Basic to-do CLI app (with JSON for persistence) using Python
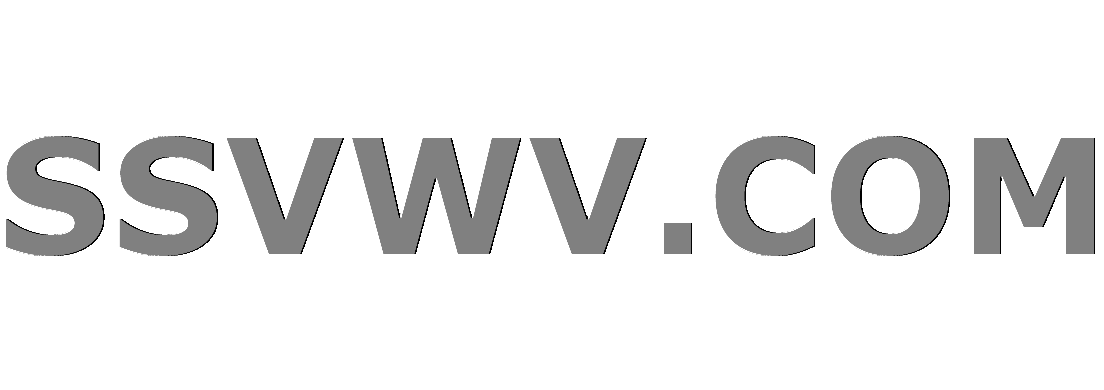
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
2
down vote
favorite
I’m very new to Python. I wanted to start out with a simple project: a CLI app that keeps track of my to-do’s.
The code works as intended, but is what I have written is any good?
File structure:
task.py
defines the task classtask_handler.py
defines a class that helps retrieve/store the taskscommand.py
ties everything together and processes the command
Files:
task.py
class Task:
def __init__(self, description):
self.description = description
self.completed = False
def complete(self):
self.completed = True
task_handler.py
import json
from task import Task
class TaskHandler:
def __init__(self, path):
self.path = path
self.stored_attributes = ['description', 'completed']
def store(self, tasks):
data =
for task in tasks:
obj =
for attr in self.stored_attributes:
obj[attr] = getattr(task, attr, None)
data.append(obj)
with open(self.path, 'w') as data_file:
json.dump(data, data_file)
def retrieve(self):
data =
try:
with open(self.path, 'r') as data_file:
data = json.load(data_file)
except FileNotFoundError:
pass
tasks =
for task in data:
obj = Task(task['description'])
for attr in self.stored_attributes:
setattr(obj, attr, task[attr])
tasks.append(obj)
return tasks
command.py
import os
import sys
import argparse
from task import Task
from task_handler import TaskHandler
def parse_args(args):
parser = argparse.ArgumentParser()
action_parser = parser.add_subparsers(title='actions', dest='action')
action_parser.add_parser('list')
add_action_parser = action_parser.add_parser('add')
add_action_parser.add_argument('description')
complete_action_parser = action_parser.add_parser('complete')
complete_action_parser.add_argument('index', type=int)
purge_action_parser = action_parser.add_parser('purge')
purge_action_parser.add_argument('index', type=int)
return parser.parse_args(args)
def main():
args = parse_args(sys.argv[1:])
task_handler = TaskHandler(os.path.expanduser('~') + '/.todo.json')
stored_tasks = task_handler.retrieve()
if args.action == 'add':
new_task = Task(args.description)
stored_tasks.append(new_task)
task_handler.store(stored_tasks)
print('Task added')
elif args.action == 'complete':
try:
stored_tasks[args.index].complete()
task_handler.store(stored_tasks)
print('Task marked as completed')
except IndexError:
print('No task with that index')
elif args.action == 'purge':
try:
del stored_tasks[args.index]
task_handler.store(stored_tasks)
print('Task purged')
except IndexError:
print('No task with that index')
else:
if not stored_tasks:
print('To-do list is empty')
else:
print('To-do:')
for idx, task in enumerate(stored_tasks):
completed = ' (completed)' if task.completed else ''
print('[] '.format(idx, task.description, completed))
if __name__ == '__main__':
main()
Any input would be highly appreciated! I want to focus on becoming a better programmer in any way I can.
python json to-do-list
add a comment |Â
up vote
2
down vote
favorite
I’m very new to Python. I wanted to start out with a simple project: a CLI app that keeps track of my to-do’s.
The code works as intended, but is what I have written is any good?
File structure:
task.py
defines the task classtask_handler.py
defines a class that helps retrieve/store the taskscommand.py
ties everything together and processes the command
Files:
task.py
class Task:
def __init__(self, description):
self.description = description
self.completed = False
def complete(self):
self.completed = True
task_handler.py
import json
from task import Task
class TaskHandler:
def __init__(self, path):
self.path = path
self.stored_attributes = ['description', 'completed']
def store(self, tasks):
data =
for task in tasks:
obj =
for attr in self.stored_attributes:
obj[attr] = getattr(task, attr, None)
data.append(obj)
with open(self.path, 'w') as data_file:
json.dump(data, data_file)
def retrieve(self):
data =
try:
with open(self.path, 'r') as data_file:
data = json.load(data_file)
except FileNotFoundError:
pass
tasks =
for task in data:
obj = Task(task['description'])
for attr in self.stored_attributes:
setattr(obj, attr, task[attr])
tasks.append(obj)
return tasks
command.py
import os
import sys
import argparse
from task import Task
from task_handler import TaskHandler
def parse_args(args):
parser = argparse.ArgumentParser()
action_parser = parser.add_subparsers(title='actions', dest='action')
action_parser.add_parser('list')
add_action_parser = action_parser.add_parser('add')
add_action_parser.add_argument('description')
complete_action_parser = action_parser.add_parser('complete')
complete_action_parser.add_argument('index', type=int)
purge_action_parser = action_parser.add_parser('purge')
purge_action_parser.add_argument('index', type=int)
return parser.parse_args(args)
def main():
args = parse_args(sys.argv[1:])
task_handler = TaskHandler(os.path.expanduser('~') + '/.todo.json')
stored_tasks = task_handler.retrieve()
if args.action == 'add':
new_task = Task(args.description)
stored_tasks.append(new_task)
task_handler.store(stored_tasks)
print('Task added')
elif args.action == 'complete':
try:
stored_tasks[args.index].complete()
task_handler.store(stored_tasks)
print('Task marked as completed')
except IndexError:
print('No task with that index')
elif args.action == 'purge':
try:
del stored_tasks[args.index]
task_handler.store(stored_tasks)
print('Task purged')
except IndexError:
print('No task with that index')
else:
if not stored_tasks:
print('To-do list is empty')
else:
print('To-do:')
for idx, task in enumerate(stored_tasks):
completed = ' (completed)' if task.completed else ''
print('[] '.format(idx, task.description, completed))
if __name__ == '__main__':
main()
Any input would be highly appreciated! I want to focus on becoming a better programmer in any way I can.
python json to-do-list
add a comment |Â
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I’m very new to Python. I wanted to start out with a simple project: a CLI app that keeps track of my to-do’s.
The code works as intended, but is what I have written is any good?
File structure:
task.py
defines the task classtask_handler.py
defines a class that helps retrieve/store the taskscommand.py
ties everything together and processes the command
Files:
task.py
class Task:
def __init__(self, description):
self.description = description
self.completed = False
def complete(self):
self.completed = True
task_handler.py
import json
from task import Task
class TaskHandler:
def __init__(self, path):
self.path = path
self.stored_attributes = ['description', 'completed']
def store(self, tasks):
data =
for task in tasks:
obj =
for attr in self.stored_attributes:
obj[attr] = getattr(task, attr, None)
data.append(obj)
with open(self.path, 'w') as data_file:
json.dump(data, data_file)
def retrieve(self):
data =
try:
with open(self.path, 'r') as data_file:
data = json.load(data_file)
except FileNotFoundError:
pass
tasks =
for task in data:
obj = Task(task['description'])
for attr in self.stored_attributes:
setattr(obj, attr, task[attr])
tasks.append(obj)
return tasks
command.py
import os
import sys
import argparse
from task import Task
from task_handler import TaskHandler
def parse_args(args):
parser = argparse.ArgumentParser()
action_parser = parser.add_subparsers(title='actions', dest='action')
action_parser.add_parser('list')
add_action_parser = action_parser.add_parser('add')
add_action_parser.add_argument('description')
complete_action_parser = action_parser.add_parser('complete')
complete_action_parser.add_argument('index', type=int)
purge_action_parser = action_parser.add_parser('purge')
purge_action_parser.add_argument('index', type=int)
return parser.parse_args(args)
def main():
args = parse_args(sys.argv[1:])
task_handler = TaskHandler(os.path.expanduser('~') + '/.todo.json')
stored_tasks = task_handler.retrieve()
if args.action == 'add':
new_task = Task(args.description)
stored_tasks.append(new_task)
task_handler.store(stored_tasks)
print('Task added')
elif args.action == 'complete':
try:
stored_tasks[args.index].complete()
task_handler.store(stored_tasks)
print('Task marked as completed')
except IndexError:
print('No task with that index')
elif args.action == 'purge':
try:
del stored_tasks[args.index]
task_handler.store(stored_tasks)
print('Task purged')
except IndexError:
print('No task with that index')
else:
if not stored_tasks:
print('To-do list is empty')
else:
print('To-do:')
for idx, task in enumerate(stored_tasks):
completed = ' (completed)' if task.completed else ''
print('[] '.format(idx, task.description, completed))
if __name__ == '__main__':
main()
Any input would be highly appreciated! I want to focus on becoming a better programmer in any way I can.
python json to-do-list
I’m very new to Python. I wanted to start out with a simple project: a CLI app that keeps track of my to-do’s.
The code works as intended, but is what I have written is any good?
File structure:
task.py
defines the task classtask_handler.py
defines a class that helps retrieve/store the taskscommand.py
ties everything together and processes the command
Files:
task.py
class Task:
def __init__(self, description):
self.description = description
self.completed = False
def complete(self):
self.completed = True
task_handler.py
import json
from task import Task
class TaskHandler:
def __init__(self, path):
self.path = path
self.stored_attributes = ['description', 'completed']
def store(self, tasks):
data =
for task in tasks:
obj =
for attr in self.stored_attributes:
obj[attr] = getattr(task, attr, None)
data.append(obj)
with open(self.path, 'w') as data_file:
json.dump(data, data_file)
def retrieve(self):
data =
try:
with open(self.path, 'r') as data_file:
data = json.load(data_file)
except FileNotFoundError:
pass
tasks =
for task in data:
obj = Task(task['description'])
for attr in self.stored_attributes:
setattr(obj, attr, task[attr])
tasks.append(obj)
return tasks
command.py
import os
import sys
import argparse
from task import Task
from task_handler import TaskHandler
def parse_args(args):
parser = argparse.ArgumentParser()
action_parser = parser.add_subparsers(title='actions', dest='action')
action_parser.add_parser('list')
add_action_parser = action_parser.add_parser('add')
add_action_parser.add_argument('description')
complete_action_parser = action_parser.add_parser('complete')
complete_action_parser.add_argument('index', type=int)
purge_action_parser = action_parser.add_parser('purge')
purge_action_parser.add_argument('index', type=int)
return parser.parse_args(args)
def main():
args = parse_args(sys.argv[1:])
task_handler = TaskHandler(os.path.expanduser('~') + '/.todo.json')
stored_tasks = task_handler.retrieve()
if args.action == 'add':
new_task = Task(args.description)
stored_tasks.append(new_task)
task_handler.store(stored_tasks)
print('Task added')
elif args.action == 'complete':
try:
stored_tasks[args.index].complete()
task_handler.store(stored_tasks)
print('Task marked as completed')
except IndexError:
print('No task with that index')
elif args.action == 'purge':
try:
del stored_tasks[args.index]
task_handler.store(stored_tasks)
print('Task purged')
except IndexError:
print('No task with that index')
else:
if not stored_tasks:
print('To-do list is empty')
else:
print('To-do:')
for idx, task in enumerate(stored_tasks):
completed = ' (completed)' if task.completed else ''
print('[] '.format(idx, task.description, completed))
if __name__ == '__main__':
main()
Any input would be highly appreciated! I want to focus on becoming a better programmer in any way I can.
python json to-do-list
edited Apr 3 at 19:22


Sam Onela
5,81961544
5,81961544
asked Apr 3 at 19:06


VirtualRaccoon
384
384
add a comment |Â
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f191190%2fbasic-to-do-cli-app-with-json-for-persistence-using-python%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password