Using BufferedReader to read lines of a file into a list
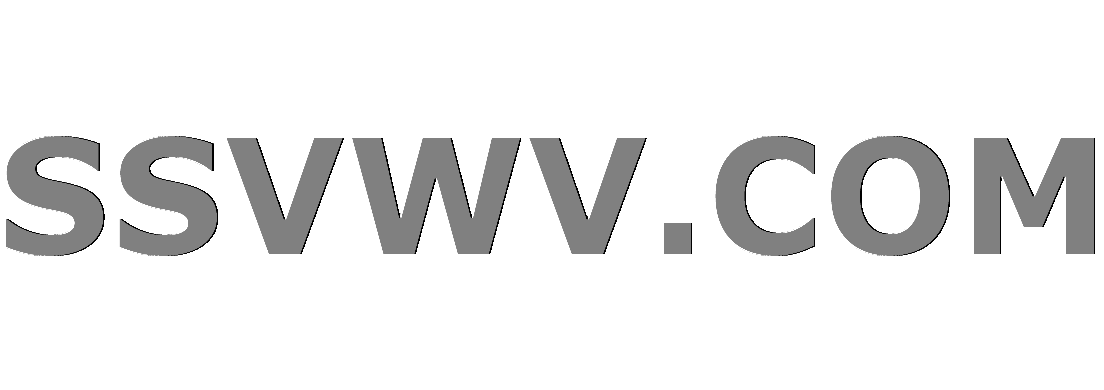
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
4
down vote
favorite
To help improve my proficiency with Java, I would like to know how should I close these FileReaders as I have come across multiple answers, and what could I do better with this block of code?
/**
* Returns a Vector<String> object that that can be parsed into classes.
* The file arugment must specify a realative or aboustle adress to the file.
* @param file to read
* @return
*/
public static ArrayList<String> read(File file)
ArrayList<String> data = new ArrayList<String>();
String lineRead;
try
FileReader fr = new FileReader(file);
BufferedReader br = new BufferedReader(fr);
while ( (lineRead = br.readLine()) != null )
data.add(lineRead);
br.close();
fr.close();
catch(IOException e)
e.printStackTrace();
return data;
java file
add a comment |Â
up vote
4
down vote
favorite
To help improve my proficiency with Java, I would like to know how should I close these FileReaders as I have come across multiple answers, and what could I do better with this block of code?
/**
* Returns a Vector<String> object that that can be parsed into classes.
* The file arugment must specify a realative or aboustle adress to the file.
* @param file to read
* @return
*/
public static ArrayList<String> read(File file)
ArrayList<String> data = new ArrayList<String>();
String lineRead;
try
FileReader fr = new FileReader(file);
BufferedReader br = new BufferedReader(fr);
while ( (lineRead = br.readLine()) != null )
data.add(lineRead);
br.close();
fr.close();
catch(IOException e)
e.printStackTrace();
return data;
java file
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00
add a comment |Â
up vote
4
down vote
favorite
up vote
4
down vote
favorite
To help improve my proficiency with Java, I would like to know how should I close these FileReaders as I have come across multiple answers, and what could I do better with this block of code?
/**
* Returns a Vector<String> object that that can be parsed into classes.
* The file arugment must specify a realative or aboustle adress to the file.
* @param file to read
* @return
*/
public static ArrayList<String> read(File file)
ArrayList<String> data = new ArrayList<String>();
String lineRead;
try
FileReader fr = new FileReader(file);
BufferedReader br = new BufferedReader(fr);
while ( (lineRead = br.readLine()) != null )
data.add(lineRead);
br.close();
fr.close();
catch(IOException e)
e.printStackTrace();
return data;
java file
To help improve my proficiency with Java, I would like to know how should I close these FileReaders as I have come across multiple answers, and what could I do better with this block of code?
/**
* Returns a Vector<String> object that that can be parsed into classes.
* The file arugment must specify a realative or aboustle adress to the file.
* @param file to read
* @return
*/
public static ArrayList<String> read(File file)
ArrayList<String> data = new ArrayList<String>();
String lineRead;
try
FileReader fr = new FileReader(file);
BufferedReader br = new BufferedReader(fr);
while ( (lineRead = br.readLine()) != null )
data.add(lineRead);
br.close();
fr.close();
catch(IOException e)
e.printStackTrace();
return data;
java file
edited May 1 at 21:09


200_success
123k14142399
123k14142399
asked May 1 at 20:53
Kyle
194
194
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00
add a comment |Â
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00
add a comment |Â
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
Since Java 7, you can make use of try-with-resources, which would change the implementation to:
try (BufferedReader br = new BufferedReader(new FileReader(file)))
while ((lineRead = br.readLine()) != null)
data.add(lineRead);
catch (IOException e)
...
Some other tips:
- Your documentation is not telling the truth and incomplete, you have to add some return documentation. Also, for parameters, the first string represents the name, and what follows is the explanation. So it should be more like e.g.
@param file the file to read all lines from
. - Return the interface instead of the implementation, i.e. return
List
instead ofArrayList
, which means you can always change the implementation. Never just print the stack trace, wrap and throw an (unchecked) exception or log. For more information as to why, see why printStackTrace is considered bad practice. In case you want to propagate the exception nonetheless, you can wrap it in another exception, e.g.:
try
...
catch (IOException e)
throw new UncheckedIOException(e);
This example may be used when the method this code is executed in does not declare to throw a checked Exception
. But it all depends on the case at hand.
BufferedReader
has alines
method returning allString
lines as a stream. With this, you could also implement it as follows:return br.lines().collect(toList());
I statically imported Collectors#toList
. Notice how this results in a List
and not an ArrayList
.
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
add a comment |Â
up vote
1
down vote
First of all, your call to
fr.close()
has no effect, becausefr
has already been closed bybr.close()
, sinceclose()
releases all underlying resources, which in this case includes other streams or readers thatbr
is wrapped around.Also, and more importantly, it is imperative that
br.close()
is called regardless of whether thetry
block succeeds or throws an exception. In your code, ifbr.readLine()
throws an exception, the reader will never be closed.To rectify this, the call to
br.readLine()
has to go into afinally
block instead of thetry
block, which would mean thatbr
has to be declared outside thetry
block:BufferedReader br = null; //need to initialize it here, or else the compiler will complain later
try
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
//...
catch (IOException e )
//...
finally
br.close();And this is what the try-with-resources statement mentioned by Koekje is a shortcut for.
add a comment |Â
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
Since Java 7, you can make use of try-with-resources, which would change the implementation to:
try (BufferedReader br = new BufferedReader(new FileReader(file)))
while ((lineRead = br.readLine()) != null)
data.add(lineRead);
catch (IOException e)
...
Some other tips:
- Your documentation is not telling the truth and incomplete, you have to add some return documentation. Also, for parameters, the first string represents the name, and what follows is the explanation. So it should be more like e.g.
@param file the file to read all lines from
. - Return the interface instead of the implementation, i.e. return
List
instead ofArrayList
, which means you can always change the implementation. Never just print the stack trace, wrap and throw an (unchecked) exception or log. For more information as to why, see why printStackTrace is considered bad practice. In case you want to propagate the exception nonetheless, you can wrap it in another exception, e.g.:
try
...
catch (IOException e)
throw new UncheckedIOException(e);
This example may be used when the method this code is executed in does not declare to throw a checked Exception
. But it all depends on the case at hand.
BufferedReader
has alines
method returning allString
lines as a stream. With this, you could also implement it as follows:return br.lines().collect(toList());
I statically imported Collectors#toList
. Notice how this results in a List
and not an ArrayList
.
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
add a comment |Â
up vote
3
down vote
accepted
Since Java 7, you can make use of try-with-resources, which would change the implementation to:
try (BufferedReader br = new BufferedReader(new FileReader(file)))
while ((lineRead = br.readLine()) != null)
data.add(lineRead);
catch (IOException e)
...
Some other tips:
- Your documentation is not telling the truth and incomplete, you have to add some return documentation. Also, for parameters, the first string represents the name, and what follows is the explanation. So it should be more like e.g.
@param file the file to read all lines from
. - Return the interface instead of the implementation, i.e. return
List
instead ofArrayList
, which means you can always change the implementation. Never just print the stack trace, wrap and throw an (unchecked) exception or log. For more information as to why, see why printStackTrace is considered bad practice. In case you want to propagate the exception nonetheless, you can wrap it in another exception, e.g.:
try
...
catch (IOException e)
throw new UncheckedIOException(e);
This example may be used when the method this code is executed in does not declare to throw a checked Exception
. But it all depends on the case at hand.
BufferedReader
has alines
method returning allString
lines as a stream. With this, you could also implement it as follows:return br.lines().collect(toList());
I statically imported Collectors#toList
. Notice how this results in a List
and not an ArrayList
.
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
add a comment |Â
up vote
3
down vote
accepted
up vote
3
down vote
accepted
Since Java 7, you can make use of try-with-resources, which would change the implementation to:
try (BufferedReader br = new BufferedReader(new FileReader(file)))
while ((lineRead = br.readLine()) != null)
data.add(lineRead);
catch (IOException e)
...
Some other tips:
- Your documentation is not telling the truth and incomplete, you have to add some return documentation. Also, for parameters, the first string represents the name, and what follows is the explanation. So it should be more like e.g.
@param file the file to read all lines from
. - Return the interface instead of the implementation, i.e. return
List
instead ofArrayList
, which means you can always change the implementation. Never just print the stack trace, wrap and throw an (unchecked) exception or log. For more information as to why, see why printStackTrace is considered bad practice. In case you want to propagate the exception nonetheless, you can wrap it in another exception, e.g.:
try
...
catch (IOException e)
throw new UncheckedIOException(e);
This example may be used when the method this code is executed in does not declare to throw a checked Exception
. But it all depends on the case at hand.
BufferedReader
has alines
method returning allString
lines as a stream. With this, you could also implement it as follows:return br.lines().collect(toList());
I statically imported Collectors#toList
. Notice how this results in a List
and not an ArrayList
.
Since Java 7, you can make use of try-with-resources, which would change the implementation to:
try (BufferedReader br = new BufferedReader(new FileReader(file)))
while ((lineRead = br.readLine()) != null)
data.add(lineRead);
catch (IOException e)
...
Some other tips:
- Your documentation is not telling the truth and incomplete, you have to add some return documentation. Also, for parameters, the first string represents the name, and what follows is the explanation. So it should be more like e.g.
@param file the file to read all lines from
. - Return the interface instead of the implementation, i.e. return
List
instead ofArrayList
, which means you can always change the implementation. Never just print the stack trace, wrap and throw an (unchecked) exception or log. For more information as to why, see why printStackTrace is considered bad practice. In case you want to propagate the exception nonetheless, you can wrap it in another exception, e.g.:
try
...
catch (IOException e)
throw new UncheckedIOException(e);
This example may be used when the method this code is executed in does not declare to throw a checked Exception
. But it all depends on the case at hand.
BufferedReader
has alines
method returning allString
lines as a stream. With this, you could also implement it as follows:return br.lines().collect(toList());
I statically imported Collectors#toList
. Notice how this results in a List
and not an ArrayList
.
edited May 1 at 23:05
answered May 1 at 21:45
Koekje
1,017211
1,017211
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
add a comment |Â
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
Could you talk about the throwing of an unchecked exception or log a little more?
– Kyle
May 1 at 22:04
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
You are welcome :) I was updating my answer until I though there must be an answer for this on StackOverflow, so I added a link for clarification. It is explained in depth over there!
– Koekje
May 1 at 22:44
add a comment |Â
up vote
1
down vote
First of all, your call to
fr.close()
has no effect, becausefr
has already been closed bybr.close()
, sinceclose()
releases all underlying resources, which in this case includes other streams or readers thatbr
is wrapped around.Also, and more importantly, it is imperative that
br.close()
is called regardless of whether thetry
block succeeds or throws an exception. In your code, ifbr.readLine()
throws an exception, the reader will never be closed.To rectify this, the call to
br.readLine()
has to go into afinally
block instead of thetry
block, which would mean thatbr
has to be declared outside thetry
block:BufferedReader br = null; //need to initialize it here, or else the compiler will complain later
try
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
//...
catch (IOException e )
//...
finally
br.close();And this is what the try-with-resources statement mentioned by Koekje is a shortcut for.
add a comment |Â
up vote
1
down vote
First of all, your call to
fr.close()
has no effect, becausefr
has already been closed bybr.close()
, sinceclose()
releases all underlying resources, which in this case includes other streams or readers thatbr
is wrapped around.Also, and more importantly, it is imperative that
br.close()
is called regardless of whether thetry
block succeeds or throws an exception. In your code, ifbr.readLine()
throws an exception, the reader will never be closed.To rectify this, the call to
br.readLine()
has to go into afinally
block instead of thetry
block, which would mean thatbr
has to be declared outside thetry
block:BufferedReader br = null; //need to initialize it here, or else the compiler will complain later
try
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
//...
catch (IOException e )
//...
finally
br.close();And this is what the try-with-resources statement mentioned by Koekje is a shortcut for.
add a comment |Â
up vote
1
down vote
up vote
1
down vote
First of all, your call to
fr.close()
has no effect, becausefr
has already been closed bybr.close()
, sinceclose()
releases all underlying resources, which in this case includes other streams or readers thatbr
is wrapped around.Also, and more importantly, it is imperative that
br.close()
is called regardless of whether thetry
block succeeds or throws an exception. In your code, ifbr.readLine()
throws an exception, the reader will never be closed.To rectify this, the call to
br.readLine()
has to go into afinally
block instead of thetry
block, which would mean thatbr
has to be declared outside thetry
block:BufferedReader br = null; //need to initialize it here, or else the compiler will complain later
try
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
//...
catch (IOException e )
//...
finally
br.close();And this is what the try-with-resources statement mentioned by Koekje is a shortcut for.
First of all, your call to
fr.close()
has no effect, becausefr
has already been closed bybr.close()
, sinceclose()
releases all underlying resources, which in this case includes other streams or readers thatbr
is wrapped around.Also, and more importantly, it is imperative that
br.close()
is called regardless of whether thetry
block succeeds or throws an exception. In your code, ifbr.readLine()
throws an exception, the reader will never be closed.To rectify this, the call to
br.readLine()
has to go into afinally
block instead of thetry
block, which would mean thatbr
has to be declared outside thetry
block:BufferedReader br = null; //need to initialize it here, or else the compiler will complain later
try
FileReader fr = new FileReader(file);
br = new BufferedReader(fr);
//...
catch (IOException e )
//...
finally
br.close();And this is what the try-with-resources statement mentioned by Koekje is a shortcut for.
answered May 1 at 22:57
Stingy
1,888212
1,888212
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f193393%2fusing-bufferedreader-to-read-lines-of-a-file-into-a-list%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Welcome to Code Review! I hope you get some great answers.
– Phrancis
May 1 at 20:58
Thank you, I am a noobie to Code Review, so please forgive me!
– Kyle
May 1 at 21:00