Getting and setting data using Firestore
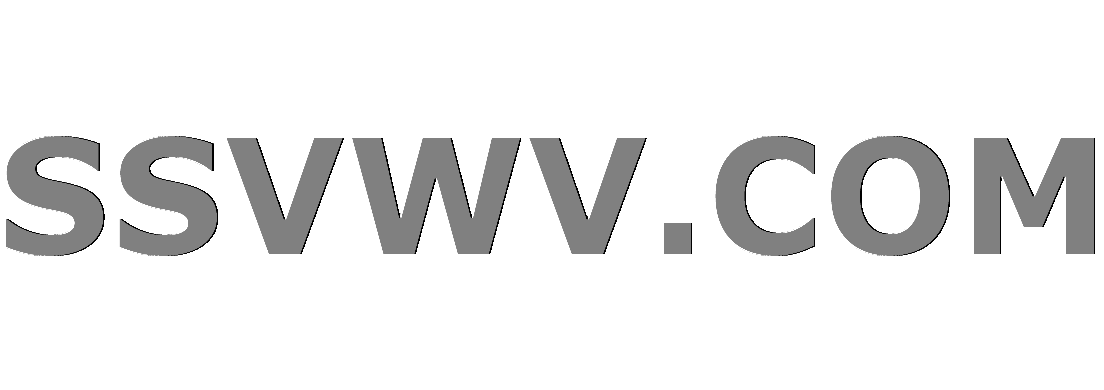
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
0
down vote
favorite
I am saving a string input in firebase and updating the front end in real time with firestore. How can I do the same thing in fewer lines of code? Is there a better way to do this?
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
javascript firebase nosql
add a comment |Â
up vote
0
down vote
favorite
I am saving a string input in firebase and updating the front end in real time with firestore. How can I do the same thing in fewer lines of code? Is there a better way to do this?
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
javascript firebase nosql
2
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25
add a comment |Â
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am saving a string input in firebase and updating the front end in real time with firestore. How can I do the same thing in fewer lines of code? Is there a better way to do this?
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
javascript firebase nosql
I am saving a string input in firebase and updating the front end in real time with firestore. How can I do the same thing in fewer lines of code? Is there a better way to do this?
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
// Initialize Firebase
var config =
apiKey: "xxxxxxxxxxx-83Oiv5HVhioLsyhQ",
authDomain: "xxxxxxxxx.firebaseapp.com",
databaseURL: "xxxxxxxxxx.firebaseio.com",
projectId: "xxxxxxxxxxxxxx",
storageBucket: "xxxxxxxxxxxxx.appspot.com",
messagingSenderId: "xxxxxxxxxxxxx"
;
firebase.initializeApp(config);
// initialize firestore
var fireStore = firebase.firestore();
// set the file to write changes
const docRef = fireStore.doc("todo/tasks");
// get target elements
const hotDogOutput = document.getElementById("hotDogOutput");
const textfield = document.getElementById("latestHotDogStatus");
const saveButton = document.getElementById("saveButton");
// empty arr for fetchting all tasks from db
var tasksList = ; // : on window load get data from db via getRealTimeUpdate()
// save task event
saveButton.addEventListener('click', function()
var textToSave = textfield.value; // get input val
tasksList.push(textToSave); // push to exiting data (from db)
hotDogOutput.innerHTML = ""; // empty the list html for +=
console.log(tasksList);
console.log("I am going to save" + textToSave + "to fire store");
docRef.set( // write to db : object > arr
tasksArr: tasksList
,
merge: true
) // dont overwrite, merge
.then(function() // returned promise
console.log("status saved");
textfield.value = ""; // empty the inputs currnet text after saving successfully
)
.catch(function(error) // errors
console.log("got an error" + error);
);
);
// get update from firestore and update ui
var getRealTimeUpdates = function()
docRef.onSnapshot(function(doc) // on document change
if (doc && doc.exists)
const myData = doc.data();
tasksList = myData.tasksArr; // populating that empty array with existing db data
// updating the ui with recently updated data
myData.tasksArr.forEach((element) =>
hotDogOutput.innerHTML += "<p>" + element + "</p>";
);
)
// fire getRealTimeUpdate on document load,
// which will get existing data from db and
// populate the front end , then
// continuously look for changes in db & update when necessary
getRealTimeUpdates();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Todo App</title>
</head>
<body>
<div id="hotDogOutput">Hot dog status : </div>
<input type="textfield" id="latestHotDogStatus">
<button id="saveButton">Button</button>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.8.2/firebase-firestore.js"></script>
<script src="js/app.js"></script>
</body>
</html>
javascript firebase nosql
edited Jan 18 at 6:08
asked Jan 17 at 19:16


Walid Omonos
1033
1033
2
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25
add a comment |Â
2
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25
2
2
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f185339%2fgetting-and-setting-data-using-firestore%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
2
In the future, I would advise against including your API Keys... refer to this meta post and the answers for more context...
– Sam Onela
Jan 17 at 19:25