Check of simple application in DDD architecture [on hold]
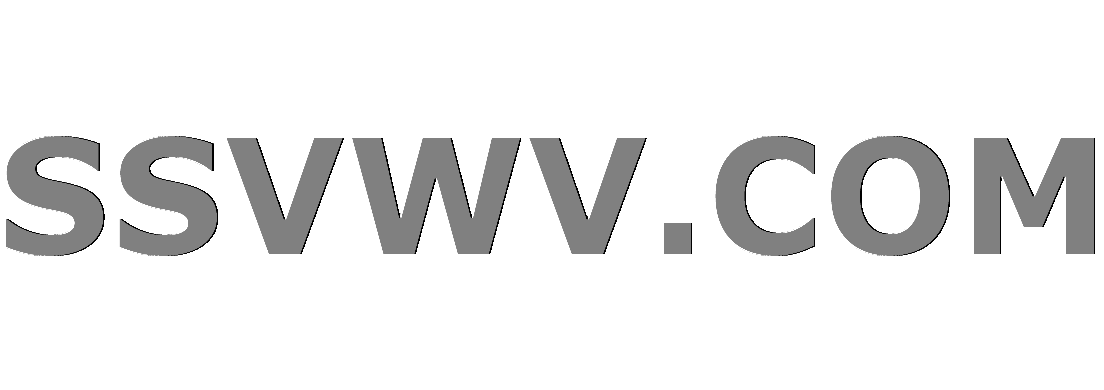
Multi tool use
Clash Royale CLAN TAG#URR8PPP
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty margin-bottom:0;
up vote
-2
down vote
favorite
I try learning about DDD architecture, I'm not sure if I correctly understand this idea.
I wrote some simple code in C#, maybe anybody can look for this, and give me some feedback, I would be very glad for any advice.
This is simple DDD without domain event, only Controler, DTO, Service and Domain with connect to DB using EF.
I don't know anybody who can tell me which is right.
Git repository
Can you tell me is this solution is good practise to write code like this:
Controller:
public class ItemController
private readonly IItemService _itemService;
public ItemController(IItemService itemService)
_itemService = itemService;
[HttpPost]
[Route("add")]
public ResponseDto<ItemDto> Add(ItemDto itemDto)
return _itemService.Add(itemDto);
[HttpGet]
[Route("getAll")]
public ResponseListDto<ItemDto> GetAll()
return _itemService.GetAll();
Service:
public class ItemService : IItemService
private readonly IRepository<Item> _repository;
private readonly IMapper _mapper;
public ItemService(IRepository<Item> repository, IMapper mapper)
_repository = repository;
_mapper = mapper;
public ResponseDto<ItemDto> Add(ItemDto newItems)
var response = new ResponseDto<ItemDto>();
try
var item = Item.Create(newItems.Name, newItems.Type, newItems.Price, newItems.Place);
_repository.Add(item);
_repository.Save();
newItems.Id = item.Id;
response.Result = newItems;
catch (Exception ex)
response.Error(ex);
return response;
public ResponseListDto<ItemDto> GetAll()
var response = new ResponseListDto<ItemDto>();
try
response.Result = _repository.GetAll()
.ToList()
.Select(x => _mapper.Map<Item, ItemDto>(x))
.ToList();
catch (Exception ex)
response.Error(ex);
return response;
Item class - DB model
public class Item : IEntity
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
public IEnumerable<Reservation> Reservations get; set;
public static Item Create(string name, int type, decimal price, string place)
Guard(name, type, price, place);
var newItem = new Item
Name = name,
Place = place,
Price = price,
Type = type
;
return newItem;
#region Private
private static void Guard(string name, int type, decimal price, string place)
if (string.IsNullOrEmpty(name))
throw new ArgumentNullException(nameof(name));
if (type < 0)
throw new ArgumentNullException(nameof(type));
if (price <= 0)
throw new ArgumentNullException(nameof(price));
if (string.IsNullOrEmpty(place))
throw new ArgumentNullException(nameof(place));
#endregion Private
ItemDto
public class ItemDto
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
c# ddd .net-core
put on hold as off-topic by t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight Aug 3 at 8:29
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight
add a comment |Â
up vote
-2
down vote
favorite
I try learning about DDD architecture, I'm not sure if I correctly understand this idea.
I wrote some simple code in C#, maybe anybody can look for this, and give me some feedback, I would be very glad for any advice.
This is simple DDD without domain event, only Controler, DTO, Service and Domain with connect to DB using EF.
I don't know anybody who can tell me which is right.
Git repository
Can you tell me is this solution is good practise to write code like this:
Controller:
public class ItemController
private readonly IItemService _itemService;
public ItemController(IItemService itemService)
_itemService = itemService;
[HttpPost]
[Route("add")]
public ResponseDto<ItemDto> Add(ItemDto itemDto)
return _itemService.Add(itemDto);
[HttpGet]
[Route("getAll")]
public ResponseListDto<ItemDto> GetAll()
return _itemService.GetAll();
Service:
public class ItemService : IItemService
private readonly IRepository<Item> _repository;
private readonly IMapper _mapper;
public ItemService(IRepository<Item> repository, IMapper mapper)
_repository = repository;
_mapper = mapper;
public ResponseDto<ItemDto> Add(ItemDto newItems)
var response = new ResponseDto<ItemDto>();
try
var item = Item.Create(newItems.Name, newItems.Type, newItems.Price, newItems.Place);
_repository.Add(item);
_repository.Save();
newItems.Id = item.Id;
response.Result = newItems;
catch (Exception ex)
response.Error(ex);
return response;
public ResponseListDto<ItemDto> GetAll()
var response = new ResponseListDto<ItemDto>();
try
response.Result = _repository.GetAll()
.ToList()
.Select(x => _mapper.Map<Item, ItemDto>(x))
.ToList();
catch (Exception ex)
response.Error(ex);
return response;
Item class - DB model
public class Item : IEntity
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
public IEnumerable<Reservation> Reservations get; set;
public static Item Create(string name, int type, decimal price, string place)
Guard(name, type, price, place);
var newItem = new Item
Name = name,
Place = place,
Price = price,
Type = type
;
return newItem;
#region Private
private static void Guard(string name, int type, decimal price, string place)
if (string.IsNullOrEmpty(name))
throw new ArgumentNullException(nameof(name));
if (type < 0)
throw new ArgumentNullException(nameof(type));
if (price <= 0)
throw new ArgumentNullException(nameof(price));
if (string.IsNullOrEmpty(place))
throw new ArgumentNullException(nameof(place));
#endregion Private
ItemDto
public class ItemDto
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
c# ddd .net-core
put on hold as off-topic by t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight Aug 3 at 8:29
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29
add a comment |Â
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I try learning about DDD architecture, I'm not sure if I correctly understand this idea.
I wrote some simple code in C#, maybe anybody can look for this, and give me some feedback, I would be very glad for any advice.
This is simple DDD without domain event, only Controler, DTO, Service and Domain with connect to DB using EF.
I don't know anybody who can tell me which is right.
Git repository
Can you tell me is this solution is good practise to write code like this:
Controller:
public class ItemController
private readonly IItemService _itemService;
public ItemController(IItemService itemService)
_itemService = itemService;
[HttpPost]
[Route("add")]
public ResponseDto<ItemDto> Add(ItemDto itemDto)
return _itemService.Add(itemDto);
[HttpGet]
[Route("getAll")]
public ResponseListDto<ItemDto> GetAll()
return _itemService.GetAll();
Service:
public class ItemService : IItemService
private readonly IRepository<Item> _repository;
private readonly IMapper _mapper;
public ItemService(IRepository<Item> repository, IMapper mapper)
_repository = repository;
_mapper = mapper;
public ResponseDto<ItemDto> Add(ItemDto newItems)
var response = new ResponseDto<ItemDto>();
try
var item = Item.Create(newItems.Name, newItems.Type, newItems.Price, newItems.Place);
_repository.Add(item);
_repository.Save();
newItems.Id = item.Id;
response.Result = newItems;
catch (Exception ex)
response.Error(ex);
return response;
public ResponseListDto<ItemDto> GetAll()
var response = new ResponseListDto<ItemDto>();
try
response.Result = _repository.GetAll()
.ToList()
.Select(x => _mapper.Map<Item, ItemDto>(x))
.ToList();
catch (Exception ex)
response.Error(ex);
return response;
Item class - DB model
public class Item : IEntity
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
public IEnumerable<Reservation> Reservations get; set;
public static Item Create(string name, int type, decimal price, string place)
Guard(name, type, price, place);
var newItem = new Item
Name = name,
Place = place,
Price = price,
Type = type
;
return newItem;
#region Private
private static void Guard(string name, int type, decimal price, string place)
if (string.IsNullOrEmpty(name))
throw new ArgumentNullException(nameof(name));
if (type < 0)
throw new ArgumentNullException(nameof(type));
if (price <= 0)
throw new ArgumentNullException(nameof(price));
if (string.IsNullOrEmpty(place))
throw new ArgumentNullException(nameof(place));
#endregion Private
ItemDto
public class ItemDto
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
c# ddd .net-core
I try learning about DDD architecture, I'm not sure if I correctly understand this idea.
I wrote some simple code in C#, maybe anybody can look for this, and give me some feedback, I would be very glad for any advice.
This is simple DDD without domain event, only Controler, DTO, Service and Domain with connect to DB using EF.
I don't know anybody who can tell me which is right.
Git repository
Can you tell me is this solution is good practise to write code like this:
Controller:
public class ItemController
private readonly IItemService _itemService;
public ItemController(IItemService itemService)
_itemService = itemService;
[HttpPost]
[Route("add")]
public ResponseDto<ItemDto> Add(ItemDto itemDto)
return _itemService.Add(itemDto);
[HttpGet]
[Route("getAll")]
public ResponseListDto<ItemDto> GetAll()
return _itemService.GetAll();
Service:
public class ItemService : IItemService
private readonly IRepository<Item> _repository;
private readonly IMapper _mapper;
public ItemService(IRepository<Item> repository, IMapper mapper)
_repository = repository;
_mapper = mapper;
public ResponseDto<ItemDto> Add(ItemDto newItems)
var response = new ResponseDto<ItemDto>();
try
var item = Item.Create(newItems.Name, newItems.Type, newItems.Price, newItems.Place);
_repository.Add(item);
_repository.Save();
newItems.Id = item.Id;
response.Result = newItems;
catch (Exception ex)
response.Error(ex);
return response;
public ResponseListDto<ItemDto> GetAll()
var response = new ResponseListDto<ItemDto>();
try
response.Result = _repository.GetAll()
.ToList()
.Select(x => _mapper.Map<Item, ItemDto>(x))
.ToList();
catch (Exception ex)
response.Error(ex);
return response;
Item class - DB model
public class Item : IEntity
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
public IEnumerable<Reservation> Reservations get; set;
public static Item Create(string name, int type, decimal price, string place)
Guard(name, type, price, place);
var newItem = new Item
Name = name,
Place = place,
Price = price,
Type = type
;
return newItem;
#region Private
private static void Guard(string name, int type, decimal price, string place)
if (string.IsNullOrEmpty(name))
throw new ArgumentNullException(nameof(name));
if (type < 0)
throw new ArgumentNullException(nameof(type));
if (price <= 0)
throw new ArgumentNullException(nameof(price));
if (string.IsNullOrEmpty(place))
throw new ArgumentNullException(nameof(place));
#endregion Private
ItemDto
public class ItemDto
public int Id get; set;
public string Name get; set;
public int Type get; set;
public decimal Price get; set;
public string Place get; set;
c# ddd .net-core
edited Aug 2 at 12:42
asked Aug 2 at 12:28
DawidP
373
373
put on hold as off-topic by t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight Aug 3 at 8:29
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight
put on hold as off-topic by t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight Aug 3 at 8:29
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – t3chb0t, yuri, Stephen Rauch, Sam Onela, Toby Speight
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29
add a comment |Â
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29
add a comment |Â
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
This feels like stub code, i.e. not "real" code, which would make it off-topic here.
– BCdotWEB
Aug 2 at 13:50
This is real code, I wrote simple application, where I won't learn DDD principal, I put their git repository. I wont only to someone told me if I good understand this principal, becouse I don't have anyone spec in work.
– DawidP
Aug 3 at 5:37
This question is incomplete. To help reviewers give you better answers, please add sufficient context to your question. The more you tell us about what your code does and what the purpose of doing that is, the easier it will be for reviewers to help you. The current title states your concerns about the code; it needs an edit to simply state the task; see How to get the best value out of Code Review: Asking Questions for guidance on writing good question titles.
– Toby Speight
Aug 3 at 8:29