Multiplication of any two matrices using lists and for in loop
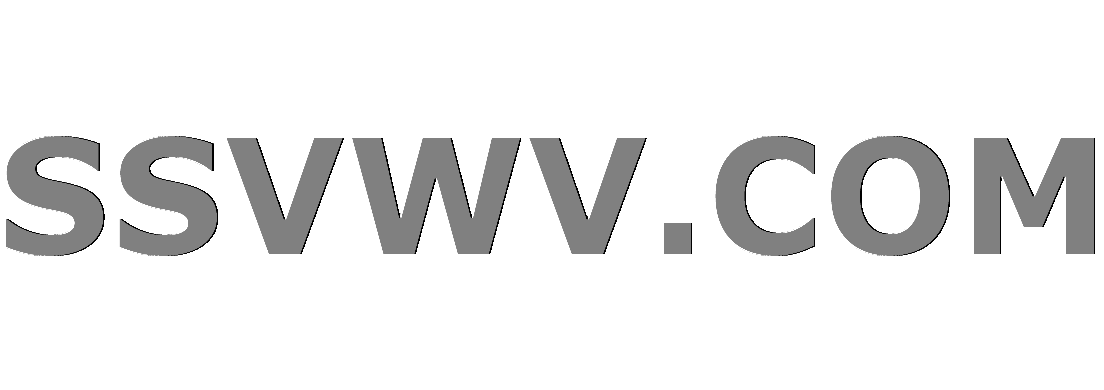
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
3
down vote
favorite
I've just started learning Python and after few lessons I grasped the idea of lists and loop and decided to create my first project.
Objective: Calculate multiplication of any two matrices from user's input.
The code is working as intended but as this is my first one, I would be happy for some hints as far what is written well and what is e.g. hard to read by someone, wrongly used method or unnecessary complication.
I specifically wanted to stay within basic knowledge and not use for example NumPy for learning reason. There will be time for libraries in future.
Thanks all in advance and my code is below:
# This program multiplicates two matrices of any number of rows and columns.
# The matrix elements are filled by User
# The result is calculated and saved as list
print('MATRIX MULTIPLICATIONn')
# Ask user for dimensions of first matrix
r = int(input("Matrix1: Number of rows?: "))
c = int(input("Matrix1: Number of columns?: "))
# Print design of first Matrix for better understanding of elements' naming convention
print('''nElements of matrix1:''')
element = '' # Later will be used to construct each row of the matrix
for vr in range(0, r): # vr - variable row
for vc in range(0, c): # vc - variable column
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create empty Matrix1 and fill it with user input element by element
Matrix1 = [ for vr in range(0, r)]
for vr in range(0, r):
Matrix1[vr] = [0 for vc in range(0, c)]
for vr in range(0, r):
for vc in range(0, c):
Matrix1[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': ')) # + 1 because vr and vc starts at 0 and we want elements be named from 1
# Ask the user for dimension of second matrix
v = int(input("Matrix2: Number of columns?: ")) # Only for columns since M2 rows = M1 columns by definition
print('''nElements of matrix Matrix2:''')
element = ''
for vr in range(0, c): # vr in c because Matrix 2 must have the same number of rows as Matrix1 columns.
for vc in range(0, v):
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create Empty Matrix2 and fill it with user input element by element
Matrix2 = [ for vr in range(0, c)]
for vr in range(0, c):
Matrix2[vr] = [0 for vc in range(0, v)]
for vr in range(0, c):
for vc in range(0, v):
Matrix2[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': '))
# Create empty Product Matrix for Result of multiplication of Matrix1 and Matrix2
Product = [ for vr in range(0, r)] # Number of rows defined by number of rows in Matrix1
for vr in range(0, r):
Product[vr] = [0 for columns in range(v)] # Number of columns defined by number of columns in Matrix2
# Calculate the Product of two tables
Products = 0 # dummy variable for calculating sum of product of each row and column
for vv in range(0, v):
for vr in range(0, r):
for vc in range(0, c):
Products += Matrix1[vr][vc] * Matrix2[vc][vv]
Product[vr][vv] = Products
Products = 0 # clear the variable when looped for whole column of Matrix2
print('Matrix1: ', Matrix1)
print('Matrix2: ', Matrix2)
print('Result of Matrix Multiplication:', Product)
# Footnotes
print('nnCreated by: MK')
input('Press "Enter" to Exit') # Easy way to Exit program If run from script
python python-3.x matrix
add a comment |Â
up vote
3
down vote
favorite
I've just started learning Python and after few lessons I grasped the idea of lists and loop and decided to create my first project.
Objective: Calculate multiplication of any two matrices from user's input.
The code is working as intended but as this is my first one, I would be happy for some hints as far what is written well and what is e.g. hard to read by someone, wrongly used method or unnecessary complication.
I specifically wanted to stay within basic knowledge and not use for example NumPy for learning reason. There will be time for libraries in future.
Thanks all in advance and my code is below:
# This program multiplicates two matrices of any number of rows and columns.
# The matrix elements are filled by User
# The result is calculated and saved as list
print('MATRIX MULTIPLICATIONn')
# Ask user for dimensions of first matrix
r = int(input("Matrix1: Number of rows?: "))
c = int(input("Matrix1: Number of columns?: "))
# Print design of first Matrix for better understanding of elements' naming convention
print('''nElements of matrix1:''')
element = '' # Later will be used to construct each row of the matrix
for vr in range(0, r): # vr - variable row
for vc in range(0, c): # vc - variable column
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create empty Matrix1 and fill it with user input element by element
Matrix1 = [ for vr in range(0, r)]
for vr in range(0, r):
Matrix1[vr] = [0 for vc in range(0, c)]
for vr in range(0, r):
for vc in range(0, c):
Matrix1[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': ')) # + 1 because vr and vc starts at 0 and we want elements be named from 1
# Ask the user for dimension of second matrix
v = int(input("Matrix2: Number of columns?: ")) # Only for columns since M2 rows = M1 columns by definition
print('''nElements of matrix Matrix2:''')
element = ''
for vr in range(0, c): # vr in c because Matrix 2 must have the same number of rows as Matrix1 columns.
for vc in range(0, v):
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create Empty Matrix2 and fill it with user input element by element
Matrix2 = [ for vr in range(0, c)]
for vr in range(0, c):
Matrix2[vr] = [0 for vc in range(0, v)]
for vr in range(0, c):
for vc in range(0, v):
Matrix2[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': '))
# Create empty Product Matrix for Result of multiplication of Matrix1 and Matrix2
Product = [ for vr in range(0, r)] # Number of rows defined by number of rows in Matrix1
for vr in range(0, r):
Product[vr] = [0 for columns in range(v)] # Number of columns defined by number of columns in Matrix2
# Calculate the Product of two tables
Products = 0 # dummy variable for calculating sum of product of each row and column
for vv in range(0, v):
for vr in range(0, r):
for vc in range(0, c):
Products += Matrix1[vr][vc] * Matrix2[vc][vv]
Product[vr][vv] = Products
Products = 0 # clear the variable when looped for whole column of Matrix2
print('Matrix1: ', Matrix1)
print('Matrix2: ', Matrix2)
print('Result of Matrix Multiplication:', Product)
# Footnotes
print('nnCreated by: MK')
input('Press "Enter" to Exit') # Easy way to Exit program If run from script
python python-3.x matrix
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06
add a comment |Â
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I've just started learning Python and after few lessons I grasped the idea of lists and loop and decided to create my first project.
Objective: Calculate multiplication of any two matrices from user's input.
The code is working as intended but as this is my first one, I would be happy for some hints as far what is written well and what is e.g. hard to read by someone, wrongly used method or unnecessary complication.
I specifically wanted to stay within basic knowledge and not use for example NumPy for learning reason. There will be time for libraries in future.
Thanks all in advance and my code is below:
# This program multiplicates two matrices of any number of rows and columns.
# The matrix elements are filled by User
# The result is calculated and saved as list
print('MATRIX MULTIPLICATIONn')
# Ask user for dimensions of first matrix
r = int(input("Matrix1: Number of rows?: "))
c = int(input("Matrix1: Number of columns?: "))
# Print design of first Matrix for better understanding of elements' naming convention
print('''nElements of matrix1:''')
element = '' # Later will be used to construct each row of the matrix
for vr in range(0, r): # vr - variable row
for vc in range(0, c): # vc - variable column
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create empty Matrix1 and fill it with user input element by element
Matrix1 = [ for vr in range(0, r)]
for vr in range(0, r):
Matrix1[vr] = [0 for vc in range(0, c)]
for vr in range(0, r):
for vc in range(0, c):
Matrix1[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': ')) # + 1 because vr and vc starts at 0 and we want elements be named from 1
# Ask the user for dimension of second matrix
v = int(input("Matrix2: Number of columns?: ")) # Only for columns since M2 rows = M1 columns by definition
print('''nElements of matrix Matrix2:''')
element = ''
for vr in range(0, c): # vr in c because Matrix 2 must have the same number of rows as Matrix1 columns.
for vc in range(0, v):
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create Empty Matrix2 and fill it with user input element by element
Matrix2 = [ for vr in range(0, c)]
for vr in range(0, c):
Matrix2[vr] = [0 for vc in range(0, v)]
for vr in range(0, c):
for vc in range(0, v):
Matrix2[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': '))
# Create empty Product Matrix for Result of multiplication of Matrix1 and Matrix2
Product = [ for vr in range(0, r)] # Number of rows defined by number of rows in Matrix1
for vr in range(0, r):
Product[vr] = [0 for columns in range(v)] # Number of columns defined by number of columns in Matrix2
# Calculate the Product of two tables
Products = 0 # dummy variable for calculating sum of product of each row and column
for vv in range(0, v):
for vr in range(0, r):
for vc in range(0, c):
Products += Matrix1[vr][vc] * Matrix2[vc][vv]
Product[vr][vv] = Products
Products = 0 # clear the variable when looped for whole column of Matrix2
print('Matrix1: ', Matrix1)
print('Matrix2: ', Matrix2)
print('Result of Matrix Multiplication:', Product)
# Footnotes
print('nnCreated by: MK')
input('Press "Enter" to Exit') # Easy way to Exit program If run from script
python python-3.x matrix
I've just started learning Python and after few lessons I grasped the idea of lists and loop and decided to create my first project.
Objective: Calculate multiplication of any two matrices from user's input.
The code is working as intended but as this is my first one, I would be happy for some hints as far what is written well and what is e.g. hard to read by someone, wrongly used method or unnecessary complication.
I specifically wanted to stay within basic knowledge and not use for example NumPy for learning reason. There will be time for libraries in future.
Thanks all in advance and my code is below:
# This program multiplicates two matrices of any number of rows and columns.
# The matrix elements are filled by User
# The result is calculated and saved as list
print('MATRIX MULTIPLICATIONn')
# Ask user for dimensions of first matrix
r = int(input("Matrix1: Number of rows?: "))
c = int(input("Matrix1: Number of columns?: "))
# Print design of first Matrix for better understanding of elements' naming convention
print('''nElements of matrix1:''')
element = '' # Later will be used to construct each row of the matrix
for vr in range(0, r): # vr - variable row
for vc in range(0, c): # vc - variable column
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create empty Matrix1 and fill it with user input element by element
Matrix1 = [ for vr in range(0, r)]
for vr in range(0, r):
Matrix1[vr] = [0 for vc in range(0, c)]
for vr in range(0, r):
for vc in range(0, c):
Matrix1[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': ')) # + 1 because vr and vc starts at 0 and we want elements be named from 1
# Ask the user for dimension of second matrix
v = int(input("Matrix2: Number of columns?: ")) # Only for columns since M2 rows = M1 columns by definition
print('''nElements of matrix Matrix2:''')
element = ''
for vr in range(0, c): # vr in c because Matrix 2 must have the same number of rows as Matrix1 columns.
for vc in range(0, v):
element += 'M' + str(vr + 1) + str(vc + 1) + ' '
print('|', element, 'b|')
element = ''
# Create Empty Matrix2 and fill it with user input element by element
Matrix2 = [ for vr in range(0, c)]
for vr in range(0, c):
Matrix2[vr] = [0 for vc in range(0, v)]
for vr in range(0, c):
for vc in range(0, v):
Matrix2[vr][vc] = int(input('M' + str(vr + 1) + str(vc + 1) + ': '))
# Create empty Product Matrix for Result of multiplication of Matrix1 and Matrix2
Product = [ for vr in range(0, r)] # Number of rows defined by number of rows in Matrix1
for vr in range(0, r):
Product[vr] = [0 for columns in range(v)] # Number of columns defined by number of columns in Matrix2
# Calculate the Product of two tables
Products = 0 # dummy variable for calculating sum of product of each row and column
for vv in range(0, v):
for vr in range(0, r):
for vc in range(0, c):
Products += Matrix1[vr][vc] * Matrix2[vc][vv]
Product[vr][vv] = Products
Products = 0 # clear the variable when looped for whole column of Matrix2
print('Matrix1: ', Matrix1)
print('Matrix2: ', Matrix2)
print('Result of Matrix Multiplication:', Product)
# Footnotes
print('nnCreated by: MK')
input('Press "Enter" to Exit') # Easy way to Exit program If run from script
python python-3.x matrix
asked Jan 24 at 22:55


Mateusz Konopelski
654
654
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06
add a comment |Â
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06
add a comment |Â
3 Answers
3
active
oldest
votes
up vote
3
down vote
accepted
I have basically two comments:
- Naming.
- Use functions to follow the single responsibility principle.
Naming
Python has an official style-guide, PEP8. Some of its recommendations are:
- Using
lower_case
for variables and functions,PascalCase
for classes andALL_CAPS
for global constants. - To limit your line length to 80 (or at the most 120) characters
Your names which you use to iterate through the rows and columns are not clear at all. Why vc
and vr
(I guess the c and r stand for column and row, but what does the v stand for?)? Instead I would use rows
and columns
for the number of rows and columns and use i
and j
to iterate over them, because that is the convention used in mathematics when describing matrices.
Single responsibility principle
As for your actual code, you basically have four major responsibilities:
- Asking the user for input
- Creating a matrix
- Calculating the matrix product
- Printing a matrix scheme
I would make this into four functions:
def ask_user(message):
while True:
try:
return int(input(message))
except TypeError:
pass
This function will ask the user the message
until he inputs something that can be cast to an int
.
def matrix_from_user():
rows = ask_user("Number of rows?: ")
columns = ask_user("Number of columns?: ")
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = ask_user("M: ".format(i+1, j+1))
return matrix
Here I used str.format
to make the construction of the string easier.
def matrix_mul(matrix1, matrix2):
rows, columns = len(matrix1), len(matrix2[0])
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = sum(matrix1[i][k] * matrix2[k][j]
for k in range(len(matrix2)))
return matrix
This is a straightforward implementation of the formula $(AB)_ij = sum_k=1^m A_ik B_kj$ for two matrices $A$ and $B$. It uses the built-in sum
function that takes an iterable to sum. This iterable is a generator comprehension here.
def matrix_print(matrix):
print('nElements of matrix:')
rows, columns = len(matrix), len(matrix[0])
for i in range(rows):
row = ' '.join('M'.format(i + 1, j + 1) for j in range(columns))
print('|', row, '|')
Here I used str.join
to easily join an iterable of strings with a given delimiter (' '
here).
Your main program becomes then simply:
if __name__ == "__main__":
matrix1 = matrix_from_user()
matrix_print(matrix1)
matrix2 = matrix_from_user()
product = matrix_mul(matrix1, matrix2)
print('Matrix1: ', matrix1)
print('Matrix2: ', matrix2)
print('Result of Matrix Multiplication:', product)
This code I put under an if __name__ == "__main__":
guard which allows you to import this script from another script without this part of the code being run (so you get access to the functions).
add a comment |Â
up vote
2
down vote
For learning reason, you can create 2d array in one row like:
matrix2 = [[vc + v*10*vr for vc in range(0, v)] for vr in range(0, r)]
Since python is a funcational language, for learning reason, you can define a map/ reduce over matrix like:
def map(matrix, r,c, fn,summ):
for vr in range(0, r):
for vc in range(0, c):
fn(matrix[r][c],summ)
or with additional map-reduce over the row:
def map(matrix, r,c, fn,fnRow,startVal,summ):
for vr in range(0, r):
summRow = startVal
for vc in range(0, c):
fn(matrix[r][c],summRow)
fnRow(summRow, summ)
for learning reason you could implement Strassen matrix multiplication algorithm (google please), not naive one. I doubt you learn something like that.
For doing real project, use Numpy for initiations, not for high-performance operations.
add a comment |Â
up vote
1
down vote
I suggest following matrix_mul
implementation
def matrix_mul(matrix1, matrix2):
from operator import mul
return [[sum(map(mul, row, col)) for col in zip(*matrix2)] for row in matrix1]
That is a nice and compact way of writing it. But I would put theimport
outside the function, it does cause some small overhead (even though it will only be imported once).
– Graipher
Jan 25 at 9:44
add a comment |Â
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
I have basically two comments:
- Naming.
- Use functions to follow the single responsibility principle.
Naming
Python has an official style-guide, PEP8. Some of its recommendations are:
- Using
lower_case
for variables and functions,PascalCase
for classes andALL_CAPS
for global constants. - To limit your line length to 80 (or at the most 120) characters
Your names which you use to iterate through the rows and columns are not clear at all. Why vc
and vr
(I guess the c and r stand for column and row, but what does the v stand for?)? Instead I would use rows
and columns
for the number of rows and columns and use i
and j
to iterate over them, because that is the convention used in mathematics when describing matrices.
Single responsibility principle
As for your actual code, you basically have four major responsibilities:
- Asking the user for input
- Creating a matrix
- Calculating the matrix product
- Printing a matrix scheme
I would make this into four functions:
def ask_user(message):
while True:
try:
return int(input(message))
except TypeError:
pass
This function will ask the user the message
until he inputs something that can be cast to an int
.
def matrix_from_user():
rows = ask_user("Number of rows?: ")
columns = ask_user("Number of columns?: ")
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = ask_user("M: ".format(i+1, j+1))
return matrix
Here I used str.format
to make the construction of the string easier.
def matrix_mul(matrix1, matrix2):
rows, columns = len(matrix1), len(matrix2[0])
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = sum(matrix1[i][k] * matrix2[k][j]
for k in range(len(matrix2)))
return matrix
This is a straightforward implementation of the formula $(AB)_ij = sum_k=1^m A_ik B_kj$ for two matrices $A$ and $B$. It uses the built-in sum
function that takes an iterable to sum. This iterable is a generator comprehension here.
def matrix_print(matrix):
print('nElements of matrix:')
rows, columns = len(matrix), len(matrix[0])
for i in range(rows):
row = ' '.join('M'.format(i + 1, j + 1) for j in range(columns))
print('|', row, '|')
Here I used str.join
to easily join an iterable of strings with a given delimiter (' '
here).
Your main program becomes then simply:
if __name__ == "__main__":
matrix1 = matrix_from_user()
matrix_print(matrix1)
matrix2 = matrix_from_user()
product = matrix_mul(matrix1, matrix2)
print('Matrix1: ', matrix1)
print('Matrix2: ', matrix2)
print('Result of Matrix Multiplication:', product)
This code I put under an if __name__ == "__main__":
guard which allows you to import this script from another script without this part of the code being run (so you get access to the functions).
add a comment |Â
up vote
3
down vote
accepted
I have basically two comments:
- Naming.
- Use functions to follow the single responsibility principle.
Naming
Python has an official style-guide, PEP8. Some of its recommendations are:
- Using
lower_case
for variables and functions,PascalCase
for classes andALL_CAPS
for global constants. - To limit your line length to 80 (or at the most 120) characters
Your names which you use to iterate through the rows and columns are not clear at all. Why vc
and vr
(I guess the c and r stand for column and row, but what does the v stand for?)? Instead I would use rows
and columns
for the number of rows and columns and use i
and j
to iterate over them, because that is the convention used in mathematics when describing matrices.
Single responsibility principle
As for your actual code, you basically have four major responsibilities:
- Asking the user for input
- Creating a matrix
- Calculating the matrix product
- Printing a matrix scheme
I would make this into four functions:
def ask_user(message):
while True:
try:
return int(input(message))
except TypeError:
pass
This function will ask the user the message
until he inputs something that can be cast to an int
.
def matrix_from_user():
rows = ask_user("Number of rows?: ")
columns = ask_user("Number of columns?: ")
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = ask_user("M: ".format(i+1, j+1))
return matrix
Here I used str.format
to make the construction of the string easier.
def matrix_mul(matrix1, matrix2):
rows, columns = len(matrix1), len(matrix2[0])
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = sum(matrix1[i][k] * matrix2[k][j]
for k in range(len(matrix2)))
return matrix
This is a straightforward implementation of the formula $(AB)_ij = sum_k=1^m A_ik B_kj$ for two matrices $A$ and $B$. It uses the built-in sum
function that takes an iterable to sum. This iterable is a generator comprehension here.
def matrix_print(matrix):
print('nElements of matrix:')
rows, columns = len(matrix), len(matrix[0])
for i in range(rows):
row = ' '.join('M'.format(i + 1, j + 1) for j in range(columns))
print('|', row, '|')
Here I used str.join
to easily join an iterable of strings with a given delimiter (' '
here).
Your main program becomes then simply:
if __name__ == "__main__":
matrix1 = matrix_from_user()
matrix_print(matrix1)
matrix2 = matrix_from_user()
product = matrix_mul(matrix1, matrix2)
print('Matrix1: ', matrix1)
print('Matrix2: ', matrix2)
print('Result of Matrix Multiplication:', product)
This code I put under an if __name__ == "__main__":
guard which allows you to import this script from another script without this part of the code being run (so you get access to the functions).
add a comment |Â
up vote
3
down vote
accepted
up vote
3
down vote
accepted
I have basically two comments:
- Naming.
- Use functions to follow the single responsibility principle.
Naming
Python has an official style-guide, PEP8. Some of its recommendations are:
- Using
lower_case
for variables and functions,PascalCase
for classes andALL_CAPS
for global constants. - To limit your line length to 80 (or at the most 120) characters
Your names which you use to iterate through the rows and columns are not clear at all. Why vc
and vr
(I guess the c and r stand for column and row, but what does the v stand for?)? Instead I would use rows
and columns
for the number of rows and columns and use i
and j
to iterate over them, because that is the convention used in mathematics when describing matrices.
Single responsibility principle
As for your actual code, you basically have four major responsibilities:
- Asking the user for input
- Creating a matrix
- Calculating the matrix product
- Printing a matrix scheme
I would make this into four functions:
def ask_user(message):
while True:
try:
return int(input(message))
except TypeError:
pass
This function will ask the user the message
until he inputs something that can be cast to an int
.
def matrix_from_user():
rows = ask_user("Number of rows?: ")
columns = ask_user("Number of columns?: ")
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = ask_user("M: ".format(i+1, j+1))
return matrix
Here I used str.format
to make the construction of the string easier.
def matrix_mul(matrix1, matrix2):
rows, columns = len(matrix1), len(matrix2[0])
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = sum(matrix1[i][k] * matrix2[k][j]
for k in range(len(matrix2)))
return matrix
This is a straightforward implementation of the formula $(AB)_ij = sum_k=1^m A_ik B_kj$ for two matrices $A$ and $B$. It uses the built-in sum
function that takes an iterable to sum. This iterable is a generator comprehension here.
def matrix_print(matrix):
print('nElements of matrix:')
rows, columns = len(matrix), len(matrix[0])
for i in range(rows):
row = ' '.join('M'.format(i + 1, j + 1) for j in range(columns))
print('|', row, '|')
Here I used str.join
to easily join an iterable of strings with a given delimiter (' '
here).
Your main program becomes then simply:
if __name__ == "__main__":
matrix1 = matrix_from_user()
matrix_print(matrix1)
matrix2 = matrix_from_user()
product = matrix_mul(matrix1, matrix2)
print('Matrix1: ', matrix1)
print('Matrix2: ', matrix2)
print('Result of Matrix Multiplication:', product)
This code I put under an if __name__ == "__main__":
guard which allows you to import this script from another script without this part of the code being run (so you get access to the functions).
I have basically two comments:
- Naming.
- Use functions to follow the single responsibility principle.
Naming
Python has an official style-guide, PEP8. Some of its recommendations are:
- Using
lower_case
for variables and functions,PascalCase
for classes andALL_CAPS
for global constants. - To limit your line length to 80 (or at the most 120) characters
Your names which you use to iterate through the rows and columns are not clear at all. Why vc
and vr
(I guess the c and r stand for column and row, but what does the v stand for?)? Instead I would use rows
and columns
for the number of rows and columns and use i
and j
to iterate over them, because that is the convention used in mathematics when describing matrices.
Single responsibility principle
As for your actual code, you basically have four major responsibilities:
- Asking the user for input
- Creating a matrix
- Calculating the matrix product
- Printing a matrix scheme
I would make this into four functions:
def ask_user(message):
while True:
try:
return int(input(message))
except TypeError:
pass
This function will ask the user the message
until he inputs something that can be cast to an int
.
def matrix_from_user():
rows = ask_user("Number of rows?: ")
columns = ask_user("Number of columns?: ")
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = ask_user("M: ".format(i+1, j+1))
return matrix
Here I used str.format
to make the construction of the string easier.
def matrix_mul(matrix1, matrix2):
rows, columns = len(matrix1), len(matrix2[0])
matrix = [[0] * columns for _ in range(rows)]
for i in range(rows):
for j in range(columns):
matrix[i][j] = sum(matrix1[i][k] * matrix2[k][j]
for k in range(len(matrix2)))
return matrix
This is a straightforward implementation of the formula $(AB)_ij = sum_k=1^m A_ik B_kj$ for two matrices $A$ and $B$. It uses the built-in sum
function that takes an iterable to sum. This iterable is a generator comprehension here.
def matrix_print(matrix):
print('nElements of matrix:')
rows, columns = len(matrix), len(matrix[0])
for i in range(rows):
row = ' '.join('M'.format(i + 1, j + 1) for j in range(columns))
print('|', row, '|')
Here I used str.join
to easily join an iterable of strings with a given delimiter (' '
here).
Your main program becomes then simply:
if __name__ == "__main__":
matrix1 = matrix_from_user()
matrix_print(matrix1)
matrix2 = matrix_from_user()
product = matrix_mul(matrix1, matrix2)
print('Matrix1: ', matrix1)
print('Matrix2: ', matrix2)
print('Result of Matrix Multiplication:', product)
This code I put under an if __name__ == "__main__":
guard which allows you to import this script from another script without this part of the code being run (so you get access to the functions).
edited Jan 25 at 9:35
answered Jan 25 at 8:00
Graipher
20.5k43081
20.5k43081
add a comment |Â
add a comment |Â
up vote
2
down vote
For learning reason, you can create 2d array in one row like:
matrix2 = [[vc + v*10*vr for vc in range(0, v)] for vr in range(0, r)]
Since python is a funcational language, for learning reason, you can define a map/ reduce over matrix like:
def map(matrix, r,c, fn,summ):
for vr in range(0, r):
for vc in range(0, c):
fn(matrix[r][c],summ)
or with additional map-reduce over the row:
def map(matrix, r,c, fn,fnRow,startVal,summ):
for vr in range(0, r):
summRow = startVal
for vc in range(0, c):
fn(matrix[r][c],summRow)
fnRow(summRow, summ)
for learning reason you could implement Strassen matrix multiplication algorithm (google please), not naive one. I doubt you learn something like that.
For doing real project, use Numpy for initiations, not for high-performance operations.
add a comment |Â
up vote
2
down vote
For learning reason, you can create 2d array in one row like:
matrix2 = [[vc + v*10*vr for vc in range(0, v)] for vr in range(0, r)]
Since python is a funcational language, for learning reason, you can define a map/ reduce over matrix like:
def map(matrix, r,c, fn,summ):
for vr in range(0, r):
for vc in range(0, c):
fn(matrix[r][c],summ)
or with additional map-reduce over the row:
def map(matrix, r,c, fn,fnRow,startVal,summ):
for vr in range(0, r):
summRow = startVal
for vc in range(0, c):
fn(matrix[r][c],summRow)
fnRow(summRow, summ)
for learning reason you could implement Strassen matrix multiplication algorithm (google please), not naive one. I doubt you learn something like that.
For doing real project, use Numpy for initiations, not for high-performance operations.
add a comment |Â
up vote
2
down vote
up vote
2
down vote
For learning reason, you can create 2d array in one row like:
matrix2 = [[vc + v*10*vr for vc in range(0, v)] for vr in range(0, r)]
Since python is a funcational language, for learning reason, you can define a map/ reduce over matrix like:
def map(matrix, r,c, fn,summ):
for vr in range(0, r):
for vc in range(0, c):
fn(matrix[r][c],summ)
or with additional map-reduce over the row:
def map(matrix, r,c, fn,fnRow,startVal,summ):
for vr in range(0, r):
summRow = startVal
for vc in range(0, c):
fn(matrix[r][c],summRow)
fnRow(summRow, summ)
for learning reason you could implement Strassen matrix multiplication algorithm (google please), not naive one. I doubt you learn something like that.
For doing real project, use Numpy for initiations, not for high-performance operations.
For learning reason, you can create 2d array in one row like:
matrix2 = [[vc + v*10*vr for vc in range(0, v)] for vr in range(0, r)]
Since python is a funcational language, for learning reason, you can define a map/ reduce over matrix like:
def map(matrix, r,c, fn,summ):
for vr in range(0, r):
for vc in range(0, c):
fn(matrix[r][c],summ)
or with additional map-reduce over the row:
def map(matrix, r,c, fn,fnRow,startVal,summ):
for vr in range(0, r):
summRow = startVal
for vc in range(0, c):
fn(matrix[r][c],summRow)
fnRow(summRow, summ)
for learning reason you could implement Strassen matrix multiplication algorithm (google please), not naive one. I doubt you learn something like that.
For doing real project, use Numpy for initiations, not for high-performance operations.
edited Jan 25 at 0:32
answered Jan 25 at 0:24
user8426627
1343
1343
add a comment |Â
add a comment |Â
up vote
1
down vote
I suggest following matrix_mul
implementation
def matrix_mul(matrix1, matrix2):
from operator import mul
return [[sum(map(mul, row, col)) for col in zip(*matrix2)] for row in matrix1]
That is a nice and compact way of writing it. But I would put theimport
outside the function, it does cause some small overhead (even though it will only be imported once).
– Graipher
Jan 25 at 9:44
add a comment |Â
up vote
1
down vote
I suggest following matrix_mul
implementation
def matrix_mul(matrix1, matrix2):
from operator import mul
return [[sum(map(mul, row, col)) for col in zip(*matrix2)] for row in matrix1]
That is a nice and compact way of writing it. But I would put theimport
outside the function, it does cause some small overhead (even though it will only be imported once).
– Graipher
Jan 25 at 9:44
add a comment |Â
up vote
1
down vote
up vote
1
down vote
I suggest following matrix_mul
implementation
def matrix_mul(matrix1, matrix2):
from operator import mul
return [[sum(map(mul, row, col)) for col in zip(*matrix2)] for row in matrix1]
I suggest following matrix_mul
implementation
def matrix_mul(matrix1, matrix2):
from operator import mul
return [[sum(map(mul, row, col)) for col in zip(*matrix2)] for row in matrix1]
answered Jan 25 at 9:30
JulStrat
1135
1135
That is a nice and compact way of writing it. But I would put theimport
outside the function, it does cause some small overhead (even though it will only be imported once).
– Graipher
Jan 25 at 9:44
add a comment |Â
That is a nice and compact way of writing it. But I would put theimport
outside the function, it does cause some small overhead (even though it will only be imported once).
– Graipher
Jan 25 at 9:44
That is a nice and compact way of writing it. But I would put the
import
outside the function, it does cause some small overhead (even though it will only be imported once).– Graipher
Jan 25 at 9:44
That is a nice and compact way of writing it. But I would put the
import
outside the function, it does cause some small overhead (even though it will only be imported once).– Graipher
Jan 25 at 9:44
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f185916%2fmultiplication-of-any-two-matrices-using-lists-and-for-in-loop%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Don't use lists for numerical calculations if you don't have to. There are only some rare cases, where using lists in numerical calculations is feasable. So, a nice learning example would be to programm a matrix multiplication which beats numpy.dot in small matrices, but using ndim arrays provided by numpy...
– max9111
Feb 8 at 9:06