Node.js/Express middleware to relay requests to various APIs
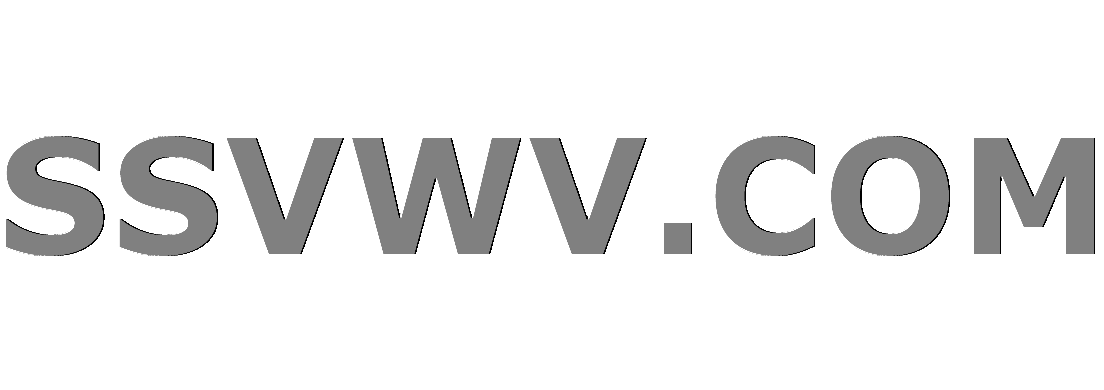
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
I am creating a nodejs middleware server that will handle api transactions from a frontend and relay them to various api's (internal and external). The primary goal is to hide api keys from the frontend. It can also consolidate requests that may require 2 or more endpoints, and modify data structures, thus simplifying our frontend code.
I am hoping for feedback on overall design/structure which will allow us to create a project that will be flexible as we add more apis while keeping it DRY
URLs, keys, etc. are stored in a .env file
index.js
require('dotenv').load();
const express = require('express'),
app = express(),
request = require("./request").request,
bodyParser = require('body-parser');
app.use(bodyParser.json());
//returns all locations
app.get("/locations", (req, res) =>
let URL = '...';
request(
method: 'get',
url: URL,
api: 'apiName'
).then((resp) =>
res.json(resp);
)
);
//send password reset link
app.post("/resetemail", (req, res) =>
let URL = '...';
let DATA = req.body;
request(
method: 'post',
url: URL,
api: 'apiName',
data: DATA
).then((resp) =>
res.json(resp);
)
);
app.listen(3000);
request.js
module.exports.request = function (options)
const client = require('./' + options.api).instance;
const handleError = require('./' + options.api).handleError;
const onSuccess = function (response)
console.log('Request Successful!', response);
return response.data;
return client(options)
.then(onSuccess)
.catch(handleError);
apiName.js
const axios = require('axios');
const axiosInstance = axios.create(
headers: "token": process.env.API_TOKEN ,
baseURL: process.env.API_BASE_URL,
timeout: 2000
);
const handleError = function (error) error.message);
module.exports =
instance: axiosInstance,
handleError: handleError
javascript node.js api express.js proxy
add a comment |Â
up vote
2
down vote
favorite
I am creating a nodejs middleware server that will handle api transactions from a frontend and relay them to various api's (internal and external). The primary goal is to hide api keys from the frontend. It can also consolidate requests that may require 2 or more endpoints, and modify data structures, thus simplifying our frontend code.
I am hoping for feedback on overall design/structure which will allow us to create a project that will be flexible as we add more apis while keeping it DRY
URLs, keys, etc. are stored in a .env file
index.js
require('dotenv').load();
const express = require('express'),
app = express(),
request = require("./request").request,
bodyParser = require('body-parser');
app.use(bodyParser.json());
//returns all locations
app.get("/locations", (req, res) =>
let URL = '...';
request(
method: 'get',
url: URL,
api: 'apiName'
).then((resp) =>
res.json(resp);
)
);
//send password reset link
app.post("/resetemail", (req, res) =>
let URL = '...';
let DATA = req.body;
request(
method: 'post',
url: URL,
api: 'apiName',
data: DATA
).then((resp) =>
res.json(resp);
)
);
app.listen(3000);
request.js
module.exports.request = function (options)
const client = require('./' + options.api).instance;
const handleError = require('./' + options.api).handleError;
const onSuccess = function (response)
console.log('Request Successful!', response);
return response.data;
return client(options)
.then(onSuccess)
.catch(handleError);
apiName.js
const axios = require('axios');
const axiosInstance = axios.create(
headers: "token": process.env.API_TOKEN ,
baseURL: process.env.API_BASE_URL,
timeout: 2000
);
const handleError = function (error) error.message);
module.exports =
instance: axiosInstance,
handleError: handleError
javascript node.js api express.js proxy
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
2
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am creating a nodejs middleware server that will handle api transactions from a frontend and relay them to various api's (internal and external). The primary goal is to hide api keys from the frontend. It can also consolidate requests that may require 2 or more endpoints, and modify data structures, thus simplifying our frontend code.
I am hoping for feedback on overall design/structure which will allow us to create a project that will be flexible as we add more apis while keeping it DRY
URLs, keys, etc. are stored in a .env file
index.js
require('dotenv').load();
const express = require('express'),
app = express(),
request = require("./request").request,
bodyParser = require('body-parser');
app.use(bodyParser.json());
//returns all locations
app.get("/locations", (req, res) =>
let URL = '...';
request(
method: 'get',
url: URL,
api: 'apiName'
).then((resp) =>
res.json(resp);
)
);
//send password reset link
app.post("/resetemail", (req, res) =>
let URL = '...';
let DATA = req.body;
request(
method: 'post',
url: URL,
api: 'apiName',
data: DATA
).then((resp) =>
res.json(resp);
)
);
app.listen(3000);
request.js
module.exports.request = function (options)
const client = require('./' + options.api).instance;
const handleError = require('./' + options.api).handleError;
const onSuccess = function (response)
console.log('Request Successful!', response);
return response.data;
return client(options)
.then(onSuccess)
.catch(handleError);
apiName.js
const axios = require('axios');
const axiosInstance = axios.create(
headers: "token": process.env.API_TOKEN ,
baseURL: process.env.API_BASE_URL,
timeout: 2000
);
const handleError = function (error) error.message);
module.exports =
instance: axiosInstance,
handleError: handleError
javascript node.js api express.js proxy
I am creating a nodejs middleware server that will handle api transactions from a frontend and relay them to various api's (internal and external). The primary goal is to hide api keys from the frontend. It can also consolidate requests that may require 2 or more endpoints, and modify data structures, thus simplifying our frontend code.
I am hoping for feedback on overall design/structure which will allow us to create a project that will be flexible as we add more apis while keeping it DRY
URLs, keys, etc. are stored in a .env file
index.js
require('dotenv').load();
const express = require('express'),
app = express(),
request = require("./request").request,
bodyParser = require('body-parser');
app.use(bodyParser.json());
//returns all locations
app.get("/locations", (req, res) =>
let URL = '...';
request(
method: 'get',
url: URL,
api: 'apiName'
).then((resp) =>
res.json(resp);
)
);
//send password reset link
app.post("/resetemail", (req, res) =>
let URL = '...';
let DATA = req.body;
request(
method: 'post',
url: URL,
api: 'apiName',
data: DATA
).then((resp) =>
res.json(resp);
)
);
app.listen(3000);
request.js
module.exports.request = function (options)
const client = require('./' + options.api).instance;
const handleError = require('./' + options.api).handleError;
const onSuccess = function (response)
console.log('Request Successful!', response);
return response.data;
return client(options)
.then(onSuccess)
.catch(handleError);
apiName.js
const axios = require('axios');
const axiosInstance = axios.create(
headers: "token": process.env.API_TOKEN ,
baseURL: process.env.API_BASE_URL,
timeout: 2000
);
const handleError = function (error) error.message);
module.exports =
instance: axiosInstance,
handleError: handleError
javascript node.js api express.js proxy
edited Jan 12 at 22:08


200_success
123k14143401
123k14143401
asked Jan 12 at 21:47


jriggs
285
285
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
2
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24
add a comment |Â
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
2
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
2
2
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
0
down vote
I think you need to add some middle-wares that helps to run your API code after that middle-ware with proper structuring way.
I would suggest the NPM Module express-app-generator
The advantages of it include:
- Code Management in clean way.
- Structured Routing.
- Add Multiple Middle-Wares in filters array.
- Create CRUD API's with
REST
orCRUD
Keyword.
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I think you need to add some middle-wares that helps to run your API code after that middle-ware with proper structuring way.
I would suggest the NPM Module express-app-generator
The advantages of it include:
- Code Management in clean way.
- Structured Routing.
- Add Multiple Middle-Wares in filters array.
- Create CRUD API's with
REST
orCRUD
Keyword.
add a comment |Â
up vote
0
down vote
I think you need to add some middle-wares that helps to run your API code after that middle-ware with proper structuring way.
I would suggest the NPM Module express-app-generator
The advantages of it include:
- Code Management in clean way.
- Structured Routing.
- Add Multiple Middle-Wares in filters array.
- Create CRUD API's with
REST
orCRUD
Keyword.
add a comment |Â
up vote
0
down vote
up vote
0
down vote
I think you need to add some middle-wares that helps to run your API code after that middle-ware with proper structuring way.
I would suggest the NPM Module express-app-generator
The advantages of it include:
- Code Management in clean way.
- Structured Routing.
- Add Multiple Middle-Wares in filters array.
- Create CRUD API's with
REST
orCRUD
Keyword.
I think you need to add some middle-wares that helps to run your API code after that middle-ware with proper structuring way.
I would suggest the NPM Module express-app-generator
The advantages of it include:
- Code Management in clean way.
- Structured Routing.
- Add Multiple Middle-Wares in filters array.
- Create CRUD API's with
REST
orCRUD
Keyword.
edited Jan 17 at 21:09


Sam Onela
5,88361545
5,88361545
answered Jan 17 at 16:56
cop
1
1
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f184991%2fnode-js-express-middleware-to-relay-requests-to-various-apis%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Just so I understand you correctly, "The primary goal is to hide api keys from the frontend." - it's providing the authentication layer for untrusted external clients?
– ferada
Jan 16 at 21:31
2
Yes. We want to prevent users from making requests directly to a vendor's api using our api keys. This node/express app will be on the same server as the frontend so the frontend can make api requests to 'localhost' which this code will then 'proxy' to the vendor's API. Does that make sense?
– jriggs
Jan 16 at 22:04
"It can also consolidate requests that may require 2 or more endpoints" - am I missing this in the code, or is there an example of this at all in the sample code?
– Sam Onela
Jan 22 at 20:24