Find minimum depth in a binary tree
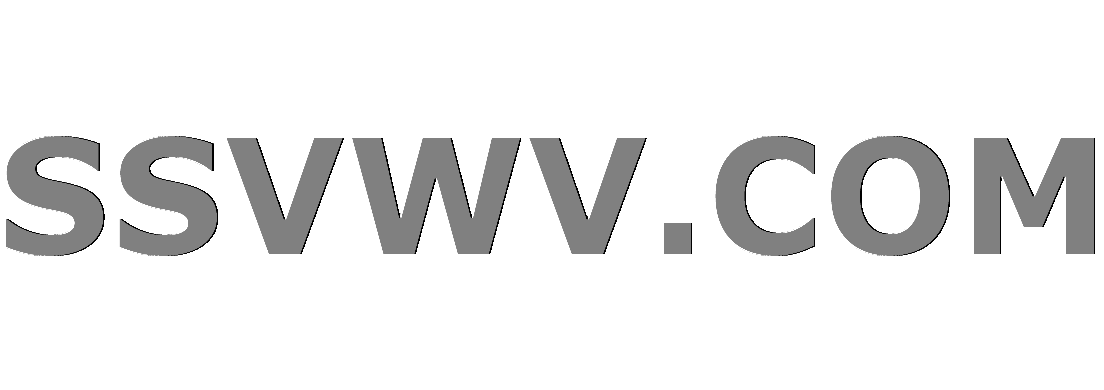
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
Description:
Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Leetcode
Code:
class Solution
public int minDepth(TreeNode root)
if (root == null) return 0;
return recur(root, 1);
private int recur(TreeNode root, int depth)
Question:
I need to know if the given logic can be simplified?
java algorithm recursion tree interview-questions
add a comment |Â
up vote
3
down vote
favorite
Description:
Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Leetcode
Code:
class Solution
public int minDepth(TreeNode root)
if (root == null) return 0;
return recur(root, 1);
private int recur(TreeNode root, int depth)
Question:
I need to know if the given logic can be simplified?
java algorithm recursion tree interview-questions
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
Description:
Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Leetcode
Code:
class Solution
public int minDepth(TreeNode root)
if (root == null) return 0;
return recur(root, 1);
private int recur(TreeNode root, int depth)
Question:
I need to know if the given logic can be simplified?
java algorithm recursion tree interview-questions
Description:
Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Leetcode
Code:
class Solution
public int minDepth(TreeNode root)
if (root == null) return 0;
return recur(root, 1);
private int recur(TreeNode root, int depth)
Question:
I need to know if the given logic can be simplified?
java algorithm recursion tree interview-questions
asked Jun 21 at 19:21


CodeYogi
1,99932060
1,99932060
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
If by simplification you mean optimization, then only one thing came to my mind:
Whenever you find a leaf node, remember its depth in
minmalFound
variable.When again you hit the next leaf node you compare its depth with previously remembered node. And save the minimum of both into
minmalFound
.
And the main logic to add is whenever you are searching a certain path and find out that the depth becomes as great as the existing minmalFound
, then you stop searching that path.
Here is code to demonstrate this technique:
public class Solution
public static int minDepth(TreeNode root)
reccallscount = 0;
minmalFound = -1;
if (root == null)
return 0;
return recur(root, 1);
public static boolean doFaster = false;
private static int minmalFound = -1;
private static int reccallscount = 0;
private static int recur(TreeNode root, int depth)
public static void main(String args)
TreeNode root = new TreeNode();
root.left = new TreeNode();
root.left.right = new TreeNode();
root.right = new TreeNode();
root.right.left = new TreeNode();
root.right.left.right = new TreeNode();
root.right.left.right.left = new TreeNode();
Solution.doFaster = false;
int min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls");
Solution.doFaster = true;
min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls with faster approach");
class TreeNode
TreeNode left = null;
TreeNode right = null;
In the code above, I created a tree with left side depth = 3 and right side depth = 5. I ran minDepth()
twice; first using your algorithm, and again with my addition. The result is then printed:
Minimum 3 found with 11 recursive calls
Minimum 3 found with 5 recursive calls with faster approach
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
If by simplification you mean optimization, then only one thing came to my mind:
Whenever you find a leaf node, remember its depth in
minmalFound
variable.When again you hit the next leaf node you compare its depth with previously remembered node. And save the minimum of both into
minmalFound
.
And the main logic to add is whenever you are searching a certain path and find out that the depth becomes as great as the existing minmalFound
, then you stop searching that path.
Here is code to demonstrate this technique:
public class Solution
public static int minDepth(TreeNode root)
reccallscount = 0;
minmalFound = -1;
if (root == null)
return 0;
return recur(root, 1);
public static boolean doFaster = false;
private static int minmalFound = -1;
private static int reccallscount = 0;
private static int recur(TreeNode root, int depth)
public static void main(String args)
TreeNode root = new TreeNode();
root.left = new TreeNode();
root.left.right = new TreeNode();
root.right = new TreeNode();
root.right.left = new TreeNode();
root.right.left.right = new TreeNode();
root.right.left.right.left = new TreeNode();
Solution.doFaster = false;
int min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls");
Solution.doFaster = true;
min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls with faster approach");
class TreeNode
TreeNode left = null;
TreeNode right = null;
In the code above, I created a tree with left side depth = 3 and right side depth = 5. I ran minDepth()
twice; first using your algorithm, and again with my addition. The result is then printed:
Minimum 3 found with 11 recursive calls
Minimum 3 found with 5 recursive calls with faster approach
add a comment |Â
up vote
2
down vote
If by simplification you mean optimization, then only one thing came to my mind:
Whenever you find a leaf node, remember its depth in
minmalFound
variable.When again you hit the next leaf node you compare its depth with previously remembered node. And save the minimum of both into
minmalFound
.
And the main logic to add is whenever you are searching a certain path and find out that the depth becomes as great as the existing minmalFound
, then you stop searching that path.
Here is code to demonstrate this technique:
public class Solution
public static int minDepth(TreeNode root)
reccallscount = 0;
minmalFound = -1;
if (root == null)
return 0;
return recur(root, 1);
public static boolean doFaster = false;
private static int minmalFound = -1;
private static int reccallscount = 0;
private static int recur(TreeNode root, int depth)
public static void main(String args)
TreeNode root = new TreeNode();
root.left = new TreeNode();
root.left.right = new TreeNode();
root.right = new TreeNode();
root.right.left = new TreeNode();
root.right.left.right = new TreeNode();
root.right.left.right.left = new TreeNode();
Solution.doFaster = false;
int min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls");
Solution.doFaster = true;
min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls with faster approach");
class TreeNode
TreeNode left = null;
TreeNode right = null;
In the code above, I created a tree with left side depth = 3 and right side depth = 5. I ran minDepth()
twice; first using your algorithm, and again with my addition. The result is then printed:
Minimum 3 found with 11 recursive calls
Minimum 3 found with 5 recursive calls with faster approach
add a comment |Â
up vote
2
down vote
up vote
2
down vote
If by simplification you mean optimization, then only one thing came to my mind:
Whenever you find a leaf node, remember its depth in
minmalFound
variable.When again you hit the next leaf node you compare its depth with previously remembered node. And save the minimum of both into
minmalFound
.
And the main logic to add is whenever you are searching a certain path and find out that the depth becomes as great as the existing minmalFound
, then you stop searching that path.
Here is code to demonstrate this technique:
public class Solution
public static int minDepth(TreeNode root)
reccallscount = 0;
minmalFound = -1;
if (root == null)
return 0;
return recur(root, 1);
public static boolean doFaster = false;
private static int minmalFound = -1;
private static int reccallscount = 0;
private static int recur(TreeNode root, int depth)
public static void main(String args)
TreeNode root = new TreeNode();
root.left = new TreeNode();
root.left.right = new TreeNode();
root.right = new TreeNode();
root.right.left = new TreeNode();
root.right.left.right = new TreeNode();
root.right.left.right.left = new TreeNode();
Solution.doFaster = false;
int min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls");
Solution.doFaster = true;
min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls with faster approach");
class TreeNode
TreeNode left = null;
TreeNode right = null;
In the code above, I created a tree with left side depth = 3 and right side depth = 5. I ran minDepth()
twice; first using your algorithm, and again with my addition. The result is then printed:
Minimum 3 found with 11 recursive calls
Minimum 3 found with 5 recursive calls with faster approach
If by simplification you mean optimization, then only one thing came to my mind:
Whenever you find a leaf node, remember its depth in
minmalFound
variable.When again you hit the next leaf node you compare its depth with previously remembered node. And save the minimum of both into
minmalFound
.
And the main logic to add is whenever you are searching a certain path and find out that the depth becomes as great as the existing minmalFound
, then you stop searching that path.
Here is code to demonstrate this technique:
public class Solution
public static int minDepth(TreeNode root)
reccallscount = 0;
minmalFound = -1;
if (root == null)
return 0;
return recur(root, 1);
public static boolean doFaster = false;
private static int minmalFound = -1;
private static int reccallscount = 0;
private static int recur(TreeNode root, int depth)
public static void main(String args)
TreeNode root = new TreeNode();
root.left = new TreeNode();
root.left.right = new TreeNode();
root.right = new TreeNode();
root.right.left = new TreeNode();
root.right.left.right = new TreeNode();
root.right.left.right.left = new TreeNode();
Solution.doFaster = false;
int min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls");
Solution.doFaster = true;
min = minDepth(root);
System.out.println("Minimum " + min + " found with " + reccallscount + " recursive calls with faster approach");
class TreeNode
TreeNode left = null;
TreeNode right = null;
In the code above, I created a tree with left side depth = 3 and right side depth = 5. I ran minDepth()
twice; first using your algorithm, and again with my addition. The result is then printed:
Minimum 3 found with 11 recursive calls
Minimum 3 found with 5 recursive calls with faster approach
edited Jun 22 at 9:46
Toby Speight
17.2k13487
17.2k13487
answered Jun 22 at 8:52
maximus
1212
1212
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f197005%2ffind-minimum-depth-in-a-binary-tree%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password