PHP Iterate over Child's Child Efficiently
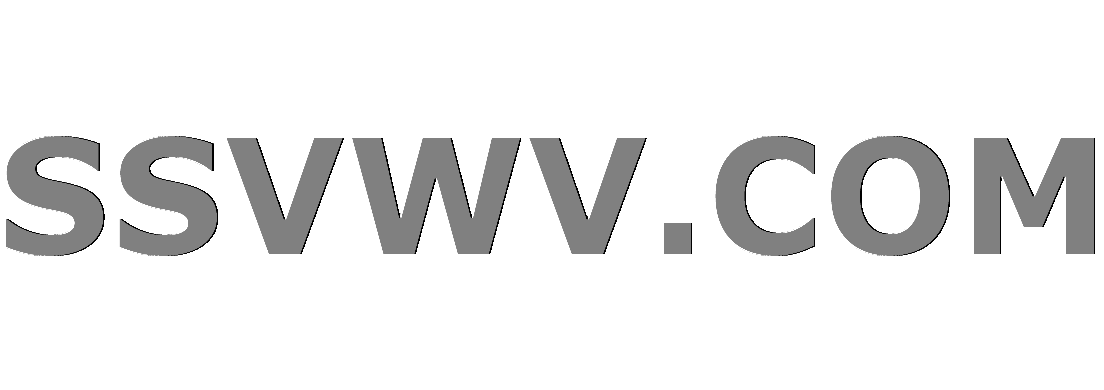
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I have this code:
foreach($list as $id => $item_array) $is_admin)
include('item.php');
$temp = $item_array;
while(!empty($temp['child']))
foreach($temp['child'] as $id => $item_array)
if(empty($temp['child']['child']))
unset($temp);
which functions but I'm going to need to duplicate the while
block 5 times because my item
s can have up to six levels. There must be a more efficient way to do this, right? (The item.php
just outputs HTML based on the array values)
In the simplest form $list
would be:
Array
(
[id] => 1
[name] => Water
[date] => 2018-05-14 13:24:22
[content] => It's Good
[approved] => 1
[level] => 0
[child] => Array([0] => Array(
[id] => 2
[name] => Hydrogen
[date] => 2017-05-07 15:12:14
[content] => Part air
[approved] => 1
[level] => 1)
)
)
If needed I build $list
with:
if($parent != -1)
$list[$parent]['child'] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
else
$list[$id] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
function build_comment_array($id, $date, $content, $name, $approved, $level)
return array('id' => $id,
'name' => $name,
'date' => $date,
'content' => $content,
'approved' => $approved,
'level' => $level);
php recursion
add a comment |Â
up vote
1
down vote
favorite
I have this code:
foreach($list as $id => $item_array) $is_admin)
include('item.php');
$temp = $item_array;
while(!empty($temp['child']))
foreach($temp['child'] as $id => $item_array)
if(empty($temp['child']['child']))
unset($temp);
which functions but I'm going to need to duplicate the while
block 5 times because my item
s can have up to six levels. There must be a more efficient way to do this, right? (The item.php
just outputs HTML based on the array values)
In the simplest form $list
would be:
Array
(
[id] => 1
[name] => Water
[date] => 2018-05-14 13:24:22
[content] => It's Good
[approved] => 1
[level] => 0
[child] => Array([0] => Array(
[id] => 2
[name] => Hydrogen
[date] => 2017-05-07 15:12:14
[content] => Part air
[approved] => 1
[level] => 1)
)
)
If needed I build $list
with:
if($parent != -1)
$list[$parent]['child'] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
else
$list[$id] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
function build_comment_array($id, $date, $content, $name, $approved, $level)
return array('id' => $id,
'name' => $name,
'date' => $date,
'content' => $content,
'approved' => $approved,
'level' => $level);
php recursion
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have this code:
foreach($list as $id => $item_array) $is_admin)
include('item.php');
$temp = $item_array;
while(!empty($temp['child']))
foreach($temp['child'] as $id => $item_array)
if(empty($temp['child']['child']))
unset($temp);
which functions but I'm going to need to duplicate the while
block 5 times because my item
s can have up to six levels. There must be a more efficient way to do this, right? (The item.php
just outputs HTML based on the array values)
In the simplest form $list
would be:
Array
(
[id] => 1
[name] => Water
[date] => 2018-05-14 13:24:22
[content] => It's Good
[approved] => 1
[level] => 0
[child] => Array([0] => Array(
[id] => 2
[name] => Hydrogen
[date] => 2017-05-07 15:12:14
[content] => Part air
[approved] => 1
[level] => 1)
)
)
If needed I build $list
with:
if($parent != -1)
$list[$parent]['child'] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
else
$list[$id] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
function build_comment_array($id, $date, $content, $name, $approved, $level)
return array('id' => $id,
'name' => $name,
'date' => $date,
'content' => $content,
'approved' => $approved,
'level' => $level);
php recursion
I have this code:
foreach($list as $id => $item_array) $is_admin)
include('item.php');
$temp = $item_array;
while(!empty($temp['child']))
foreach($temp['child'] as $id => $item_array)
if(empty($temp['child']['child']))
unset($temp);
which functions but I'm going to need to duplicate the while
block 5 times because my item
s can have up to six levels. There must be a more efficient way to do this, right? (The item.php
just outputs HTML based on the array values)
In the simplest form $list
would be:
Array
(
[id] => 1
[name] => Water
[date] => 2018-05-14 13:24:22
[content] => It's Good
[approved] => 1
[level] => 0
[child] => Array([0] => Array(
[id] => 2
[name] => Hydrogen
[date] => 2017-05-07 15:12:14
[content] => Part air
[approved] => 1
[level] => 1)
)
)
If needed I build $list
with:
if($parent != -1)
$list[$parent]['child'] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
else
$list[$id] = $this->build_list_array($id, $date, $content, $name, $approved, $level);
function build_comment_array($id, $date, $content, $name, $approved, $level)
return array('id' => $id,
'name' => $name,
'date' => $date,
'content' => $content,
'approved' => $approved,
'level' => $level);
php recursion
asked May 14 at 20:22
user3783243
1083
1083
add a comment |Â
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
4
down vote
accepted
You can do it recursively. Create a function (let's say, iterateChildren(array)
) that iterates over the given array and when the children key is found you call iterateChildren(item['children'])
.
This way you can have as many levels as you'd like, since the function calls itself at each level.
Be cautious, though, because with too many levels you may run out of memory.
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
You can do it recursively. Create a function (let's say, iterateChildren(array)
) that iterates over the given array and when the children key is found you call iterateChildren(item['children'])
.
This way you can have as many levels as you'd like, since the function calls itself at each level.
Be cautious, though, because with too many levels you may run out of memory.
add a comment |Â
up vote
4
down vote
accepted
You can do it recursively. Create a function (let's say, iterateChildren(array)
) that iterates over the given array and when the children key is found you call iterateChildren(item['children'])
.
This way you can have as many levels as you'd like, since the function calls itself at each level.
Be cautious, though, because with too many levels you may run out of memory.
add a comment |Â
up vote
4
down vote
accepted
up vote
4
down vote
accepted
You can do it recursively. Create a function (let's say, iterateChildren(array)
) that iterates over the given array and when the children key is found you call iterateChildren(item['children'])
.
This way you can have as many levels as you'd like, since the function calls itself at each level.
Be cautious, though, because with too many levels you may run out of memory.
You can do it recursively. Create a function (let's say, iterateChildren(array)
) that iterates over the given array and when the children key is found you call iterateChildren(item['children'])
.
This way you can have as many levels as you'd like, since the function calls itself at each level.
Be cautious, though, because with too many levels you may run out of memory.
edited May 14 at 22:51


Sam Onela
5,76961543
5,76961543
answered May 14 at 22:38


Stefan Manciu
1562
1562
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f194394%2fphp-iterate-over-childs-child-efficiently%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password