PHP function to extract numbers from the list whose squares are exceed some threshold
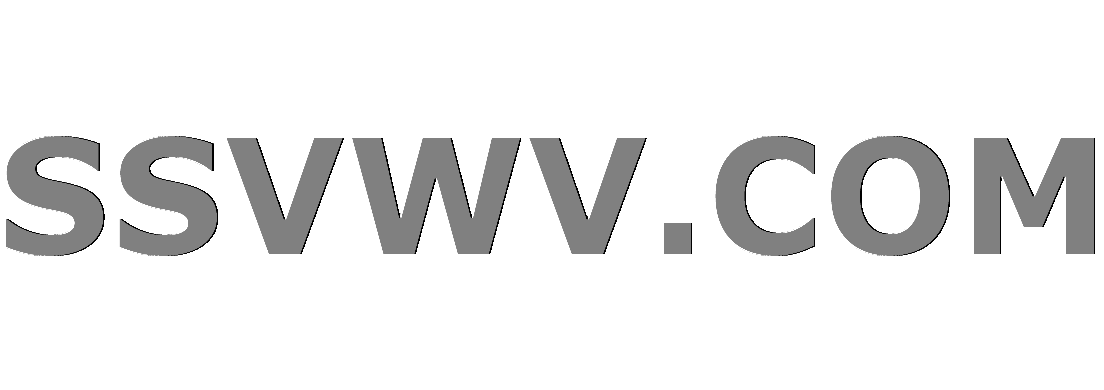
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
This is my naive approach:
$sample1 = [3, -20, 1, -19, -4, 6, 77, -1, 0, 6];
$sample3 = [4, 5, -7, 0, 2, 44, -3, -5, 66, -7];
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
$sample2 = ;
for ($i = 0; $i < mt_rand(10, 30); $i++)
$sample2 = mt_rand(-1000, 1000);
extractNumbersThatSquaresAreGreaterThan($sample1);
extractNumbersThatSquaresAreGreaterThan($sample2);
extractNumbersThatSquaresAreGreaterThan($sample3);
extractNumbersThatSquaresAreGreaterThan($sample4);
var_dump($sample1);
//var_dump($sample2);
var_dump($sample3);
var_dump($sample4);
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
foreach ($list as $key => $value)
if (pow($value, 2) <= $boundary)
unset($list[$key]);
Any hints for optimization? Is it possible, I've tried few, but in the end it was more complicated (and slower) solution
php algorithm array
add a comment |Â
up vote
1
down vote
favorite
This is my naive approach:
$sample1 = [3, -20, 1, -19, -4, 6, 77, -1, 0, 6];
$sample3 = [4, 5, -7, 0, 2, 44, -3, -5, 66, -7];
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
$sample2 = ;
for ($i = 0; $i < mt_rand(10, 30); $i++)
$sample2 = mt_rand(-1000, 1000);
extractNumbersThatSquaresAreGreaterThan($sample1);
extractNumbersThatSquaresAreGreaterThan($sample2);
extractNumbersThatSquaresAreGreaterThan($sample3);
extractNumbersThatSquaresAreGreaterThan($sample4);
var_dump($sample1);
//var_dump($sample2);
var_dump($sample3);
var_dump($sample4);
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
foreach ($list as $key => $value)
if (pow($value, 2) <= $boundary)
unset($list[$key]);
Any hints for optimization? Is it possible, I've tried few, but in the end it was more complicated (and slower) solution
php algorithm array
Please fix the code
– Your Common Sense
Jun 26 at 12:39
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
This is my naive approach:
$sample1 = [3, -20, 1, -19, -4, 6, 77, -1, 0, 6];
$sample3 = [4, 5, -7, 0, 2, 44, -3, -5, 66, -7];
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
$sample2 = ;
for ($i = 0; $i < mt_rand(10, 30); $i++)
$sample2 = mt_rand(-1000, 1000);
extractNumbersThatSquaresAreGreaterThan($sample1);
extractNumbersThatSquaresAreGreaterThan($sample2);
extractNumbersThatSquaresAreGreaterThan($sample3);
extractNumbersThatSquaresAreGreaterThan($sample4);
var_dump($sample1);
//var_dump($sample2);
var_dump($sample3);
var_dump($sample4);
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
foreach ($list as $key => $value)
if (pow($value, 2) <= $boundary)
unset($list[$key]);
Any hints for optimization? Is it possible, I've tried few, but in the end it was more complicated (and slower) solution
php algorithm array
This is my naive approach:
$sample1 = [3, -20, 1, -19, -4, 6, 77, -1, 0, 6];
$sample3 = [4, 5, -7, 0, 2, 44, -3, -5, 66, -7];
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
$sample2 = ;
for ($i = 0; $i < mt_rand(10, 30); $i++)
$sample2 = mt_rand(-1000, 1000);
extractNumbersThatSquaresAreGreaterThan($sample1);
extractNumbersThatSquaresAreGreaterThan($sample2);
extractNumbersThatSquaresAreGreaterThan($sample3);
extractNumbersThatSquaresAreGreaterThan($sample4);
var_dump($sample1);
//var_dump($sample2);
var_dump($sample3);
var_dump($sample4);
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
foreach ($list as $key => $value)
if (pow($value, 2) <= $boundary)
unset($list[$key]);
Any hints for optimization? Is it possible, I've tried few, but in the end it was more complicated (and slower) solution
php algorithm array
edited Jun 26 at 21:09


200_success
123k14143399
123k14143399
asked Jun 26 at 12:00
drupality
1236
1236
Please fix the code
– Your Common Sense
Jun 26 at 12:39
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13
add a comment |Â
Please fix the code
– Your Common Sense
Jun 26 at 12:39
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13
Please fix the code
– Your Common Sense
Jun 26 at 12:39
Please fix the code
– Your Common Sense
Jun 26 at 12:39
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13
add a comment |Â
3 Answers
3
active
oldest
votes
up vote
2
down vote
accepted
Hints? Ok.
Don’t square the values, O(n), instead square-root the boundary. You’ll need an
or
in your test, though.Don’t
unset
values in the list. That requires shifting values in memory. Make a new list of same size, fill in values, without gaps, and then resize result.
add a comment |Â
up vote
1
down vote
I don't know about how fast this solution will be, but anyway I suggest to use functional approach to solve your problem. At least you will have concise code:
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
var_dump(extractNumbers($sample4, 26));
function extractNumbers($list, $boundary)
return array_filter($list, function($x) use ($boundary)
return ($x * $x) > $boundary;
);
add a comment |Â
up vote
1
down vote
Instead of remove items with unset
, I would use array_filter
. And in fact, you could factor out the pow
function, if you use basic maths.
$$
num^2 leq boundary\
implies |num| leq sqrtboundary
$$
combining this would result in
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
$list = array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
In addition, I would avoid the use of referenes for this task and just return your value.
function extractNumbersThatSquaresAreGreaterThan($list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
return array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
add a comment |Â
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Hints? Ok.
Don’t square the values, O(n), instead square-root the boundary. You’ll need an
or
in your test, though.Don’t
unset
values in the list. That requires shifting values in memory. Make a new list of same size, fill in values, without gaps, and then resize result.
add a comment |Â
up vote
2
down vote
accepted
Hints? Ok.
Don’t square the values, O(n), instead square-root the boundary. You’ll need an
or
in your test, though.Don’t
unset
values in the list. That requires shifting values in memory. Make a new list of same size, fill in values, without gaps, and then resize result.
add a comment |Â
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Hints? Ok.
Don’t square the values, O(n), instead square-root the boundary. You’ll need an
or
in your test, though.Don’t
unset
values in the list. That requires shifting values in memory. Make a new list of same size, fill in values, without gaps, and then resize result.
Hints? Ok.
Don’t square the values, O(n), instead square-root the boundary. You’ll need an
or
in your test, though.Don’t
unset
values in the list. That requires shifting values in memory. Make a new list of same size, fill in values, without gaps, and then resize result.
answered Jun 26 at 13:38
AJNeufeld
1,378312
1,378312
add a comment |Â
add a comment |Â
up vote
1
down vote
I don't know about how fast this solution will be, but anyway I suggest to use functional approach to solve your problem. At least you will have concise code:
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
var_dump(extractNumbers($sample4, 26));
function extractNumbers($list, $boundary)
return array_filter($list, function($x) use ($boundary)
return ($x * $x) > $boundary;
);
add a comment |Â
up vote
1
down vote
I don't know about how fast this solution will be, but anyway I suggest to use functional approach to solve your problem. At least you will have concise code:
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
var_dump(extractNumbers($sample4, 26));
function extractNumbers($list, $boundary)
return array_filter($list, function($x) use ($boundary)
return ($x * $x) > $boundary;
);
add a comment |Â
up vote
1
down vote
up vote
1
down vote
I don't know about how fast this solution will be, but anyway I suggest to use functional approach to solve your problem. At least you will have concise code:
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
var_dump(extractNumbers($sample4, 26));
function extractNumbers($list, $boundary)
return array_filter($list, function($x) use ($boundary)
return ($x * $x) > $boundary;
);
I don't know about how fast this solution will be, but anyway I suggest to use functional approach to solve your problem. At least you will have concise code:
$sample4 = [4, 25, 1, 6, 9, 5, 999, 4, 43, 2];
var_dump(extractNumbers($sample4, 26));
function extractNumbers($list, $boundary)
return array_filter($list, function($x) use ($boundary)
return ($x * $x) > $boundary;
);
edited Jun 26 at 21:02
answered Jun 26 at 20:22


Stexxe
3067
3067
add a comment |Â
add a comment |Â
up vote
1
down vote
Instead of remove items with unset
, I would use array_filter
. And in fact, you could factor out the pow
function, if you use basic maths.
$$
num^2 leq boundary\
implies |num| leq sqrtboundary
$$
combining this would result in
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
$list = array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
In addition, I would avoid the use of referenes for this task and just return your value.
function extractNumbersThatSquaresAreGreaterThan($list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
return array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
add a comment |Â
up vote
1
down vote
Instead of remove items with unset
, I would use array_filter
. And in fact, you could factor out the pow
function, if you use basic maths.
$$
num^2 leq boundary\
implies |num| leq sqrtboundary
$$
combining this would result in
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
$list = array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
In addition, I would avoid the use of referenes for this task and just return your value.
function extractNumbersThatSquaresAreGreaterThan($list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
return array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
add a comment |Â
up vote
1
down vote
up vote
1
down vote
Instead of remove items with unset
, I would use array_filter
. And in fact, you could factor out the pow
function, if you use basic maths.
$$
num^2 leq boundary\
implies |num| leq sqrtboundary
$$
combining this would result in
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
$list = array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
In addition, I would avoid the use of referenes for this task and just return your value.
function extractNumbersThatSquaresAreGreaterThan($list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
return array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
Instead of remove items with unset
, I would use array_filter
. And in fact, you could factor out the pow
function, if you use basic maths.
$$
num^2 leq boundary\
implies |num| leq sqrtboundary
$$
combining this would result in
function extractNumbersThatSquaresAreGreaterThan(&$list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
$list = array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
In addition, I would avoid the use of referenes for this task and just return your value.
function extractNumbersThatSquaresAreGreaterThan($list, $boundary = 26)
$sqrt_boundary = sqrt($boundary);
return array_filter($list, function ($item) use ($sqrt_boundary)
return abs($item) > $sqrt_boundary;
);
answered Jun 29 at 10:00


Philipp
1112
1112
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
add a comment |Â
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
Thanks a lot for this. Especially reminding me, that something like ABS exists ;)
– drupality
Jun 30 at 19:18
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f197276%2fphp-function-to-extract-numbers-from-the-list-whose-squares-are-exceed-some-thre%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Please fix the code
– Your Common Sense
Jun 26 at 12:39
@YourCommonSense please provide me more test data, than I'll fix
– drupality
Jun 26 at 13:13