Text menu to browse CSV data about seal species
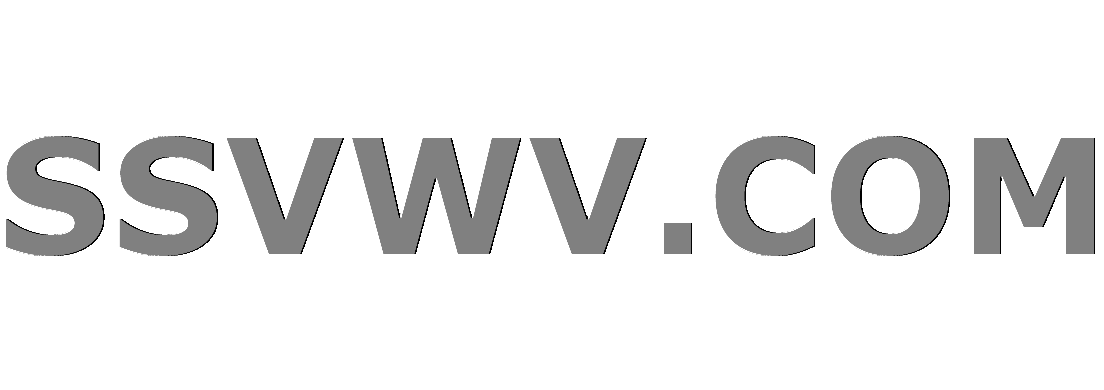
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
8
down vote
favorite
I am writing in C++, I have completed a small program which parses CSV files from IUCN and presents the species data using menus. I used a lot of loops. It works, however it is buggy when processing certain input errors.
I would like to know if there are better ways to write this program. Is there a way to use OOP? Would it be helpful to use OOP?
//EndangeredSeals.cpp
#include <iostream>
#include <sstream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
int main()
std::string entry;
std::vector<std::vector<std::string> > database;
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
if (file)
while (std::getline(file, entry))
size_t dbsize = database.size();
database.resize(dbsize + 1);
std::istringstream ss(entry);
std::string field, push_field("");
bool no_quotes = true;
while (std::getline(ss, field, ','))
if (static_cast<size_t>(std::count(field.begin(), field.end(), '"')) % 2 != 0)
no_quotes = !no_quotes;
field.erase(remove(field.begin(), field.end(), '"'), field.end());
push_field += field + (no_quotes ? "" : ",");
if (no_quotes)
database[dbsize].push_back(push_field);
push_field.resize(0);
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
int sealChoice;
int dataChoice;
int switchChoice;
bool moreData;
bool moreSeal = true;
while (moreSeal == true)
return 0;
c++ csv
add a comment |Â
up vote
8
down vote
favorite
I am writing in C++, I have completed a small program which parses CSV files from IUCN and presents the species data using menus. I used a lot of loops. It works, however it is buggy when processing certain input errors.
I would like to know if there are better ways to write this program. Is there a way to use OOP? Would it be helpful to use OOP?
//EndangeredSeals.cpp
#include <iostream>
#include <sstream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
int main()
std::string entry;
std::vector<std::vector<std::string> > database;
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
if (file)
while (std::getline(file, entry))
size_t dbsize = database.size();
database.resize(dbsize + 1);
std::istringstream ss(entry);
std::string field, push_field("");
bool no_quotes = true;
while (std::getline(ss, field, ','))
if (static_cast<size_t>(std::count(field.begin(), field.end(), '"')) % 2 != 0)
no_quotes = !no_quotes;
field.erase(remove(field.begin(), field.end(), '"'), field.end());
push_field += field + (no_quotes ? "" : ",");
if (no_quotes)
database[dbsize].push_back(push_field);
push_field.resize(0);
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
int sealChoice;
int dataChoice;
int switchChoice;
bool moreData;
bool moreSeal = true;
while (moreSeal == true)
return 0;
c++ csv
add a comment |Â
up vote
8
down vote
favorite
up vote
8
down vote
favorite
I am writing in C++, I have completed a small program which parses CSV files from IUCN and presents the species data using menus. I used a lot of loops. It works, however it is buggy when processing certain input errors.
I would like to know if there are better ways to write this program. Is there a way to use OOP? Would it be helpful to use OOP?
//EndangeredSeals.cpp
#include <iostream>
#include <sstream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
int main()
std::string entry;
std::vector<std::vector<std::string> > database;
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
if (file)
while (std::getline(file, entry))
size_t dbsize = database.size();
database.resize(dbsize + 1);
std::istringstream ss(entry);
std::string field, push_field("");
bool no_quotes = true;
while (std::getline(ss, field, ','))
if (static_cast<size_t>(std::count(field.begin(), field.end(), '"')) % 2 != 0)
no_quotes = !no_quotes;
field.erase(remove(field.begin(), field.end(), '"'), field.end());
push_field += field + (no_quotes ? "" : ",");
if (no_quotes)
database[dbsize].push_back(push_field);
push_field.resize(0);
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
int sealChoice;
int dataChoice;
int switchChoice;
bool moreData;
bool moreSeal = true;
while (moreSeal == true)
return 0;
c++ csv
I am writing in C++, I have completed a small program which parses CSV files from IUCN and presents the species data using menus. I used a lot of loops. It works, however it is buggy when processing certain input errors.
I would like to know if there are better ways to write this program. Is there a way to use OOP? Would it be helpful to use OOP?
//EndangeredSeals.cpp
#include <iostream>
#include <sstream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
int main()
std::string entry;
std::vector<std::vector<std::string> > database;
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
if (file)
while (std::getline(file, entry))
size_t dbsize = database.size();
database.resize(dbsize + 1);
std::istringstream ss(entry);
std::string field, push_field("");
bool no_quotes = true;
while (std::getline(ss, field, ','))
if (static_cast<size_t>(std::count(field.begin(), field.end(), '"')) % 2 != 0)
no_quotes = !no_quotes;
field.erase(remove(field.begin(), field.end(), '"'), field.end());
push_field += field + (no_quotes ? "" : ",");
if (no_quotes)
database[dbsize].push_back(push_field);
push_field.resize(0);
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
int sealChoice;
int dataChoice;
int switchChoice;
bool moreData;
bool moreSeal = true;
while (moreSeal == true)
return 0;
c++ csv
edited Aug 4 at 15:15


200_success
123k14143401
123k14143401
asked Jan 5 at 12:18
user157343
411
411
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
5
down vote
Reformat code
Try to use IDE options where you can reformat code, right now it is hard to read. There is everything in one place.
Create small functions
Right now, your code is a one big function. Firstly, divide your code into small functions that are doing one thing. You could end up with 10 or 15 really small functions. After that, thing about functions that are similar to each other, they are part of one bigger responsibility. You might be able to see some "objects" and create appropriate classes.
Hardcoding values
You have code like this
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
This can be done for example using command line arguments. You will be passing path to file when you start running your program, that will be much more flexible.
Next examples of hardcoding values
std::cout << database[sealChoice][14] << std::endl;
while (switchChoice <= 0 || switchChoice >= 4 || std::cin.fail())
Try to give these every number meaningful name, it will much more easier for readers to understand.
Strange code
I cannot get why do you do this
size_t dbsize = database.size();
database.resize(dbsize + 1);
Personally, I use resize in only one case.
Suppose that I know that my container will be filled with 10 numbers, so before starting pushing into the vector I resize to the mentioned size.
You are doing something that will be done better and automatically (no need to write code like this).
Next
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
I know that works, but I would go with simply
auto dbval = database.size();
auto entryval = database[0].size();
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
Reformat code
Try to use IDE options where you can reformat code, right now it is hard to read. There is everything in one place.
Create small functions
Right now, your code is a one big function. Firstly, divide your code into small functions that are doing one thing. You could end up with 10 or 15 really small functions. After that, thing about functions that are similar to each other, they are part of one bigger responsibility. You might be able to see some "objects" and create appropriate classes.
Hardcoding values
You have code like this
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
This can be done for example using command line arguments. You will be passing path to file when you start running your program, that will be much more flexible.
Next examples of hardcoding values
std::cout << database[sealChoice][14] << std::endl;
while (switchChoice <= 0 || switchChoice >= 4 || std::cin.fail())
Try to give these every number meaningful name, it will much more easier for readers to understand.
Strange code
I cannot get why do you do this
size_t dbsize = database.size();
database.resize(dbsize + 1);
Personally, I use resize in only one case.
Suppose that I know that my container will be filled with 10 numbers, so before starting pushing into the vector I resize to the mentioned size.
You are doing something that will be done better and automatically (no need to write code like this).
Next
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
I know that works, but I would go with simply
auto dbval = database.size();
auto entryval = database[0].size();
add a comment |Â
up vote
5
down vote
Reformat code
Try to use IDE options where you can reformat code, right now it is hard to read. There is everything in one place.
Create small functions
Right now, your code is a one big function. Firstly, divide your code into small functions that are doing one thing. You could end up with 10 or 15 really small functions. After that, thing about functions that are similar to each other, they are part of one bigger responsibility. You might be able to see some "objects" and create appropriate classes.
Hardcoding values
You have code like this
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
This can be done for example using command line arguments. You will be passing path to file when you start running your program, that will be much more flexible.
Next examples of hardcoding values
std::cout << database[sealChoice][14] << std::endl;
while (switchChoice <= 0 || switchChoice >= 4 || std::cin.fail())
Try to give these every number meaningful name, it will much more easier for readers to understand.
Strange code
I cannot get why do you do this
size_t dbsize = database.size();
database.resize(dbsize + 1);
Personally, I use resize in only one case.
Suppose that I know that my container will be filled with 10 numbers, so before starting pushing into the vector I resize to the mentioned size.
You are doing something that will be done better and automatically (no need to write code like this).
Next
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
I know that works, but I would go with simply
auto dbval = database.size();
auto entryval = database[0].size();
add a comment |Â
up vote
5
down vote
up vote
5
down vote
Reformat code
Try to use IDE options where you can reformat code, right now it is hard to read. There is everything in one place.
Create small functions
Right now, your code is a one big function. Firstly, divide your code into small functions that are doing one thing. You could end up with 10 or 15 really small functions. After that, thing about functions that are similar to each other, they are part of one bigger responsibility. You might be able to see some "objects" and create appropriate classes.
Hardcoding values
You have code like this
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
This can be done for example using command line arguments. You will be passing path to file when you start running your program, that will be much more flexible.
Next examples of hardcoding values
std::cout << database[sealChoice][14] << std::endl;
while (switchChoice <= 0 || switchChoice >= 4 || std::cin.fail())
Try to give these every number meaningful name, it will much more easier for readers to understand.
Strange code
I cannot get why do you do this
size_t dbsize = database.size();
database.resize(dbsize + 1);
Personally, I use resize in only one case.
Suppose that I know that my container will be filled with 10 numbers, so before starting pushing into the vector I resize to the mentioned size.
You are doing something that will be done better and automatically (no need to write code like this).
Next
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
I know that works, but I would go with simply
auto dbval = database.size();
auto entryval = database[0].size();
Reformat code
Try to use IDE options where you can reformat code, right now it is hard to read. There is everything in one place.
Create small functions
Right now, your code is a one big function. Firstly, divide your code into small functions that are doing one thing. You could end up with 10 or 15 really small functions. After that, thing about functions that are similar to each other, they are part of one bigger responsibility. You might be able to see some "objects" and create appropriate classes.
Hardcoding values
You have code like this
std::ifstream file("C:\Users\MyPC\Desktop\Programs\Seals.csv");
This can be done for example using command line arguments. You will be passing path to file when you start running your program, that will be much more flexible.
Next examples of hardcoding values
std::cout << database[sealChoice][14] << std::endl;
while (switchChoice <= 0 || switchChoice >= 4 || std::cin.fail())
Try to give these every number meaningful name, it will much more easier for readers to understand.
Strange code
I cannot get why do you do this
size_t dbsize = database.size();
database.resize(dbsize + 1);
Personally, I use resize in only one case.
Suppose that I know that my container will be filled with 10 numbers, so before starting pushing into the vector I resize to the mentioned size.
You are doing something that will be done better and automatically (no need to write code like this).
Next
int dbval = static_cast<int>(database.size());
int entryval = static_cast<int>(database[0].size());
I know that works, but I would go with simply
auto dbval = database.size();
auto entryval = database[0].size();
answered Aug 4 at 8:28
Newbie
478314
478314
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f184359%2ftext-menu-to-browse-csv-data-about-seal-species%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password