Dice roller in Ruby (mainly for D&D)
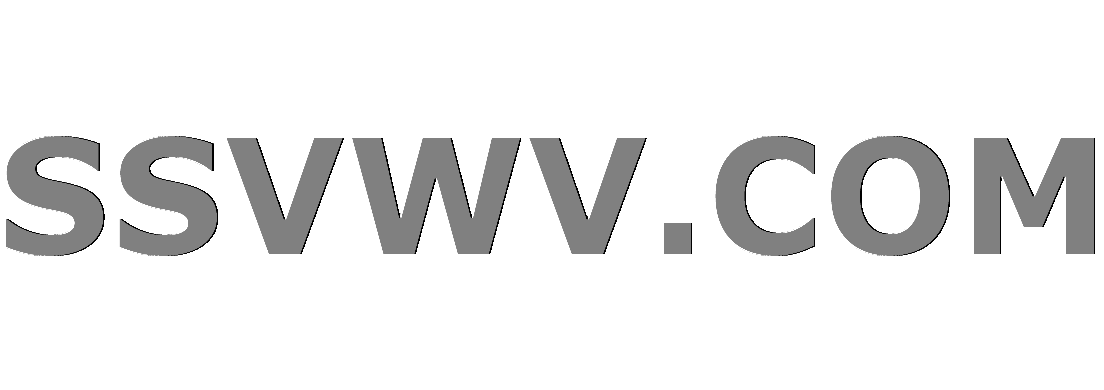
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I have been working with Ruby for about a week or two now, and I wanted to create a dice-rolling application that intakes any string (e.g. 1d4 + 1 or 3d20 + 4) and outputs the correct dice rolling.
def roll(amount = 0, sides = 0)
#this is the function that gets a random roll with the amount of sides and the amount of dice inputted.
srand
total = 0
(amount.to_i).times do
total += rand(1..sides.to_i)
end
return total
end
while true
raw_input = gets.chomp.to_s
if raw_input == "exit"
abort("May your rolls be ever natural.")
end
proc_input = raw_input.tr("^0-9", " ")
#this keeps all the numbers from the string input.
output = proc_input.split()
if (output[2]) == nil
mod = 0
else
mod = output[2]
end
fin = roll(output[0], output[1]) + mod.to_i
puts "#fin.to_s"
end
ruby random dice
add a comment |Â
up vote
1
down vote
favorite
I have been working with Ruby for about a week or two now, and I wanted to create a dice-rolling application that intakes any string (e.g. 1d4 + 1 or 3d20 + 4) and outputs the correct dice rolling.
def roll(amount = 0, sides = 0)
#this is the function that gets a random roll with the amount of sides and the amount of dice inputted.
srand
total = 0
(amount.to_i).times do
total += rand(1..sides.to_i)
end
return total
end
while true
raw_input = gets.chomp.to_s
if raw_input == "exit"
abort("May your rolls be ever natural.")
end
proc_input = raw_input.tr("^0-9", " ")
#this keeps all the numbers from the string input.
output = proc_input.split()
if (output[2]) == nil
mod = 0
else
mod = output[2]
end
fin = roll(output[0], output[1]) + mod.to_i
puts "#fin.to_s"
end
ruby random dice
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have been working with Ruby for about a week or two now, and I wanted to create a dice-rolling application that intakes any string (e.g. 1d4 + 1 or 3d20 + 4) and outputs the correct dice rolling.
def roll(amount = 0, sides = 0)
#this is the function that gets a random roll with the amount of sides and the amount of dice inputted.
srand
total = 0
(amount.to_i).times do
total += rand(1..sides.to_i)
end
return total
end
while true
raw_input = gets.chomp.to_s
if raw_input == "exit"
abort("May your rolls be ever natural.")
end
proc_input = raw_input.tr("^0-9", " ")
#this keeps all the numbers from the string input.
output = proc_input.split()
if (output[2]) == nil
mod = 0
else
mod = output[2]
end
fin = roll(output[0], output[1]) + mod.to_i
puts "#fin.to_s"
end
ruby random dice
I have been working with Ruby for about a week or two now, and I wanted to create a dice-rolling application that intakes any string (e.g. 1d4 + 1 or 3d20 + 4) and outputs the correct dice rolling.
def roll(amount = 0, sides = 0)
#this is the function that gets a random roll with the amount of sides and the amount of dice inputted.
srand
total = 0
(amount.to_i).times do
total += rand(1..sides.to_i)
end
return total
end
while true
raw_input = gets.chomp.to_s
if raw_input == "exit"
abort("May your rolls be ever natural.")
end
proc_input = raw_input.tr("^0-9", " ")
#this keeps all the numbers from the string input.
output = proc_input.split()
if (output[2]) == nil
mod = 0
else
mod = output[2]
end
fin = roll(output[0], output[1]) + mod.to_i
puts "#fin.to_s"
end
ruby random dice
edited Jan 15 at 4:58


Jamal♦
30.1k11114225
30.1k11114225
asked Jan 15 at 4:43


cricketts
484
484
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Nice code overall, pretty readable, though there is space for improvements.
1) You don't have to return from ruby methods.
2) Good use of Enumerable
methods will make code cleaner.
3) Use loop
instead of while true
4) Also consider keyword arguments, as calling roll(2, 4)
looks kind of cryptic.
Will all that in mind, we can turn your code into something like:
def roll(amount = 0, sides = 0)
# rand(1..0) will return nil btw
amount.to_i.times.sum rand(1..sides.to_i)
end
loop do
input = gets.chomp.to_s
abort("May your rolls be ever natural.") if input == "exit"
amount, sides, mod = input.tr("^0-9", " ").split
fin = roll(amount, sides) + mod.to_i # nil converts to 0
puts fin
end
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Nice code overall, pretty readable, though there is space for improvements.
1) You don't have to return from ruby methods.
2) Good use of Enumerable
methods will make code cleaner.
3) Use loop
instead of while true
4) Also consider keyword arguments, as calling roll(2, 4)
looks kind of cryptic.
Will all that in mind, we can turn your code into something like:
def roll(amount = 0, sides = 0)
# rand(1..0) will return nil btw
amount.to_i.times.sum rand(1..sides.to_i)
end
loop do
input = gets.chomp.to_s
abort("May your rolls be ever natural.") if input == "exit"
amount, sides, mod = input.tr("^0-9", " ").split
fin = roll(amount, sides) + mod.to_i # nil converts to 0
puts fin
end
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
add a comment |Â
up vote
1
down vote
accepted
Nice code overall, pretty readable, though there is space for improvements.
1) You don't have to return from ruby methods.
2) Good use of Enumerable
methods will make code cleaner.
3) Use loop
instead of while true
4) Also consider keyword arguments, as calling roll(2, 4)
looks kind of cryptic.
Will all that in mind, we can turn your code into something like:
def roll(amount = 0, sides = 0)
# rand(1..0) will return nil btw
amount.to_i.times.sum rand(1..sides.to_i)
end
loop do
input = gets.chomp.to_s
abort("May your rolls be ever natural.") if input == "exit"
amount, sides, mod = input.tr("^0-9", " ").split
fin = roll(amount, sides) + mod.to_i # nil converts to 0
puts fin
end
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
add a comment |Â
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Nice code overall, pretty readable, though there is space for improvements.
1) You don't have to return from ruby methods.
2) Good use of Enumerable
methods will make code cleaner.
3) Use loop
instead of while true
4) Also consider keyword arguments, as calling roll(2, 4)
looks kind of cryptic.
Will all that in mind, we can turn your code into something like:
def roll(amount = 0, sides = 0)
# rand(1..0) will return nil btw
amount.to_i.times.sum rand(1..sides.to_i)
end
loop do
input = gets.chomp.to_s
abort("May your rolls be ever natural.") if input == "exit"
amount, sides, mod = input.tr("^0-9", " ").split
fin = roll(amount, sides) + mod.to_i # nil converts to 0
puts fin
end
Nice code overall, pretty readable, though there is space for improvements.
1) You don't have to return from ruby methods.
2) Good use of Enumerable
methods will make code cleaner.
3) Use loop
instead of while true
4) Also consider keyword arguments, as calling roll(2, 4)
looks kind of cryptic.
Will all that in mind, we can turn your code into something like:
def roll(amount = 0, sides = 0)
# rand(1..0) will return nil btw
amount.to_i.times.sum rand(1..sides.to_i)
end
loop do
input = gets.chomp.to_s
abort("May your rolls be ever natural.") if input == "exit"
amount, sides, mod = input.tr("^0-9", " ").split
fin = roll(amount, sides) + mod.to_i # nil converts to 0
puts fin
end
answered Jan 16 at 22:03
user1610127
1016
1016
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
add a comment |Â
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
1
1
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
Thank you! I looked back at the code, and some of my past projects and realized that I had written it like C or Java, instead of using a more powerful language's syntax and rules. Thanks again.
– cricketts
Jan 18 at 5:27
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f185121%2fdice-roller-in-ruby-mainly-for-dd%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password