Java: Constructor- and Method-overloading exercise
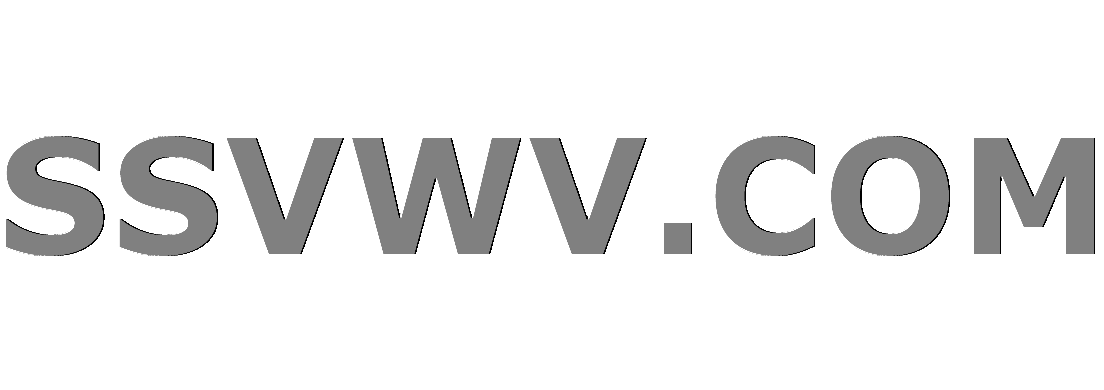
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
It's from a Java-course I'm enrolled into.
The classes "Instructor" and "Book" are already provided.
You got to write the different constructors of "Instructor" as well as the methods "getMostRecentBookTitle" and overloaded "updateBook".
Here's are the final classes expanded with my additional code:
// ------- Class Instructor ----------------------------------------
public class Instructor
public long id;
public String name;
public String title;
public String department;
public Book books;
public Instructor(long id, String name, String title, String department, Book books)
this(id, name, title, department);
this.books = books;
public Instructor(long id, String name, String title, String department)
this(id, name, title);
this.department = department;
public Instructor(long id, String name, String title)
this(id, name);
this.title = title;
public Instructor(long id, String name)
this.id = id;
this.name = name;
public String getMostRecentBookTitle()
return books[books.length - 1].title;
public Book updateBook(int index, String title)
Book retBook = books[0];
Book updatedBooks = new Book[books.length + 1];
int i = 0;
int j = 0;
while (i < updatedBooks.length)
if (i == index)
updatedBooks[i] = new Book(title);
retBook = books[i];
else
updatedBooks[i] = books[j];
i++;
if (i != index)
j++;
books = updatedBooks;
return retBook;
public Book updateBook(int index, Book book)
for (int i = 0; i < books.length; i++)
if (i == index)
Book oldBook = books[i];
books[i] = book;
return oldBook;
return new Book("");
public static void main(String args)
Book book1 = new Book("Wind Energy Design");
Book book2 = new Book("Aerodynamics");
Book book3 = new Book("Effective Java");
Instructor instructor =
new Instructor( 248,
"Walter", "Lecturer", "Mechanical Engineering",
new Bookbook1, book2, book3);
System.out.println(instructor.getMostRecentBookTitle());
System.out.println("Title of old book: " + instructor.updateBook(1, "Vector mechanics").getTitle());
Book book4 = new Book("Thermodynamics");
System.out.println("Title of old book: " + instructor.updateBook(1, book4).getTitle());
// ------- Class Book ----------------------------------------
public class Book
public String title;
public Book(String title)
this.title = title;
public void setTitle(String title)
this.title = title;
public String getTitle()
return title;
It works well and it has passed the automated tests.
But I'm unsatisfied with the method "updateBook(int index, String title)". That's just too much code for to accomplish such a simple task. In my opinion.
In JavaScript they have these method "splice". I guess that won't work in Java because Array-sizes can't be changed after construction.
Nevertheless: Is there a better way to write that method in Java?
Other hints, comments and recommendations concerning my implementation welcomed too.
java beginner algorithm
add a comment |Â
up vote
2
down vote
favorite
It's from a Java-course I'm enrolled into.
The classes "Instructor" and "Book" are already provided.
You got to write the different constructors of "Instructor" as well as the methods "getMostRecentBookTitle" and overloaded "updateBook".
Here's are the final classes expanded with my additional code:
// ------- Class Instructor ----------------------------------------
public class Instructor
public long id;
public String name;
public String title;
public String department;
public Book books;
public Instructor(long id, String name, String title, String department, Book books)
this(id, name, title, department);
this.books = books;
public Instructor(long id, String name, String title, String department)
this(id, name, title);
this.department = department;
public Instructor(long id, String name, String title)
this(id, name);
this.title = title;
public Instructor(long id, String name)
this.id = id;
this.name = name;
public String getMostRecentBookTitle()
return books[books.length - 1].title;
public Book updateBook(int index, String title)
Book retBook = books[0];
Book updatedBooks = new Book[books.length + 1];
int i = 0;
int j = 0;
while (i < updatedBooks.length)
if (i == index)
updatedBooks[i] = new Book(title);
retBook = books[i];
else
updatedBooks[i] = books[j];
i++;
if (i != index)
j++;
books = updatedBooks;
return retBook;
public Book updateBook(int index, Book book)
for (int i = 0; i < books.length; i++)
if (i == index)
Book oldBook = books[i];
books[i] = book;
return oldBook;
return new Book("");
public static void main(String args)
Book book1 = new Book("Wind Energy Design");
Book book2 = new Book("Aerodynamics");
Book book3 = new Book("Effective Java");
Instructor instructor =
new Instructor( 248,
"Walter", "Lecturer", "Mechanical Engineering",
new Bookbook1, book2, book3);
System.out.println(instructor.getMostRecentBookTitle());
System.out.println("Title of old book: " + instructor.updateBook(1, "Vector mechanics").getTitle());
Book book4 = new Book("Thermodynamics");
System.out.println("Title of old book: " + instructor.updateBook(1, book4).getTitle());
// ------- Class Book ----------------------------------------
public class Book
public String title;
public Book(String title)
this.title = title;
public void setTitle(String title)
this.title = title;
public String getTitle()
return title;
It works well and it has passed the automated tests.
But I'm unsatisfied with the method "updateBook(int index, String title)". That's just too much code for to accomplish such a simple task. In my opinion.
In JavaScript they have these method "splice". I guess that won't work in Java because Array-sizes can't be changed after construction.
Nevertheless: Is there a better way to write that method in Java?
Other hints, comments and recommendations concerning my implementation welcomed too.
java beginner algorithm
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
It's from a Java-course I'm enrolled into.
The classes "Instructor" and "Book" are already provided.
You got to write the different constructors of "Instructor" as well as the methods "getMostRecentBookTitle" and overloaded "updateBook".
Here's are the final classes expanded with my additional code:
// ------- Class Instructor ----------------------------------------
public class Instructor
public long id;
public String name;
public String title;
public String department;
public Book books;
public Instructor(long id, String name, String title, String department, Book books)
this(id, name, title, department);
this.books = books;
public Instructor(long id, String name, String title, String department)
this(id, name, title);
this.department = department;
public Instructor(long id, String name, String title)
this(id, name);
this.title = title;
public Instructor(long id, String name)
this.id = id;
this.name = name;
public String getMostRecentBookTitle()
return books[books.length - 1].title;
public Book updateBook(int index, String title)
Book retBook = books[0];
Book updatedBooks = new Book[books.length + 1];
int i = 0;
int j = 0;
while (i < updatedBooks.length)
if (i == index)
updatedBooks[i] = new Book(title);
retBook = books[i];
else
updatedBooks[i] = books[j];
i++;
if (i != index)
j++;
books = updatedBooks;
return retBook;
public Book updateBook(int index, Book book)
for (int i = 0; i < books.length; i++)
if (i == index)
Book oldBook = books[i];
books[i] = book;
return oldBook;
return new Book("");
public static void main(String args)
Book book1 = new Book("Wind Energy Design");
Book book2 = new Book("Aerodynamics");
Book book3 = new Book("Effective Java");
Instructor instructor =
new Instructor( 248,
"Walter", "Lecturer", "Mechanical Engineering",
new Bookbook1, book2, book3);
System.out.println(instructor.getMostRecentBookTitle());
System.out.println("Title of old book: " + instructor.updateBook(1, "Vector mechanics").getTitle());
Book book4 = new Book("Thermodynamics");
System.out.println("Title of old book: " + instructor.updateBook(1, book4).getTitle());
// ------- Class Book ----------------------------------------
public class Book
public String title;
public Book(String title)
this.title = title;
public void setTitle(String title)
this.title = title;
public String getTitle()
return title;
It works well and it has passed the automated tests.
But I'm unsatisfied with the method "updateBook(int index, String title)". That's just too much code for to accomplish such a simple task. In my opinion.
In JavaScript they have these method "splice". I guess that won't work in Java because Array-sizes can't be changed after construction.
Nevertheless: Is there a better way to write that method in Java?
Other hints, comments and recommendations concerning my implementation welcomed too.
java beginner algorithm
It's from a Java-course I'm enrolled into.
The classes "Instructor" and "Book" are already provided.
You got to write the different constructors of "Instructor" as well as the methods "getMostRecentBookTitle" and overloaded "updateBook".
Here's are the final classes expanded with my additional code:
// ------- Class Instructor ----------------------------------------
public class Instructor
public long id;
public String name;
public String title;
public String department;
public Book books;
public Instructor(long id, String name, String title, String department, Book books)
this(id, name, title, department);
this.books = books;
public Instructor(long id, String name, String title, String department)
this(id, name, title);
this.department = department;
public Instructor(long id, String name, String title)
this(id, name);
this.title = title;
public Instructor(long id, String name)
this.id = id;
this.name = name;
public String getMostRecentBookTitle()
return books[books.length - 1].title;
public Book updateBook(int index, String title)
Book retBook = books[0];
Book updatedBooks = new Book[books.length + 1];
int i = 0;
int j = 0;
while (i < updatedBooks.length)
if (i == index)
updatedBooks[i] = new Book(title);
retBook = books[i];
else
updatedBooks[i] = books[j];
i++;
if (i != index)
j++;
books = updatedBooks;
return retBook;
public Book updateBook(int index, Book book)
for (int i = 0; i < books.length; i++)
if (i == index)
Book oldBook = books[i];
books[i] = book;
return oldBook;
return new Book("");
public static void main(String args)
Book book1 = new Book("Wind Energy Design");
Book book2 = new Book("Aerodynamics");
Book book3 = new Book("Effective Java");
Instructor instructor =
new Instructor( 248,
"Walter", "Lecturer", "Mechanical Engineering",
new Bookbook1, book2, book3);
System.out.println(instructor.getMostRecentBookTitle());
System.out.println("Title of old book: " + instructor.updateBook(1, "Vector mechanics").getTitle());
Book book4 = new Book("Thermodynamics");
System.out.println("Title of old book: " + instructor.updateBook(1, book4).getTitle());
// ------- Class Book ----------------------------------------
public class Book
public String title;
public Book(String title)
this.title = title;
public void setTitle(String title)
this.title = title;
public String getTitle()
return title;
It works well and it has passed the automated tests.
But I'm unsatisfied with the method "updateBook(int index, String title)". That's just too much code for to accomplish such a simple task. In my opinion.
In JavaScript they have these method "splice". I guess that won't work in Java because Array-sizes can't be changed after construction.
Nevertheless: Is there a better way to write that method in Java?
Other hints, comments and recommendations concerning my implementation welcomed too.
java beginner algorithm
asked Apr 27 at 9:14
michael.zech
1,5911028
1,5911028
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
In java, you can just set the value at the given index using that index. Thus, starting out with the simpler update book (which uses the book object), this becomes:
public Book updateBook(int index, Book book)
Book oldBook = books[index];
books[index] = book;
return oldBook;
Alike in the ... string title ... method, but now, you can even use the method from before:
public Book updateBook(int index, String title)
return updateBook(index, new Book(title));
Disclaimer: this has not been typed in an IDE or compiled, but just done on the fly, thus it might include bugs. But the concept should be clear.
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
In java, you can just set the value at the given index using that index. Thus, starting out with the simpler update book (which uses the book object), this becomes:
public Book updateBook(int index, Book book)
Book oldBook = books[index];
books[index] = book;
return oldBook;
Alike in the ... string title ... method, but now, you can even use the method from before:
public Book updateBook(int index, String title)
return updateBook(index, new Book(title));
Disclaimer: this has not been typed in an IDE or compiled, but just done on the fly, thus it might include bugs. But the concept should be clear.
add a comment |Â
up vote
3
down vote
accepted
In java, you can just set the value at the given index using that index. Thus, starting out with the simpler update book (which uses the book object), this becomes:
public Book updateBook(int index, Book book)
Book oldBook = books[index];
books[index] = book;
return oldBook;
Alike in the ... string title ... method, but now, you can even use the method from before:
public Book updateBook(int index, String title)
return updateBook(index, new Book(title));
Disclaimer: this has not been typed in an IDE or compiled, but just done on the fly, thus it might include bugs. But the concept should be clear.
add a comment |Â
up vote
3
down vote
accepted
up vote
3
down vote
accepted
In java, you can just set the value at the given index using that index. Thus, starting out with the simpler update book (which uses the book object), this becomes:
public Book updateBook(int index, Book book)
Book oldBook = books[index];
books[index] = book;
return oldBook;
Alike in the ... string title ... method, but now, you can even use the method from before:
public Book updateBook(int index, String title)
return updateBook(index, new Book(title));
Disclaimer: this has not been typed in an IDE or compiled, but just done on the fly, thus it might include bugs. But the concept should be clear.
In java, you can just set the value at the given index using that index. Thus, starting out with the simpler update book (which uses the book object), this becomes:
public Book updateBook(int index, Book book)
Book oldBook = books[index];
books[index] = book;
return oldBook;
Alike in the ... string title ... method, but now, you can even use the method from before:
public Book updateBook(int index, String title)
return updateBook(index, new Book(title));
Disclaimer: this has not been typed in an IDE or compiled, but just done on the fly, thus it might include bugs. But the concept should be clear.
edited Apr 27 at 9:31
answered Apr 27 at 9:26
mtj
2,675212
2,675212
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f193061%2fjava-constructor-and-method-overloading-exercise%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password