Overloading Java function (insert into binary tree)
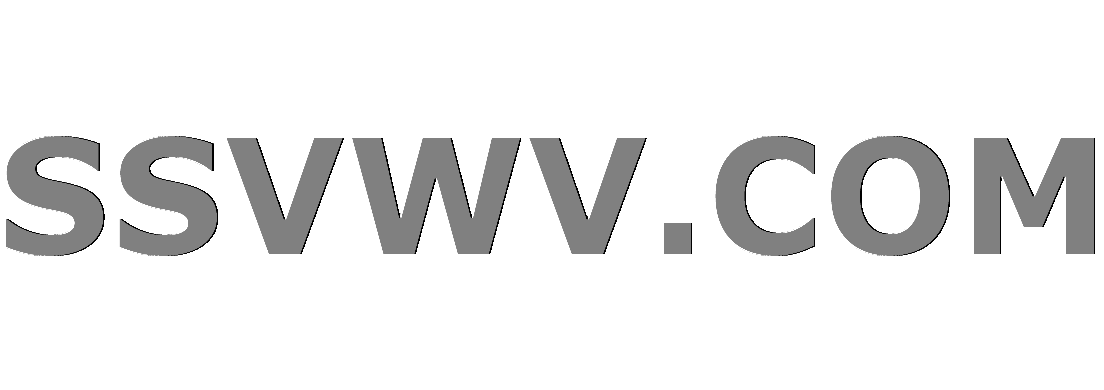
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I have a binary tree below and it seems to make sense to overload the insert()
method. If insert()
is called without a Node
specified, it will just work with head
, otherwise if it receives a Node
, it will do the insert in the subtree starting with that Node
. This second variant is then called recursively, see below.
The repetition that happens in the code below seems wrong to me (not DRY). Is there a better way to do this or is this a limitation in Java?
public class probBinaryTree
public Node head;
public probBinaryTree()
this.head = null;
private class Node
int data;
Node left;
Node right;
int size;
public Node(int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(int d)
if (head == null)
head = new Node(d);
return head;
head.size++;
if (head.left == null) return insert(head.left, d);
if (head.right == null) return insert(head.right, d);
if (head.left.size <= head.right.size) return insert(head.left, d);
if (head.left.size > head.right.size) return insert(head.right, d);
return null;
private Node insert(Node n, int d)
if (n == null)
n = new Node(d);
return n;
n.size++;
if (n.left == null) return insert(n.left, d);
if (n.right == null) return insert(n.right, d);
if (n.left.size <= n.right.size) return insert(n.left, d);
if (n.left.size > n.right.size) return insert(n.right, d);
return null;
java algorithm tree overloading
add a comment |Â
up vote
1
down vote
favorite
I have a binary tree below and it seems to make sense to overload the insert()
method. If insert()
is called without a Node
specified, it will just work with head
, otherwise if it receives a Node
, it will do the insert in the subtree starting with that Node
. This second variant is then called recursively, see below.
The repetition that happens in the code below seems wrong to me (not DRY). Is there a better way to do this or is this a limitation in Java?
public class probBinaryTree
public Node head;
public probBinaryTree()
this.head = null;
private class Node
int data;
Node left;
Node right;
int size;
public Node(int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(int d)
if (head == null)
head = new Node(d);
return head;
head.size++;
if (head.left == null) return insert(head.left, d);
if (head.right == null) return insert(head.right, d);
if (head.left.size <= head.right.size) return insert(head.left, d);
if (head.left.size > head.right.size) return insert(head.right, d);
return null;
private Node insert(Node n, int d)
if (n == null)
n = new Node(d);
return n;
n.size++;
if (n.left == null) return insert(n.left, d);
if (n.right == null) return insert(n.right, d);
if (n.left.size <= n.right.size) return insert(n.left, d);
if (n.left.size > n.right.size) return insert(n.right, d);
return null;
java algorithm tree overloading
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a binary tree below and it seems to make sense to overload the insert()
method. If insert()
is called without a Node
specified, it will just work with head
, otherwise if it receives a Node
, it will do the insert in the subtree starting with that Node
. This second variant is then called recursively, see below.
The repetition that happens in the code below seems wrong to me (not DRY). Is there a better way to do this or is this a limitation in Java?
public class probBinaryTree
public Node head;
public probBinaryTree()
this.head = null;
private class Node
int data;
Node left;
Node right;
int size;
public Node(int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(int d)
if (head == null)
head = new Node(d);
return head;
head.size++;
if (head.left == null) return insert(head.left, d);
if (head.right == null) return insert(head.right, d);
if (head.left.size <= head.right.size) return insert(head.left, d);
if (head.left.size > head.right.size) return insert(head.right, d);
return null;
private Node insert(Node n, int d)
if (n == null)
n = new Node(d);
return n;
n.size++;
if (n.left == null) return insert(n.left, d);
if (n.right == null) return insert(n.right, d);
if (n.left.size <= n.right.size) return insert(n.left, d);
if (n.left.size > n.right.size) return insert(n.right, d);
return null;
java algorithm tree overloading
I have a binary tree below and it seems to make sense to overload the insert()
method. If insert()
is called without a Node
specified, it will just work with head
, otherwise if it receives a Node
, it will do the insert in the subtree starting with that Node
. This second variant is then called recursively, see below.
The repetition that happens in the code below seems wrong to me (not DRY). Is there a better way to do this or is this a limitation in Java?
public class probBinaryTree
public Node head;
public probBinaryTree()
this.head = null;
private class Node
int data;
Node left;
Node right;
int size;
public Node(int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(int d)
if (head == null)
head = new Node(d);
return head;
head.size++;
if (head.left == null) return insert(head.left, d);
if (head.right == null) return insert(head.right, d);
if (head.left.size <= head.right.size) return insert(head.left, d);
if (head.left.size > head.right.size) return insert(head.right, d);
return null;
private Node insert(Node n, int d)
if (n == null)
n = new Node(d);
return n;
n.size++;
if (n.left == null) return insert(n.left, d);
if (n.right == null) return insert(n.right, d);
if (n.left.size <= n.right.size) return insert(n.left, d);
if (n.left.size > n.right.size) return insert(n.right, d);
return null;
java algorithm tree overloading
edited May 21 at 9:05
asked May 21 at 8:12
Andras
1184
1184
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
1
down vote
Just have one insert
method call the other. In your code, that would look like:
public final class BinaryTree
public Node head;
public BinaryTree()
this.head = null;
private class Node
private final int data;
private final Node left;
private final Node right;
private int size;
public Node(final int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(final int d)
if (this.head == null)
this.head = new Node(d);
return this.head;
return this.insert(this.head, d);
private Node insert(final Node n, final int d)
if (n == null)
return new Node(d);
n.size++;
if (n.left == null)
return this.insert(n.left, d);
if (n.right == null)
return this.insert(n.right, d);
if (n.left.size <= n.right.size)
return this.insert(n.left, d);
if (n.left.size > n.right.size)
return this.insert(n.right, d);
return null;
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Just have one insert
method call the other. In your code, that would look like:
public final class BinaryTree
public Node head;
public BinaryTree()
this.head = null;
private class Node
private final int data;
private final Node left;
private final Node right;
private int size;
public Node(final int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(final int d)
if (this.head == null)
this.head = new Node(d);
return this.head;
return this.insert(this.head, d);
private Node insert(final Node n, final int d)
if (n == null)
return new Node(d);
n.size++;
if (n.left == null)
return this.insert(n.left, d);
if (n.right == null)
return this.insert(n.right, d);
if (n.left.size <= n.right.size)
return this.insert(n.left, d);
if (n.left.size > n.right.size)
return this.insert(n.right, d);
return null;
add a comment |Â
up vote
1
down vote
Just have one insert
method call the other. In your code, that would look like:
public final class BinaryTree
public Node head;
public BinaryTree()
this.head = null;
private class Node
private final int data;
private final Node left;
private final Node right;
private int size;
public Node(final int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(final int d)
if (this.head == null)
this.head = new Node(d);
return this.head;
return this.insert(this.head, d);
private Node insert(final Node n, final int d)
if (n == null)
return new Node(d);
n.size++;
if (n.left == null)
return this.insert(n.left, d);
if (n.right == null)
return this.insert(n.right, d);
if (n.left.size <= n.right.size)
return this.insert(n.left, d);
if (n.left.size > n.right.size)
return this.insert(n.right, d);
return null;
add a comment |Â
up vote
1
down vote
up vote
1
down vote
Just have one insert
method call the other. In your code, that would look like:
public final class BinaryTree
public Node head;
public BinaryTree()
this.head = null;
private class Node
private final int data;
private final Node left;
private final Node right;
private int size;
public Node(final int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(final int d)
if (this.head == null)
this.head = new Node(d);
return this.head;
return this.insert(this.head, d);
private Node insert(final Node n, final int d)
if (n == null)
return new Node(d);
n.size++;
if (n.left == null)
return this.insert(n.left, d);
if (n.right == null)
return this.insert(n.right, d);
if (n.left.size <= n.right.size)
return this.insert(n.left, d);
if (n.left.size > n.right.size)
return this.insert(n.right, d);
return null;
Just have one insert
method call the other. In your code, that would look like:
public final class BinaryTree
public Node head;
public BinaryTree()
this.head = null;
private class Node
private final int data;
private final Node left;
private final Node right;
private int size;
public Node(final int d)
this.data = d;
this.left = null;
this.right = null;
this.size = 1;
public Node insert(final int d)
if (this.head == null)
this.head = new Node(d);
return this.head;
return this.insert(this.head, d);
private Node insert(final Node n, final int d)
if (n == null)
return new Node(d);
n.size++;
if (n.left == null)
return this.insert(n.left, d);
if (n.right == null)
return this.insert(n.right, d);
if (n.left.size <= n.right.size)
return this.insert(n.left, d);
if (n.left.size > n.right.size)
return this.insert(n.right, d);
return null;
answered May 21 at 11:15


Eric Stein
3,703512
3,703512
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f194850%2foverloading-java-function-insert-into-binary-tree%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password