a binary representation. Then I have to find the maximum number if consecutive 1's in it.
Can you please evaluate the implementation? Any advice on how to achieve better performance?
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class Solution
static void Main(String args)
int n = Convert.ToInt32(Console.ReadLine());
string bin = "";
int conmax = 0;
while (n > 0)
if (n % 2 == 0)
bin += "0";
else
bin += "1";
n = n / 2;
string result = bin.Split('0');
for (int i = 0; i < result.Length; i++)
if (result[i].Length > conmax)
conmax = result[i].Length;
Console.WriteLine(conmax);
c# beginner algorithm
add a comment |Â
up vote
-4
down vote
favorite
I am given an integer "n" as an input and must convert it to a binary representation. Then I have to find the maximum number if consecutive 1's in it.
Can you please evaluate the implementation? Any advice on how to achieve better performance?
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class Solution
static void Main(String args)
int n = Convert.ToInt32(Console.ReadLine());
string bin = "";
int conmax = 0;
while (n > 0)
if (n % 2 == 0)
bin += "0";
else
bin += "1";
n = n / 2;
string result = bin.Split('0');
for (int i = 0; i < result.Length; i++)
if (result[i].Length > conmax)
conmax = result[i].Length;
Console.WriteLine(conmax);
c# beginner algorithm
3
This code does not work in the sense that it creates the binary representation wrong.... for example it converts128
to be00000001
instead of10000000
....
– rolfl♦
Apr 13 at 22:16
add a comment |Â
up vote
-4
down vote
favorite
up vote
-4
down vote
favorite
I am given an integer "n" as an input and must convert it to a binary representation. Then I have to find the maximum number if consecutive 1's in it.
Can you please evaluate the implementation? Any advice on how to achieve better performance?
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class Solution
static void Main(String args)
int n = Convert.ToInt32(Console.ReadLine());
string bin = "";
int conmax = 0;
while (n > 0)
if (n % 2 == 0)
bin += "0";
else
bin += "1";
n = n / 2;
string result = bin.Split('0');
for (int i = 0; i < result.Length; i++)
if (result[i].Length > conmax)
conmax = result[i].Length;
Console.WriteLine(conmax);
c# beginner algorithm
I am given an integer "n" as an input and must convert it to a binary representation. Then I have to find the maximum number if consecutive 1's in it.
Can you please evaluate the implementation? Any advice on how to achieve better performance?
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class Solution
static void Main(String args)
int n = Convert.ToInt32(Console.ReadLine());
string bin = "";
int conmax = 0;
while (n > 0)
if (n % 2 == 0)
bin += "0";
else
bin += "1";
n = n / 2;
string result = bin.Split('0');
for (int i = 0; i < result.Length; i++)
if (result[i].Length > conmax)
conmax = result[i].Length;
Console.WriteLine(conmax);
c# beginner algorithm
asked Apr 13 at 21:45
Nikolay Okhlopkov
12
12
3
This code does not work in the sense that it creates the binary representation wrong.... for example it converts128
to be00000001
instead of10000000
....
– rolfl♦
Apr 13 at 22:16
add a comment |Â
3
This code does not work in the sense that it creates the binary representation wrong.... for example it converts128
to be00000001
instead of10000000
....
– rolfl♦
Apr 13 at 22:16
3
3
This code does not work in the sense that it creates the binary representation wrong.... for example it converts
128
to be 00000001
instead of 10000000
....– rolfl♦
Apr 13 at 22:16
This code does not work in the sense that it creates the binary representation wrong.... for example it converts
128
to be 00000001
instead of 10000000
....– rolfl♦
Apr 13 at 22:16
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
While this implementation works, unless you are purposely reinventing the wheel it is best to take advantage of built in functions.
Converting the input integer to binary can be done with a simple
string bin = Convert.ToString(n, 2);
. As rolfl mentioned in the comments, your implementation is technically incorrect (it still works since you only care about sequential ones) - using built in functions helps avoid this.Instead of writing your own
Max
implementation, you can use LINQ'sMax
.Try to choose more descriptive variable names - presumably
conmax
stands for something likeconsecutiveMax
, but I had to read the rest of your code to make that guess - it wasn't immediately evident. I would probably name the variablemaxConsecutiveOnes
.A good next step for this program is to handle invalid user input - what should happen if I enter
a
when you ask for a number? Right now, the program will crash. This probably isn't desired behavior. You could achieve this by usingInt32.TryParse
and just accept a zero if you receive invalid input or by catching the exception.Don't worry about performance here. With your data types you can have at most 32 consecutive ones. The most optimization that could be done is switching to a bit shifting approach instead of converting the integer to a string. Since this would seriously hurt readability I wouldn't recommend it.
You should remove unnecessary
using
statements to avoid importing code you don't need to. Visual Studio will give you a bunch by default. In this case, you only needusing System;
andusing System.Linq;
.
Here's an alternative solution, using the Int32.TryParse
suggestion to avoid crashes on invalid input. You might want to check the return value and warn the user to provide a better user experience.
using System;
using System.Linq;
class Solution
static void Main(String args)
int input;
Int32.TryParse(Console.ReadLine(), out input);
int maxConsecutiveOnes = Convert.ToString(input, 2)
.Split('0')
.Max(s => s.Length);
Console.WriteLine(maxConsecutiveOnes);
Convert.ToString(input, 2)
- cheater :-P
– t3chb0t
Apr 14 at 13:51
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
While this implementation works, unless you are purposely reinventing the wheel it is best to take advantage of built in functions.
Converting the input integer to binary can be done with a simple
string bin = Convert.ToString(n, 2);
. As rolfl mentioned in the comments, your implementation is technically incorrect (it still works since you only care about sequential ones) - using built in functions helps avoid this.Instead of writing your own
Max
implementation, you can use LINQ'sMax
.Try to choose more descriptive variable names - presumably
conmax
stands for something likeconsecutiveMax
, but I had to read the rest of your code to make that guess - it wasn't immediately evident. I would probably name the variablemaxConsecutiveOnes
.A good next step for this program is to handle invalid user input - what should happen if I enter
a
when you ask for a number? Right now, the program will crash. This probably isn't desired behavior. You could achieve this by usingInt32.TryParse
and just accept a zero if you receive invalid input or by catching the exception.Don't worry about performance here. With your data types you can have at most 32 consecutive ones. The most optimization that could be done is switching to a bit shifting approach instead of converting the integer to a string. Since this would seriously hurt readability I wouldn't recommend it.
You should remove unnecessary
using
statements to avoid importing code you don't need to. Visual Studio will give you a bunch by default. In this case, you only needusing System;
andusing System.Linq;
.
Here's an alternative solution, using the Int32.TryParse
suggestion to avoid crashes on invalid input. You might want to check the return value and warn the user to provide a better user experience.
using System;
using System.Linq;
class Solution
static void Main(String args)
int input;
Int32.TryParse(Console.ReadLine(), out input);
int maxConsecutiveOnes = Convert.ToString(input, 2)
.Split('0')
.Max(s => s.Length);
Console.WriteLine(maxConsecutiveOnes);
Convert.ToString(input, 2)
- cheater :-P
– t3chb0t
Apr 14 at 13:51
add a comment |Â
up vote
2
down vote
accepted
While this implementation works, unless you are purposely reinventing the wheel it is best to take advantage of built in functions.
Converting the input integer to binary can be done with a simple
string bin = Convert.ToString(n, 2);
. As rolfl mentioned in the comments, your implementation is technically incorrect (it still works since you only care about sequential ones) - using built in functions helps avoid this.Instead of writing your own
Max
implementation, you can use LINQ'sMax
.Try to choose more descriptive variable names - presumably
conmax
stands for something likeconsecutiveMax
, but I had to read the rest of your code to make that guess - it wasn't immediately evident. I would probably name the variablemaxConsecutiveOnes
.A good next step for this program is to handle invalid user input - what should happen if I enter
a
when you ask for a number? Right now, the program will crash. This probably isn't desired behavior. You could achieve this by usingInt32.TryParse
and just accept a zero if you receive invalid input or by catching the exception.Don't worry about performance here. With your data types you can have at most 32 consecutive ones. The most optimization that could be done is switching to a bit shifting approach instead of converting the integer to a string. Since this would seriously hurt readability I wouldn't recommend it.
You should remove unnecessary
using
statements to avoid importing code you don't need to. Visual Studio will give you a bunch by default. In this case, you only needusing System;
andusing System.Linq;
.
Here's an alternative solution, using the Int32.TryParse
suggestion to avoid crashes on invalid input. You might want to check the return value and warn the user to provide a better user experience.
using System;
using System.Linq;
class Solution
static void Main(String args)
int input;
Int32.TryParse(Console.ReadLine(), out input);
int maxConsecutiveOnes = Convert.ToString(input, 2)
.Split('0')
.Max(s => s.Length);
Console.WriteLine(maxConsecutiveOnes);
Convert.ToString(input, 2)
- cheater :-P
– t3chb0t
Apr 14 at 13:51
add a comment |Â
up vote
2
down vote
accepted
up vote
2
down vote
accepted
While this implementation works, unless you are purposely reinventing the wheel it is best to take advantage of built in functions.
Converting the input integer to binary can be done with a simple
string bin = Convert.ToString(n, 2);
. As rolfl mentioned in the comments, your implementation is technically incorrect (it still works since you only care about sequential ones) - using built in functions helps avoid this.Instead of writing your own
Max
implementation, you can use LINQ'sMax
.Try to choose more descriptive variable names - presumably
conmax
stands for something likeconsecutiveMax
, but I had to read the rest of your code to make that guess - it wasn't immediately evident. I would probably name the variablemaxConsecutiveOnes
.A good next step for this program is to handle invalid user input - what should happen if I enter
a
when you ask for a number? Right now, the program will crash. This probably isn't desired behavior. You could achieve this by usingInt32.TryParse
and just accept a zero if you receive invalid input or by catching the exception.Don't worry about performance here. With your data types you can have at most 32 consecutive ones. The most optimization that could be done is switching to a bit shifting approach instead of converting the integer to a string. Since this would seriously hurt readability I wouldn't recommend it.
You should remove unnecessary
using
statements to avoid importing code you don't need to. Visual Studio will give you a bunch by default. In this case, you only needusing System;
andusing System.Linq;
.
Here's an alternative solution, using the Int32.TryParse
suggestion to avoid crashes on invalid input. You might want to check the return value and warn the user to provide a better user experience.
using System;
using System.Linq;
class Solution
static void Main(String args)
int input;
Int32.TryParse(Console.ReadLine(), out input);
int maxConsecutiveOnes = Convert.ToString(input, 2)
.Split('0')
.Max(s => s.Length);
Console.WriteLine(maxConsecutiveOnes);
While this implementation works, unless you are purposely reinventing the wheel it is best to take advantage of built in functions.
Converting the input integer to binary can be done with a simple
string bin = Convert.ToString(n, 2);
. As rolfl mentioned in the comments, your implementation is technically incorrect (it still works since you only care about sequential ones) - using built in functions helps avoid this.Instead of writing your own
Max
implementation, you can use LINQ'sMax
.Try to choose more descriptive variable names - presumably
conmax
stands for something likeconsecutiveMax
, but I had to read the rest of your code to make that guess - it wasn't immediately evident. I would probably name the variablemaxConsecutiveOnes
.A good next step for this program is to handle invalid user input - what should happen if I enter
a
when you ask for a number? Right now, the program will crash. This probably isn't desired behavior. You could achieve this by usingInt32.TryParse
and just accept a zero if you receive invalid input or by catching the exception.Don't worry about performance here. With your data types you can have at most 32 consecutive ones. The most optimization that could be done is switching to a bit shifting approach instead of converting the integer to a string. Since this would seriously hurt readability I wouldn't recommend it.
You should remove unnecessary
using
statements to avoid importing code you don't need to. Visual Studio will give you a bunch by default. In this case, you only needusing System;
andusing System.Linq;
.
Here's an alternative solution, using the Int32.TryParse
suggestion to avoid crashes on invalid input. You might want to check the return value and warn the user to provide a better user experience.
using System;
using System.Linq;
class Solution
static void Main(String args)
int input;
Int32.TryParse(Console.ReadLine(), out input);
int maxConsecutiveOnes = Convert.ToString(input, 2)
.Split('0')
.Max(s => s.Length);
Console.WriteLine(maxConsecutiveOnes);
answered Apr 13 at 22:21
Gerrit0
2,6601518
2,6601518
Convert.ToString(input, 2)
- cheater :-P
– t3chb0t
Apr 14 at 13:51
add a comment |Â
Convert.ToString(input, 2)
- cheater :-P
– t3chb0t
Apr 14 at 13:51
Convert.ToString(input, 2)
- cheater :-P– t3chb0t
Apr 14 at 13:51
Convert.ToString(input, 2)
- cheater :-P– t3chb0t
Apr 14 at 13:51
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f192012%2fconvert-decimal-to-binary-and-find-maximum-consecuitive-1s%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
3
This code does not work in the sense that it creates the binary representation wrong.... for example it converts
128
to be00000001
instead of10000000
....– rolfl♦
Apr 13 at 22:16