Calculating linux based octal file permission
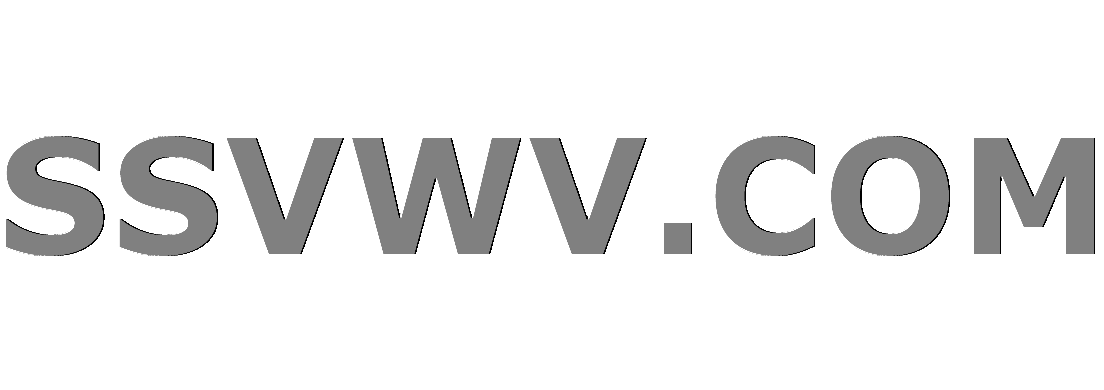
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
I'm applying for a mid level devloper position.
In order to progress to 2nd interview, you need to pass a technical test.
One of the questions was to write a function to work out linux based octal file permissions.
How would you guys improve it, and why?
using System;
using System.Collections;
using System.Linq;
public class ReadWriteExecute
public static int CalculatePermissionSum(string permString)
int octalPerm = 0;
foreach (char permission in permString.ToArray())
switch (permission)
case 'r':
octalPerm += 4;
break;
case 'w':
octalPerm += 2;
break;
case 'x':
octalPerm += 1;
break;
case '-':
octalPerm += 0;
break;
return octalPerm;
public static string SymbolicToOctal(string permString)
string octalPerm = string.Empty;
for(int x=0; x<=6;x+=3)
octalPerm += CalculatePermissionSum(new string(permString.Skip(x).Take(3).ToArray())).ToString();
return octalPerm;
public static void Main(string args)
// Should write 752
Console.WriteLine(ReadWriteExecute.SymbolicToOctal("rwx-x--r-"));
c# interview-questions bitwise posix
add a comment |Â
up vote
3
down vote
favorite
I'm applying for a mid level devloper position.
In order to progress to 2nd interview, you need to pass a technical test.
One of the questions was to write a function to work out linux based octal file permissions.
How would you guys improve it, and why?
using System;
using System.Collections;
using System.Linq;
public class ReadWriteExecute
public static int CalculatePermissionSum(string permString)
int octalPerm = 0;
foreach (char permission in permString.ToArray())
switch (permission)
case 'r':
octalPerm += 4;
break;
case 'w':
octalPerm += 2;
break;
case 'x':
octalPerm += 1;
break;
case '-':
octalPerm += 0;
break;
return octalPerm;
public static string SymbolicToOctal(string permString)
string octalPerm = string.Empty;
for(int x=0; x<=6;x+=3)
octalPerm += CalculatePermissionSum(new string(permString.Skip(x).Take(3).ToArray())).ToString();
return octalPerm;
public static void Main(string args)
// Should write 752
Console.WriteLine(ReadWriteExecute.SymbolicToOctal("rwx-x--r-"));
c# interview-questions bitwise posix
4
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I'm applying for a mid level devloper position.
In order to progress to 2nd interview, you need to pass a technical test.
One of the questions was to write a function to work out linux based octal file permissions.
How would you guys improve it, and why?
using System;
using System.Collections;
using System.Linq;
public class ReadWriteExecute
public static int CalculatePermissionSum(string permString)
int octalPerm = 0;
foreach (char permission in permString.ToArray())
switch (permission)
case 'r':
octalPerm += 4;
break;
case 'w':
octalPerm += 2;
break;
case 'x':
octalPerm += 1;
break;
case '-':
octalPerm += 0;
break;
return octalPerm;
public static string SymbolicToOctal(string permString)
string octalPerm = string.Empty;
for(int x=0; x<=6;x+=3)
octalPerm += CalculatePermissionSum(new string(permString.Skip(x).Take(3).ToArray())).ToString();
return octalPerm;
public static void Main(string args)
// Should write 752
Console.WriteLine(ReadWriteExecute.SymbolicToOctal("rwx-x--r-"));
c# interview-questions bitwise posix
I'm applying for a mid level devloper position.
In order to progress to 2nd interview, you need to pass a technical test.
One of the questions was to write a function to work out linux based octal file permissions.
How would you guys improve it, and why?
using System;
using System.Collections;
using System.Linq;
public class ReadWriteExecute
public static int CalculatePermissionSum(string permString)
int octalPerm = 0;
foreach (char permission in permString.ToArray())
switch (permission)
case 'r':
octalPerm += 4;
break;
case 'w':
octalPerm += 2;
break;
case 'x':
octalPerm += 1;
break;
case '-':
octalPerm += 0;
break;
return octalPerm;
public static string SymbolicToOctal(string permString)
string octalPerm = string.Empty;
for(int x=0; x<=6;x+=3)
octalPerm += CalculatePermissionSum(new string(permString.Skip(x).Take(3).ToArray())).ToString();
return octalPerm;
public static void Main(string args)
// Should write 752
Console.WriteLine(ReadWriteExecute.SymbolicToOctal("rwx-x--r-"));
c# interview-questions bitwise posix
edited Feb 7 at 4:03


200_success
123k14143401
123k14143401
asked Feb 7 at 0:30
Ageis
1161
1161
4
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05
add a comment |Â
4
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05
4
4
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05
add a comment |Â
3 Answers
3
active
oldest
votes
up vote
2
down vote
My thoughts for improvement:
You don't need to run ToArray() on your string to loop through it. Plus, it seems like CalculatePermissionSum() is expecting a single permission string like "rwx" or "r-x", so in a fixed-length situation like this (where the flags are also in a predefined order), don't bother with a loop.
int octalPerm = 0;
octalPerm += (permString[0] == 'r') ? 4 : 0;
octalPerm += (permString[1] == 'w') ? 2 : 0;
octalPerm += (permString[2] == 'x') ? 1 : 0;
return octalPerm;SymbolicToOctal doesn't account for permission representations where the first character is a non-permission flag, like a directory "drwx-x--r-"
There's no error-checking in case an unexpected value comes through. If I ran SymbolicToOctal("Hello World"), I should really get an exception of some kind.
I disagree with the previous comment about using regular expressions to validate the input. Validation is good, but regular expressions would add a (relatively) huge amount of overhead for such simple validation. Code that works on file permissions isn't likely to be run a couple times here and there - it's more likely to be used as part of something that might run a LOT, so every inefficiency will add up. If you validate, just check the length and the expected individual characters. It might be more code compared to a regex, but it'll run a LOT faster.
If I was an interviewer, I would give a LOT of brownie points to the interviewee who threw in some unit-testing code.
add a comment |Â
up vote
2
down vote
I find in general your implementation is a good start because it separates splitting the string into batches from calculating the octal value. You are not doing it in a single method.
Using a switch
is also not such a bad idea here but it lacks the case-insensivity that should be achieved with char.ToUpperInvariant
If your application is unaffected by the current culture and depends on the case of a character changing in a predictable way, use the ToUpperInvariant method. The ToUpperInvariant method is equivalent to ToUpper(Char, CultureInfo.InvariantCulture).
You can push the separation of concerns a little bit further and extract two utility methods here.
The first one would be an extension that splits a collection into batches:
public static IEnumerable<IList<T>> Split<T>(this IEnumerable<T> source, int batchLength)
var batch = new List<T>();
foreach (var item in source)
batch.Add(item);
if (batch.Count == batchLength)
yield return batch;
batch = new List<T>();
and the second one would convert char
into its octal value:
public static int ToOctal(this char value)
switch (char.ToUpperInvariant(value))
case 'R': return 4;
case 'W': return 2;
case 'X': return 1;
case '-': return 0;
default: throw new ArgumentOutOfRangeException(paramName: nameof(value), message: "Value must be: R, W, X or -");
Now you have two specialized methods that you can easily test.
You achieve the final result by combining the above two methods and LINQ into a third one:
public static IEnumerable<int> CalcPermissions(this string value)
const int batchLength = 3;
if (value.Length % batchLength != 0)
throw new ArgumentException(paramName: nameof(value), message: $"Value length must be divisible by batchLength.");
return
from tripple in value.Split(batchLength)
select tripple.Select(c => c.ToOctal()).Sum();
Three methods is what I would expect as an answer to this interview-question. Whether you should use a switch
, a dictionary, regex or array indexing is an entirely different topic that would need proper benchmarks - if performance should be taken into account.
To me the most important thing is that you can separate a larger problem into smaller and testable ones. At this point it doesn't really matter how you implement the Split
or ToOctal
. As long as they are separate methods you can test other implementations later without changing the final result and without having to worry about breaking other functionalities because there is only one at a time you are working on.
add a comment |Â
up vote
1
down vote
I would not be impressed with this submission. Here are some of my notes:
- Way too many data type conversations. You're converting from string to array and back several times.
- No validation that the value is correct. I think you actually have an error in the permission string in your example. The format is well-known and you could use a regular expression.
- Since you'll be validating with a regular expression, you can get rid of the
skip(x)
take(3)
stuff. Just useSubstring()
. I wouldn't necessarily knock this point for an interview task, but I'd be more impressed with a separate class dealing with file permission strings:
PermissionString permissionString = new PermissionString(permString);
return permissionString.ToOctal();
add a comment |Â
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
My thoughts for improvement:
You don't need to run ToArray() on your string to loop through it. Plus, it seems like CalculatePermissionSum() is expecting a single permission string like "rwx" or "r-x", so in a fixed-length situation like this (where the flags are also in a predefined order), don't bother with a loop.
int octalPerm = 0;
octalPerm += (permString[0] == 'r') ? 4 : 0;
octalPerm += (permString[1] == 'w') ? 2 : 0;
octalPerm += (permString[2] == 'x') ? 1 : 0;
return octalPerm;SymbolicToOctal doesn't account for permission representations where the first character is a non-permission flag, like a directory "drwx-x--r-"
There's no error-checking in case an unexpected value comes through. If I ran SymbolicToOctal("Hello World"), I should really get an exception of some kind.
I disagree with the previous comment about using regular expressions to validate the input. Validation is good, but regular expressions would add a (relatively) huge amount of overhead for such simple validation. Code that works on file permissions isn't likely to be run a couple times here and there - it's more likely to be used as part of something that might run a LOT, so every inefficiency will add up. If you validate, just check the length and the expected individual characters. It might be more code compared to a regex, but it'll run a LOT faster.
If I was an interviewer, I would give a LOT of brownie points to the interviewee who threw in some unit-testing code.
add a comment |Â
up vote
2
down vote
My thoughts for improvement:
You don't need to run ToArray() on your string to loop through it. Plus, it seems like CalculatePermissionSum() is expecting a single permission string like "rwx" or "r-x", so in a fixed-length situation like this (where the flags are also in a predefined order), don't bother with a loop.
int octalPerm = 0;
octalPerm += (permString[0] == 'r') ? 4 : 0;
octalPerm += (permString[1] == 'w') ? 2 : 0;
octalPerm += (permString[2] == 'x') ? 1 : 0;
return octalPerm;SymbolicToOctal doesn't account for permission representations where the first character is a non-permission flag, like a directory "drwx-x--r-"
There's no error-checking in case an unexpected value comes through. If I ran SymbolicToOctal("Hello World"), I should really get an exception of some kind.
I disagree with the previous comment about using regular expressions to validate the input. Validation is good, but regular expressions would add a (relatively) huge amount of overhead for such simple validation. Code that works on file permissions isn't likely to be run a couple times here and there - it's more likely to be used as part of something that might run a LOT, so every inefficiency will add up. If you validate, just check the length and the expected individual characters. It might be more code compared to a regex, but it'll run a LOT faster.
If I was an interviewer, I would give a LOT of brownie points to the interviewee who threw in some unit-testing code.
add a comment |Â
up vote
2
down vote
up vote
2
down vote
My thoughts for improvement:
You don't need to run ToArray() on your string to loop through it. Plus, it seems like CalculatePermissionSum() is expecting a single permission string like "rwx" or "r-x", so in a fixed-length situation like this (where the flags are also in a predefined order), don't bother with a loop.
int octalPerm = 0;
octalPerm += (permString[0] == 'r') ? 4 : 0;
octalPerm += (permString[1] == 'w') ? 2 : 0;
octalPerm += (permString[2] == 'x') ? 1 : 0;
return octalPerm;SymbolicToOctal doesn't account for permission representations where the first character is a non-permission flag, like a directory "drwx-x--r-"
There's no error-checking in case an unexpected value comes through. If I ran SymbolicToOctal("Hello World"), I should really get an exception of some kind.
I disagree with the previous comment about using regular expressions to validate the input. Validation is good, but regular expressions would add a (relatively) huge amount of overhead for such simple validation. Code that works on file permissions isn't likely to be run a couple times here and there - it's more likely to be used as part of something that might run a LOT, so every inefficiency will add up. If you validate, just check the length and the expected individual characters. It might be more code compared to a regex, but it'll run a LOT faster.
If I was an interviewer, I would give a LOT of brownie points to the interviewee who threw in some unit-testing code.
My thoughts for improvement:
You don't need to run ToArray() on your string to loop through it. Plus, it seems like CalculatePermissionSum() is expecting a single permission string like "rwx" or "r-x", so in a fixed-length situation like this (where the flags are also in a predefined order), don't bother with a loop.
int octalPerm = 0;
octalPerm += (permString[0] == 'r') ? 4 : 0;
octalPerm += (permString[1] == 'w') ? 2 : 0;
octalPerm += (permString[2] == 'x') ? 1 : 0;
return octalPerm;SymbolicToOctal doesn't account for permission representations where the first character is a non-permission flag, like a directory "drwx-x--r-"
There's no error-checking in case an unexpected value comes through. If I ran SymbolicToOctal("Hello World"), I should really get an exception of some kind.
I disagree with the previous comment about using regular expressions to validate the input. Validation is good, but regular expressions would add a (relatively) huge amount of overhead for such simple validation. Code that works on file permissions isn't likely to be run a couple times here and there - it's more likely to be used as part of something that might run a LOT, so every inefficiency will add up. If you validate, just check the length and the expected individual characters. It might be more code compared to a regex, but it'll run a LOT faster.
If I was an interviewer, I would give a LOT of brownie points to the interviewee who threw in some unit-testing code.
answered Feb 8 at 6:18
jhilgeman
1614
1614
add a comment |Â
add a comment |Â
up vote
2
down vote
I find in general your implementation is a good start because it separates splitting the string into batches from calculating the octal value. You are not doing it in a single method.
Using a switch
is also not such a bad idea here but it lacks the case-insensivity that should be achieved with char.ToUpperInvariant
If your application is unaffected by the current culture and depends on the case of a character changing in a predictable way, use the ToUpperInvariant method. The ToUpperInvariant method is equivalent to ToUpper(Char, CultureInfo.InvariantCulture).
You can push the separation of concerns a little bit further and extract two utility methods here.
The first one would be an extension that splits a collection into batches:
public static IEnumerable<IList<T>> Split<T>(this IEnumerable<T> source, int batchLength)
var batch = new List<T>();
foreach (var item in source)
batch.Add(item);
if (batch.Count == batchLength)
yield return batch;
batch = new List<T>();
and the second one would convert char
into its octal value:
public static int ToOctal(this char value)
switch (char.ToUpperInvariant(value))
case 'R': return 4;
case 'W': return 2;
case 'X': return 1;
case '-': return 0;
default: throw new ArgumentOutOfRangeException(paramName: nameof(value), message: "Value must be: R, W, X or -");
Now you have two specialized methods that you can easily test.
You achieve the final result by combining the above two methods and LINQ into a third one:
public static IEnumerable<int> CalcPermissions(this string value)
const int batchLength = 3;
if (value.Length % batchLength != 0)
throw new ArgumentException(paramName: nameof(value), message: $"Value length must be divisible by batchLength.");
return
from tripple in value.Split(batchLength)
select tripple.Select(c => c.ToOctal()).Sum();
Three methods is what I would expect as an answer to this interview-question. Whether you should use a switch
, a dictionary, regex or array indexing is an entirely different topic that would need proper benchmarks - if performance should be taken into account.
To me the most important thing is that you can separate a larger problem into smaller and testable ones. At this point it doesn't really matter how you implement the Split
or ToOctal
. As long as they are separate methods you can test other implementations later without changing the final result and without having to worry about breaking other functionalities because there is only one at a time you are working on.
add a comment |Â
up vote
2
down vote
I find in general your implementation is a good start because it separates splitting the string into batches from calculating the octal value. You are not doing it in a single method.
Using a switch
is also not such a bad idea here but it lacks the case-insensivity that should be achieved with char.ToUpperInvariant
If your application is unaffected by the current culture and depends on the case of a character changing in a predictable way, use the ToUpperInvariant method. The ToUpperInvariant method is equivalent to ToUpper(Char, CultureInfo.InvariantCulture).
You can push the separation of concerns a little bit further and extract two utility methods here.
The first one would be an extension that splits a collection into batches:
public static IEnumerable<IList<T>> Split<T>(this IEnumerable<T> source, int batchLength)
var batch = new List<T>();
foreach (var item in source)
batch.Add(item);
if (batch.Count == batchLength)
yield return batch;
batch = new List<T>();
and the second one would convert char
into its octal value:
public static int ToOctal(this char value)
switch (char.ToUpperInvariant(value))
case 'R': return 4;
case 'W': return 2;
case 'X': return 1;
case '-': return 0;
default: throw new ArgumentOutOfRangeException(paramName: nameof(value), message: "Value must be: R, W, X or -");
Now you have two specialized methods that you can easily test.
You achieve the final result by combining the above two methods and LINQ into a third one:
public static IEnumerable<int> CalcPermissions(this string value)
const int batchLength = 3;
if (value.Length % batchLength != 0)
throw new ArgumentException(paramName: nameof(value), message: $"Value length must be divisible by batchLength.");
return
from tripple in value.Split(batchLength)
select tripple.Select(c => c.ToOctal()).Sum();
Three methods is what I would expect as an answer to this interview-question. Whether you should use a switch
, a dictionary, regex or array indexing is an entirely different topic that would need proper benchmarks - if performance should be taken into account.
To me the most important thing is that you can separate a larger problem into smaller and testable ones. At this point it doesn't really matter how you implement the Split
or ToOctal
. As long as they are separate methods you can test other implementations later without changing the final result and without having to worry about breaking other functionalities because there is only one at a time you are working on.
add a comment |Â
up vote
2
down vote
up vote
2
down vote
I find in general your implementation is a good start because it separates splitting the string into batches from calculating the octal value. You are not doing it in a single method.
Using a switch
is also not such a bad idea here but it lacks the case-insensivity that should be achieved with char.ToUpperInvariant
If your application is unaffected by the current culture and depends on the case of a character changing in a predictable way, use the ToUpperInvariant method. The ToUpperInvariant method is equivalent to ToUpper(Char, CultureInfo.InvariantCulture).
You can push the separation of concerns a little bit further and extract two utility methods here.
The first one would be an extension that splits a collection into batches:
public static IEnumerable<IList<T>> Split<T>(this IEnumerable<T> source, int batchLength)
var batch = new List<T>();
foreach (var item in source)
batch.Add(item);
if (batch.Count == batchLength)
yield return batch;
batch = new List<T>();
and the second one would convert char
into its octal value:
public static int ToOctal(this char value)
switch (char.ToUpperInvariant(value))
case 'R': return 4;
case 'W': return 2;
case 'X': return 1;
case '-': return 0;
default: throw new ArgumentOutOfRangeException(paramName: nameof(value), message: "Value must be: R, W, X or -");
Now you have two specialized methods that you can easily test.
You achieve the final result by combining the above two methods and LINQ into a third one:
public static IEnumerable<int> CalcPermissions(this string value)
const int batchLength = 3;
if (value.Length % batchLength != 0)
throw new ArgumentException(paramName: nameof(value), message: $"Value length must be divisible by batchLength.");
return
from tripple in value.Split(batchLength)
select tripple.Select(c => c.ToOctal()).Sum();
Three methods is what I would expect as an answer to this interview-question. Whether you should use a switch
, a dictionary, regex or array indexing is an entirely different topic that would need proper benchmarks - if performance should be taken into account.
To me the most important thing is that you can separate a larger problem into smaller and testable ones. At this point it doesn't really matter how you implement the Split
or ToOctal
. As long as they are separate methods you can test other implementations later without changing the final result and without having to worry about breaking other functionalities because there is only one at a time you are working on.
I find in general your implementation is a good start because it separates splitting the string into batches from calculating the octal value. You are not doing it in a single method.
Using a switch
is also not such a bad idea here but it lacks the case-insensivity that should be achieved with char.ToUpperInvariant
If your application is unaffected by the current culture and depends on the case of a character changing in a predictable way, use the ToUpperInvariant method. The ToUpperInvariant method is equivalent to ToUpper(Char, CultureInfo.InvariantCulture).
You can push the separation of concerns a little bit further and extract two utility methods here.
The first one would be an extension that splits a collection into batches:
public static IEnumerable<IList<T>> Split<T>(this IEnumerable<T> source, int batchLength)
var batch = new List<T>();
foreach (var item in source)
batch.Add(item);
if (batch.Count == batchLength)
yield return batch;
batch = new List<T>();
and the second one would convert char
into its octal value:
public static int ToOctal(this char value)
switch (char.ToUpperInvariant(value))
case 'R': return 4;
case 'W': return 2;
case 'X': return 1;
case '-': return 0;
default: throw new ArgumentOutOfRangeException(paramName: nameof(value), message: "Value must be: R, W, X or -");
Now you have two specialized methods that you can easily test.
You achieve the final result by combining the above two methods and LINQ into a third one:
public static IEnumerable<int> CalcPermissions(this string value)
const int batchLength = 3;
if (value.Length % batchLength != 0)
throw new ArgumentException(paramName: nameof(value), message: $"Value length must be divisible by batchLength.");
return
from tripple in value.Split(batchLength)
select tripple.Select(c => c.ToOctal()).Sum();
Three methods is what I would expect as an answer to this interview-question. Whether you should use a switch
, a dictionary, regex or array indexing is an entirely different topic that would need proper benchmarks - if performance should be taken into account.
To me the most important thing is that you can separate a larger problem into smaller and testable ones. At this point it doesn't really matter how you implement the Split
or ToOctal
. As long as they are separate methods you can test other implementations later without changing the final result and without having to worry about breaking other functionalities because there is only one at a time you are working on.
edited Feb 8 at 15:57


Sam Onela
5,88461545
5,88461545
answered Feb 8 at 8:03


t3chb0t
32.1k54195
32.1k54195
add a comment |Â
add a comment |Â
up vote
1
down vote
I would not be impressed with this submission. Here are some of my notes:
- Way too many data type conversations. You're converting from string to array and back several times.
- No validation that the value is correct. I think you actually have an error in the permission string in your example. The format is well-known and you could use a regular expression.
- Since you'll be validating with a regular expression, you can get rid of the
skip(x)
take(3)
stuff. Just useSubstring()
. I wouldn't necessarily knock this point for an interview task, but I'd be more impressed with a separate class dealing with file permission strings:
PermissionString permissionString = new PermissionString(permString);
return permissionString.ToOctal();
add a comment |Â
up vote
1
down vote
I would not be impressed with this submission. Here are some of my notes:
- Way too many data type conversations. You're converting from string to array and back several times.
- No validation that the value is correct. I think you actually have an error in the permission string in your example. The format is well-known and you could use a regular expression.
- Since you'll be validating with a regular expression, you can get rid of the
skip(x)
take(3)
stuff. Just useSubstring()
. I wouldn't necessarily knock this point for an interview task, but I'd be more impressed with a separate class dealing with file permission strings:
PermissionString permissionString = new PermissionString(permString);
return permissionString.ToOctal();
add a comment |Â
up vote
1
down vote
up vote
1
down vote
I would not be impressed with this submission. Here are some of my notes:
- Way too many data type conversations. You're converting from string to array and back several times.
- No validation that the value is correct. I think you actually have an error in the permission string in your example. The format is well-known and you could use a regular expression.
- Since you'll be validating with a regular expression, you can get rid of the
skip(x)
take(3)
stuff. Just useSubstring()
. I wouldn't necessarily knock this point for an interview task, but I'd be more impressed with a separate class dealing with file permission strings:
PermissionString permissionString = new PermissionString(permString);
return permissionString.ToOctal();
I would not be impressed with this submission. Here are some of my notes:
- Way too many data type conversations. You're converting from string to array and back several times.
- No validation that the value is correct. I think you actually have an error in the permission string in your example. The format is well-known and you could use a regular expression.
- Since you'll be validating with a regular expression, you can get rid of the
skip(x)
take(3)
stuff. Just useSubstring()
. I wouldn't necessarily knock this point for an interview task, but I'd be more impressed with a separate class dealing with file permission strings:
PermissionString permissionString = new PermissionString(permString);
return permissionString.ToOctal();
edited Feb 8 at 1:31


Jamal♦
30.1k11114225
30.1k11114225
answered Feb 8 at 1:12
AndrewR
1112
1112
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f186960%2fcalculating-linux-based-octal-file-permission%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
4
Have you gotten feedback from your interviewers about your solution yet?
– 200_success
Feb 7 at 4:05