Implement a queue class in Python
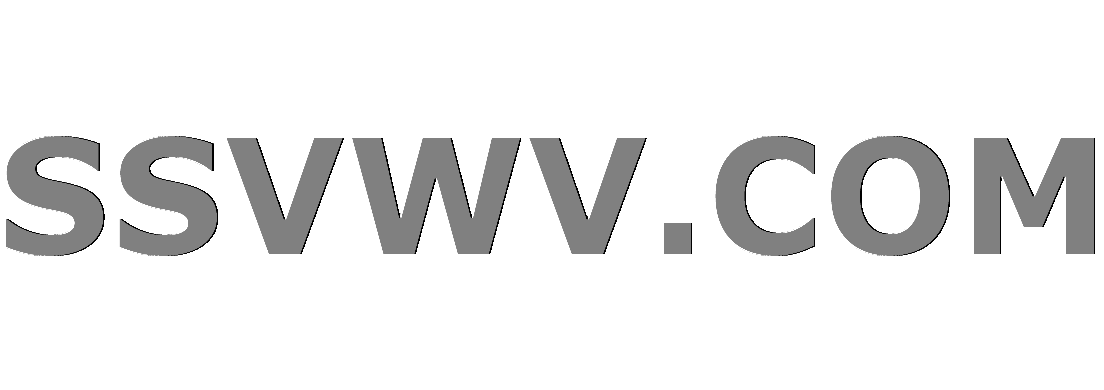
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
Problem:Implement a queue class in Python: It should support 3 APIs:
queue.top(): prints current element at front of queue
queue.pop(): takes out an element from front of queue
queue.add(): adds a new element at end of queue
class Queue:
def __init__(self):
"""initialise a Queue class"""
self.items =
self.index = len(self.items) - 1
def top(self):
"""returns the current element at front of queue"""
if self.items:
return self.items[0]
else:
raise Exception("Empty Queue")
def pop(self):
"""takes out an element from front of queue"""
if self.items:
self.items.pop(0)
else :
raise Exception("Empty Queue")
def add(self , item):
"""adds a new element at the end of queue"""
self.items.append(item)
def __str__(self):
"""returns the string representation"""
return str(self.items)
def __iter__(self):
"""iterates over the sequence"""
return self
def __next__(self):
"""returns the next value in sequence"""
if self.index == 0 :
raise StopIteration
self.index -= 1
return self.items[self.index]
queue_1 = Queue()
queue_1.add(12)
queue_1.add(11)
queue_1.add(55)
queue_1.add(66)
queue_1.add(56)
queue_1.add(43)
queue_1.add(33)
queue_1.add(88)
queue_1.add(56)
queue_1.add(34)
iter(queue_1)
for i in queue_1:
print i
print queue_1
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
The code works as intended and executes the 3 APIs specified in the problem . But it gives ""TypeError: instance has no next() method"" when i try to use next method to iterate over the values in queue. What am i missing in defining the next method?
python queue
add a comment |Â
up vote
1
down vote
favorite
Problem:Implement a queue class in Python: It should support 3 APIs:
queue.top(): prints current element at front of queue
queue.pop(): takes out an element from front of queue
queue.add(): adds a new element at end of queue
class Queue:
def __init__(self):
"""initialise a Queue class"""
self.items =
self.index = len(self.items) - 1
def top(self):
"""returns the current element at front of queue"""
if self.items:
return self.items[0]
else:
raise Exception("Empty Queue")
def pop(self):
"""takes out an element from front of queue"""
if self.items:
self.items.pop(0)
else :
raise Exception("Empty Queue")
def add(self , item):
"""adds a new element at the end of queue"""
self.items.append(item)
def __str__(self):
"""returns the string representation"""
return str(self.items)
def __iter__(self):
"""iterates over the sequence"""
return self
def __next__(self):
"""returns the next value in sequence"""
if self.index == 0 :
raise StopIteration
self.index -= 1
return self.items[self.index]
queue_1 = Queue()
queue_1.add(12)
queue_1.add(11)
queue_1.add(55)
queue_1.add(66)
queue_1.add(56)
queue_1.add(43)
queue_1.add(33)
queue_1.add(88)
queue_1.add(56)
queue_1.add(34)
iter(queue_1)
for i in queue_1:
print i
print queue_1
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
The code works as intended and executes the 3 APIs specified in the problem . But it gives ""TypeError: instance has no next() method"" when i try to use next method to iterate over the values in queue. What am i missing in defining the next method?
python queue
1
Python has a built-in queue class,collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.
– Gareth Rees
Jan 24 at 19:15
1
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Problem:Implement a queue class in Python: It should support 3 APIs:
queue.top(): prints current element at front of queue
queue.pop(): takes out an element from front of queue
queue.add(): adds a new element at end of queue
class Queue:
def __init__(self):
"""initialise a Queue class"""
self.items =
self.index = len(self.items) - 1
def top(self):
"""returns the current element at front of queue"""
if self.items:
return self.items[0]
else:
raise Exception("Empty Queue")
def pop(self):
"""takes out an element from front of queue"""
if self.items:
self.items.pop(0)
else :
raise Exception("Empty Queue")
def add(self , item):
"""adds a new element at the end of queue"""
self.items.append(item)
def __str__(self):
"""returns the string representation"""
return str(self.items)
def __iter__(self):
"""iterates over the sequence"""
return self
def __next__(self):
"""returns the next value in sequence"""
if self.index == 0 :
raise StopIteration
self.index -= 1
return self.items[self.index]
queue_1 = Queue()
queue_1.add(12)
queue_1.add(11)
queue_1.add(55)
queue_1.add(66)
queue_1.add(56)
queue_1.add(43)
queue_1.add(33)
queue_1.add(88)
queue_1.add(56)
queue_1.add(34)
iter(queue_1)
for i in queue_1:
print i
print queue_1
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
The code works as intended and executes the 3 APIs specified in the problem . But it gives ""TypeError: instance has no next() method"" when i try to use next method to iterate over the values in queue. What am i missing in defining the next method?
python queue
Problem:Implement a queue class in Python: It should support 3 APIs:
queue.top(): prints current element at front of queue
queue.pop(): takes out an element from front of queue
queue.add(): adds a new element at end of queue
class Queue:
def __init__(self):
"""initialise a Queue class"""
self.items =
self.index = len(self.items) - 1
def top(self):
"""returns the current element at front of queue"""
if self.items:
return self.items[0]
else:
raise Exception("Empty Queue")
def pop(self):
"""takes out an element from front of queue"""
if self.items:
self.items.pop(0)
else :
raise Exception("Empty Queue")
def add(self , item):
"""adds a new element at the end of queue"""
self.items.append(item)
def __str__(self):
"""returns the string representation"""
return str(self.items)
def __iter__(self):
"""iterates over the sequence"""
return self
def __next__(self):
"""returns the next value in sequence"""
if self.index == 0 :
raise StopIteration
self.index -= 1
return self.items[self.index]
queue_1 = Queue()
queue_1.add(12)
queue_1.add(11)
queue_1.add(55)
queue_1.add(66)
queue_1.add(56)
queue_1.add(43)
queue_1.add(33)
queue_1.add(88)
queue_1.add(56)
queue_1.add(34)
iter(queue_1)
for i in queue_1:
print i
print queue_1
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
print queue_1.top()
queue_1.pop()
The code works as intended and executes the 3 APIs specified in the problem . But it gives ""TypeError: instance has no next() method"" when i try to use next method to iterate over the values in queue. What am i missing in defining the next method?
python queue
asked Jan 24 at 19:02
Latika Agarwal
861216
861216
1
Python has a built-in queue class,collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.
– Gareth Rees
Jan 24 at 19:15
1
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42
add a comment |Â
1
Python has a built-in queue class,collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.
– Gareth Rees
Jan 24 at 19:15
1
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42
1
1
Python has a built-in queue class,
collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.– Gareth Rees
Jan 24 at 19:15
Python has a built-in queue class,
collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.– Gareth Rees
Jan 24 at 19:15
1
1
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
I would have just inherited from the built-in collections.deque
:
from collections import deque
class Queue(deque):
def add(self, value):
self.append(value)
def top(self):
return self[0]
def pop(self):
return self.popleft()
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I would have just inherited from the built-in collections.deque
:
from collections import deque
class Queue(deque):
def add(self, value):
self.append(value)
def top(self):
return self[0]
def pop(self):
return self.popleft()
add a comment |Â
up vote
0
down vote
accepted
I would have just inherited from the built-in collections.deque
:
from collections import deque
class Queue(deque):
def add(self, value):
self.append(value)
def top(self):
return self[0]
def pop(self):
return self.popleft()
add a comment |Â
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I would have just inherited from the built-in collections.deque
:
from collections import deque
class Queue(deque):
def add(self, value):
self.append(value)
def top(self):
return self[0]
def pop(self):
return self.popleft()
I would have just inherited from the built-in collections.deque
:
from collections import deque
class Queue(deque):
def add(self, value):
self.append(value)
def top(self):
return self[0]
def pop(self):
return self.popleft()
answered Jan 24 at 19:39
Graipher
20.5k43081
20.5k43081
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f185896%2fimplement-a-queue-class-in-python%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
1
Python has a built-in queue class,
collections.deque
. The implementation is in C rather than Python, but still worth a look. If you prefer a quick-and-simple implementation, then there's a standard approach using two stacks (stacks = lists in Python) which at least has the right complexity.– Gareth Rees
Jan 24 at 19:15
1
Questions about Errors belong on stackoverflow
– Ludisposed
Jan 24 at 19:42