spiral copy input matrix snake
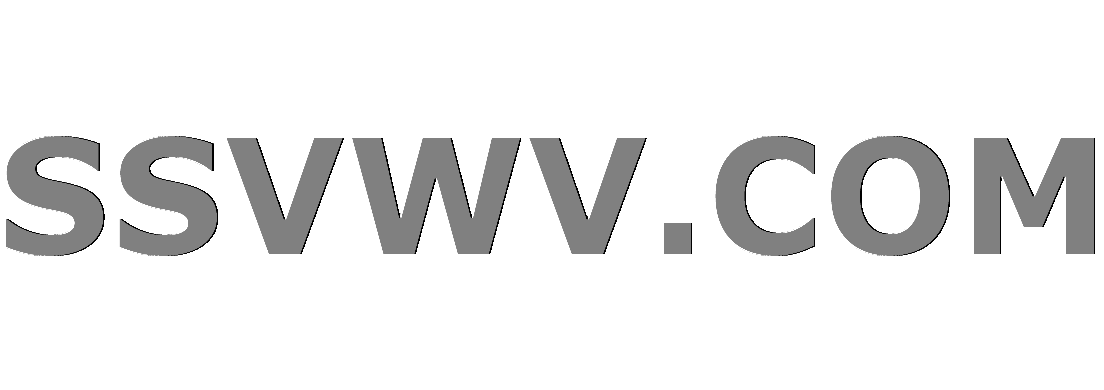
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
0
down vote
favorite
I found this interview question online at Pramp:
Given a 2D array (matrix) inputMatrix of integers, create a function spiralCopy that copies inputMatrix’s values into a 1D array in a spiral order, clockwise. Your function then should return that array. Analyze the time and space complexities of your solution.
Example:
input: inputMatrix = [ [1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15], [16, 17, 18, 19, 20] ]
output: [1, 2, 3, 4, 5, 10, 15, 20, 19, 18, 17, 16, 11, 6, 7, 8, 9, 14, 13, 12]
See the illustration below to understand better what a clockwise spiral order looks like. Clockwise spiral order
[input] array.array.integer inputMatrix
$1 ≤ inputMatrix[0].length ≤ 100 1 ≤ inputMatrix.length ≤ 100 $
[output] array.integer
My solution
def spiral_copy(inputMatrix):
output =
top_row = 0
bottom_row = len(inputMatrix) - 1
left_col = 0
right_col = len(inputMatrix[0]) - 1
while top_row <= bottom_row and left_col <= right_col:
for i in range(left_col, right_col + 1):
output.append(inputMatrix[top_row][i])
top_row += 1
for i in range(top_row, bottom_row + 1):
output.append(inputMatrix[i][right_col])
right_col -= 1
if top_row > bottom_row: break
for i in range(right_col, left_col - 1, -1):
output.append(inputMatrix[bottom_row][i])
bottom_row -= 1
if left_col > right_col: break
for i in range(bottom_row, top_row - 1, -1):
output.append(inputMatrix[i][left_col])
left_col += 1
return output
Passed these Test cases:
Input
[[1,2],[3,4]]
Expected Result
[1, 2, 4, 3]
Input
[[1,2],[3,4]
Expected Result
[1, 2, 4, 3]
Input
[[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Expected Result
[1, 2, 3, 4, 8, 12, 11, 10, 9, 5, 6, 7]
input
[[1,2],[3,4]
Expected Result
[1, 2, 3, 4, 5, 6, 12, 18, 17, 16, 15, 14, 13, 7, 8, 9, 10, 11]
Input
[[1,0],[0,1]]
Expected Result
[1, 0, 1, 0]
Input
[[1,2,3],[4,5,6],[7,8,9]]
Expected Result
[1, 2, 3, 6, 9, 8, 7, 4, 5]
python interview-questions
add a comment |Â
up vote
0
down vote
favorite
I found this interview question online at Pramp:
Given a 2D array (matrix) inputMatrix of integers, create a function spiralCopy that copies inputMatrix’s values into a 1D array in a spiral order, clockwise. Your function then should return that array. Analyze the time and space complexities of your solution.
Example:
input: inputMatrix = [ [1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15], [16, 17, 18, 19, 20] ]
output: [1, 2, 3, 4, 5, 10, 15, 20, 19, 18, 17, 16, 11, 6, 7, 8, 9, 14, 13, 12]
See the illustration below to understand better what a clockwise spiral order looks like. Clockwise spiral order
[input] array.array.integer inputMatrix
$1 ≤ inputMatrix[0].length ≤ 100 1 ≤ inputMatrix.length ≤ 100 $
[output] array.integer
My solution
def spiral_copy(inputMatrix):
output =
top_row = 0
bottom_row = len(inputMatrix) - 1
left_col = 0
right_col = len(inputMatrix[0]) - 1
while top_row <= bottom_row and left_col <= right_col:
for i in range(left_col, right_col + 1):
output.append(inputMatrix[top_row][i])
top_row += 1
for i in range(top_row, bottom_row + 1):
output.append(inputMatrix[i][right_col])
right_col -= 1
if top_row > bottom_row: break
for i in range(right_col, left_col - 1, -1):
output.append(inputMatrix[bottom_row][i])
bottom_row -= 1
if left_col > right_col: break
for i in range(bottom_row, top_row - 1, -1):
output.append(inputMatrix[i][left_col])
left_col += 1
return output
Passed these Test cases:
Input
[[1,2],[3,4]]
Expected Result
[1, 2, 4, 3]
Input
[[1,2],[3,4]
Expected Result
[1, 2, 4, 3]
Input
[[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Expected Result
[1, 2, 3, 4, 8, 12, 11, 10, 9, 5, 6, 7]
input
[[1,2],[3,4]
Expected Result
[1, 2, 3, 4, 5, 6, 12, 18, 17, 16, 15, 14, 13, 7, 8, 9, 10, 11]
Input
[[1,0],[0,1]]
Expected Result
[1, 0, 1, 0]
Input
[[1,2,3],[4,5,6],[7,8,9]]
Expected Result
[1, 2, 3, 6, 9, 8, 7, 4, 5]
python interview-questions
add a comment |Â
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I found this interview question online at Pramp:
Given a 2D array (matrix) inputMatrix of integers, create a function spiralCopy that copies inputMatrix’s values into a 1D array in a spiral order, clockwise. Your function then should return that array. Analyze the time and space complexities of your solution.
Example:
input: inputMatrix = [ [1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15], [16, 17, 18, 19, 20] ]
output: [1, 2, 3, 4, 5, 10, 15, 20, 19, 18, 17, 16, 11, 6, 7, 8, 9, 14, 13, 12]
See the illustration below to understand better what a clockwise spiral order looks like. Clockwise spiral order
[input] array.array.integer inputMatrix
$1 ≤ inputMatrix[0].length ≤ 100 1 ≤ inputMatrix.length ≤ 100 $
[output] array.integer
My solution
def spiral_copy(inputMatrix):
output =
top_row = 0
bottom_row = len(inputMatrix) - 1
left_col = 0
right_col = len(inputMatrix[0]) - 1
while top_row <= bottom_row and left_col <= right_col:
for i in range(left_col, right_col + 1):
output.append(inputMatrix[top_row][i])
top_row += 1
for i in range(top_row, bottom_row + 1):
output.append(inputMatrix[i][right_col])
right_col -= 1
if top_row > bottom_row: break
for i in range(right_col, left_col - 1, -1):
output.append(inputMatrix[bottom_row][i])
bottom_row -= 1
if left_col > right_col: break
for i in range(bottom_row, top_row - 1, -1):
output.append(inputMatrix[i][left_col])
left_col += 1
return output
Passed these Test cases:
Input
[[1,2],[3,4]]
Expected Result
[1, 2, 4, 3]
Input
[[1,2],[3,4]
Expected Result
[1, 2, 4, 3]
Input
[[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Expected Result
[1, 2, 3, 4, 8, 12, 11, 10, 9, 5, 6, 7]
input
[[1,2],[3,4]
Expected Result
[1, 2, 3, 4, 5, 6, 12, 18, 17, 16, 15, 14, 13, 7, 8, 9, 10, 11]
Input
[[1,0],[0,1]]
Expected Result
[1, 0, 1, 0]
Input
[[1,2,3],[4,5,6],[7,8,9]]
Expected Result
[1, 2, 3, 6, 9, 8, 7, 4, 5]
python interview-questions
I found this interview question online at Pramp:
Given a 2D array (matrix) inputMatrix of integers, create a function spiralCopy that copies inputMatrix’s values into a 1D array in a spiral order, clockwise. Your function then should return that array. Analyze the time and space complexities of your solution.
Example:
input: inputMatrix = [ [1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15], [16, 17, 18, 19, 20] ]
output: [1, 2, 3, 4, 5, 10, 15, 20, 19, 18, 17, 16, 11, 6, 7, 8, 9, 14, 13, 12]
See the illustration below to understand better what a clockwise spiral order looks like. Clockwise spiral order
[input] array.array.integer inputMatrix
$1 ≤ inputMatrix[0].length ≤ 100 1 ≤ inputMatrix.length ≤ 100 $
[output] array.integer
My solution
def spiral_copy(inputMatrix):
output =
top_row = 0
bottom_row = len(inputMatrix) - 1
left_col = 0
right_col = len(inputMatrix[0]) - 1
while top_row <= bottom_row and left_col <= right_col:
for i in range(left_col, right_col + 1):
output.append(inputMatrix[top_row][i])
top_row += 1
for i in range(top_row, bottom_row + 1):
output.append(inputMatrix[i][right_col])
right_col -= 1
if top_row > bottom_row: break
for i in range(right_col, left_col - 1, -1):
output.append(inputMatrix[bottom_row][i])
bottom_row -= 1
if left_col > right_col: break
for i in range(bottom_row, top_row - 1, -1):
output.append(inputMatrix[i][left_col])
left_col += 1
return output
Passed these Test cases:
Input
[[1,2],[3,4]]
Expected Result
[1, 2, 4, 3]
Input
[[1,2],[3,4]
Expected Result
[1, 2, 4, 3]
Input
[[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Expected Result
[1, 2, 3, 4, 8, 12, 11, 10, 9, 5, 6, 7]
input
[[1,2],[3,4]
Expected Result
[1, 2, 3, 4, 5, 6, 12, 18, 17, 16, 15, 14, 13, 7, 8, 9, 10, 11]
Input
[[1,0],[0,1]]
Expected Result
[1, 0, 1, 0]
Input
[[1,2,3],[4,5,6],[7,8,9]]
Expected Result
[1, 2, 3, 6, 9, 8, 7, 4, 5]
python interview-questions
edited Mar 20 at 23:13


Tola
1,78551135
1,78551135
asked Mar 20 at 17:40
NinjaG
756221
756221
add a comment |Â
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f190053%2fspiral-copy-input-matrix-snake%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password