Implementing Passport authentication
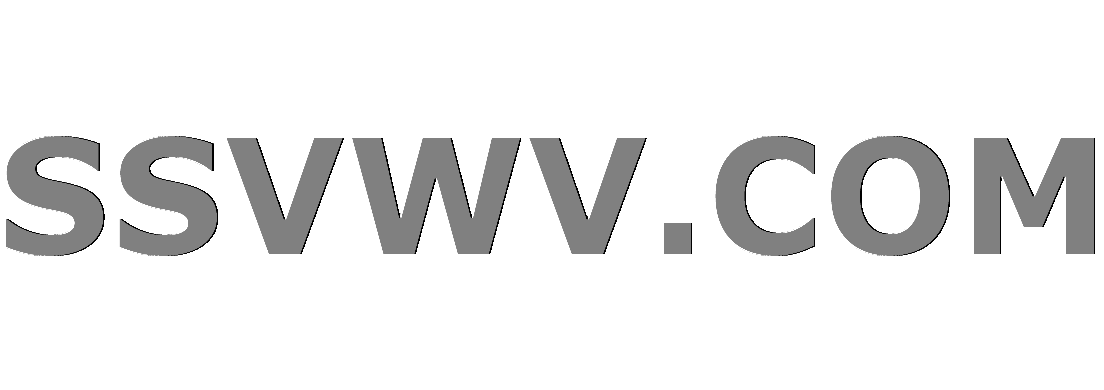
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
Here is code implementing Passport authentication with a Google Strategy. It uses Mongoose to store and retrieve user data. Note with Mongoose you can choose to use promises or not to use promises. I chose to use promises.
You might need to be familiar with both of these technologies to review this code if not check out the two links I provided.
I have included both files. I'm trying to find a way to refactor so that I don't handle errors for Mongoose in my Passport file. Currently I'm passing the promise to my Passport file, but I'm not sure if there is a better way to do this.
The code is full of comments below. Please let me know if more clarification or context is needed.
Passport File
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth').OAuth2Strategy;
const DBM = require('../../database/mongoose');
const helper = require('../../config/helper');
passport.use('google', new GoogleStrategy(helper.getPassport(), getOrCreateProfile));
// called during authorization
// google will give us an id on profile which is guaranteed to be unique
// we will use this to store each user's profile in mongoDB
// call getUser to see if the person has already been stored
function getOrCreateProfile (accessToken, refreshToken, profile, done)
let user_props = obtainProps(profile);
DBM.getUser(profile.id).then((res) =>
const user = res[0];
return user ? done(null, user) : createUser(done, user_props);
).catch( error =>
return done(error, null);
);
;
// the user was not found
// create the user then return user_props
function createUser (done, user_props)
DBM.createUser(user_props).then(() =>
return done(null, user_props);
).catch( error =>
return done(error, null);
);
// serializeUser required by passport to identify the user uniquely on the client
passport.serializeUser( (profile, done) =>
done(null, profile.id_google);
);
// deserializeUser required by passport to retrieve data on the server using unique
// identifier created by serializeUser
passport.deserializeUser( (id_google, done) =>
DBM.getUser(id_google).then((res) =>
done(null, res[0]);
).catch( (error) =>
console.error('DBM.getUser() Error: ', error);
);
);
// takes a profile from Google and extracts parameters to save
// Mongoose Schema should match MySQL database
function obtainProps (profile)
let props =
props.id_google = profile.id;
props.email = profile.emails[0].value;
props.name = profile.displayName;
props.pic_url = profile.photos[0].value;
props.type = profile._json.objectType;
return props;
module.exports = passport;
Mongoose File
const mongoose = require('mongoose');
const helper = require('../config/helper');
const Schema = mongoose.Schema;
/* Connect
*/
mongoose.connect(helper.getMongoose()).then(() =>
console.log('DEBUG: Mongoose Server Connected');
).catch((err) =>
console.log('DEBUG: Mongoose Server Did Not Connect');
);
/* Application Interface
*/
// create a single user
exports.createUser = (user) =>
return createSchema('User', schema.User, user);
;
// get a single user
exports.getUser = (id_google) =>
return getSchema('User', schema.User, id_google);
;
// get all domains
exports.getAllDomains = () =>
// return getSchema('Domains', schema.Domain);
/* Schema Interface
*/
// create a single schema
const createSchema = (name, schema, dataObj) =>
const Class = mongoose.model(name, schema);
const Instance = new Class(dataObj);
return Instance.save();
;
// get a single schema
const getSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find( id_google: id_google );
;
// get all from a schema
const getAllSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find();
;
/* Create schemas
*/
const schema = ;
// for relevant data from google profile
schema.User = new Schema(
id_google: type: String, required: true, unique: true ,
type: type: String, required: true ,
name: type: String, required: true ,
email: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
pic_url: type: String, required: true
);
// stub, finish making this
schema.Bookmark = new Schema(
url: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
);
javascript node.js mongoose oauth passport
add a comment |Â
up vote
2
down vote
favorite
Here is code implementing Passport authentication with a Google Strategy. It uses Mongoose to store and retrieve user data. Note with Mongoose you can choose to use promises or not to use promises. I chose to use promises.
You might need to be familiar with both of these technologies to review this code if not check out the two links I provided.
I have included both files. I'm trying to find a way to refactor so that I don't handle errors for Mongoose in my Passport file. Currently I'm passing the promise to my Passport file, but I'm not sure if there is a better way to do this.
The code is full of comments below. Please let me know if more clarification or context is needed.
Passport File
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth').OAuth2Strategy;
const DBM = require('../../database/mongoose');
const helper = require('../../config/helper');
passport.use('google', new GoogleStrategy(helper.getPassport(), getOrCreateProfile));
// called during authorization
// google will give us an id on profile which is guaranteed to be unique
// we will use this to store each user's profile in mongoDB
// call getUser to see if the person has already been stored
function getOrCreateProfile (accessToken, refreshToken, profile, done)
let user_props = obtainProps(profile);
DBM.getUser(profile.id).then((res) =>
const user = res[0];
return user ? done(null, user) : createUser(done, user_props);
).catch( error =>
return done(error, null);
);
;
// the user was not found
// create the user then return user_props
function createUser (done, user_props)
DBM.createUser(user_props).then(() =>
return done(null, user_props);
).catch( error =>
return done(error, null);
);
// serializeUser required by passport to identify the user uniquely on the client
passport.serializeUser( (profile, done) =>
done(null, profile.id_google);
);
// deserializeUser required by passport to retrieve data on the server using unique
// identifier created by serializeUser
passport.deserializeUser( (id_google, done) =>
DBM.getUser(id_google).then((res) =>
done(null, res[0]);
).catch( (error) =>
console.error('DBM.getUser() Error: ', error);
);
);
// takes a profile from Google and extracts parameters to save
// Mongoose Schema should match MySQL database
function obtainProps (profile)
let props =
props.id_google = profile.id;
props.email = profile.emails[0].value;
props.name = profile.displayName;
props.pic_url = profile.photos[0].value;
props.type = profile._json.objectType;
return props;
module.exports = passport;
Mongoose File
const mongoose = require('mongoose');
const helper = require('../config/helper');
const Schema = mongoose.Schema;
/* Connect
*/
mongoose.connect(helper.getMongoose()).then(() =>
console.log('DEBUG: Mongoose Server Connected');
).catch((err) =>
console.log('DEBUG: Mongoose Server Did Not Connect');
);
/* Application Interface
*/
// create a single user
exports.createUser = (user) =>
return createSchema('User', schema.User, user);
;
// get a single user
exports.getUser = (id_google) =>
return getSchema('User', schema.User, id_google);
;
// get all domains
exports.getAllDomains = () =>
// return getSchema('Domains', schema.Domain);
/* Schema Interface
*/
// create a single schema
const createSchema = (name, schema, dataObj) =>
const Class = mongoose.model(name, schema);
const Instance = new Class(dataObj);
return Instance.save();
;
// get a single schema
const getSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find( id_google: id_google );
;
// get all from a schema
const getAllSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find();
;
/* Create schemas
*/
const schema = ;
// for relevant data from google profile
schema.User = new Schema(
id_google: type: String, required: true, unique: true ,
type: type: String, required: true ,
name: type: String, required: true ,
email: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
pic_url: type: String, required: true
);
// stub, finish making this
schema.Bookmark = new Schema(
url: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
);
javascript node.js mongoose oauth passport
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Here is code implementing Passport authentication with a Google Strategy. It uses Mongoose to store and retrieve user data. Note with Mongoose you can choose to use promises or not to use promises. I chose to use promises.
You might need to be familiar with both of these technologies to review this code if not check out the two links I provided.
I have included both files. I'm trying to find a way to refactor so that I don't handle errors for Mongoose in my Passport file. Currently I'm passing the promise to my Passport file, but I'm not sure if there is a better way to do this.
The code is full of comments below. Please let me know if more clarification or context is needed.
Passport File
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth').OAuth2Strategy;
const DBM = require('../../database/mongoose');
const helper = require('../../config/helper');
passport.use('google', new GoogleStrategy(helper.getPassport(), getOrCreateProfile));
// called during authorization
// google will give us an id on profile which is guaranteed to be unique
// we will use this to store each user's profile in mongoDB
// call getUser to see if the person has already been stored
function getOrCreateProfile (accessToken, refreshToken, profile, done)
let user_props = obtainProps(profile);
DBM.getUser(profile.id).then((res) =>
const user = res[0];
return user ? done(null, user) : createUser(done, user_props);
).catch( error =>
return done(error, null);
);
;
// the user was not found
// create the user then return user_props
function createUser (done, user_props)
DBM.createUser(user_props).then(() =>
return done(null, user_props);
).catch( error =>
return done(error, null);
);
// serializeUser required by passport to identify the user uniquely on the client
passport.serializeUser( (profile, done) =>
done(null, profile.id_google);
);
// deserializeUser required by passport to retrieve data on the server using unique
// identifier created by serializeUser
passport.deserializeUser( (id_google, done) =>
DBM.getUser(id_google).then((res) =>
done(null, res[0]);
).catch( (error) =>
console.error('DBM.getUser() Error: ', error);
);
);
// takes a profile from Google and extracts parameters to save
// Mongoose Schema should match MySQL database
function obtainProps (profile)
let props =
props.id_google = profile.id;
props.email = profile.emails[0].value;
props.name = profile.displayName;
props.pic_url = profile.photos[0].value;
props.type = profile._json.objectType;
return props;
module.exports = passport;
Mongoose File
const mongoose = require('mongoose');
const helper = require('../config/helper');
const Schema = mongoose.Schema;
/* Connect
*/
mongoose.connect(helper.getMongoose()).then(() =>
console.log('DEBUG: Mongoose Server Connected');
).catch((err) =>
console.log('DEBUG: Mongoose Server Did Not Connect');
);
/* Application Interface
*/
// create a single user
exports.createUser = (user) =>
return createSchema('User', schema.User, user);
;
// get a single user
exports.getUser = (id_google) =>
return getSchema('User', schema.User, id_google);
;
// get all domains
exports.getAllDomains = () =>
// return getSchema('Domains', schema.Domain);
/* Schema Interface
*/
// create a single schema
const createSchema = (name, schema, dataObj) =>
const Class = mongoose.model(name, schema);
const Instance = new Class(dataObj);
return Instance.save();
;
// get a single schema
const getSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find( id_google: id_google );
;
// get all from a schema
const getAllSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find();
;
/* Create schemas
*/
const schema = ;
// for relevant data from google profile
schema.User = new Schema(
id_google: type: String, required: true, unique: true ,
type: type: String, required: true ,
name: type: String, required: true ,
email: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
pic_url: type: String, required: true
);
// stub, finish making this
schema.Bookmark = new Schema(
url: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
);
javascript node.js mongoose oauth passport
Here is code implementing Passport authentication with a Google Strategy. It uses Mongoose to store and retrieve user data. Note with Mongoose you can choose to use promises or not to use promises. I chose to use promises.
You might need to be familiar with both of these technologies to review this code if not check out the two links I provided.
I have included both files. I'm trying to find a way to refactor so that I don't handle errors for Mongoose in my Passport file. Currently I'm passing the promise to my Passport file, but I'm not sure if there is a better way to do this.
The code is full of comments below. Please let me know if more clarification or context is needed.
Passport File
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth').OAuth2Strategy;
const DBM = require('../../database/mongoose');
const helper = require('../../config/helper');
passport.use('google', new GoogleStrategy(helper.getPassport(), getOrCreateProfile));
// called during authorization
// google will give us an id on profile which is guaranteed to be unique
// we will use this to store each user's profile in mongoDB
// call getUser to see if the person has already been stored
function getOrCreateProfile (accessToken, refreshToken, profile, done)
let user_props = obtainProps(profile);
DBM.getUser(profile.id).then((res) =>
const user = res[0];
return user ? done(null, user) : createUser(done, user_props);
).catch( error =>
return done(error, null);
);
;
// the user was not found
// create the user then return user_props
function createUser (done, user_props)
DBM.createUser(user_props).then(() =>
return done(null, user_props);
).catch( error =>
return done(error, null);
);
// serializeUser required by passport to identify the user uniquely on the client
passport.serializeUser( (profile, done) =>
done(null, profile.id_google);
);
// deserializeUser required by passport to retrieve data on the server using unique
// identifier created by serializeUser
passport.deserializeUser( (id_google, done) =>
DBM.getUser(id_google).then((res) =>
done(null, res[0]);
).catch( (error) =>
console.error('DBM.getUser() Error: ', error);
);
);
// takes a profile from Google and extracts parameters to save
// Mongoose Schema should match MySQL database
function obtainProps (profile)
let props =
props.id_google = profile.id;
props.email = profile.emails[0].value;
props.name = profile.displayName;
props.pic_url = profile.photos[0].value;
props.type = profile._json.objectType;
return props;
module.exports = passport;
Mongoose File
const mongoose = require('mongoose');
const helper = require('../config/helper');
const Schema = mongoose.Schema;
/* Connect
*/
mongoose.connect(helper.getMongoose()).then(() =>
console.log('DEBUG: Mongoose Server Connected');
).catch((err) =>
console.log('DEBUG: Mongoose Server Did Not Connect');
);
/* Application Interface
*/
// create a single user
exports.createUser = (user) =>
return createSchema('User', schema.User, user);
;
// get a single user
exports.getUser = (id_google) =>
return getSchema('User', schema.User, id_google);
;
// get all domains
exports.getAllDomains = () =>
// return getSchema('Domains', schema.Domain);
/* Schema Interface
*/
// create a single schema
const createSchema = (name, schema, dataObj) =>
const Class = mongoose.model(name, schema);
const Instance = new Class(dataObj);
return Instance.save();
;
// get a single schema
const getSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find( id_google: id_google );
;
// get all from a schema
const getAllSchema = (name, schema, id_google) =>
const Class = mongoose.model(name, schema);
return Class.find();
;
/* Create schemas
*/
const schema = ;
// for relevant data from google profile
schema.User = new Schema(
id_google: type: String, required: true, unique: true ,
type: type: String, required: true ,
name: type: String, required: true ,
email: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
pic_url: type: String, required: true
);
// stub, finish making this
schema.Bookmark = new Schema(
url: type: String, required: true ,
timestamp: type: Date, default: Date.now ,
);
javascript node.js mongoose oauth passport
edited May 20 at 19:59


Jamal♦
30.1k11114225
30.1k11114225
asked May 20 at 19:41


john morsey
113
113
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16
add a comment |Â
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f194824%2fimplementing-passport-authentication%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Something has to handle those errors, looks to me like given passport is the file that uses it should be the guy. What's the concern? Polluting the passport file with Mongoose error handling? If so you could always wrap the errors with something more store-agnostic.
– James
Jun 28 at 0:16