PHP RESTful API for a student repository
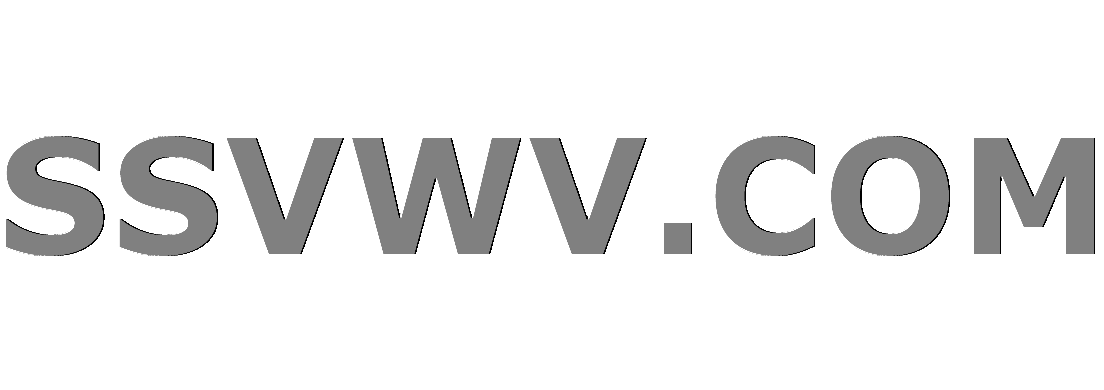
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
All of the codes are based on the PSR-2 standard. Also Dependency-Injection and Repository patterns are followed. I would love to hear some advices or comments.
StudentRepository Class
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct();
public function getStudentNames($request, $response, array $args);
public function getAll($request, $response, array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct()
//Database connection
$dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
$usr = 'root';
$pwd = '';
$this->pdo = new SlimPDODatabase($dsn, $usr, $pwd);
public function getStudentNames($request, $response, array $args)
$array_val = array();
$sql = 'SELECT name FROM Students';
$cntrl=0;
foreach ($this->pdo->query($sql) as $row)
$array_val[$cntrl]=$row["name"];
$cntrl++;
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function getAll($request, $response, array $args)
$array_val = array();
$sql = 'SELECT * FROM Students';
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
StudentController Class
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
return $this->Student_DB->getAll($request, $response, $args);
public function getStudentNames($request, $response, array $args)
return $this->Student_DB->getStudentNames($request, $response, $args);
public function addStudent($request, $response, array $args)
if (array_key_exists("id", $args) && array_key_exists("name", $args)) !ctype_alpha($name))
$n_response = $response->withStatus(400);
return $n_response;
$this->Student_DB->addStudent($id, $name);
$n_response = $response->withStatus(200);
return $n_response;
Edit : I have edited the code in order to return JSON data with spesific status codes.
php api pdo rest slim
add a comment |Â
up vote
3
down vote
favorite
All of the codes are based on the PSR-2 standard. Also Dependency-Injection and Repository patterns are followed. I would love to hear some advices or comments.
StudentRepository Class
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct();
public function getStudentNames($request, $response, array $args);
public function getAll($request, $response, array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct()
//Database connection
$dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
$usr = 'root';
$pwd = '';
$this->pdo = new SlimPDODatabase($dsn, $usr, $pwd);
public function getStudentNames($request, $response, array $args)
$array_val = array();
$sql = 'SELECT name FROM Students';
$cntrl=0;
foreach ($this->pdo->query($sql) as $row)
$array_val[$cntrl]=$row["name"];
$cntrl++;
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function getAll($request, $response, array $args)
$array_val = array();
$sql = 'SELECT * FROM Students';
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
StudentController Class
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
return $this->Student_DB->getAll($request, $response, $args);
public function getStudentNames($request, $response, array $args)
return $this->Student_DB->getStudentNames($request, $response, $args);
public function addStudent($request, $response, array $args)
if (array_key_exists("id", $args) && array_key_exists("name", $args)) !ctype_alpha($name))
$n_response = $response->withStatus(400);
return $n_response;
$this->Student_DB->addStudent($id, $name);
$n_response = $response->withStatus(200);
return $n_response;
Edit : I have edited the code in order to return JSON data with spesific status codes.
php api pdo rest slim
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
All of the codes are based on the PSR-2 standard. Also Dependency-Injection and Repository patterns are followed. I would love to hear some advices or comments.
StudentRepository Class
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct();
public function getStudentNames($request, $response, array $args);
public function getAll($request, $response, array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct()
//Database connection
$dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
$usr = 'root';
$pwd = '';
$this->pdo = new SlimPDODatabase($dsn, $usr, $pwd);
public function getStudentNames($request, $response, array $args)
$array_val = array();
$sql = 'SELECT name FROM Students';
$cntrl=0;
foreach ($this->pdo->query($sql) as $row)
$array_val[$cntrl]=$row["name"];
$cntrl++;
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function getAll($request, $response, array $args)
$array_val = array();
$sql = 'SELECT * FROM Students';
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
StudentController Class
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
return $this->Student_DB->getAll($request, $response, $args);
public function getStudentNames($request, $response, array $args)
return $this->Student_DB->getStudentNames($request, $response, $args);
public function addStudent($request, $response, array $args)
if (array_key_exists("id", $args) && array_key_exists("name", $args)) !ctype_alpha($name))
$n_response = $response->withStatus(400);
return $n_response;
$this->Student_DB->addStudent($id, $name);
$n_response = $response->withStatus(200);
return $n_response;
Edit : I have edited the code in order to return JSON data with spesific status codes.
php api pdo rest slim
All of the codes are based on the PSR-2 standard. Also Dependency-Injection and Repository patterns are followed. I would love to hear some advices or comments.
StudentRepository Class
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct();
public function getStudentNames($request, $response, array $args);
public function getAll($request, $response, array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct()
//Database connection
$dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
$usr = 'root';
$pwd = '';
$this->pdo = new SlimPDODatabase($dsn, $usr, $pwd);
public function getStudentNames($request, $response, array $args)
$array_val = array();
$sql = 'SELECT name FROM Students';
$cntrl=0;
foreach ($this->pdo->query($sql) as $row)
$array_val[$cntrl]=$row["name"];
$cntrl++;
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function getAll($request, $response, array $args)
$array_val = array();
$sql = 'SELECT * FROM Students';
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
$n_response = $response->withJson($array_val, 200);
return $n_response;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
StudentController Class
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
return $this->Student_DB->getAll($request, $response, $args);
public function getStudentNames($request, $response, array $args)
return $this->Student_DB->getStudentNames($request, $response, $args);
public function addStudent($request, $response, array $args)
if (array_key_exists("id", $args) && array_key_exists("name", $args)) !ctype_alpha($name))
$n_response = $response->withStatus(400);
return $n_response;
$this->Student_DB->addStudent($id, $name);
$n_response = $response->withStatus(200);
return $n_response;
Edit : I have edited the code in order to return JSON data with spesific status codes.
php api pdo rest slim
edited Feb 12 at 8:01
asked Feb 7 at 13:45


MeteHan
635
635
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
The StudentRepository is all about database, and shouldn't know about $request and $response objects, that is the job of the controller.
What if you were fetching data to put in an excel file, then you can't use the StudentRepository as it was to fetch that data.
<?php
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct(array $credentials);
public function getStudentNames(array $args);
public function getAll(array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct(array $credentials)
// TODO configuration should be stored outside the class, in a config object or file
// //Database connection
// $dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
// $usr = 'root';
// $pwd = '';
$this->pdo = new SlimPDODatabase($credentials['dsn'], $credentials['usr'], $credentials['pwd']);
public function getStudentNames(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT name FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val=$row["name"];
return $array_val;
public function getAll(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT * FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
return $array_val;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
?>
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
$all = $this->Student_DB->getAll();
return $response->withJson($all, 200);
public function getStudentNames($request, $response, array $args)
$names = $this->Student_DB->getStudentNames();
return $response->withJson($names, 200);
public function addStudent($request, $response, array $args)
// improve tests, as it was possible for $id and $name to be undefined with previous tests
if (!array_key_exists("id", $args)
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
The StudentRepository is all about database, and shouldn't know about $request and $response objects, that is the job of the controller.
What if you were fetching data to put in an excel file, then you can't use the StudentRepository as it was to fetch that data.
<?php
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct(array $credentials);
public function getStudentNames(array $args);
public function getAll(array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct(array $credentials)
// TODO configuration should be stored outside the class, in a config object or file
// //Database connection
// $dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
// $usr = 'root';
// $pwd = '';
$this->pdo = new SlimPDODatabase($credentials['dsn'], $credentials['usr'], $credentials['pwd']);
public function getStudentNames(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT name FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val=$row["name"];
return $array_val;
public function getAll(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT * FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
return $array_val;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
?>
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
$all = $this->Student_DB->getAll();
return $response->withJson($all, 200);
public function getStudentNames($request, $response, array $args)
$names = $this->Student_DB->getStudentNames();
return $response->withJson($names, 200);
public function addStudent($request, $response, array $args)
// improve tests, as it was possible for $id and $name to be undefined with previous tests
if (!array_key_exists("id", $args)
add a comment |Â
up vote
2
down vote
The StudentRepository is all about database, and shouldn't know about $request and $response objects, that is the job of the controller.
What if you were fetching data to put in an excel file, then you can't use the StudentRepository as it was to fetch that data.
<?php
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct(array $credentials);
public function getStudentNames(array $args);
public function getAll(array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct(array $credentials)
// TODO configuration should be stored outside the class, in a config object or file
// //Database connection
// $dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
// $usr = 'root';
// $pwd = '';
$this->pdo = new SlimPDODatabase($credentials['dsn'], $credentials['usr'], $credentials['pwd']);
public function getStudentNames(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT name FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val=$row["name"];
return $array_val;
public function getAll(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT * FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
return $array_val;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
?>
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
$all = $this->Student_DB->getAll();
return $response->withJson($all, 200);
public function getStudentNames($request, $response, array $args)
$names = $this->Student_DB->getStudentNames();
return $response->withJson($names, 200);
public function addStudent($request, $response, array $args)
// improve tests, as it was possible for $id and $name to be undefined with previous tests
if (!array_key_exists("id", $args)
add a comment |Â
up vote
2
down vote
up vote
2
down vote
The StudentRepository is all about database, and shouldn't know about $request and $response objects, that is the job of the controller.
What if you were fetching data to put in an excel file, then you can't use the StudentRepository as it was to fetch that data.
<?php
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct(array $credentials);
public function getStudentNames(array $args);
public function getAll(array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct(array $credentials)
// TODO configuration should be stored outside the class, in a config object or file
// //Database connection
// $dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
// $usr = 'root';
// $pwd = '';
$this->pdo = new SlimPDODatabase($credentials['dsn'], $credentials['usr'], $credentials['pwd']);
public function getStudentNames(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT name FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val=$row["name"];
return $array_val;
public function getAll(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT * FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
return $array_val;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
?>
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
$all = $this->Student_DB->getAll();
return $response->withJson($all, 200);
public function getStudentNames($request, $response, array $args)
$names = $this->Student_DB->getStudentNames();
return $response->withJson($names, 200);
public function addStudent($request, $response, array $args)
// improve tests, as it was possible for $id and $name to be undefined with previous tests
if (!array_key_exists("id", $args)
The StudentRepository is all about database, and shouldn't know about $request and $response objects, that is the job of the controller.
What if you were fetching data to put in an excel file, then you can't use the StudentRepository as it was to fetch that data.
<?php
namespace REST;
use SlimPDODatabase;
interface IStudentRepository
//Functions to be defined
public function __construct(array $credentials);
public function getStudentNames(array $args);
public function getAll(array $args);
public function addStudent($name, $id);
public function updateName($id, $new_name);
class StudentRepository implements IStudentRepository
//Queries and MYSQL connection
protected $pdo;
public function __construct(array $credentials)
// TODO configuration should be stored outside the class, in a config object or file
// //Database connection
// $dsn = 'mysql:host=localhost;dbname=College_DB;charset=utf8';
// $usr = 'root';
// $pwd = '';
$this->pdo = new SlimPDODatabase($credentials['dsn'], $credentials['usr'], $credentials['pwd']);
public function getStudentNames(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT name FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val=$row["name"];
return $array_val;
public function getAll(array $args)
// TODO recommend you use ORDER BY to get a consistent order from request, to request
$sql = 'SELECT * FROM Students';
$array_val = array();
foreach ($this->pdo->query($sql) as $row)
$array_val[$row["id"]]=$row["name"];
return $array_val;
public function addStudent($id, $name)
$stmt = $this->pdo->prepare('INSERT into Students(id,name) values(:id,:namee)');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
public function updateName($id, $new_name)
$stmt = $this->pdo->prepare('UPDATE Students set name=:namee where id=:id');
$stmt->bindParam(":id", $id, SlimPDODatabase::PARAM_STR);
$stmt->bindParam(":namee", $new_name, SlimPDODatabase::PARAM_STR);
$stmt->execute();
?>
<?php
namespace REST;
class StudentController
protected $Student_DB;
public function __construct(IStudentRepository $Student_DB)
$this->Student_DB=$Student_DB;
public function getStudents($request, $response, array $args)
$all = $this->Student_DB->getAll();
return $response->withJson($all, 200);
public function getStudentNames($request, $response, array $args)
$names = $this->Student_DB->getStudentNames();
return $response->withJson($names, 200);
public function addStudent($request, $response, array $args)
// improve tests, as it was possible for $id and $name to be undefined with previous tests
if (!array_key_exists("id", $args)
answered Feb 9 at 7:10
bumperbox
1,800614
1,800614
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f187005%2fphp-restful-api-for-a-student-repository%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password