Find Simplest Sum
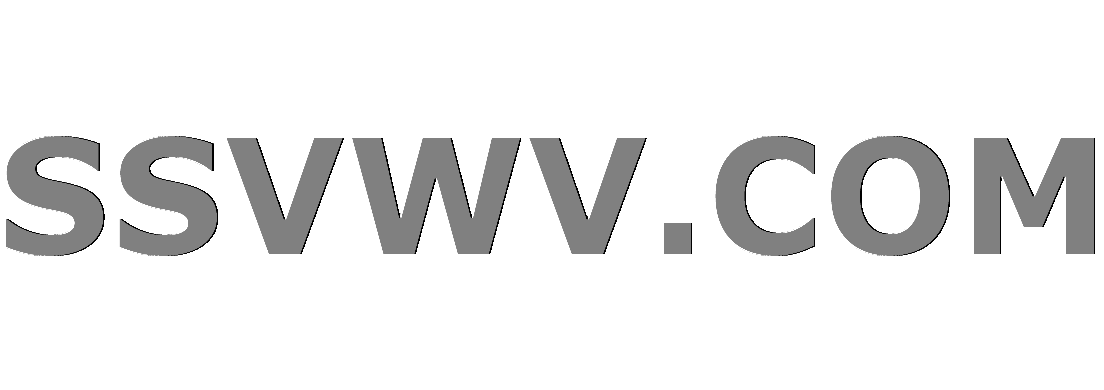
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
1
down vote
favorite
I was trying to solve one of the question. Could anyone please let me know how I can make this code much effective in terms of time and space complexity?
You are just learning to code and are finished with loops and
functions. Now, you are given the following pseudocode:/*
* The function has two integer parameters: k and n
* The function returns the value of sum
*/
function f(k, n)
sum = 0;
for (i = 1; i ≤ n; i += 1)
sum += i;
i *= k;
return sum;
For three given integers k,a, and b, find the value of S mod (10^9 +
7), where S is defined as:
$$s=sum_n=a^b▒〖f(k,n)$$
Input Format
The first line of the input is an integer Q, the total number of
queries. Each of the next Q lines contains three space separated
integers k,a,and b
Output Format
For each query, print the value of S mod (10^9 + 7) on a new line.
Sample Input
4
2 1 5
3 1 5
4 1 5
5 1 5
Sample Output
14
13
10
5
Explanation
Query 2 1 5
f(2,1) = 1
f(2,2) = 1
f(2,3) = 4
f(2,4) = 4
f(2,5) = 4
So, s = f(2,1)+f(2,2)+f(2,3)+f(2,4)+f(2,5) = 14
Query 3 1 5
f(3,1) = 1
f(3,2) = 1
f(3,3) = 1
f(3,4) = 5
f(3,5) = 5
So, s = f(3,1)+f(3,2)+f(3,3)+f(3,4)+f(3,5) = 13
Query 4 1 5
f(4,1) = 1
f(4,2) = 1
f(4,3) = 1
f(4,4) = 1
f(4,5) = 6
So, s = f(4,1)+f(4,2)+f(4,3)+f(4,4)+f(4,5) = 10
Query 5 1 5
f(5,1) = 1
f(5,2) = 1
f(5,3) = 1
f(5,4) = 1
f(5,5) = 1
So, s = f(5,1)+f(5,2)+f(5,3)+f(5,4)+f(5,5) = 5
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class maxSum
static int simplestSum(int k, int a, int b)
int count = 1;
int totalSum = 0;
while(count <= b)
int z = maxSum(k, a);
int mul = k*a;
if(mul < b)
totalSum = totalSum + (z*(k*a));
else
totalSum = totalSum + (z*((b-count)+1));
count = count + (k*a);
a = count;
return totalSum;
static int maxSum(int k , int a)
int sum = 0;
for (int i = 1; i <= a; i += 1)
sum += i;
i *= k;
return sum;
public static void main(String args)
Scanner in = new Scanner(System.in);
int q = in.nextInt();
for(int a0 = 0; a0 < q; a0++)
int k = in.nextInt();
int a = in.nextInt();
int b = in.nextInt();
int result = simplestSum(k, a, b);
System.out.println(result);
in.close();
java algorithm
add a comment |Â
up vote
1
down vote
favorite
I was trying to solve one of the question. Could anyone please let me know how I can make this code much effective in terms of time and space complexity?
You are just learning to code and are finished with loops and
functions. Now, you are given the following pseudocode:/*
* The function has two integer parameters: k and n
* The function returns the value of sum
*/
function f(k, n)
sum = 0;
for (i = 1; i ≤ n; i += 1)
sum += i;
i *= k;
return sum;
For three given integers k,a, and b, find the value of S mod (10^9 +
7), where S is defined as:
$$s=sum_n=a^b▒〖f(k,n)$$
Input Format
The first line of the input is an integer Q, the total number of
queries. Each of the next Q lines contains three space separated
integers k,a,and b
Output Format
For each query, print the value of S mod (10^9 + 7) on a new line.
Sample Input
4
2 1 5
3 1 5
4 1 5
5 1 5
Sample Output
14
13
10
5
Explanation
Query 2 1 5
f(2,1) = 1
f(2,2) = 1
f(2,3) = 4
f(2,4) = 4
f(2,5) = 4
So, s = f(2,1)+f(2,2)+f(2,3)+f(2,4)+f(2,5) = 14
Query 3 1 5
f(3,1) = 1
f(3,2) = 1
f(3,3) = 1
f(3,4) = 5
f(3,5) = 5
So, s = f(3,1)+f(3,2)+f(3,3)+f(3,4)+f(3,5) = 13
Query 4 1 5
f(4,1) = 1
f(4,2) = 1
f(4,3) = 1
f(4,4) = 1
f(4,5) = 6
So, s = f(4,1)+f(4,2)+f(4,3)+f(4,4)+f(4,5) = 10
Query 5 1 5
f(5,1) = 1
f(5,2) = 1
f(5,3) = 1
f(5,4) = 1
f(5,5) = 1
So, s = f(5,1)+f(5,2)+f(5,3)+f(5,4)+f(5,5) = 5
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class maxSum
static int simplestSum(int k, int a, int b)
int count = 1;
int totalSum = 0;
while(count <= b)
int z = maxSum(k, a);
int mul = k*a;
if(mul < b)
totalSum = totalSum + (z*(k*a));
else
totalSum = totalSum + (z*((b-count)+1));
count = count + (k*a);
a = count;
return totalSum;
static int maxSum(int k , int a)
int sum = 0;
for (int i = 1; i <= a; i += 1)
sum += i;
i *= k;
return sum;
public static void main(String args)
Scanner in = new Scanner(System.in);
int q = in.nextInt();
for(int a0 = 0; a0 < q; a0++)
int k = in.nextInt();
int a = in.nextInt();
int b = in.nextInt();
int result = simplestSum(k, a, b);
System.out.println(result);
in.close();
java algorithm
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24
add a comment |Â
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I was trying to solve one of the question. Could anyone please let me know how I can make this code much effective in terms of time and space complexity?
You are just learning to code and are finished with loops and
functions. Now, you are given the following pseudocode:/*
* The function has two integer parameters: k and n
* The function returns the value of sum
*/
function f(k, n)
sum = 0;
for (i = 1; i ≤ n; i += 1)
sum += i;
i *= k;
return sum;
For three given integers k,a, and b, find the value of S mod (10^9 +
7), where S is defined as:
$$s=sum_n=a^b▒〖f(k,n)$$
Input Format
The first line of the input is an integer Q, the total number of
queries. Each of the next Q lines contains three space separated
integers k,a,and b
Output Format
For each query, print the value of S mod (10^9 + 7) on a new line.
Sample Input
4
2 1 5
3 1 5
4 1 5
5 1 5
Sample Output
14
13
10
5
Explanation
Query 2 1 5
f(2,1) = 1
f(2,2) = 1
f(2,3) = 4
f(2,4) = 4
f(2,5) = 4
So, s = f(2,1)+f(2,2)+f(2,3)+f(2,4)+f(2,5) = 14
Query 3 1 5
f(3,1) = 1
f(3,2) = 1
f(3,3) = 1
f(3,4) = 5
f(3,5) = 5
So, s = f(3,1)+f(3,2)+f(3,3)+f(3,4)+f(3,5) = 13
Query 4 1 5
f(4,1) = 1
f(4,2) = 1
f(4,3) = 1
f(4,4) = 1
f(4,5) = 6
So, s = f(4,1)+f(4,2)+f(4,3)+f(4,4)+f(4,5) = 10
Query 5 1 5
f(5,1) = 1
f(5,2) = 1
f(5,3) = 1
f(5,4) = 1
f(5,5) = 1
So, s = f(5,1)+f(5,2)+f(5,3)+f(5,4)+f(5,5) = 5
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class maxSum
static int simplestSum(int k, int a, int b)
int count = 1;
int totalSum = 0;
while(count <= b)
int z = maxSum(k, a);
int mul = k*a;
if(mul < b)
totalSum = totalSum + (z*(k*a));
else
totalSum = totalSum + (z*((b-count)+1));
count = count + (k*a);
a = count;
return totalSum;
static int maxSum(int k , int a)
int sum = 0;
for (int i = 1; i <= a; i += 1)
sum += i;
i *= k;
return sum;
public static void main(String args)
Scanner in = new Scanner(System.in);
int q = in.nextInt();
for(int a0 = 0; a0 < q; a0++)
int k = in.nextInt();
int a = in.nextInt();
int b = in.nextInt();
int result = simplestSum(k, a, b);
System.out.println(result);
in.close();
java algorithm
I was trying to solve one of the question. Could anyone please let me know how I can make this code much effective in terms of time and space complexity?
You are just learning to code and are finished with loops and
functions. Now, you are given the following pseudocode:/*
* The function has two integer parameters: k and n
* The function returns the value of sum
*/
function f(k, n)
sum = 0;
for (i = 1; i ≤ n; i += 1)
sum += i;
i *= k;
return sum;
For three given integers k,a, and b, find the value of S mod (10^9 +
7), where S is defined as:
$$s=sum_n=a^b▒〖f(k,n)$$
Input Format
The first line of the input is an integer Q, the total number of
queries. Each of the next Q lines contains three space separated
integers k,a,and b
Output Format
For each query, print the value of S mod (10^9 + 7) on a new line.
Sample Input
4
2 1 5
3 1 5
4 1 5
5 1 5
Sample Output
14
13
10
5
Explanation
Query 2 1 5
f(2,1) = 1
f(2,2) = 1
f(2,3) = 4
f(2,4) = 4
f(2,5) = 4
So, s = f(2,1)+f(2,2)+f(2,3)+f(2,4)+f(2,5) = 14
Query 3 1 5
f(3,1) = 1
f(3,2) = 1
f(3,3) = 1
f(3,4) = 5
f(3,5) = 5
So, s = f(3,1)+f(3,2)+f(3,3)+f(3,4)+f(3,5) = 13
Query 4 1 5
f(4,1) = 1
f(4,2) = 1
f(4,3) = 1
f(4,4) = 1
f(4,5) = 6
So, s = f(4,1)+f(4,2)+f(4,3)+f(4,4)+f(4,5) = 10
Query 5 1 5
f(5,1) = 1
f(5,2) = 1
f(5,3) = 1
f(5,4) = 1
f(5,5) = 1
So, s = f(5,1)+f(5,2)+f(5,3)+f(5,4)+f(5,5) = 5
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class maxSum
static int simplestSum(int k, int a, int b)
int count = 1;
int totalSum = 0;
while(count <= b)
int z = maxSum(k, a);
int mul = k*a;
if(mul < b)
totalSum = totalSum + (z*(k*a));
else
totalSum = totalSum + (z*((b-count)+1));
count = count + (k*a);
a = count;
return totalSum;
static int maxSum(int k , int a)
int sum = 0;
for (int i = 1; i <= a; i += 1)
sum += i;
i *= k;
return sum;
public static void main(String args)
Scanner in = new Scanner(System.in);
int q = in.nextInt();
for(int a0 = 0; a0 < q; a0++)
int k = in.nextInt();
int a = in.nextInt();
int b = in.nextInt();
int result = simplestSum(k, a, b);
System.out.println(result);
in.close();
java algorithm
edited Jan 27 at 21:11


Jamal♦
30.1k11114225
30.1k11114225
asked Jan 27 at 20:12
vikash srivastava
112
112
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24
add a comment |Â
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
2
down vote
function f(k, n)
summ = 0
max = n*k;
for (i = 0; i < max; i += k)
summ += i
return sum;
or even simpler, using the formula of summ:
summ = k + 2k + ... + nk = (k + nk) + (2k + (n-1)k) +... = k*(n+1)*n/2
function f(k, n)
return (n+1)*n/2 *k;
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
function f(k, n)
summ = 0
max = n*k;
for (i = 0; i < max; i += k)
summ += i
return sum;
or even simpler, using the formula of summ:
summ = k + 2k + ... + nk = (k + nk) + (2k + (n-1)k) +... = k*(n+1)*n/2
function f(k, n)
return (n+1)*n/2 *k;
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
add a comment |Â
up vote
2
down vote
function f(k, n)
summ = 0
max = n*k;
for (i = 0; i < max; i += k)
summ += i
return sum;
or even simpler, using the formula of summ:
summ = k + 2k + ... + nk = (k + nk) + (2k + (n-1)k) +... = k*(n+1)*n/2
function f(k, n)
return (n+1)*n/2 *k;
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
add a comment |Â
up vote
2
down vote
up vote
2
down vote
function f(k, n)
summ = 0
max = n*k;
for (i = 0; i < max; i += k)
summ += i
return sum;
or even simpler, using the formula of summ:
summ = k + 2k + ... + nk = (k + nk) + (2k + (n-1)k) +... = k*(n+1)*n/2
function f(k, n)
return (n+1)*n/2 *k;
function f(k, n)
summ = 0
max = n*k;
for (i = 0; i < max; i += k)
summ += i
return sum;
or even simpler, using the formula of summ:
summ = k + 2k + ... + nk = (k + nk) + (2k + (n-1)k) +... = k*(n+1)*n/2
function f(k, n)
return (n+1)*n/2 *k;
answered Jan 28 at 0:04
user8426627
1343
1343
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
add a comment |Â
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
Hi user8426627, in case of f(2,3) expected output is 4 but using this formula it will be 12 . So , using this formula we are not getting the expected output .
– vikash srivastava
Jan 28 at 2:29
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
I find inconsistent use of semicolons and indentation irritating.
– greybeard
Jan 29 at 7:20
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f186154%2ffind-simplest-sum%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
(Welcome to CR!) It would be appropriate to name the origin of this problem statement and may be helpful to provide a link (there is a grey area in the formula?!). With a successfully designed "mathematical programming problem", no amount of cleverness in coding a brute force approach should be fast enough: you need a better algorithm.
– greybeard
Jan 28 at 23:07
@greybeard Please , find the link for problem statement hackerrank.com/contests/hackerrank-hiring-contest/challenges/… . I understand brute force approach is not the best one for this but i am not sure about other algorithm for this problem .
– vikash srivastava
Jan 29 at 4:24