Looping over a bidimensional array and extract data to a new one
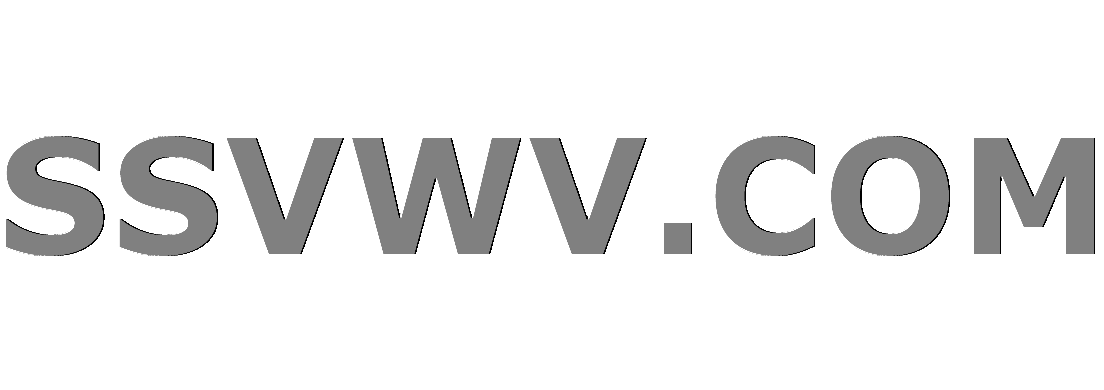
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
I have a bidimensional array like this:
const bArray =
[ [ 'Hello World',
'Hi everybody',
'How are you?'
],
[ text: 'Hola Mundo',
from: [Object],
raw: '' ,
text: 'Hola a todos',
from: [Object],
raw: '' ,
text: 'Cómo estás?',
from: [Object],
raw: '' ,
]
]
And I need to get as a result, only one array that should look like this:
[
en: 'Hello World',
es: 'Hola Mundo' ,
en: 'Hi everybody',
es: 'Hola a todos' ,
en: 'How are you?',
es: 'Cómo estás?' ,
]
This is how I do it:
let val1 = bArray[0].map(tuple => tuple);
let val2 = bArray[1].map(tuple => tuple);
let result = val1.reduce((arr, v, i) => arr.concat("en" : v, "es" : val2[i].text), );
And now in the result
variable, I have only one array with the result showed before.
My question?
Is there any improved way in which I can get the same result but with fewer lines of code? I mean, something like a combination of map
with reduce
, filter
or concat
in only one or two lines of code.
javascript mapreduce
add a comment |Â
up vote
2
down vote
favorite
I have a bidimensional array like this:
const bArray =
[ [ 'Hello World',
'Hi everybody',
'How are you?'
],
[ text: 'Hola Mundo',
from: [Object],
raw: '' ,
text: 'Hola a todos',
from: [Object],
raw: '' ,
text: 'Cómo estás?',
from: [Object],
raw: '' ,
]
]
And I need to get as a result, only one array that should look like this:
[
en: 'Hello World',
es: 'Hola Mundo' ,
en: 'Hi everybody',
es: 'Hola a todos' ,
en: 'How are you?',
es: 'Cómo estás?' ,
]
This is how I do it:
let val1 = bArray[0].map(tuple => tuple);
let val2 = bArray[1].map(tuple => tuple);
let result = val1.reduce((arr, v, i) => arr.concat("en" : v, "es" : val2[i].text), );
And now in the result
variable, I have only one array with the result showed before.
My question?
Is there any improved way in which I can get the same result but with fewer lines of code? I mean, something like a combination of map
with reduce
, filter
or concat
in only one or two lines of code.
javascript mapreduce
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have a bidimensional array like this:
const bArray =
[ [ 'Hello World',
'Hi everybody',
'How are you?'
],
[ text: 'Hola Mundo',
from: [Object],
raw: '' ,
text: 'Hola a todos',
from: [Object],
raw: '' ,
text: 'Cómo estás?',
from: [Object],
raw: '' ,
]
]
And I need to get as a result, only one array that should look like this:
[
en: 'Hello World',
es: 'Hola Mundo' ,
en: 'Hi everybody',
es: 'Hola a todos' ,
en: 'How are you?',
es: 'Cómo estás?' ,
]
This is how I do it:
let val1 = bArray[0].map(tuple => tuple);
let val2 = bArray[1].map(tuple => tuple);
let result = val1.reduce((arr, v, i) => arr.concat("en" : v, "es" : val2[i].text), );
And now in the result
variable, I have only one array with the result showed before.
My question?
Is there any improved way in which I can get the same result but with fewer lines of code? I mean, something like a combination of map
with reduce
, filter
or concat
in only one or two lines of code.
javascript mapreduce
I have a bidimensional array like this:
const bArray =
[ [ 'Hello World',
'Hi everybody',
'How are you?'
],
[ text: 'Hola Mundo',
from: [Object],
raw: '' ,
text: 'Hola a todos',
from: [Object],
raw: '' ,
text: 'Cómo estás?',
from: [Object],
raw: '' ,
]
]
And I need to get as a result, only one array that should look like this:
[
en: 'Hello World',
es: 'Hola Mundo' ,
en: 'Hi everybody',
es: 'Hola a todos' ,
en: 'How are you?',
es: 'Cómo estás?' ,
]
This is how I do it:
let val1 = bArray[0].map(tuple => tuple);
let val2 = bArray[1].map(tuple => tuple);
let result = val1.reduce((arr, v, i) => arr.concat("en" : v, "es" : val2[i].text), );
And now in the result
variable, I have only one array with the result showed before.
My question?
Is there any improved way in which I can get the same result but with fewer lines of code? I mean, something like a combination of map
with reduce
, filter
or concat
in only one or two lines of code.
javascript mapreduce
edited May 23 at 19:49
asked May 23 at 19:41
robe007
1135
1135
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You can compact a lot into a single line, but that is most times not the best thing to do in both source code quality and execution efficiency.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
However I would do it as follows.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
Which is easier to read and quicker when run.
Expanding it to idiomatic JS style.
const result = ;
let i = 0;
for (const en of bArray[0])
result.push(
en,
es : bArray[1][i++].text
);
// or
const result = ;
let i = 0;
for (const item of bArray[1])
result.push(
es : item.text,
en : bArray[0][i++]
);
1
In the last example, you need to fixen : bArray[i++]
toen : bArray[0][i++]
to get it work.
– robe007
May 24 at 17:47
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You can compact a lot into a single line, but that is most times not the best thing to do in both source code quality and execution efficiency.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
However I would do it as follows.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
Which is easier to read and quicker when run.
Expanding it to idiomatic JS style.
const result = ;
let i = 0;
for (const en of bArray[0])
result.push(
en,
es : bArray[1][i++].text
);
// or
const result = ;
let i = 0;
for (const item of bArray[1])
result.push(
es : item.text,
en : bArray[0][i++]
);
1
In the last example, you need to fixen : bArray[i++]
toen : bArray[0][i++]
to get it work.
– robe007
May 24 at 17:47
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
add a comment |Â
up vote
2
down vote
accepted
You can compact a lot into a single line, but that is most times not the best thing to do in both source code quality and execution efficiency.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
However I would do it as follows.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
Which is easier to read and quicker when run.
Expanding it to idiomatic JS style.
const result = ;
let i = 0;
for (const en of bArray[0])
result.push(
en,
es : bArray[1][i++].text
);
// or
const result = ;
let i = 0;
for (const item of bArray[1])
result.push(
es : item.text,
en : bArray[0][i++]
);
1
In the last example, you need to fixen : bArray[i++]
toen : bArray[0][i++]
to get it work.
– robe007
May 24 at 17:47
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
add a comment |Â
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You can compact a lot into a single line, but that is most times not the best thing to do in both source code quality and execution efficiency.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
However I would do it as follows.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
Which is easier to read and quicker when run.
Expanding it to idiomatic JS style.
const result = ;
let i = 0;
for (const en of bArray[0])
result.push(
en,
es : bArray[1][i++].text
);
// or
const result = ;
let i = 0;
for (const item of bArray[1])
result.push(
es : item.text,
en : bArray[0][i++]
);
You can compact a lot into a single line, but that is most times not the best thing to do in both source code quality and execution efficiency.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
However I would do it as follows.
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
Which is easier to read and quicker when run.
Expanding it to idiomatic JS style.
const result = ;
let i = 0;
for (const en of bArray[0])
result.push(
en,
es : bArray[1][i++].text
);
// or
const result = ;
let i = 0;
for (const item of bArray[1])
result.push(
es : item.text,
en : bArray[0][i++]
);
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,]];
const resultArray = bArray[0].reduce((arr, item, i) => (arr.push(en : item, es : bArray[1][i].text), arr), );
console.log(resultArray);
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
const bArray = [['Hello World', 'Hi everybody', 'How are you?'], [ text: 'Hola Mundo', from: [Object], raw: '' , text: 'Hola a todos', from: [Object], raw: '' , text: 'Cómo estás?', from: [Object], raw: '' ,] ];
const result = , aArr = bArray[0];
var i = 0;
for (const item of bArray[1]) result.push(es : item.text, en : aArr[i++])
console.log(result);
edited May 24 at 17:50
answered May 24 at 8:43


Blindman67
5,2961320
5,2961320
1
In the last example, you need to fixen : bArray[i++]
toen : bArray[0][i++]
to get it work.
– robe007
May 24 at 17:47
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
add a comment |Â
1
In the last example, you need to fixen : bArray[i++]
toen : bArray[0][i++]
to get it work.
– robe007
May 24 at 17:47
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
1
1
In the last example, you need to fix
en : bArray[i++]
to en : bArray[0][i++]
to get it work.– robe007
May 24 at 17:47
In the last example, you need to fix
en : bArray[i++]
to en : bArray[0][i++]
to get it work.– robe007
May 24 at 17:47
1
1
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
@robe007 Thanks for the heads up. :)
– Blindman67
May 24 at 17:51
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f195044%2flooping-over-a-bidimensional-array-and-extract-data-to-a-new-one%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password