Merge Sort Cleanup
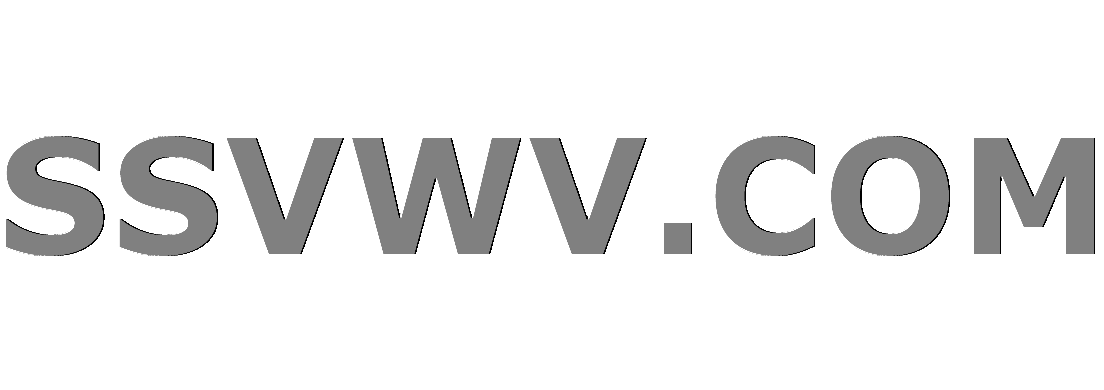
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I am re-learning my fundamental algorithms, and have been told my Python is overly verbose.
Can you take a look at my merge sort algorithm and let me know how you would clean it up and make it more pythonic, if applicable?
def merge(left,right):
out =
l_rest =
i = left[0]
while i is not None:
if right:
if i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
else:
out.append(i)
left.remove(i)
if len(left) > 0:
i = left[0]
else:
i = None
for i in l_rest:
out.append(i)
for i in right:
out.append(i)
return out
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
python python-3.x mergesort
add a comment |Â
up vote
1
down vote
favorite
I am re-learning my fundamental algorithms, and have been told my Python is overly verbose.
Can you take a look at my merge sort algorithm and let me know how you would clean it up and make it more pythonic, if applicable?
def merge(left,right):
out =
l_rest =
i = left[0]
while i is not None:
if right:
if i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
else:
out.append(i)
left.remove(i)
if len(left) > 0:
i = left[0]
else:
i = None
for i in l_rest:
out.append(i)
for i in right:
out.append(i)
return out
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
python python-3.x mergesort
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
1
why not justout += l_rest + right
instead offor i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am re-learning my fundamental algorithms, and have been told my Python is overly verbose.
Can you take a look at my merge sort algorithm and let me know how you would clean it up and make it more pythonic, if applicable?
def merge(left,right):
out =
l_rest =
i = left[0]
while i is not None:
if right:
if i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
else:
out.append(i)
left.remove(i)
if len(left) > 0:
i = left[0]
else:
i = None
for i in l_rest:
out.append(i)
for i in right:
out.append(i)
return out
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
python python-3.x mergesort
I am re-learning my fundamental algorithms, and have been told my Python is overly verbose.
Can you take a look at my merge sort algorithm and let me know how you would clean it up and make it more pythonic, if applicable?
def merge(left,right):
out =
l_rest =
i = left[0]
while i is not None:
if right:
if i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
else:
out.append(i)
left.remove(i)
if len(left) > 0:
i = left[0]
else:
i = None
for i in l_rest:
out.append(i)
for i in right:
out.append(i)
return out
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
python python-3.x mergesort
edited Apr 5 at 4:59
asked Apr 4 at 14:03
Chris
1063
1063
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
1
why not justout += l_rest + right
instead offor i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01
add a comment |Â
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
1
why not justout += l_rest + right
instead offor i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
1
1
why not just
out += l_rest + right
instead of for i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
why not just
out += l_rest + right
instead of for i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
-1
down vote
Starting a wiki with the suggested edits in the comments so far.
def merge(left,right):
out =
i = left[0]
while i is not None:
if right and i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
if len(left) > 0:
i = left[0]
else:
i = None
return out + left + right
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
Starting a wiki with the suggested edits in the comments so far.
def merge(left,right):
out =
i = left[0]
while i is not None:
if right and i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
if len(left) > 0:
i = left[0]
else:
i = None
return out + left + right
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
add a comment |Â
up vote
-1
down vote
Starting a wiki with the suggested edits in the comments so far.
def merge(left,right):
out =
i = left[0]
while i is not None:
if right and i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
if len(left) > 0:
i = left[0]
else:
i = None
return out + left + right
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
add a comment |Â
up vote
-1
down vote
up vote
-1
down vote
Starting a wiki with the suggested edits in the comments so far.
def merge(left,right):
out =
i = left[0]
while i is not None:
if right and i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
if len(left) > 0:
i = left[0]
else:
i = None
return out + left + right
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
Starting a wiki with the suggested edits in the comments so far.
def merge(left,right):
out =
i = left[0]
while i is not None:
if right and i < right[0]:
out.append(i)
left.remove(i)
else:
out.append(right[0])
right.remove(right[0])
if len(left) > 0:
i = left[0]
else:
i = None
return out + left + right
def sort(lst):
if len(lst) == 1:
return lst
left = sort(lst[:len(lst)//2])
right = sort(lst[len(lst)//2:])
return merge(left,right)
answered Apr 10 at 3:18
community wiki
Chris
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f191254%2fmerge-sort-cleanup%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Your code seems to have a bug: it doesn't include the pivot in the result.
– Solomon Ucko
Apr 4 at 16:18
@SolomonUcko Thanks, not sure I follow, but I will debug tonight.
– Chris
Apr 4 at 16:49
Sorry, at first I thought it was quicksort. What I meant was that, for example, if I sort a list of the numbers 0-99, repeated 100 times, then shuffled, the 0s are missing.
– Solomon Ucko
Apr 4 at 17:01
1
why not just
out += l_rest + right
instead offor i in l_rest: out.append(i); for i in right: out.append(i)
– Yulia V
Apr 4 at 18:21
@SolomonUcko fixed, that was nasty. Thanks. I chose to fix that and not fix YuliaV's suggestion inline... Not sure the best practice for implementing incremental improvements, but I like their idea.
– Chris
Apr 5 at 5:01