Manipulating an ES6 static class property that is an array
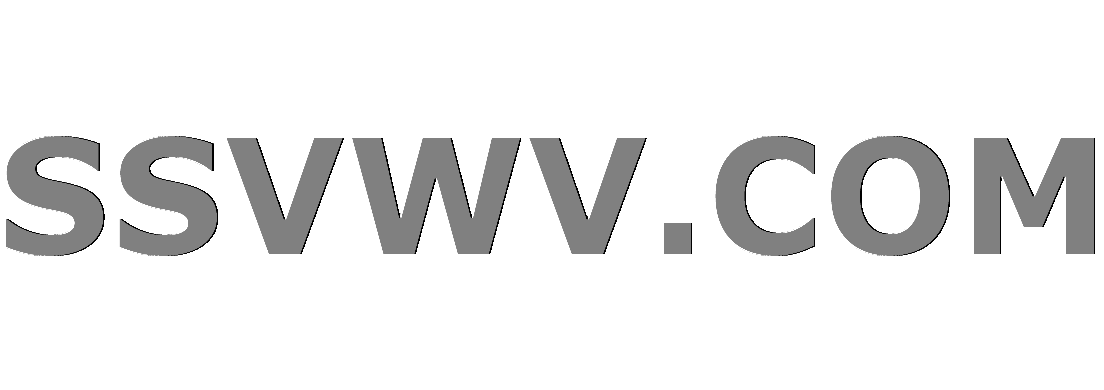
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I've been trying to find a way to create static properties in ES6 classes and have found a solution with the help of various Stack Overflow answers (namely this one and this one).
However, I also wanted to take this a step further and make the static property an array that can be updated/added to using a special static method. I've managed to come up with something that works. Here is a complete demonstration using the class Apple and static property possibleColors:
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
The possibleColors property belongs to the class only and will not be a part of new instances. I've also taken measures to ensure that its value is always an array. It defaults to red, green, & yellow, but you can view/change possible colors using the regular getter/setter:
Apple.possibleColors = ['color', 'othercolor']
You can also add new possible colors like this:
Apple.addPossibleColor('newcolor')
In this example, the whole point of having the possibleColors static property is to have a definitive list of valid colors stored in the main class and prevent any Apple instances from being set to a color not in the array. Of course, I can think of other use cases for this kind of technique.
For example, I'm currently trying to write my own basic component system that uses templates to draw/render each component as HTML on the page. The main class (Component or similar) would have a static property for storing the templates in an array, and you'd choose a template from this array when instantiating a component (or it would choose a default if none specified).
What I'd like to know:
Could this approach cause any problems I haven't foreseen (either in this specific scenario or a more complex one)?
Are there better ways to do anything I've demonstrated here?
Is the way I'm handling static properties overall considered good practice?
javascript object-oriented array ecmascript-6 static
add a comment |Â
up vote
1
down vote
favorite
I've been trying to find a way to create static properties in ES6 classes and have found a solution with the help of various Stack Overflow answers (namely this one and this one).
However, I also wanted to take this a step further and make the static property an array that can be updated/added to using a special static method. I've managed to come up with something that works. Here is a complete demonstration using the class Apple and static property possibleColors:
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
The possibleColors property belongs to the class only and will not be a part of new instances. I've also taken measures to ensure that its value is always an array. It defaults to red, green, & yellow, but you can view/change possible colors using the regular getter/setter:
Apple.possibleColors = ['color', 'othercolor']
You can also add new possible colors like this:
Apple.addPossibleColor('newcolor')
In this example, the whole point of having the possibleColors static property is to have a definitive list of valid colors stored in the main class and prevent any Apple instances from being set to a color not in the array. Of course, I can think of other use cases for this kind of technique.
For example, I'm currently trying to write my own basic component system that uses templates to draw/render each component as HTML on the page. The main class (Component or similar) would have a static property for storing the templates in an array, and you'd choose a template from this array when instantiating a component (or it would choose a default if none specified).
What I'd like to know:
Could this approach cause any problems I haven't foreseen (either in this specific scenario or a more complex one)?
Are there better ways to do anything I've demonstrated here?
Is the way I'm handling static properties overall considered good practice?
javascript object-oriented array ecmascript-6 static
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I've been trying to find a way to create static properties in ES6 classes and have found a solution with the help of various Stack Overflow answers (namely this one and this one).
However, I also wanted to take this a step further and make the static property an array that can be updated/added to using a special static method. I've managed to come up with something that works. Here is a complete demonstration using the class Apple and static property possibleColors:
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
The possibleColors property belongs to the class only and will not be a part of new instances. I've also taken measures to ensure that its value is always an array. It defaults to red, green, & yellow, but you can view/change possible colors using the regular getter/setter:
Apple.possibleColors = ['color', 'othercolor']
You can also add new possible colors like this:
Apple.addPossibleColor('newcolor')
In this example, the whole point of having the possibleColors static property is to have a definitive list of valid colors stored in the main class and prevent any Apple instances from being set to a color not in the array. Of course, I can think of other use cases for this kind of technique.
For example, I'm currently trying to write my own basic component system that uses templates to draw/render each component as HTML on the page. The main class (Component or similar) would have a static property for storing the templates in an array, and you'd choose a template from this array when instantiating a component (or it would choose a default if none specified).
What I'd like to know:
Could this approach cause any problems I haven't foreseen (either in this specific scenario or a more complex one)?
Are there better ways to do anything I've demonstrated here?
Is the way I'm handling static properties overall considered good practice?
javascript object-oriented array ecmascript-6 static
I've been trying to find a way to create static properties in ES6 classes and have found a solution with the help of various Stack Overflow answers (namely this one and this one).
However, I also wanted to take this a step further and make the static property an array that can be updated/added to using a special static method. I've managed to come up with something that works. Here is a complete demonstration using the class Apple and static property possibleColors:
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
The possibleColors property belongs to the class only and will not be a part of new instances. I've also taken measures to ensure that its value is always an array. It defaults to red, green, & yellow, but you can view/change possible colors using the regular getter/setter:
Apple.possibleColors = ['color', 'othercolor']
You can also add new possible colors like this:
Apple.addPossibleColor('newcolor')
In this example, the whole point of having the possibleColors static property is to have a definitive list of valid colors stored in the main class and prevent any Apple instances from being set to a color not in the array. Of course, I can think of other use cases for this kind of technique.
For example, I'm currently trying to write my own basic component system that uses templates to draw/render each component as HTML on the page. The main class (Component or similar) would have a static property for storing the templates in an array, and you'd choose a template from this array when instantiating a component (or it would choose a default if none specified).
What I'd like to know:
Could this approach cause any problems I haven't foreseen (either in this specific scenario or a more complex one)?
Are there better ways to do anything I've demonstrated here?
Is the way I'm handling static properties overall considered good practice?
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
class Apple
constructor(args = )
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
static set possibleColors(arr)
if (Array.isArray(arr))
this._possibleColors = arr;
else
console.log("Possible colors must be an array.")
static addPossibleColor(c)
if (!this._possibleColors)
this._possibleColors = this.possibleColors;
this._possibleColors.push(c);
get color()
if (!this._color)
this._color = '';
return this._color;
set color(c)
if (Apple.possibleColors.includes(c))
this._color = c;
else
console.log(`Color '$c' is not a valid apple color.`);
if (!this._color)
this._color = 'red';
javascript object-oriented array ecmascript-6 static
asked Feb 4 at 23:40
ReeseyCup
234
234
add a comment |Â
add a comment |Â
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
It is a bad idea to change values in in a static context, static basically means that it doesn't change anything
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
You would want to change this value elsewhere. Maybe getting the colors from localStorage
or a database etc. Lets do it with localStorage
I am using CamelCase and Java syntax, then update where it could be improved
class Apple
//If the values are undefined, then it will default to these values
constructor(color = "red", description = "An apple")
this._color = color;
this._description = description;
//getters and setters
get color()
return this._color; // Always has a colour
set color(color) //Don't shorten parameters
if (Apple.possibleColors.includes(color))
this._color = color;
else
console.log(`Color '$c' is not a valid apple color.`);
//Don't set the color if it isn't applicable
//added
get description()
return this._description;
//Static methods
static get possibleColors()
const possibleColors = localStorage.getItem('possibleColors');
if(possibleColors)
return JSON.parse(possibleColors);
return ['red', 'green', 'yellow'];
static set possibleColors(colorArray)
if (Array.isArray(colorArray))
localStorage.setItem('possibleColors', JSON.stringify(colorArray));
else
console.log("Possible colors must be an array.");
static addPossibleColor(color)
const possibleColors = Apple.possibleColors;
possibleColors.push(color);
Apple.possibleColors = possibleColors;
Initiating the class is the same
const greenApple = new Apple(color:"green");
console.log(greenApple.color); //green
console.log(greenApple.description); //An apple
Notice that the getters and setters come before the static methods. Generally they come first, then other methods come afterwards.
Also you should probably have the static methods in another class, because Apple
is feels like a model object that just contains data.
this.color
-> this._color
otherwise it will collide with get/set, you can see in your code that your get thinks the color is undefined
first time get is called
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
add a comment |Â
up vote
0
down vote
Protected states require enforcement..
Why would you use the possible colors and complicated apple color vetting process when there is no requirement in your code to do so?
You are using a static list (array) in an effort to protect the state of the Apple, or associated states that rely on apples being specific colors.
Maintaining trust in object states is important and allows you to make assumptions and reduce the code complexity. It is also one of the most important methods coders have of controlling our arch nemeses.. The Bug!
But a chain is only as strong as its weakest link. Even if you put a big sign above it saying "Don't yank this pitifully weak link!" you can't trust coders, not even yourself, for one day the convenience is too tempting, and while in a rush to meet a dead line you yank that chain.
const myApple = new Apple(); // Needs to be "Reddish" not "red"
myApple._color = "Reddish"; // Done, so much easier than wrangling the
// possibleColors static and its dificult siblings
And just like that all the work put into protecting the state of your apple is out the window.
Coders are lazy they will not put up with complications if there is an easy way. Coders want performant code and they are not going to use getters and setters when there is direct access. Putting a sign up "Do not use" (_
as the metaphoric sign "private") does not stop use.
If you want to control the color of the apple you must make it impossible to change it without the vetting process, or it is pointless, and pointless code is bad code.
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
add a comment |Â
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
It is a bad idea to change values in in a static context, static basically means that it doesn't change anything
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
You would want to change this value elsewhere. Maybe getting the colors from localStorage
or a database etc. Lets do it with localStorage
I am using CamelCase and Java syntax, then update where it could be improved
class Apple
//If the values are undefined, then it will default to these values
constructor(color = "red", description = "An apple")
this._color = color;
this._description = description;
//getters and setters
get color()
return this._color; // Always has a colour
set color(color) //Don't shorten parameters
if (Apple.possibleColors.includes(color))
this._color = color;
else
console.log(`Color '$c' is not a valid apple color.`);
//Don't set the color if it isn't applicable
//added
get description()
return this._description;
//Static methods
static get possibleColors()
const possibleColors = localStorage.getItem('possibleColors');
if(possibleColors)
return JSON.parse(possibleColors);
return ['red', 'green', 'yellow'];
static set possibleColors(colorArray)
if (Array.isArray(colorArray))
localStorage.setItem('possibleColors', JSON.stringify(colorArray));
else
console.log("Possible colors must be an array.");
static addPossibleColor(color)
const possibleColors = Apple.possibleColors;
possibleColors.push(color);
Apple.possibleColors = possibleColors;
Initiating the class is the same
const greenApple = new Apple(color:"green");
console.log(greenApple.color); //green
console.log(greenApple.description); //An apple
Notice that the getters and setters come before the static methods. Generally they come first, then other methods come afterwards.
Also you should probably have the static methods in another class, because Apple
is feels like a model object that just contains data.
this.color
-> this._color
otherwise it will collide with get/set, you can see in your code that your get thinks the color is undefined
first time get is called
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
add a comment |Â
up vote
0
down vote
accepted
It is a bad idea to change values in in a static context, static basically means that it doesn't change anything
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
You would want to change this value elsewhere. Maybe getting the colors from localStorage
or a database etc. Lets do it with localStorage
I am using CamelCase and Java syntax, then update where it could be improved
class Apple
//If the values are undefined, then it will default to these values
constructor(color = "red", description = "An apple")
this._color = color;
this._description = description;
//getters and setters
get color()
return this._color; // Always has a colour
set color(color) //Don't shorten parameters
if (Apple.possibleColors.includes(color))
this._color = color;
else
console.log(`Color '$c' is not a valid apple color.`);
//Don't set the color if it isn't applicable
//added
get description()
return this._description;
//Static methods
static get possibleColors()
const possibleColors = localStorage.getItem('possibleColors');
if(possibleColors)
return JSON.parse(possibleColors);
return ['red', 'green', 'yellow'];
static set possibleColors(colorArray)
if (Array.isArray(colorArray))
localStorage.setItem('possibleColors', JSON.stringify(colorArray));
else
console.log("Possible colors must be an array.");
static addPossibleColor(color)
const possibleColors = Apple.possibleColors;
possibleColors.push(color);
Apple.possibleColors = possibleColors;
Initiating the class is the same
const greenApple = new Apple(color:"green");
console.log(greenApple.color); //green
console.log(greenApple.description); //An apple
Notice that the getters and setters come before the static methods. Generally they come first, then other methods come afterwards.
Also you should probably have the static methods in another class, because Apple
is feels like a model object that just contains data.
this.color
-> this._color
otherwise it will collide with get/set, you can see in your code that your get thinks the color is undefined
first time get is called
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
add a comment |Â
up vote
0
down vote
accepted
up vote
0
down vote
accepted
It is a bad idea to change values in in a static context, static basically means that it doesn't change anything
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
You would want to change this value elsewhere. Maybe getting the colors from localStorage
or a database etc. Lets do it with localStorage
I am using CamelCase and Java syntax, then update where it could be improved
class Apple
//If the values are undefined, then it will default to these values
constructor(color = "red", description = "An apple")
this._color = color;
this._description = description;
//getters and setters
get color()
return this._color; // Always has a colour
set color(color) //Don't shorten parameters
if (Apple.possibleColors.includes(color))
this._color = color;
else
console.log(`Color '$c' is not a valid apple color.`);
//Don't set the color if it isn't applicable
//added
get description()
return this._description;
//Static methods
static get possibleColors()
const possibleColors = localStorage.getItem('possibleColors');
if(possibleColors)
return JSON.parse(possibleColors);
return ['red', 'green', 'yellow'];
static set possibleColors(colorArray)
if (Array.isArray(colorArray))
localStorage.setItem('possibleColors', JSON.stringify(colorArray));
else
console.log("Possible colors must be an array.");
static addPossibleColor(color)
const possibleColors = Apple.possibleColors;
possibleColors.push(color);
Apple.possibleColors = possibleColors;
Initiating the class is the same
const greenApple = new Apple(color:"green");
console.log(greenApple.color); //green
console.log(greenApple.description); //An apple
Notice that the getters and setters come before the static methods. Generally they come first, then other methods come afterwards.
Also you should probably have the static methods in another class, because Apple
is feels like a model object that just contains data.
this.color
-> this._color
otherwise it will collide with get/set, you can see in your code that your get thinks the color is undefined
first time get is called
It is a bad idea to change values in in a static context, static basically means that it doesn't change anything
static get possibleColors()
if (!this._possibleColors)
this._possibleColors = ['red', 'green', 'yellow'];
return this._possibleColors;
You would want to change this value elsewhere. Maybe getting the colors from localStorage
or a database etc. Lets do it with localStorage
I am using CamelCase and Java syntax, then update where it could be improved
class Apple
//If the values are undefined, then it will default to these values
constructor(color = "red", description = "An apple")
this._color = color;
this._description = description;
//getters and setters
get color()
return this._color; // Always has a colour
set color(color) //Don't shorten parameters
if (Apple.possibleColors.includes(color))
this._color = color;
else
console.log(`Color '$c' is not a valid apple color.`);
//Don't set the color if it isn't applicable
//added
get description()
return this._description;
//Static methods
static get possibleColors()
const possibleColors = localStorage.getItem('possibleColors');
if(possibleColors)
return JSON.parse(possibleColors);
return ['red', 'green', 'yellow'];
static set possibleColors(colorArray)
if (Array.isArray(colorArray))
localStorage.setItem('possibleColors', JSON.stringify(colorArray));
else
console.log("Possible colors must be an array.");
static addPossibleColor(color)
const possibleColors = Apple.possibleColors;
possibleColors.push(color);
Apple.possibleColors = possibleColors;
Initiating the class is the same
const greenApple = new Apple(color:"green");
console.log(greenApple.color); //green
console.log(greenApple.description); //An apple
Notice that the getters and setters come before the static methods. Generally they come first, then other methods come afterwards.
Also you should probably have the static methods in another class, because Apple
is feels like a model object that just contains data.
this.color
-> this._color
otherwise it will collide with get/set, you can see in your code that your get thinks the color is undefined
first time get is called
edited Feb 5 at 3:09
answered Feb 5 at 2:54
Pavlo
3689
3689
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
add a comment |Â
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
Thanks for the suggestions. The localStorage idea is very interesting. It sounds like what I'm trying to accomplish would best be done outside the class after all. "It is a bad idea to change values in in a static context, static basically means that it doesn't change anything." I do have to ask: what, then, is the point of even having static getters and setters in the language?
– ReeseyCup
Feb 6 at 2:40
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
I've never seen static getters and setters. Static is usually just a method that doesn't depend on anything from the outside, and it can't change anything on the outside either. JavaScript is a bit lala in this area, but if you use a strongly typed language, then you will see the linter going crazy
– Pavlo
Feb 6 at 3:07
add a comment |Â
up vote
0
down vote
Protected states require enforcement..
Why would you use the possible colors and complicated apple color vetting process when there is no requirement in your code to do so?
You are using a static list (array) in an effort to protect the state of the Apple, or associated states that rely on apples being specific colors.
Maintaining trust in object states is important and allows you to make assumptions and reduce the code complexity. It is also one of the most important methods coders have of controlling our arch nemeses.. The Bug!
But a chain is only as strong as its weakest link. Even if you put a big sign above it saying "Don't yank this pitifully weak link!" you can't trust coders, not even yourself, for one day the convenience is too tempting, and while in a rush to meet a dead line you yank that chain.
const myApple = new Apple(); // Needs to be "Reddish" not "red"
myApple._color = "Reddish"; // Done, so much easier than wrangling the
// possibleColors static and its dificult siblings
And just like that all the work put into protecting the state of your apple is out the window.
Coders are lazy they will not put up with complications if there is an easy way. Coders want performant code and they are not going to use getters and setters when there is direct access. Putting a sign up "Do not use" (_
as the metaphoric sign "private") does not stop use.
If you want to control the color of the apple you must make it impossible to change it without the vetting process, or it is pointless, and pointless code is bad code.
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
add a comment |Â
up vote
0
down vote
Protected states require enforcement..
Why would you use the possible colors and complicated apple color vetting process when there is no requirement in your code to do so?
You are using a static list (array) in an effort to protect the state of the Apple, or associated states that rely on apples being specific colors.
Maintaining trust in object states is important and allows you to make assumptions and reduce the code complexity. It is also one of the most important methods coders have of controlling our arch nemeses.. The Bug!
But a chain is only as strong as its weakest link. Even if you put a big sign above it saying "Don't yank this pitifully weak link!" you can't trust coders, not even yourself, for one day the convenience is too tempting, and while in a rush to meet a dead line you yank that chain.
const myApple = new Apple(); // Needs to be "Reddish" not "red"
myApple._color = "Reddish"; // Done, so much easier than wrangling the
// possibleColors static and its dificult siblings
And just like that all the work put into protecting the state of your apple is out the window.
Coders are lazy they will not put up with complications if there is an easy way. Coders want performant code and they are not going to use getters and setters when there is direct access. Putting a sign up "Do not use" (_
as the metaphoric sign "private") does not stop use.
If you want to control the color of the apple you must make it impossible to change it without the vetting process, or it is pointless, and pointless code is bad code.
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
add a comment |Â
up vote
0
down vote
up vote
0
down vote
Protected states require enforcement..
Why would you use the possible colors and complicated apple color vetting process when there is no requirement in your code to do so?
You are using a static list (array) in an effort to protect the state of the Apple, or associated states that rely on apples being specific colors.
Maintaining trust in object states is important and allows you to make assumptions and reduce the code complexity. It is also one of the most important methods coders have of controlling our arch nemeses.. The Bug!
But a chain is only as strong as its weakest link. Even if you put a big sign above it saying "Don't yank this pitifully weak link!" you can't trust coders, not even yourself, for one day the convenience is too tempting, and while in a rush to meet a dead line you yank that chain.
const myApple = new Apple(); // Needs to be "Reddish" not "red"
myApple._color = "Reddish"; // Done, so much easier than wrangling the
// possibleColors static and its dificult siblings
And just like that all the work put into protecting the state of your apple is out the window.
Coders are lazy they will not put up with complications if there is an easy way. Coders want performant code and they are not going to use getters and setters when there is direct access. Putting a sign up "Do not use" (_
as the metaphoric sign "private") does not stop use.
If you want to control the color of the apple you must make it impossible to change it without the vetting process, or it is pointless, and pointless code is bad code.
Protected states require enforcement..
Why would you use the possible colors and complicated apple color vetting process when there is no requirement in your code to do so?
You are using a static list (array) in an effort to protect the state of the Apple, or associated states that rely on apples being specific colors.
Maintaining trust in object states is important and allows you to make assumptions and reduce the code complexity. It is also one of the most important methods coders have of controlling our arch nemeses.. The Bug!
But a chain is only as strong as its weakest link. Even if you put a big sign above it saying "Don't yank this pitifully weak link!" you can't trust coders, not even yourself, for one day the convenience is too tempting, and while in a rush to meet a dead line you yank that chain.
const myApple = new Apple(); // Needs to be "Reddish" not "red"
myApple._color = "Reddish"; // Done, so much easier than wrangling the
// possibleColors static and its dificult siblings
And just like that all the work put into protecting the state of your apple is out the window.
Coders are lazy they will not put up with complications if there is an easy way. Coders want performant code and they are not going to use getters and setters when there is direct access. Putting a sign up "Do not use" (_
as the metaphoric sign "private") does not stop use.
If you want to control the color of the apple you must make it impossible to change it without the vetting process, or it is pointless, and pointless code is bad code.
answered Feb 5 at 3:53


Blindman67
5,3701320
5,3701320
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
add a comment |Â
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
Thanks for the insight. It's a good point that you can "cheat" by setting the actual property directly. Is there any way you can suggest to enforce this kind of protection? From what I understand, immutability isn't really possible in JavaScript without a library. As I mentioned to @Pavlo, it makes me wonder why static setters are in the language to begin with.
– ReeseyCup
Feb 6 at 2:47
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f186772%2fmanipulating-an-es6-static-class-property-that-is-an-array%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password