Depth-First Search tree traversal in JavaScript
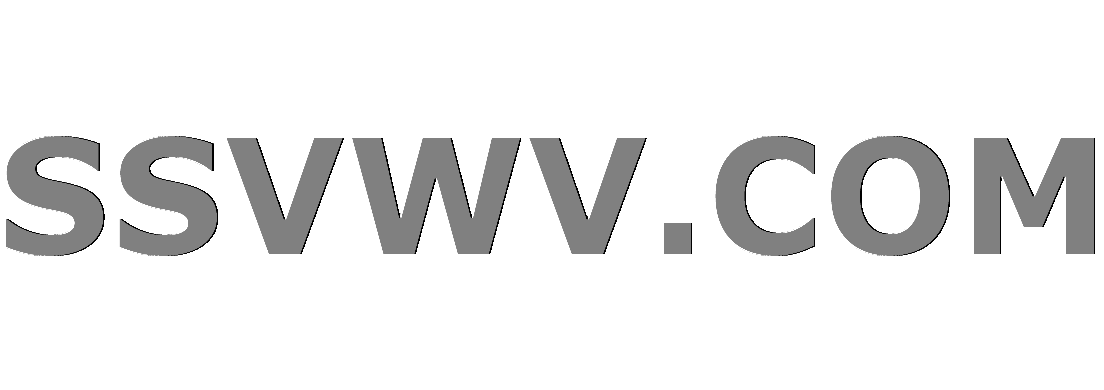
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
0
down vote
favorite
Given a graph and a starting vertex print the value of each vertex in a depth-first order.
I include the code for DFS iteratively by solving the starter problem of traversing and printing values below.
Pseudocode - While Loop and Stack
1. Create a stack to hold neighbor vertices and add the start vertex.
2. Create a set to track visited vertices and add the start vertex.
3. While stack is not empty
4. Dequeue from the queue and set to current.
5. Operate on current
6. Loop through all the vertex's neighbors.
7. For each neighbor, check if the vertex has been visited.
8. If not visited, then add it to the queue and add mark it as visited.
9. Once the stack is empty, we have completed the DFS
I look up some article and medium Resource can be found here:
Here's the code in JavaScript:
function graphDFS(graph, start)
let stack = new Stack();
let visited = new Set();
let current;
let neighbors;
stack.push(start);
visited.add(start);
while (stack.length > 0)
current = stack.pop();
console.log(current);
neighbors = graph.neighbors(current);
for (let neighbor of neighbors)
if (!visited.has(neighbor))
stack.push(neighbor);
visited.add(neighbor);
javascript depth-first-search
add a comment |Â
up vote
0
down vote
favorite
Given a graph and a starting vertex print the value of each vertex in a depth-first order.
I include the code for DFS iteratively by solving the starter problem of traversing and printing values below.
Pseudocode - While Loop and Stack
1. Create a stack to hold neighbor vertices and add the start vertex.
2. Create a set to track visited vertices and add the start vertex.
3. While stack is not empty
4. Dequeue from the queue and set to current.
5. Operate on current
6. Loop through all the vertex's neighbors.
7. For each neighbor, check if the vertex has been visited.
8. If not visited, then add it to the queue and add mark it as visited.
9. Once the stack is empty, we have completed the DFS
I look up some article and medium Resource can be found here:
Here's the code in JavaScript:
function graphDFS(graph, start)
let stack = new Stack();
let visited = new Set();
let current;
let neighbors;
stack.push(start);
visited.add(start);
while (stack.length > 0)
current = stack.pop();
console.log(current);
neighbors = graph.neighbors(current);
for (let neighbor of neighbors)
if (!visited.has(neighbor))
stack.push(neighbor);
visited.add(neighbor);
javascript depth-first-search
add a comment |Â
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Given a graph and a starting vertex print the value of each vertex in a depth-first order.
I include the code for DFS iteratively by solving the starter problem of traversing and printing values below.
Pseudocode - While Loop and Stack
1. Create a stack to hold neighbor vertices and add the start vertex.
2. Create a set to track visited vertices and add the start vertex.
3. While stack is not empty
4. Dequeue from the queue and set to current.
5. Operate on current
6. Loop through all the vertex's neighbors.
7. For each neighbor, check if the vertex has been visited.
8. If not visited, then add it to the queue and add mark it as visited.
9. Once the stack is empty, we have completed the DFS
I look up some article and medium Resource can be found here:
Here's the code in JavaScript:
function graphDFS(graph, start)
let stack = new Stack();
let visited = new Set();
let current;
let neighbors;
stack.push(start);
visited.add(start);
while (stack.length > 0)
current = stack.pop();
console.log(current);
neighbors = graph.neighbors(current);
for (let neighbor of neighbors)
if (!visited.has(neighbor))
stack.push(neighbor);
visited.add(neighbor);
javascript depth-first-search
Given a graph and a starting vertex print the value of each vertex in a depth-first order.
I include the code for DFS iteratively by solving the starter problem of traversing and printing values below.
Pseudocode - While Loop and Stack
1. Create a stack to hold neighbor vertices and add the start vertex.
2. Create a set to track visited vertices and add the start vertex.
3. While stack is not empty
4. Dequeue from the queue and set to current.
5. Operate on current
6. Loop through all the vertex's neighbors.
7. For each neighbor, check if the vertex has been visited.
8. If not visited, then add it to the queue and add mark it as visited.
9. Once the stack is empty, we have completed the DFS
I look up some article and medium Resource can be found here:
Here's the code in JavaScript:
function graphDFS(graph, start)
let stack = new Stack();
let visited = new Set();
let current;
let neighbors;
stack.push(start);
visited.add(start);
while (stack.length > 0)
current = stack.pop();
console.log(current);
neighbors = graph.neighbors(current);
for (let neighbor of neighbors)
if (!visited.has(neighbor))
stack.push(neighbor);
visited.add(neighbor);
javascript depth-first-search
edited Jul 31 at 4:16


Jamal♦
30.1k11114225
30.1k11114225
asked Jul 30 at 18:01
NinjaG
634221
634221
add a comment |Â
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f200610%2fdepth-first-search-tree-traversal-in-javascript%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password