Reform PHP associative array
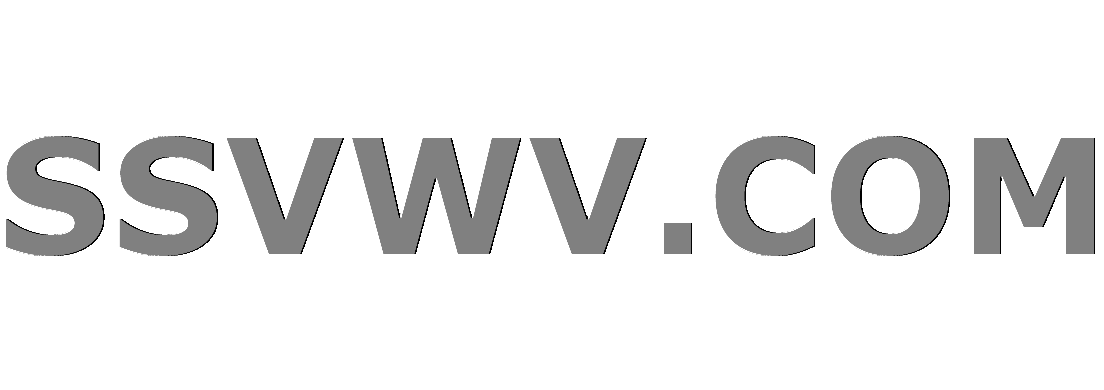
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I have an associative array:
$input = [
['key'=>'x', 'value'=>'a'],
['key'=>'x', 'value'=>'b'],
['key'=>'x', 'value'=>'c'],
['key'=>'y', 'value'=>'d'],
['key'=>'y', 'value'=>'e'],
['key'=>'z', 'value'=>'f'],
['key'=>'m', 'value'=>'n'],
];
And I want to reform it simple in:
$output = [
'x'=>['a','b','c'],
'y'=>['d','e'],
'x'=>'f',
'm'=>'n'
]
So basically, conditions are:
- If same key found then put values in an array.
- If no same key found then value remains string.
You can replace associative array with object if you are more comfortable with objects.
Here is my working solution for this problem:
foreach($input as $in)
if(!empty($output[$in['key']]))
if(is_array($output[$in['key']]))
$output[$in['key']] = $in['value'];
continue;
$output[$in['key']] = [$output[$in['key']],$in['value']];
continue;
$output[$in['key']] = $in['value'];
print_r($output);
However I believe that it can be done in much compact and efficient way.
Please comment your answers if someone has better solution.
Your help is much appreciated!
php array
add a comment |Â
up vote
1
down vote
favorite
I have an associative array:
$input = [
['key'=>'x', 'value'=>'a'],
['key'=>'x', 'value'=>'b'],
['key'=>'x', 'value'=>'c'],
['key'=>'y', 'value'=>'d'],
['key'=>'y', 'value'=>'e'],
['key'=>'z', 'value'=>'f'],
['key'=>'m', 'value'=>'n'],
];
And I want to reform it simple in:
$output = [
'x'=>['a','b','c'],
'y'=>['d','e'],
'x'=>'f',
'm'=>'n'
]
So basically, conditions are:
- If same key found then put values in an array.
- If no same key found then value remains string.
You can replace associative array with object if you are more comfortable with objects.
Here is my working solution for this problem:
foreach($input as $in)
if(!empty($output[$in['key']]))
if(is_array($output[$in['key']]))
$output[$in['key']] = $in['value'];
continue;
$output[$in['key']] = [$output[$in['key']],$in['value']];
continue;
$output[$in['key']] = $in['value'];
print_r($output);
However I believe that it can be done in much compact and efficient way.
Please comment your answers if someone has better solution.
Your help is much appreciated!
php array
1
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have an associative array:
$input = [
['key'=>'x', 'value'=>'a'],
['key'=>'x', 'value'=>'b'],
['key'=>'x', 'value'=>'c'],
['key'=>'y', 'value'=>'d'],
['key'=>'y', 'value'=>'e'],
['key'=>'z', 'value'=>'f'],
['key'=>'m', 'value'=>'n'],
];
And I want to reform it simple in:
$output = [
'x'=>['a','b','c'],
'y'=>['d','e'],
'x'=>'f',
'm'=>'n'
]
So basically, conditions are:
- If same key found then put values in an array.
- If no same key found then value remains string.
You can replace associative array with object if you are more comfortable with objects.
Here is my working solution for this problem:
foreach($input as $in)
if(!empty($output[$in['key']]))
if(is_array($output[$in['key']]))
$output[$in['key']] = $in['value'];
continue;
$output[$in['key']] = [$output[$in['key']],$in['value']];
continue;
$output[$in['key']] = $in['value'];
print_r($output);
However I believe that it can be done in much compact and efficient way.
Please comment your answers if someone has better solution.
Your help is much appreciated!
php array
I have an associative array:
$input = [
['key'=>'x', 'value'=>'a'],
['key'=>'x', 'value'=>'b'],
['key'=>'x', 'value'=>'c'],
['key'=>'y', 'value'=>'d'],
['key'=>'y', 'value'=>'e'],
['key'=>'z', 'value'=>'f'],
['key'=>'m', 'value'=>'n'],
];
And I want to reform it simple in:
$output = [
'x'=>['a','b','c'],
'y'=>['d','e'],
'x'=>'f',
'm'=>'n'
]
So basically, conditions are:
- If same key found then put values in an array.
- If no same key found then value remains string.
You can replace associative array with object if you are more comfortable with objects.
Here is my working solution for this problem:
foreach($input as $in)
if(!empty($output[$in['key']]))
if(is_array($output[$in['key']]))
$output[$in['key']] = $in['value'];
continue;
$output[$in['key']] = [$output[$in['key']],$in['value']];
continue;
$output[$in['key']] = $in['value'];
print_r($output);
However I believe that it can be done in much compact and efficient way.
Please comment your answers if someone has better solution.
Your help is much appreciated!
php array
edited Jan 17 at 23:40


Stephen Rauch
3,53551430
3,53551430
asked Jan 15 at 14:42
Learner
1091
1091
1
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43
add a comment |Â
1
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43
1
1
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f185143%2freform-php-associative-array%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
1
Your question has already been answered on Stack Overflow. Do you still want an answer here?
– Mast
Jan 15 at 15:33
Silly me, I had missed that you had a "working" solution and basically posted the same thing as an example. Duh! Where's my coffee? ;)
– Max Haaksman
Jan 16 at 11:40
:) funny..and yes it has been answered on other site, so I am good. I don't need an answer anymore. I don't know how to close the question here.
– Learner
Jan 18 at 8:43